19 Common Mistakes When Learning Your First Programming Language
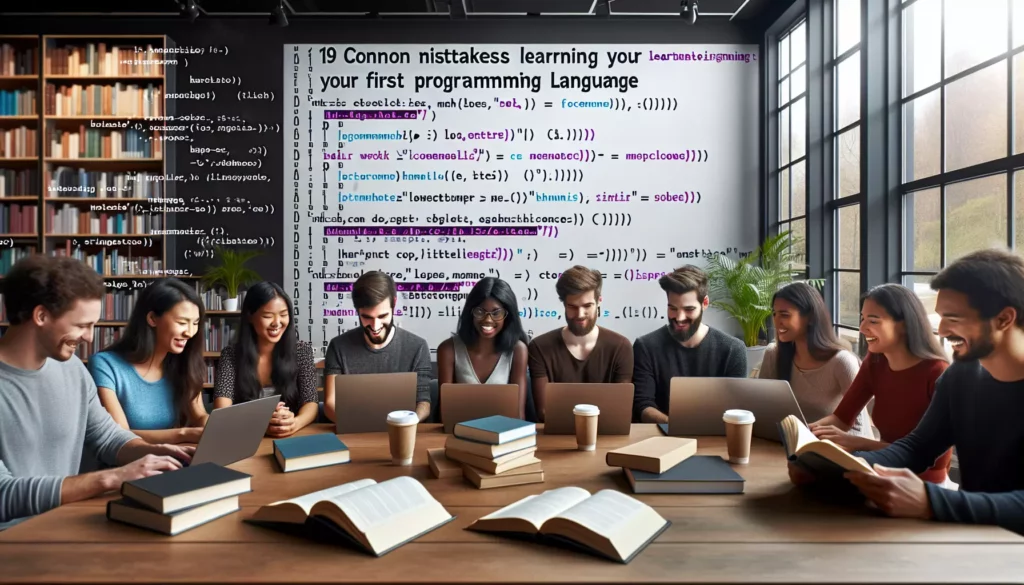
Learning your first programming language is an exciting journey, but it’s also filled with potential pitfalls. As you embark on this adventure, it’s essential to be aware of common mistakes that many beginners make. By understanding these errors, you can avoid them and accelerate your learning process. In this comprehensive guide, we’ll explore 19 common mistakes when learning your first programming language and provide practical tips to overcome them.
1. Choosing the Wrong Programming Language to Start With
One of the most crucial decisions you’ll make at the beginning of your coding journey is selecting your first programming language. Many beginners make the mistake of choosing a language based on popularity or perceived difficulty, rather than considering their goals and interests.
How to avoid this mistake:
- Assess your goals: Are you interested in web development, mobile apps, data science, or something else?
- Research language applications: Understand which languages are commonly used in your area of interest.
- Consider learning curves: Some languages, like Python, are known for being more beginner-friendly.
- Evaluate community support: Choose a language with a large, active community for better resources and help.
2. Trying to Learn Everything at Once
Programming is vast, and it’s easy to feel overwhelmed by the sheer amount of information available. Many beginners make the mistake of trying to learn multiple concepts, frameworks, or even languages simultaneously.
How to avoid this mistake:
- Focus on one language at a time: Master the basics before moving on to advanced topics or other languages.
- Create a structured learning plan: Break down your learning into manageable chunks.
- Practice regularly: Consistency is key in programming. Aim for daily practice, even if it’s just for 30 minutes.
- Build projects: Apply what you learn to real-world projects to reinforce your understanding.
3. Not Writing Code by Hand
In the age of IDEs and code editors with auto-completion, it’s tempting to rely solely on typing code on a computer. However, writing code by hand can be a valuable learning tool, especially for beginners.
How to avoid this mistake:
- Practice writing pseudocode: This helps you focus on logic rather than syntax.
- Solve coding problems on paper: This is particularly useful for interview preparation.
- Take handwritten notes: Writing key concepts by hand can improve retention.
- Use a whiteboard for planning: Sketch out your program structure before coding.
4. Neglecting to Read Documentation
Documentation is a programmer’s best friend, but many beginners overlook this valuable resource. They often rely on tutorials or Stack Overflow answers without consulting official documentation.
How to avoid this mistake:
- Make reading documentation a habit: Start with the official docs when learning a new concept or library.
- Practice navigating documentation: Learn how to quickly find the information you need.
- Contribute to documentation: As you gain experience, consider contributing to open-source documentation.
- Use documentation alongside other resources: Combine official docs with tutorials and community resources for a well-rounded understanding.
5. Copy-Pasting Code Without Understanding
It’s tempting to copy and paste code snippets from the internet when you’re stuck. While this can be a quick fix, it often leads to a lack of understanding and hinders long-term learning.
How to avoid this mistake:
- Analyze before copying: Take time to understand the code before using it.
- Type it out manually: Retyping code can help you internalize its structure and syntax.
- Modify and experiment: Try changing parts of the code to see how it affects the outcome.
- Comment your code: Explain what each part does in your own words.
6. Not Practicing Regularly
Consistency is key when learning to code. Many beginners make the mistake of studying intensively for short periods and then taking long breaks, which can lead to forgetting important concepts.
How to avoid this mistake:
- Set a daily coding goal: Even 30 minutes a day can make a significant difference.
- Use coding challenges: Platforms like LeetCode or HackerRank offer daily problems to solve.
- Join a coding community: Engage with others to stay motivated and accountable.
- Build a streak: Use apps or tools to track your daily coding habit.
7. Overlooking the Importance of Debugging Skills
Debugging is an essential skill for any programmer, but many beginners underestimate its importance. They often get frustrated when their code doesn’t work and give up too easily.
How to avoid this mistake:
- Learn to use debugging tools: Familiarize yourself with your IDE’s debugging features.
- Practice rubber duck debugging: Explain your code out loud to identify issues.
- Read error messages carefully: Don’t ignore error messages; they provide valuable information.
- Break down the problem: Isolate the issue by testing small parts of your code separately.
8. Not Writing Clean, Readable Code
When you’re just starting, it’s easy to focus solely on making your code work. However, writing clean, readable code is crucial for maintainability and collaboration.
How to avoid this mistake:
- Follow style guides: Learn and adhere to the style guide for your chosen language.
- Use meaningful variable names: Choose descriptive names that explain the purpose of your variables.
- Comment your code: Add comments to explain complex logic or the purpose of functions.
- Practice code refactoring: Regularly review and improve your code structure.
9. Ignoring Version Control
Version control systems like Git are essential tools in modern software development. Many beginners overlook the importance of learning version control early in their journey.
How to avoid this mistake:
- Start using Git early: Incorporate Git into your workflow from the beginning.
- Learn basic Git commands: Master essential commands like commit, push, pull, and branch.
- Use GitHub or similar platforms: Create repositories for your projects and collaborate with others.
- Practice branching and merging: Experiment with different Git workflows.
10. Not Seeking Help When Stuck
Programming can be challenging, and it’s common to get stuck. However, many beginners hesitate to ask for help, fearing they’ll look incompetent.
How to avoid this mistake:
- Join coding communities: Platforms like Stack Overflow or Reddit can be great resources.
- Attend coding meetups: Connect with other programmers in your local area.
- Find a mentor: Look for experienced programmers who can guide you.
- Ask specific questions: When seeking help, provide context and explain what you’ve already tried.
11. Neglecting to Learn Data Structures and Algorithms
While it’s possible to write code without a deep understanding of data structures and algorithms, this knowledge is crucial for writing efficient code and solving complex problems.
How to avoid this mistake:
- Study fundamental data structures: Learn about arrays, linked lists, stacks, queues, trees, and graphs.
- Practice implementing data structures: Try coding these structures from scratch.
- Learn basic algorithms: Study sorting, searching, and graph algorithms.
- Solve algorithm problems: Use platforms like AlgoCademy to practice algorithmic problem-solving.
12. Focusing Too Much on Syntax Instead of Logic
While syntax is important, many beginners get bogged down in memorizing every syntactical detail instead of focusing on the underlying logic and problem-solving aspects of programming.
How to avoid this mistake:
- Practice pseudocode: Write out your logic in plain language before coding.
- Focus on problem-solving: Spend time understanding the problem before jumping into code.
- Learn multiple languages: This helps you understand that logic is transferable across languages.
- Use flowcharts: Visualize your logic using flowcharts or diagrams.
13. Not Understanding Memory Management
Depending on your chosen language, memory management can be a critical concept. Many beginners overlook this, leading to inefficient code or memory leaks.
How to avoid this mistake:
- Learn about stack and heap: Understand how memory is allocated in your language.
- Study garbage collection: If your language uses it, understand how it works.
- Practice manual memory management: If applicable, learn how to allocate and deallocate memory.
- Use memory profiling tools: Learn to identify and fix memory leaks.
14. Overlooking Security Best Practices
Security is often an afterthought for beginners, but it’s crucial to develop secure coding habits from the start.
How to avoid this mistake:
- Learn about common vulnerabilities: Study concepts like SQL injection, XSS, and CSRF.
- Practice input validation: Always validate and sanitize user inputs.
- Use secure coding guidelines: Familiarize yourself with OWASP guidelines.
- Implement authentication and authorization: Learn how to secure your applications properly.
15. Not Writing Tests for Your Code
Testing is a fundamental part of software development, but many beginners skip this crucial step, leading to buggy and unreliable code.
How to avoid this mistake:
- Learn about unit testing: Understand the basics of writing and running unit tests.
- Practice Test-Driven Development (TDD): Try writing tests before implementing features.
- Use testing frameworks: Familiarize yourself with popular testing frameworks for your language.
- Automate your tests: Learn about continuous integration and how to automate your test suite.
16. Neglecting Soft Skills
Programming isn’t just about writing code. Soft skills like communication, teamwork, and problem-solving are equally important but often overlooked by beginners.
How to avoid this mistake:
- Practice explaining technical concepts: Try teaching what you’ve learned to others.
- Collaborate on projects: Work on open-source projects or team projects to improve collaboration skills.
- Develop your writing skills: Practice writing clear documentation and comments.
- Learn to give and receive code reviews: This improves both your code quality and communication skills.
17. Not Understanding Big O Notation
Big O notation is crucial for understanding algorithm efficiency, but many beginners find it intimidating and skip over it.
How to avoid this mistake:
- Start with the basics: Learn about time and space complexity.
- Analyze common algorithms: Understand the complexity of sorting and searching algorithms.
- Practice calculating Big O: Analyze your own code for efficiency.
- Use visualization tools: There are many online resources that help visualize algorithm efficiency.
18. Overengineering Solutions
As beginners gain knowledge, they often fall into the trap of overengineering solutions, making simple problems unnecessarily complex.
How to avoid this mistake:
- Start simple: Begin with the most straightforward solution that works.
- Refactor gradually: Improve your code incrementally rather than trying to perfect it from the start.
- Follow the YAGNI principle: “You Aren’t Gonna Need It” – don’t add functionality until it’s necessary.
- Seek feedback: Ask more experienced developers to review your code for unnecessary complexity.
19. Not Taking Breaks and Burning Out
Programming can be intense, and many beginners push themselves too hard, leading to burnout and diminished learning capacity.
How to avoid this mistake:
- Practice the Pomodoro Technique: Work in focused bursts with regular breaks.
- Set realistic goals: Don’t try to learn everything overnight.
- Maintain a healthy work-life balance: Make time for hobbies and relaxation.
- Stay physically active: Regular exercise can improve cognitive function and reduce stress.
Conclusion
Learning your first programming language is a challenging but rewarding journey. By being aware of these common mistakes and actively working to avoid them, you can accelerate your learning and become a more effective programmer. Remember, everyone makes mistakes – the key is to learn from them and keep improving.
As you continue your coding journey, consider using platforms like AlgoCademy to practice algorithmic thinking and problem-solving. With interactive coding tutorials, AI-powered assistance, and a focus on practical skills, AlgoCademy can help you progress from beginner-level coding to preparing for technical interviews at major tech companies.
Keep coding, stay curious, and don’t be afraid to make mistakes – they’re all part of the learning process!