The Importance of Learning to Debug in Your Chosen Language
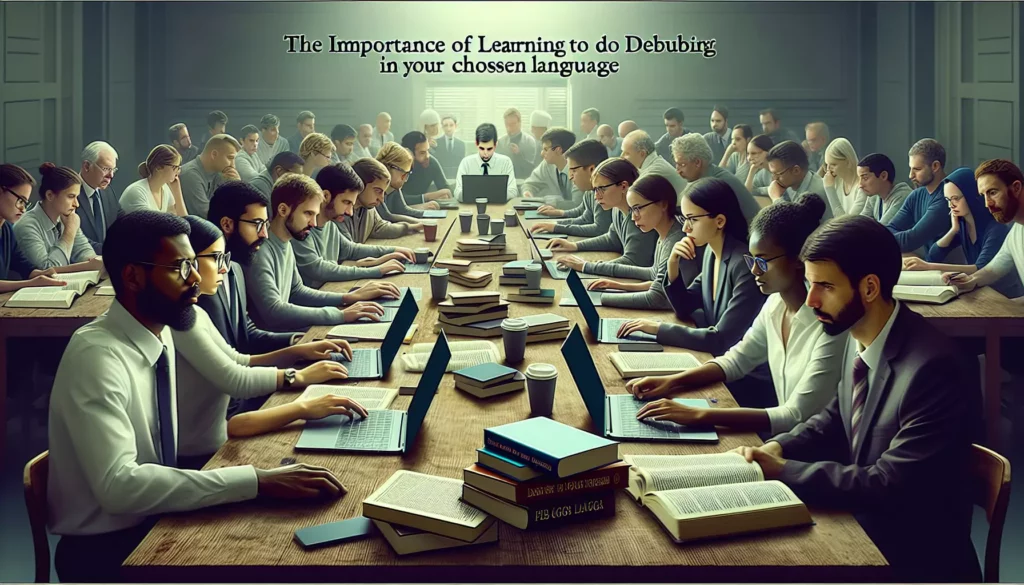
In the world of programming, writing code is only half the battle. The other crucial half is the ability to effectively debug your code when things don’t go as planned. Debugging is an essential skill that every programmer must master, regardless of their chosen programming language. In this comprehensive guide, we’ll explore why debugging is so important, how it relates to different programming languages, and provide practical tips to improve your debugging skills.
Why Debugging is Crucial for Programmers
Debugging is the process of identifying, analyzing, and fixing errors or “bugs” in your code. It’s a fundamental skill that separates novice programmers from experienced ones. Here’s why debugging is so important:
- Problem-solving: Debugging enhances your problem-solving abilities, teaching you to think critically and analytically about your code.
- Code quality: Through debugging, you learn to write cleaner, more efficient code as you understand common pitfalls and how to avoid them.
- Time-saving: Proficient debugging skills can significantly reduce the time spent on fixing issues, allowing you to be more productive.
- Understanding: Debugging deepens your understanding of how your chosen programming language works under the hood.
- Career advancement: Strong debugging skills are highly valued by employers and can lead to better job opportunities.
Debugging Across Different Programming Languages
While the core principles of debugging remain consistent across programming languages, each language has its own set of tools and techniques. Let’s explore debugging in some popular programming languages:
Python Debugging
Python offers several built-in debugging tools and techniques:
- print() statements: A simple yet effective way to output variable values and track program flow.
- pdb (Python Debugger): A powerful interactive debugger that allows you to step through your code line by line.
- logging module: For more advanced debugging and logging in larger applications.
- IDEs with integrated debuggers: PyCharm, Visual Studio Code, and others offer robust debugging features.
Example of using pdb in Python:
import pdb
def complex_function(x, y):
result = x + y
pdb.set_trace() # Debugger will pause here
return result * 2
print(complex_function(3, 4))
JavaScript Debugging
JavaScript debugging often involves browser developer tools and console methods:
- console.log(): The most common method for outputting debug information.
- debugger statement: Pauses execution and calls the debugging function.
- Browser Developer Tools: Offer powerful debugging capabilities, including breakpoints and step-through execution.
- Node.js debugging: For server-side JavaScript, tools like Node.js’s built-in debugger or VS Code’s debugger are useful.
Example of using the debugger statement in JavaScript:
function calculateTotal(price, quantity) {
let subtotal = price * quantity;
debugger; // Execution will pause here in browser dev tools
let tax = subtotal * 0.08;
return subtotal + tax;
}
console.log(calculateTotal(10, 5));
Java Debugging
Java offers robust debugging tools, especially when using IDEs:
- System.out.println(): Similar to Python’s print(), useful for quick debugging.
- IDE Debuggers: IDEs like IntelliJ IDEA and Eclipse provide powerful integrated debuggers.
- Java Debug Wire Protocol (JDWP): Allows debuggers to communicate with a JVM.
- Logging frameworks: Tools like Log4j for more sophisticated logging and debugging.
Example of using a breakpoint in Java (in an IDE):
public class DebugExample {
public static void main(String[] args) {
int x = 5;
int y = 10;
int result = multiply(x, y); // Set a breakpoint on this line
System.out.println("Result: " + result);
}
public static int multiply(int a, int b) {
return a * b;
}
}
Universal Debugging Techniques
Regardless of the programming language you’re using, there are several universal debugging techniques that can help you identify and fix issues more efficiently:
1. Reproduce the Bug
The first step in debugging is to consistently reproduce the bug. This involves:
- Identifying the exact steps that lead to the error
- Creating a minimal test case that demonstrates the issue
- Documenting the expected behavior versus the actual behavior
2. Divide and Conquer
Break down the problem into smaller parts:
- Use binary search to isolate the problematic section of code
- Comment out portions of code to identify where the issue occurs
- Test individual functions or methods in isolation
3. Use Debugging Tools
Leverage the debugging tools available in your programming environment:
- Set breakpoints to pause execution at specific lines
- Use step-through execution to analyze the program flow
- Inspect variable values and call stacks
- Utilize watch expressions to monitor specific variables or expressions
4. Log Strategically
Implement logging to track the program’s behavior:
- Use descriptive log messages to provide context
- Log input parameters, intermediate results, and final outputs
- Implement different log levels (e.g., DEBUG, INFO, ERROR) for better organization
5. Read Error Messages Carefully
Error messages often contain valuable information:
- Pay attention to line numbers and stack traces
- Research unfamiliar error messages online
- Look for patterns in recurring errors
Advanced Debugging Techniques
As you become more proficient in debugging, consider these advanced techniques:
1. Rubber Duck Debugging
This technique involves explaining your code line-by-line to an inanimate object (like a rubber duck). Often, the act of verbalization helps you spot the issue:
- Forces you to think through your logic step-by-step
- Helps identify assumptions you might have overlooked
- Can be done with a colleague instead of an object for added insight
2. Time Travel Debugging
Some advanced debugging tools allow you to step backwards through your code execution:
- Helps understand the sequence of events leading to a bug
- Useful for debugging race conditions and asynchronous code
- Available in tools like Visual Studio’s IntelliTrace and some JavaScript debuggers
3. Delta Debugging
This systematic approach helps isolate the exact change that introduced a bug:
- Useful when a bug appears after multiple changes
- Involves systematically bisecting changes until the problematic change is identified
- Can be automated with tools or done manually
4. Remote Debugging
For debugging applications running on remote servers or devices:
- Set up a debugging session over a network connection
- Useful for production issues that can’t be reproduced locally
- Requires careful setup to maintain security
Debugging Best Practices
To become a more effective debugger, consider adopting these best practices:
1. Write Testable Code
Design your code with debugging in mind:
- Write modular, loosely coupled code
- Implement unit tests to catch issues early
- Use dependency injection to make components easier to test in isolation
2. Use Version Control
Leverage version control systems like Git:
- Helps track changes over time
- Allows you to revert to a working state if needed
- Facilitates collaboration and code review, which can catch bugs early
3. Document Your Debugging Process
Keep a record of your debugging efforts:
- Document the steps you’ve taken to diagnose the problem
- Note any patterns or insights you’ve discovered
- Share your findings with your team to improve collective knowledge
4. Take Breaks
Debugging can be mentally taxing:
- Step away from difficult problems to gain a fresh perspective
- Use techniques like the Pomodoro method to maintain focus
- Collaborate with colleagues to get new ideas and approaches
Debugging in the Context of Technical Interviews
As you prepare for technical interviews, especially for major tech companies, keep in mind that debugging skills are often assessed:
- Code reviews: You may be asked to review and debug existing code.
- Live coding: Interviewers often introduce bugs intentionally to see how you approach debugging.
- System design: Debugging extends to system-level issues in distributed systems.
- Problem-solving: Your approach to debugging reflects your overall problem-solving skills.
To prepare for debugging scenarios in interviews:
- Practice debugging unfamiliar code
- Learn to articulate your thought process while debugging
- Familiarize yourself with common bug patterns in your chosen language
- Study debugging techniques for distributed systems and microservices architectures
Conclusion
Learning to debug effectively in your chosen programming language is a critical skill that will serve you throughout your career as a developer. It’s not just about fixing errors; it’s about understanding systems, improving code quality, and becoming a more efficient problem solver.
Remember that debugging is a skill that improves with practice. Don’t be discouraged by challenging bugs – each one is an opportunity to learn and grow as a programmer. Embrace the detective work involved in debugging, and you’ll find that it can be one of the most rewarding aspects of programming.
As you continue your journey with AlgoCademy, focus not just on writing code, but on honing your debugging skills. This will not only prepare you for technical interviews but also make you a more valuable and proficient developer in any role you pursue.