11 Essential Operators Every Beginner Programmer Should Know
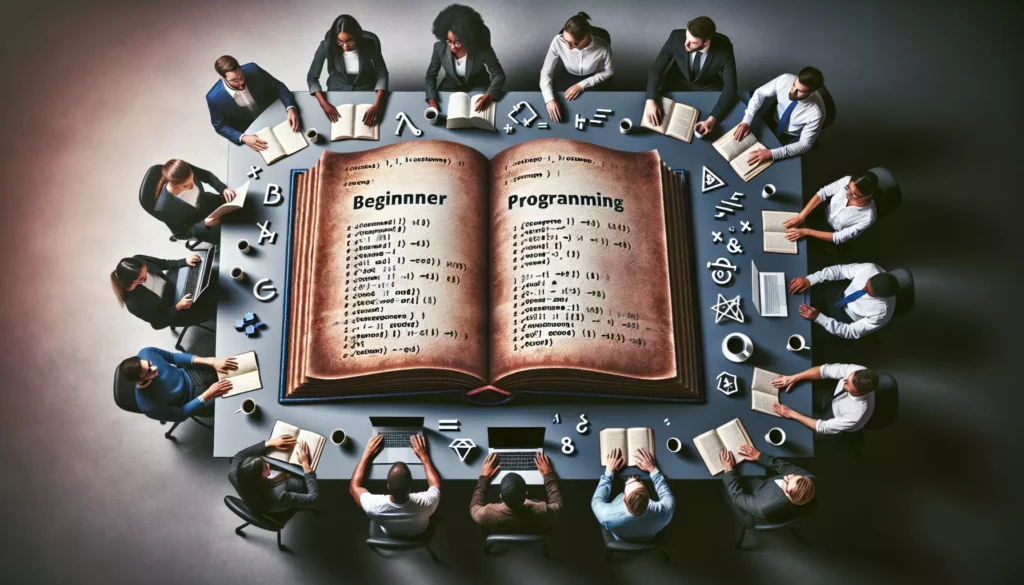
As a beginner programmer, understanding operators is crucial for writing effective and efficient code. Operators are symbols or keywords that perform specific operations on one or more operands. They are the building blocks of any programming language and play a vital role in manipulating data, performing calculations, and controlling program flow. In this comprehensive guide, we’ll explore 11 essential operators that every beginner programmer should know, regardless of the programming language they’re learning.
1. Assignment Operator (=)
The assignment operator is one of the most basic and frequently used operators in programming. It assigns a value to a variable. In most programming languages, the equal sign (=) is used for assignment.
x = 5;
name = "John";
isActive = true;
In these examples, we’re assigning the value 5 to the variable x, the string “John” to the variable name, and the boolean value true to the variable isActive.
2. Arithmetic Operators (+, -, *, /, %)
Arithmetic operators are used to perform mathematical operations on numerical values. The five most common arithmetic operators are:
- Addition (+)
- Subtraction (-)
- Multiplication (*)
- Division (/)
- Modulus (%)
a = 10;
b = 3;
sum = a + b; // 13
difference = a - b; // 7
product = a * b; // 30
quotient = a / b; // 3.33 (in languages with floating-point division)
remainder = a % b; // 1
The modulus operator (%) returns the remainder of a division operation and is particularly useful for determining if a number is even or odd, or for cycling through a range of values.
3. Comparison Operators (==, !=, <, >, <=, >=)
Comparison operators are used to compare two values and return a boolean result (true or false). These operators are essential for creating conditional statements and loops. The six main comparison operators are:
- Equal to (==)
- Not equal to (!=)
- Less than (<)
- Greater than (>)
- Less than or equal to (<=)
- Greater than or equal to (>=)
x = 5;
y = 10;
isEqual = x == y; // false
isNotEqual = x != y; // true
isLess = x < y; // true
isGreater = x > y; // false
isLessOrEqual = x <= y; // true
isGreaterOrEqual = x >= y; // false
It’s important to note that in many programming languages, the double equals sign (==) is used for comparison, while the single equals sign (=) is used for assignment.
4. Logical Operators (AND, OR, NOT)
Logical operators are used to combine multiple conditions and evaluate them to a single boolean result. The three main logical operators are:
- AND (usually represented as && or AND)
- OR (usually represented as || or OR)
- NOT (usually represented as ! or NOT)
a = true;
b = false;
andResult = a && b; // false
orResult = a || b; // true
notResult = !a; // false
These operators are crucial for creating complex conditions in if statements, while loops, and other control structures.
5. Increment and Decrement Operators (++, –)
Increment (++) and decrement (–) operators are used to increase or decrease the value of a variable by 1. These operators come in two forms: prefix and postfix.
x = 5;
// Prefix increment
++x; // x is now 6
// Postfix increment
x++; // x is now 7
// Prefix decrement
--x; // x is now 6
// Postfix decrement
x--; // x is now 5
The difference between prefix and postfix forms becomes important when these operators are used in expressions. With prefix form, the variable is incremented or decremented before its value is used in the expression. With postfix form, the original value is used in the expression, and then the variable is incremented or decremented.
6. Compound Assignment Operators (+=, -=, *=, /=, %=)
Compound assignment operators combine an arithmetic operation with assignment. They provide a shorthand way to update a variable based on its current value.
x = 10;
x += 5; // Equivalent to: x = x + 5; (x is now 15)
x -= 3; // Equivalent to: x = x - 3; (x is now 12)
x *= 2; // Equivalent to: x = x * 2; (x is now 24)
x /= 4; // Equivalent to: x = x / 4; (x is now 6)
x %= 4; // Equivalent to: x = x % 4; (x is now 2)
These operators are particularly useful when you need to update a variable based on its current value, as they make the code more concise and readable.
7. Bitwise Operators (&, |, ^, ~, <<, >>)
Bitwise operators perform operations on the individual bits of integer values. While less commonly used in high-level programming, understanding bitwise operators can be crucial for certain types of low-level programming, optimization, and working with flags or permissions. The main bitwise operators are:
- AND (&)
- OR (|)
- XOR (^)
- NOT (~)
- Left shift (<<)
- Right shift (>>)
a = 5; // Binary: 0101
b = 3; // Binary: 0011
andResult = a & b; // 1 (Binary: 0001)
orResult = a | b; // 7 (Binary: 0111)
xorResult = a ^ b; // 6 (Binary: 0110)
notResult = ~a; // -6 (Binary: 1010 in two's complement)
leftShift = a << 1; // 10 (Binary: 1010)
rightShift = a >> 1; // 2 (Binary: 0010)
Bitwise operators are often used for tasks like setting or clearing individual bits in a byte, performing fast multiplication or division by powers of 2, or working with binary flags.
8. Ternary Operator (?:)
The ternary operator, also known as the conditional operator, provides a concise way to write an if-else statement in a single line. It takes three operands: a condition, a value to return if the condition is true, and a value to return if the condition is false.
condition ? valueIfTrue : valueIfFalse
Here’s an example of how to use the ternary operator:
age = 20;
status = (age >= 18) ? "Adult" : "Minor";
// status will be "Adult"
While the ternary operator can make code more concise, it’s important to use it judiciously. For complex conditions or when readability might be compromised, it’s often better to use a traditional if-else statement.
9. String Concatenation Operator (+)
In many programming languages, the plus sign (+) is overloaded to serve as both an arithmetic addition operator and a string concatenation operator. When used with strings, it combines two or more strings into a single string.
firstName = "John";
lastName = "Doe";
fullName = firstName + " " + lastName;
// fullName will be "John Doe"
Some languages, like Python, also allow string concatenation using the += compound assignment operator:
greeting = "Hello, ";
greeting += "world!";
// greeting will be "Hello, world!"
It’s worth noting that while string concatenation with the + operator is common and intuitive, it may not always be the most efficient method, especially when dealing with many strings or in performance-critical code. In such cases, many languages offer more efficient alternatives like StringBuilder in Java or join() method in Python.
10. Type Casting Operators
Type casting operators are used to convert a value from one data type to another. The exact syntax and behavior of type casting can vary between programming languages, but the concept is universal. There are two main types of casting:
- Implicit casting (automatic type conversion)
- Explicit casting (manual type conversion)
Implicit casting occurs automatically when you assign a value of one type to a variable of another type, and no data will be lost in the conversion. For example:
int x = 5;
double y = x; // Implicit casting from int to double
Explicit casting is when you manually convert a value from one type to another. This is necessary when there’s a risk of losing data or when you want to override the default behavior. The syntax for explicit casting varies by language, but often involves putting the desired type in parentheses before the value to be converted:
double x = 5.7;
int y = (int)x; // Explicit casting from double to int
// y will be 5, losing the decimal part
In some languages, like Python, you can use functions for type casting:
x = 5.7
y = int(x) # y will be 5
Understanding type casting is crucial for working with different data types and ensuring that your operations produce the expected results.
11. Instanceof Operator
The instanceof operator is used in object-oriented programming languages to test whether an object is an instance of a specific class or interface. While the exact syntax may vary between languages, the concept is similar across many object-oriented languages.
In Java, for example, the instanceof operator is used like this:
String str = "Hello";
boolean result = str instanceof String; // true
In Python, you would use the isinstance() function to achieve the same result:
str = "Hello"
result = isinstance(str, str) # True
The instanceof operator (or its equivalent) is particularly useful when working with inheritance hierarchies or when you need to perform different operations based on the specific type of an object.
Conclusion
Understanding these 11 essential operators is crucial for any beginner programmer. They form the foundation of most programming tasks, from simple calculations to complex logical operations and type manipulations. As you progress in your programming journey, you’ll find yourself using these operators frequently, often in combination with each other.
Remember that while the concepts behind these operators are universal, the exact syntax and behavior can vary between programming languages. Always refer to the documentation of the specific language you’re working with to understand the nuances of how these operators are implemented.
Practice using these operators in various scenarios to become comfortable with them. Try combining different operators to create more complex expressions, and pay attention to operator precedence (the order in which operators are evaluated in an expression). With time and practice, using these operators will become second nature, allowing you to write more efficient and expressive code.
As you advance in your programming skills, you’ll encounter more specialized operators and learn how to overload operators in object-oriented programming. But mastering these 11 essential operators will give you a solid foundation for tackling more advanced programming concepts and challenges.
Happy coding!