Learning to Think Like a Programmer: A Comprehensive Guide
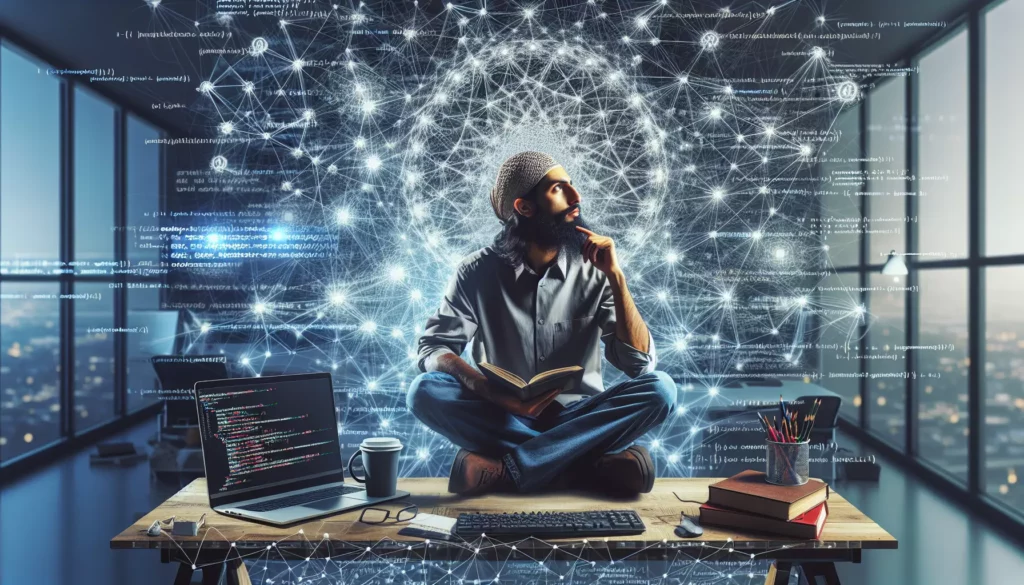
In today’s digital age, programming skills have become increasingly valuable across various industries. Whether you’re aspiring to work for a major tech company or simply want to enhance your problem-solving abilities, learning to think like a programmer is a crucial step in your journey. This comprehensive guide will explore the mindset, strategies, and practices that can help you develop the cognitive skills necessary for successful programming.
Understanding the Programmer’s Mindset
Before diving into specific techniques, it’s essential to understand what it means to “think like a programmer.” At its core, programming is about problem-solving. Programmers approach challenges with a unique blend of analytical thinking, creativity, and systematic reasoning. Here are some key aspects of the programmer’s mindset:
- Logical Thinking: Breaking down complex problems into smaller, manageable parts.
- Abstraction: Identifying patterns and creating generalizable solutions.
- Attention to Detail: Recognizing that even small errors can have significant impacts.
- Persistence: Embracing challenges and viewing errors as learning opportunities.
- Continuous Learning: Staying updated with new technologies and approaches.
Developing Problem-Solving Skills
Problem-solving is at the heart of programming. To enhance your problem-solving skills, consider the following strategies:
1. Break Down Problems
When faced with a complex problem, break it down into smaller, more manageable sub-problems. This approach, known as “divide and conquer,” allows you to focus on solving one piece at a time, making the overall task less daunting.
2. Plan Before Coding
Resist the urge to start coding immediately. Instead, take time to plan your approach. Sketch out your solution, create flowcharts, or write pseudocode. This planning phase can help you identify potential issues and streamline your coding process.
3. Practice Algorithmic Thinking
Algorithmic thinking involves creating step-by-step procedures to solve problems efficiently. Practice designing algorithms for everyday tasks, such as making a sandwich or sorting a deck of cards. This exercise will help you develop a structured approach to problem-solving.
4. Learn from Others
Study how experienced programmers approach problems. Platforms like AlgoCademy offer interactive tutorials and resources that can expose you to various problem-solving techniques used by professionals in the field.
Mastering Fundamental Programming Concepts
To think like a programmer, you need a solid understanding of fundamental programming concepts. Here are some key areas to focus on:
1. Data Structures
Data structures are the building blocks of efficient programs. Familiarize yourself with common data structures such as arrays, linked lists, stacks, queues, trees, and graphs. Understanding when and how to use these structures is crucial for solving complex problems.
2. Algorithms
Learn and practice implementing various algorithms, including sorting algorithms (e.g., bubble sort, merge sort, quicksort), search algorithms (e.g., binary search), and graph algorithms (e.g., breadth-first search, depth-first search). Understanding these algorithms will help you approach problems more systematically.
3. Time and Space Complexity
Develop an understanding of algorithmic efficiency by studying time and space complexity. This knowledge will help you write more optimized code and make informed decisions about which algorithms to use in different scenarios.
4. Object-Oriented Programming (OOP)
Grasp the principles of OOP, including encapsulation, inheritance, and polymorphism. These concepts are fundamental to many modern programming languages and can help you design more modular and maintainable code.
Cultivating Coding Best Practices
Thinking like a programmer also involves adopting best practices that lead to clean, efficient, and maintainable code. Consider the following practices:
1. Write Clean and Readable Code
Strive for clarity and simplicity in your code. Use meaningful variable names, add comments to explain complex logic, and follow consistent formatting guidelines. Remember, code is read more often than it is written, so prioritize readability.
2. Practice Modular Programming
Break your code into smaller, reusable functions or modules. This approach makes your code easier to understand, test, and maintain. It also promotes code reuse, reducing redundancy in your projects.
3. Implement Error Handling
Anticipate and handle potential errors in your code. Implement proper error handling and logging mechanisms to make your programs more robust and easier to debug.
4. Version Control
Learn to use version control systems like Git. This skill is essential for collaborating with others, tracking changes in your code, and managing different versions of your projects.
Enhancing Your Logical Thinking
Logical thinking is a cornerstone of programming. Here are some ways to sharpen your logical thinking skills:
1. Solve Puzzles and Brain Teasers
Engage in activities that challenge your logical reasoning, such as solving Sudoku puzzles, playing chess, or tackling logic problems. These exercises can improve your ability to think systematically and identify patterns.
2. Practice Debugging
Debugging is an essential skill for programmers. When you encounter errors in your code, approach them methodically. Analyze the problem, form hypotheses about the cause, and test your assumptions. This process will strengthen your logical thinking and problem-solving abilities.
3. Engage in Code Reviews
Participate in code reviews, either by reviewing others’ code or having your code reviewed. This practice exposes you to different approaches and helps you develop a critical eye for code quality and logical consistency.
4. Explore Different Programming Paradigms
Familiarize yourself with various programming paradigms, such as imperative, functional, and declarative programming. Each paradigm offers a different way of thinking about and structuring code, broadening your problem-solving toolkit.
Developing a Growth Mindset
A growth mindset is crucial for success in programming. Embrace the following attitudes:
1. Embrace Challenges
View difficult problems as opportunities for growth rather than insurmountable obstacles. Remember that every challenge you overcome makes you a better programmer.
2. Learn from Failures
Don’t be discouraged by errors or failed attempts. Instead, analyze what went wrong and use that knowledge to improve your approach in the future.
3. Seek Feedback
Actively seek feedback on your code and problem-solving approaches. Be open to constructive criticism and use it as a tool for improvement.
4. Stay Curious
Cultivate a sense of curiosity about new technologies, programming languages, and problem-solving techniques. The field of programming is constantly evolving, and staying curious will help you adapt and grow.
Practical Exercises to Think Like a Programmer
To reinforce the concepts and practices discussed, here are some practical exercises you can try:
1. Implement a Classic Algorithm
Choose a classic algorithm, such as binary search or bubble sort, and implement it from scratch. Focus on understanding the logic behind the algorithm and optimizing your implementation.
2. Solve Coding Challenges
Participate in coding challenges on platforms like AlgoCademy, LeetCode, or HackerRank. These platforms offer a wide range of problems that can help you apply your skills and learn new techniques.
3. Build a Small Project
Develop a small project from start to finish. This could be a simple game, a utility application, or a web scraper. The process of planning, implementing, and debugging a complete project will give you valuable experience in thinking like a programmer.
4. Refactor Existing Code
Take an existing piece of code (either your own or an open-source project) and refactor it to improve its efficiency, readability, or modularity. This exercise will help you practice critical thinking and code optimization skills.
Leveraging Tools and Resources
To support your journey in thinking like a programmer, take advantage of various tools and resources:
1. Integrated Development Environments (IDEs)
Use modern IDEs that offer features like code completion, debugging tools, and performance profiling. These tools can help you write better code and understand how your programs execute.
2. Online Learning Platforms
Utilize platforms like AlgoCademy that offer structured learning paths, interactive coding exercises, and AI-powered assistance. These resources can guide you through the process of developing your programming skills and preparing for technical interviews.
3. Programming Communities
Join programming communities and forums where you can ask questions, share knowledge, and collaborate with other learners and experienced programmers. Engaging with a community can provide valuable insights and support.
4. Code Visualization Tools
Explore tools that help visualize code execution and data structures. These can be particularly helpful in understanding complex algorithms and data manipulations.
Preparing for Technical Interviews
As you develop your ability to think like a programmer, you may be interested in preparing for technical interviews, particularly for major tech companies. Here are some tips to help you succeed:
1. Study Data Structures and Algorithms
Focus on mastering common data structures and algorithms, as these are frequently tested in technical interviews. Practice implementing them from scratch and solving problems that utilize these concepts.
2. Practice Whiteboard Coding
Many technical interviews involve coding on a whiteboard or in a simple text editor. Practice solving problems without the assistance of an IDE to simulate interview conditions.
3. Develop Your Problem-Solving Process
When approaching interview questions, develop a systematic process:
1. Clarify the problem and requirements
2. Discuss potential approaches
3. Choose an approach and explain your reasoning
4. Write pseudocode or outline your solution
5. Implement the solution
6. Test and optimize your code
4. Mock Interviews
Conduct mock interviews with peers or use platforms that offer simulated interview experiences. This practice will help you become more comfortable with the interview process and improve your ability to communicate your thoughts effectively.
Conclusion
Learning to think like a programmer is a journey that requires dedication, practice, and continuous learning. By developing problem-solving skills, mastering fundamental concepts, adopting best practices, and cultivating a growth mindset, you can enhance your ability to approach programming challenges effectively. Remember that becoming a proficient programmer takes time, so be patient with yourself and enjoy the process of growth and discovery.
As you continue your journey, leverage resources like AlgoCademy to guide your learning, practice your skills, and prepare for the challenges ahead. With persistence and the right approach, you can develop the cognitive skills necessary to excel in programming and tackle complex problems with confidence.
Happy coding, and may your journey in thinking like a programmer be both rewarding and enlightening!