Leveraging Past Experience: How to Bring Up Relevant Projects and Skills in a Coding Interview
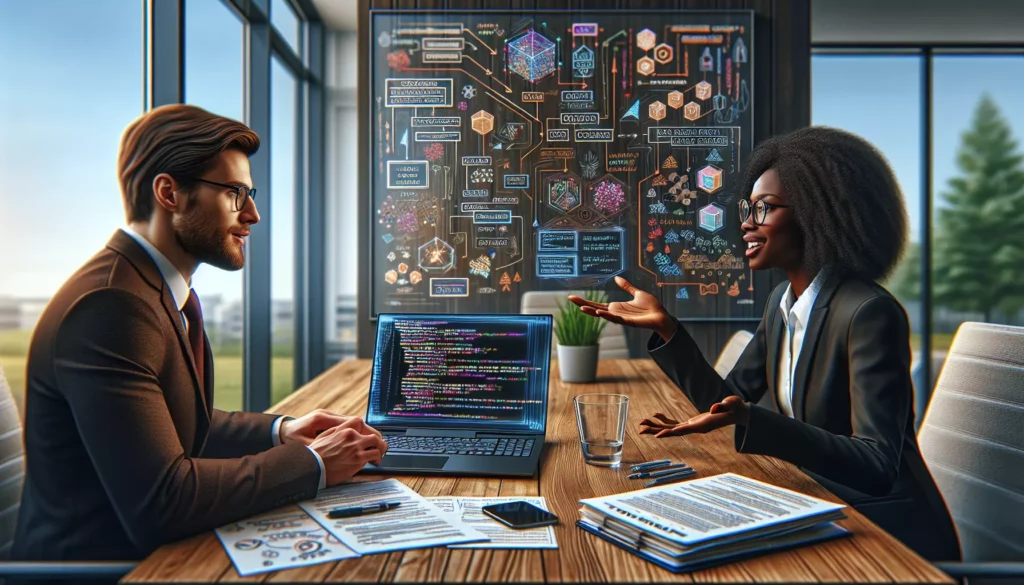
Coding interviews can be nerve-wracking experiences, especially when you’re faced with complex problems and algorithms. However, one of the most powerful tools at your disposal is often overlooked: your past experience. By effectively leveraging your previous projects and skills during an interview, you can demonstrate your problem-solving abilities, showcase your technical expertise, and stand out from other candidates. In this comprehensive guide, we’ll explore strategies for weaving your past experiences into coding interviews, helping you make a lasting impression on potential employers.
1. Understanding the Importance of Past Experience in Coding Interviews
Before diving into specific strategies, it’s crucial to understand why bringing up your past experience is so valuable in a coding interview:
- Demonstrates real-world application: Theoretical knowledge is important, but employers want to see how you’ve applied your skills in practical scenarios.
- Showcases problem-solving abilities: Past projects often involve overcoming challenges, which can highlight your problem-solving approach.
- Illustrates teamwork and communication skills: Many projects involve collaboration, allowing you to showcase your ability to work in a team.
- Provides context for your skills: It’s one thing to list skills on a resume, but discussing how you’ve used them in real projects adds depth to your expertise.
- Shows passion and initiative: Personal projects or contributions to open-source can demonstrate your enthusiasm for coding beyond just work requirements.
2. Preparing Your Experience Arsenal
Before your interview, take time to reflect on your past experiences and prepare a mental catalog of projects and skills you can draw upon:
2.1. Review Your Projects
Go through your past projects, both professional and personal, and for each one:
- Summarize the project’s goal and your role
- Identify the key technologies and languages used
- Recall specific challenges you faced and how you overcame them
- Note any measurable outcomes or improvements resulting from your work
2.2. Categorize Your Skills
Create a list of your technical skills and categorize them:
- Programming languages (e.g., Python, Java, JavaScript)
- Frameworks and libraries (e.g., React, Django, TensorFlow)
- Tools and platforms (e.g., Git, Docker, AWS)
- Concepts and paradigms (e.g., OOP, functional programming, microservices)
2.3. Identify Transferable Skills
Don’t forget about soft skills and general problem-solving approaches:
- Analytical thinking
- Debugging strategies
- Code optimization techniques
- Project management methodologies
- Communication and documentation skills
3. Strategies for Weaving in Your Experience
Now that you’ve prepared your experience arsenal, let’s explore strategies for effectively bringing up your past projects and skills during the interview:
3.1. The STAR Method
The STAR method (Situation, Task, Action, Result) is an excellent framework for discussing your experiences:
- Situation: Briefly describe the context of the project or problem.
- Task: Explain what your specific role or responsibility was.
- Action: Detail the steps you took to address the task or solve the problem.
- Result: Share the outcomes of your actions, ideally with quantifiable results.
Example:
“In my previous role, we faced a challenge with our e-commerce platform’s search functionality (Situation). I was tasked with improving the search accuracy and speed (Task). I implemented an Elasticsearch-based solution and optimized our database queries (Action). As a result, we saw a 40% improvement in search speed and a 25% increase in successful searches, leading to a 15% boost in conversion rates (Result).”
3.2. Drawing Parallels
When faced with a coding problem or system design question, look for opportunities to draw parallels with your past experiences:
- Identify similar problems you’ve solved before
- Highlight relevant technologies or algorithms you’ve used
- Explain how your past approach could be adapted to the current problem
Example:
“This reminds me of a caching problem I encountered in a previous project. We were dealing with high-traffic API endpoints, and I implemented a Redis-based caching solution. I think a similar approach could work here, with some modifications to fit this specific use case.”
3.3. Showcasing Problem-Solving Approaches
Use your past experiences to demonstrate your problem-solving methodology:
- Explain how you break down complex problems
- Describe your approach to debugging and troubleshooting
- Share examples of how you’ve optimized code or improved performance
Example:
“When I encounter a complex problem like this, I typically start by breaking it down into smaller, manageable components. For instance, in a recent project involving a large-scale data processing pipeline, I first identified the bottlenecks by profiling each stage of the pipeline. Then, I tackled each bottleneck individually, starting with the most significant one.”
3.4. Highlighting Teamwork and Communication
Many coding interviews also assess your ability to work in a team. Use your experiences to showcase these skills:
- Describe how you’ve collaborated with other developers
- Explain your experience with code reviews and providing/receiving feedback
- Share instances where you’ve mentored junior developers or contributed to documentation
Example:
“In my last role, we used a peer programming approach for complex features. When implementing a new authentication system, I paired with a colleague to design the architecture. We used Git for version control and conducted thorough code reviews, which caught several potential security issues early in the development process.”
4. Connecting Your Experience to the Problem at Hand
One of the most powerful ways to leverage your past experience is by directly connecting it to the problem or question presented in the interview. Here are some strategies to make those connections:
4.1. Identify Common Patterns
Look for similarities between the current problem and issues you’ve tackled before:
- Algorithmic patterns (e.g., dynamic programming, graph traversal)
- Design patterns (e.g., singleton, factory, observer)
- Architectural patterns (e.g., MVC, microservices)
Example:
“This problem seems to have a similar structure to a dynamic programming challenge I encountered while optimizing a recommendation engine. In that case, we used memoization to store intermediate results, which significantly improved performance. We might be able to apply a similar technique here.”
4.2. Adapt Previous Solutions
Explain how you can adapt solutions from your past projects to fit the current problem:
- Discuss how you’d modify a previous approach to suit the new context
- Highlight the thought process behind adapting solutions
- Explain trade-offs and considerations when applying past solutions to new problems
Example:
“In a previous project, we implemented a caching layer to improve database query performance. While this problem is in a different domain, I think we could adapt that caching strategy here. We’d need to consider the cache invalidation policy and storage mechanism, but the core principle of reducing repeated computations would still apply.”
4.3. Leverage Language-Specific Knowledge
If you’ve worked extensively with a particular programming language, use that experience to showcase your deep understanding:
- Discuss language-specific features or optimizations
- Explain how you’ve used advanced language concepts in real projects
- Compare and contrast approaches in different languages you’ve used
Example:
“Given that this problem involves concurrent processing, I’m reminded of a project where I used Python’s asyncio library to handle multiple I/O-bound tasks efficiently. We could apply a similar asynchronous approach here, potentially using coroutines to manage the concurrent operations.”
4.4. Discuss Scalability and Performance
Many interview questions touch on issues of scalability and performance. Use your experience to demonstrate your understanding of these concepts:
- Share examples of how you’ve optimized systems for scale
- Discuss techniques you’ve used for performance profiling and improvement
- Explain trade-offs you’ve encountered between different optimization strategies
Example:
“This reminds me of a scalability challenge we faced with our user authentication service. As our user base grew, we needed to handle an increasing number of concurrent logins. We implemented a token-based authentication system using JWT and Redis for session management, which allowed us to scale horizontally by adding more application servers without increasing database load.”
5. Practical Examples of Connecting Experience to Interview Questions
Let’s look at some concrete examples of how you might connect your past experience to common types of coding interview questions:
5.1. Algorithm Questions
Question: “Implement a function to find the longest palindromic substring in a given string.”
Experience Connection:
“This problem reminds me of a text processing feature I implemented in a content management system. While we weren’t specifically looking for palindromes, we did need to analyze substrings efficiently. I used dynamic programming to optimize the substring comparisons, which I think could be adapted here.
Let me walk you through my thought process:
1. First, I’d create a 2D table to store whether substrings are palindromes.
2. We can fill this table diagonally, starting with single characters and moving to longer substrings.
3. As we fill the table, we’ll keep track of the longest palindrome found.This approach has a time complexity of O(n^2) and space complexity of O(n^2), where n is the length of the string. In my previous project, this was an acceptable trade-off for improved speed, but we should discuss if there are any specific constraints for this problem.”
5.2. System Design Questions
Question: “Design a URL shortening service like bit.ly.”
Experience Connection:
“I actually worked on a similar internal tool at my previous company for tracking marketing campaigns. While it wasn’t a public URL shortener, many of the core concepts apply. Here’s how I would approach this:
1. For the data model, we used a NoSQL database (MongoDB) to store the mapping between short codes and original URLs. This gave us flexibility and good read performance.
2. We generated short codes using a combination of base62 encoding and a counter, which ensured uniqueness and kept the URLs short.
3. To handle high read volumes, we implemented a caching layer using Redis. This significantly reduced the load on our database.
4. For analytics, which was crucial for our marketing team, we used a separate time-series database to store click data.
5. We deployed the service using Docker containers on Kubernetes, which allowed us to scale horizontally as traffic increased.
One challenge we faced was collision in short code generation at high volumes. We solved this by using a distributed ID generation system, similar to Twitter’s Snowflake.
For a public service like bit.ly, we’d need to consider additional factors like:
– Rate limiting to prevent abuse
– SSL certificates for secure redirects
– A more robust analytics system to handle public-scale dataWould you like me to elaborate on any specific part of this design?”
5.3. Coding Implementation Questions
Question: “Implement a basic rate limiter.”
Experience Connection:
“I implemented a rate limiter for our API gateway in my last role, so I’m quite familiar with this concept. We used the token bucket algorithm, which I found to be both efficient and flexible. Let me implement a basic version in Python:
import time class RateLimiter: def __init__(self, capacity, refill_rate): self.capacity = capacity self.refill_rate = refill_rate self.tokens = capacity self.last_refill_time = time.time() def allow_request(self): self._refill_tokens() if self.tokens >= 1: self.tokens -= 1 return True return False def _refill_tokens(self): now = time.time() time_passed = now - self.last_refill_time new_tokens = time_passed * self.refill_rate self.tokens = min(self.capacity, self.tokens + new_tokens) self.last_refill_time = now # Usage limiter = RateLimiter(capacity=10, refill_rate=1) # 10 requests per second for _ in range(15): print(limiter.allow_request()) time.sleep(0.1) # Simulate time passing between requests
This implementation allows for 10 requests per second. In our production system, we extended this concept to handle different rate limits for various API endpoints and user tiers.
We also integrated this with our monitoring system to alert us when users were consistently hitting rate limits, which often indicated either abusive behavior or a need to upgrade their service tier.
Is there any specific aspect of rate limiting you’d like me to elaborate on?”
6. Common Pitfalls to Avoid
While leveraging your past experience can be incredibly beneficial, there are some potential pitfalls to be aware of:
6.1. Overreliance on Past Solutions
While drawing from past experiences is valuable, be careful not to force-fit old solutions to new problems. Always approach each question with fresh eyes and be ready to adapt or completely change your approach if necessary.
6.2. Lengthy Explanations
While it’s great to provide context, be mindful of the interview’s time constraints. Keep your examples concise and relevant to the question at hand. If the interviewer wants more details, they’ll ask.
6.3. Focusing Too Much on Domain Knowledge
If your past experience is in a different domain than the company you’re interviewing with, be careful not to delve too deeply into domain-specific details. Focus on the transferable technical concepts and problem-solving approaches.
6.4. Neglecting the Current Problem
Remember that the primary goal is to solve the problem presented in the interview. Use your past experience to inform your approach, but make sure you’re actively engaging with the current question.
6.5. Overstating Your Role
Be honest about your contributions to past projects. It’s okay to highlight your achievements, but be prepared to discuss your exact role and contributions in detail if asked.
7. Practicing Your Approach
Like any skill, effectively leveraging your past experience in coding interviews requires practice. Here are some ways to hone this ability:
7.1. Mock Interviews
Conduct mock interviews with friends, colleagues, or through online platforms. Practice integrating your experiences into your problem-solving process and ask for feedback.
7.2. Reflect on Your Projects
Regularly review your past projects and think about how they relate to common interview questions or coding challenges. This will help you build a mental map of your experiences that you can quickly draw upon during interviews.
7.3. Stay Current
Keep your skills up-to-date and continue working on new projects. This will give you fresh experiences to draw from and demonstrate your commitment to ongoing learning.
7.4. Learn from Others
Read or watch coding interview experiences shared by others in the industry. Pay attention to how they connect their experiences to interview questions and try to apply similar techniques.
8. Conclusion
Leveraging your past experience effectively in coding interviews can significantly enhance your performance and help you stand out as a candidate. By thoughtfully connecting your previous projects and skills to the problems presented, you demonstrate not just your technical abilities, but also your problem-solving approach, your ability to learn and adapt, and your potential value to the company.
Remember, the goal is not just to showcase what you’ve done, but to illustrate how your experiences have shaped your thinking and problem-solving abilities. Use your past projects as a springboard for discussing your approach to new challenges, always keeping in mind the specific needs and context of the role you’re interviewing for.
With practice and reflection, you can turn your unique experiences into a powerful tool in your interview arsenal. So, before your next coding interview, take the time to review your experiences, prepare your stories, and think about how you can connect them to potential interview questions. Your past experiences are a valuable asset – use them wisely to demonstrate why you’re the ideal candidate for the job.