How to Spot Patterns: Recognizing Common Problem Types in Coding Interviews
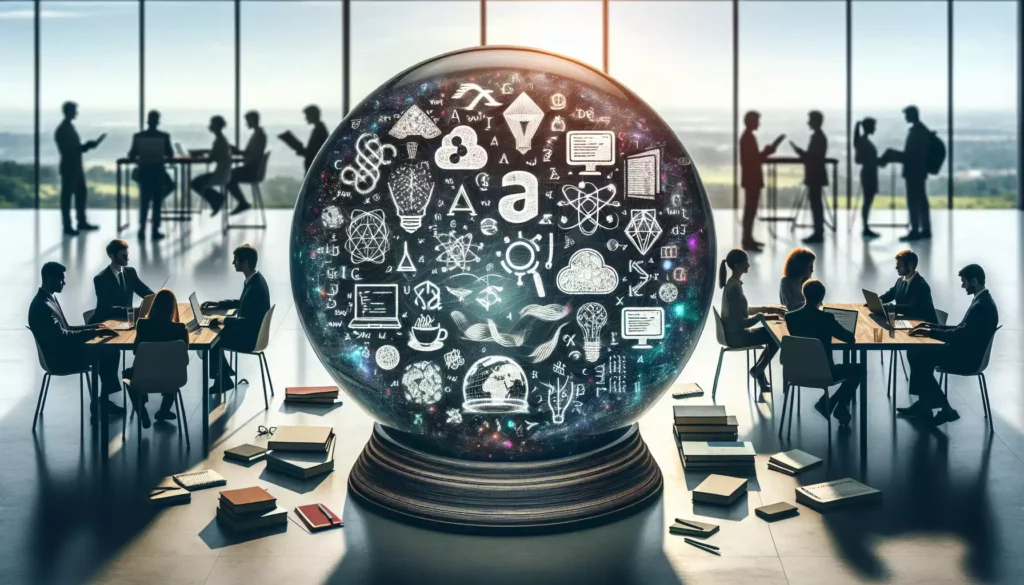
In the world of coding interviews, success often hinges on your ability to recognize patterns and apply the right problem-solving strategies. Whether you’re a seasoned developer or just starting your journey, understanding common problem types can significantly boost your performance and confidence. This comprehensive guide will help you master the art of pattern recognition in coding interviews, enabling you to tackle a wide range of challenges with ease.
1. Introduction to Pattern Recognition in Coding Interviews
Pattern recognition is a crucial skill in coding interviews. It involves identifying similarities between the problem at hand and previously encountered problems or well-known algorithmic patterns. By recognizing these patterns, you can quickly formulate an approach to solve the problem efficiently.
The benefits of mastering pattern recognition include:
- Faster problem-solving: You can quickly identify the core of the problem and apply a suitable strategy.
- Improved efficiency: Recognizing patterns helps you choose optimal algorithms and data structures.
- Enhanced confidence: Familiarity with common patterns reduces anxiety during interviews.
- Better time management: You can allocate your time more effectively during timed coding challenges.
2. Common Problem Types and Their Patterns
Let’s explore some of the most frequently encountered problem types in coding interviews and the patterns associated with them.
2.1 Dynamic Programming
Dynamic Programming (DP) is a powerful technique used to solve optimization problems by breaking them down into smaller subproblems.
Key characteristics of DP problems:
- Overlapping subproblems
- Optimal substructure
- Decision-making at each step
Common DP patterns:
- 0/1 Knapsack
- Longest Common Subsequence
- Coin Change
- Fibonacci Sequence
Example problem: “Given a set of coin denominations and a target amount, find the minimum number of coins needed to make up that amount.”
To recognize DP problems, look for questions that ask for optimization (minimization or maximization) and involve making a series of interconnected decisions.
2.2 Binary Search
Binary Search is an efficient algorithm for searching sorted arrays or solving problems with a monotonic search space.
Key characteristics of Binary Search problems:
- Sorted input or monotonic search space
- Ability to eliminate half of the remaining possibilities in each step
- Looking for a specific value or condition
Common Binary Search patterns:
- Classic Binary Search
- Search in Rotated Sorted Array
- Find First and Last Position of Element in Sorted Array
- Search in 2D Matrix
Example problem: “Given a sorted array of integers, find the index of a given target value. If the target is not found, return the index where it would be inserted to maintain the sorted order.”
To recognize Binary Search problems, look for questions involving sorted arrays or where you can establish a clear relationship between the input and the desired output that allows for systematic narrowing of the search space.
2.3 Graph Traversal
Graph traversal problems involve exploring or analyzing the structure of a graph or tree.
Key characteristics of Graph Traversal problems:
- Involve nodes and edges
- May require exploring all or part of the graph
- Often involve finding paths, cycles, or connected components
Common Graph Traversal patterns:
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
- Topological Sort
- Shortest Path Algorithms (e.g., Dijkstra’s, Floyd-Warshall)
Example problem: “Given a binary tree, return the level order traversal of its nodes’ values (i.e., from left to right, level by level).”
To recognize Graph Traversal problems, look for questions that mention relationships between entities, network connections, or hierarchical structures. Keywords like “connected,” “path,” or “tree” often indicate a graph problem.
2.4 Two Pointers
The Two Pointers technique involves using two pointers to traverse an array or string, often moving in opposite directions or at different speeds.
Key characteristics of Two Pointers problems:
- Involve arrays or strings
- Require comparing or manipulating elements at different positions
- Often used for in-place operations
Common Two Pointers patterns:
- Fast and Slow Pointers
- Left and Right Pointers
- Sliding Window
- Merging Sorted Arrays
Example problem: “Given a sorted array of integers, remove the duplicates in-place such that each element appears only once and return the new length.”
To recognize Two Pointers problems, look for questions that involve comparing elements in an array or string, or problems that can be solved by manipulating elements in-place.
2.5 Sliding Window
The Sliding Window technique is a variation of the Two Pointers approach, used to process sequential data in fixed-size or variable-size chunks.
Key characteristics of Sliding Window problems:
- Involve arrays, strings, or linked lists
- Require finding subarrays or substrings that satisfy certain conditions
- Often involve optimization of a window’s size or content
Common Sliding Window patterns:
- Fixed-size window
- Variable-size window
- String matching
- Substring problems
Example problem: “Given an array of integers and a positive integer k, find the maximum sum of any contiguous subarray of size k.”
To recognize Sliding Window problems, look for questions that involve subarrays or substrings, especially when they mention contiguous elements or a specific window size.
3. Tips for Mapping Problems to Patterns
Now that we’ve covered some common problem types, let’s discuss strategies for mapping new problems to these patterns:
3.1 Identify Key Problem Characteristics
Start by analyzing the problem statement and identifying key characteristics:
- Input type (array, string, tree, graph, etc.)
- Constraints (sorted, unsorted, size limits, etc.)
- Output requirements (optimization, search, transformation, etc.)
- Special conditions or rules mentioned in the problem
3.2 Look for Pattern Indicators
Certain keywords or phrases can hint at specific patterns:
- “Minimum” or “Maximum” often suggest Dynamic Programming or Greedy Algorithms
- “Sorted array” might indicate Binary Search
- “Connected components” or “paths” typically involve Graph Traversal
- “In-place” operations on arrays often use Two Pointers
- “Contiguous subarray” or “substring” could point to Sliding Window
3.3 Consider Multiple Patterns
Some problems may involve multiple patterns or have alternative solutions. Don’t limit yourself to a single approach:
- Consider different patterns that might apply
- Evaluate the trade-offs between different approaches (time complexity, space complexity, implementation difficulty)
- Be prepared to combine multiple patterns for more complex problems
3.4 Practice Pattern Recognition
Improving your pattern recognition skills requires practice:
- Solve a variety of problems and categorize them by pattern
- Review solutions to problems you’ve solved and identify the underlying patterns
- Study classic problems for each pattern to internalize their characteristics
3.5 Use a Systematic Approach
Develop a systematic approach to problem-solving:
- Read the problem carefully and identify key information
- Consider the input, output, and constraints
- Think about similar problems you’ve encountered
- Identify potential patterns that might apply
- Sketch out a high-level approach based on the chosen pattern
- Validate your approach with example inputs
- Implement the solution, starting with a basic structure and refining as needed
4. Common Patterns and Their Implementations
Let’s explore some common patterns and their typical implementations to help you recognize and apply them in coding interviews.
4.1 Dynamic Programming
Dynamic Programming often follows this general structure:
// Initialize DP table
dp = [...]
// Base cases
dp[0] = ...
// Fill the DP table
for i in range(1, n):
for j in range(...):
dp[i][j] = max/min(some function of previous states)
// Return the final result
return dp[n][m]
Example: Fibonacci Sequence
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
4.2 Binary Search
The typical structure of a Binary Search algorithm:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = left + (right - left) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
4.3 Graph Traversal (DFS)
A typical Depth-First Search implementation:
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start) # Process the current node
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
4.4 Two Pointers
A common Two Pointers pattern for array manipulation:
def two_pointers(arr):
left, right = 0, len(arr) - 1
while left < right:
# Process or compare arr[left] and arr[right]
# Move pointers based on some condition
if condition:
left += 1
else:
right -= 1
return result
4.5 Sliding Window
A typical Sliding Window implementation:
def sliding_window(arr, k):
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, len(arr)):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
5. Practice Problems for Pattern Recognition
To help you practice pattern recognition, here are some problems categorized by their underlying patterns:
5.1 Dynamic Programming
- Longest Increasing Subsequence
- Edit Distance
- Coin Change
- Longest Common Subsequence
5.2 Binary Search
- Search in Rotated Sorted Array
- Find First and Last Position of Element in Sorted Array
- Search a 2D Matrix
- Find the Smallest Letter Greater Than Target
5.3 Graph Traversal
- Number of Islands
- Course Schedule (Topological Sort)
- Word Ladder
- Clone Graph
5.4 Two Pointers
- Container With Most Water
- 3Sum
- Remove Duplicates from Sorted Array
- Trapping Rain Water
5.5 Sliding Window
- Longest Substring Without Repeating Characters
- Minimum Size Subarray Sum
- Permutation in String
- Longest Repeating Character Replacement
6. Advanced Pattern Recognition Techniques
As you become more comfortable with basic patterns, consider these advanced techniques to further improve your pattern recognition skills:
6.1 Hybrid Patterns
Many complex problems require combining multiple patterns. For example:
- Binary Search + Dynamic Programming
- Graph Traversal + Dynamic Programming
- Two Pointers + Sliding Window
Practice identifying when and how to combine patterns for optimal solutions.
6.2 Pattern Variations
Recognize variations of common patterns:
- Bidirectional BFS for shortest path problems
- Monotonic stack for next greater element problems
- Meet in the middle for optimization problems
6.3 Problem Transformation
Learn to transform problems into known patterns:
- Converting a problem to a graph problem
- Reducing a problem to a well-known NP-complete problem
- Using mathematical transformations to simplify problems
7. Conclusion
Mastering pattern recognition in coding interviews is a powerful skill that can significantly improve your problem-solving abilities and increase your chances of success. By familiarizing yourself with common problem types, practicing their implementations, and developing a systematic approach to problem analysis, you’ll be well-equipped to tackle a wide range of coding challenges.
Remember that pattern recognition is not about memorizing solutions but understanding the underlying principles and strategies. As you practice, focus on building a mental framework for categorizing problems and mapping them to appropriate solution patterns.
Continue to challenge yourself with diverse problems, analyze your approach, and learn from both successes and mistakes. With time and practice, you’ll develop an intuition for recognizing patterns quickly and applying the most effective solutions in coding interviews.
Happy coding, and best of luck in your future interviews!