How to Avoid Over-Engineering: Focus on Functionality First, Optimization Second
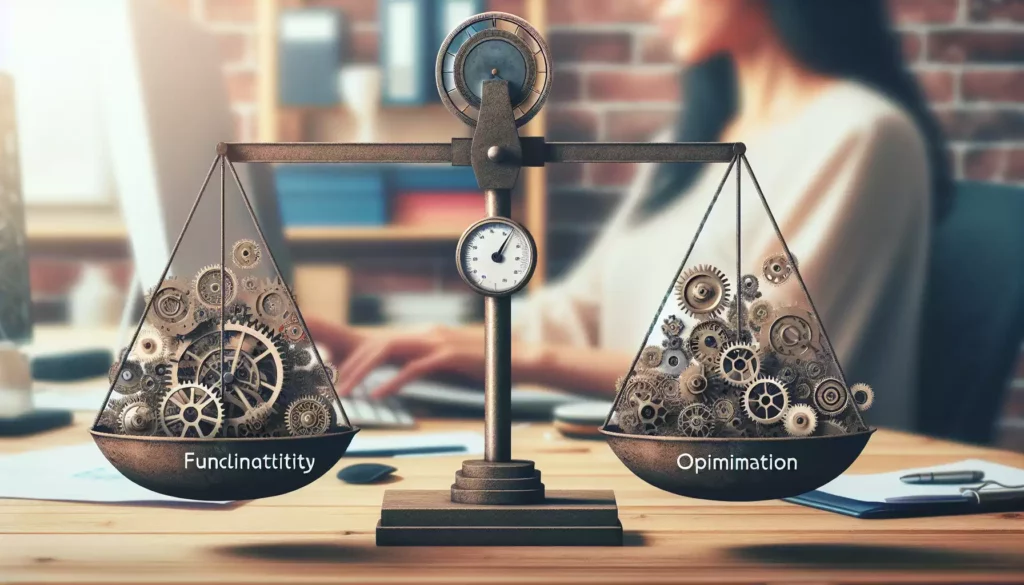
In the world of software development, particularly during technical interviews, there’s a common pitfall that many aspiring developers fall into: over-engineering. This tendency to create overly complex solutions can be a significant stumbling block, potentially costing you valuable time and even job opportunities. In this comprehensive guide, we’ll explore the concept of over-engineering, why it’s problematic, and most importantly, how to avoid it by focusing on functionality first and optimization second.
Understanding Over-Engineering
Before we dive into strategies to avoid over-engineering, let’s first understand what it means and why it’s a concern, especially in the context of coding interviews.
What is Over-Engineering?
Over-engineering occurs when a developer creates a solution that is unnecessarily complex or elaborate for the problem at hand. It often involves implementing advanced features, optimizations, or architectural patterns that aren’t required for the current scope of the problem.
Why is Over-Engineering Problematic?
While it might seem like showcasing advanced skills would impress interviewers, over-engineering can actually have several negative consequences:
- Time Consumption: Complex solutions take longer to implement, which can be critical in time-constrained interviews.
- Increased Bug Potential: More complex code is often more prone to bugs and errors.
- Reduced Readability: Overly complex solutions can be harder for others (including interviewers) to understand and evaluate.
- Maintenance Difficulties: In real-world scenarios, over-engineered solutions are often harder to maintain and update.
- Misalignment with Requirements: Over-engineering might lead to solutions that don’t precisely address the given problem.
The Importance of Functionality First
Now that we understand the pitfalls of over-engineering, let’s explore why focusing on functionality first is crucial, especially in interview settings.
1. Meeting Basic Requirements
The primary goal in any coding challenge or interview question is to solve the problem at hand. By focusing on functionality first, you ensure that you’re meeting the basic requirements of the task. This is crucial because a working solution, even if it’s not the most efficient, is always better than an incomplete or non-functional complex solution.
2. Demonstrating Problem-Solving Skills
Interviewers are often more interested in your problem-solving approach than in seeing the most optimized solution right away. By focusing on getting a working solution first, you demonstrate your ability to break down problems and implement solutions effectively.
3. Time Management
In the limited time of an interview, it’s critical to show that you can produce working code. Starting with a simpler, functional solution allows you to manage your time more effectively and ensures you have something to show by the end of the interview.
4. Establishing a Base for Optimization
Having a working solution provides a solid foundation from which you can discuss and implement optimizations. It’s much easier to optimize existing functional code than to try to build an optimized solution from scratch.
Strategies to Focus on Functionality First
Let’s explore some practical strategies to help you focus on functionality and avoid the temptation of over-engineering during coding interviews.
1. Start with a Brute Force Approach
Don’t be afraid to start with the simplest possible solution, even if it’s not the most efficient. A brute force approach can help you:
- Clarify your understanding of the problem
- Identify edge cases
- Provide a working solution quickly
Here’s an example of a brute force approach to find the maximum subarray sum:
def max_subarray_sum_brute_force(arr):
n = len(arr)
max_sum = float('-inf')
for i in range(n):
for j in range(i, n):
current_sum = sum(arr[i:j+1])
max_sum = max(max_sum, current_sum)
return max_sum
# Example usage
arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
print(max_subarray_sum_brute_force(arr)) # Output: 6
While this solution has a time complexity of O(n^3), it’s straightforward and easy to implement. It provides a working solution that you can optimize later.
2. Use Simple Data Structures Initially
Start with basic data structures like arrays or dictionaries before considering more complex ones. This approach helps in:
- Quickly implementing a working solution
- Keeping the code readable and easy to explain
- Avoiding unnecessary complexity
For example, when solving a problem that requires counting elements, start with a simple dictionary before considering more complex data structures:
def count_elements(arr):
count_dict = {}
for element in arr:
if element in count_dict:
count_dict[element] += 1
else:
count_dict[element] = 1
return count_dict
# Example usage
arr = [1, 2, 3, 1, 2, 1, 3, 4]
print(count_elements(arr)) # Output: {1: 3, 2: 2, 3: 2, 4: 1}
3. Implement Core Functionality Before Edge Cases
Focus on implementing the main functionality of your solution before handling edge cases. This approach allows you to:
- Demonstrate your understanding of the core problem
- Have a working solution for the majority of inputs
- Discuss potential edge cases with the interviewer
Here’s an example of implementing a basic binary search before handling edge cases:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Example usage
arr = [1, 3, 5, 7, 9, 11, 13]
print(binary_search(arr, 7)) # Output: 3
print(binary_search(arr, 6)) # Output: -1
After implementing this basic version, you can discuss potential improvements, such as handling empty arrays or implementing it recursively.
4. Write Modular Code
Break down your solution into smaller, manageable functions. This approach:
- Improves code readability
- Makes it easier to identify and fix issues
- Allows for easier optimization of specific parts later
Here’s an example of a modular approach to solving the “Two Sum” problem:
def two_sum(nums, target):
num_dict = create_number_dictionary(nums)
return find_pair(nums, num_dict, target)
def create_number_dictionary(nums):
num_dict = {}
for i, num in enumerate(nums):
num_dict[num] = i
return num_dict
def find_pair(nums, num_dict, target):
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict and num_dict[complement] != i:
return [i, num_dict[complement]]
return [] # No solution found
# Example usage
nums = [2, 7, 11, 15]
target = 9
print(two_sum(nums, target)) # Output: [0, 1]
The Role of Optimization
While focusing on functionality first is crucial, optimization still plays an important role in the development process. Let’s explore when and how to approach optimization without falling into the over-engineering trap.
When to Consider Optimization
Optimization should be considered:
- After achieving a working solution: Once you have a functional solution, you can start thinking about ways to improve it.
- When prompted by the interviewer: Sometimes, interviewers might ask you to optimize your initial solution.
- If there’s remaining time: If you’ve implemented a working solution and have time left, you can discuss potential optimizations.
Approaches to Optimization
When it’s time to optimize, consider the following approaches:
1. Time Complexity Optimization
Look for ways to reduce the time complexity of your algorithm. This often involves:
- Using more efficient data structures
- Implementing dynamic programming techniques
- Utilizing divide-and-conquer strategies
For example, let’s optimize our earlier max subarray sum problem:
def max_subarray_sum_optimized(arr):
max_sum = current_sum = arr[0]
for num in arr[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
# Example usage
arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
print(max_subarray_sum_optimized(arr)) # Output: 6
This optimized version has a time complexity of O(n), a significant improvement over the O(n^3) brute force approach.
2. Space Complexity Optimization
Consider ways to reduce the memory usage of your solution. This might involve:
- Using in-place algorithms
- Optimizing data structure usage
- Implementing space-efficient algorithms
Here’s an example of optimizing space complexity for the “Reverse String” problem:
def reverse_string(s):
# Convert string to list for in-place modification
s = list(s)
left, right = 0, len(s) - 1
while left < right:
s[left], s[right] = s[right], s[left]
left += 1
right -= 1
return ''.join(s)
# Example usage
input_string = "hello"
print(reverse_string(input_string)) # Output: "olleh"
This in-place reversal uses O(1) extra space, compared to creating a new string which would use O(n) extra space.
3. Code Readability and Maintainability
Optimization isn’t just about performance. Consider ways to make your code more readable and maintainable:
- Use meaningful variable and function names
- Add comments to explain complex logic
- Refactor repeated code into functions
Here’s an example of improving code readability:
def is_palindrome(s):
# Remove non-alphanumeric characters and convert to lowercase
s = ''.join(char.lower() for char in s if char.isalnum())
# Check if the string is equal to its reverse
return s == s[::-1]
# Example usage
test_strings = ["A man, a plan, a canal: Panama", "race a car", ""]
for string in test_strings:
print(f"'{string}' is palindrome: {is_palindrome(string)}")
# Output:
# 'A man, a plan, a canal: Panama' is palindrome: True
# 'race a car' is palindrome: False
# '' is palindrome: True
Balancing Functionality and Optimization
The key to successful problem-solving in coding interviews (and in real-world development) is finding the right balance between functionality and optimization. Here are some tips to help you strike that balance:
1. Communicate Your Approach
Always communicate your thought process to the interviewer. Explain that you’re starting with a simple, functional solution and that you plan to optimize it later. This shows that you’re aware of potential improvements but are prioritizing a working solution first.
2. Implement in Stages
Consider implementing your solution in stages:
- Start with a basic, functional solution
- Identify and handle edge cases
- Optimize for time complexity
- Optimize for space complexity (if necessary)
- Improve code readability and structure
This staged approach ensures that you always have a working solution while progressively improving it.
3. Know When to Stop
It’s important to recognize when further optimization might lead to diminishing returns or unnecessary complexity. If your solution meets the requirements and performs efficiently for the given constraints, it might be better to stop and discuss potential further optimizations theoretically rather than implementing them.
4. Learn from Each Problem
After each coding challenge or interview, reflect on your approach. Ask yourself:
- Did I focus too much on optimization at the expense of functionality?
- Did I miss any obvious optimizations in my initial solution?
- How can I improve my balance between functionality and optimization in future problems?
Conclusion
Avoiding over-engineering by focusing on functionality first and optimization second is a crucial skill for success in coding interviews and real-world software development. By implementing a working solution before diving into optimizations, you demonstrate your problem-solving abilities, manage your time effectively, and provide a solid foundation for further improvements.
Remember, the goal in most coding interviews is not to create the perfect, most optimized solution right away. Instead, it’s to show your thought process, problem-solving skills, and ability to write functional code. By following the strategies outlined in this guide, you’ll be well-equipped to tackle coding challenges with confidence, avoiding the pitfalls of over-engineering while still showcasing your ability to optimize when necessary.
As you continue to practice and refine your coding skills, always keep in mind the balance between functionality and optimization. With time and experience, you’ll develop an intuition for when to keep things simple and when to implement more complex solutions. This balance is not just crucial for acing coding interviews, but also for becoming a more effective and efficient software developer in your future career.