Prioritizing Simplicity: Why Simple Solutions Often Win in Coding Interviews
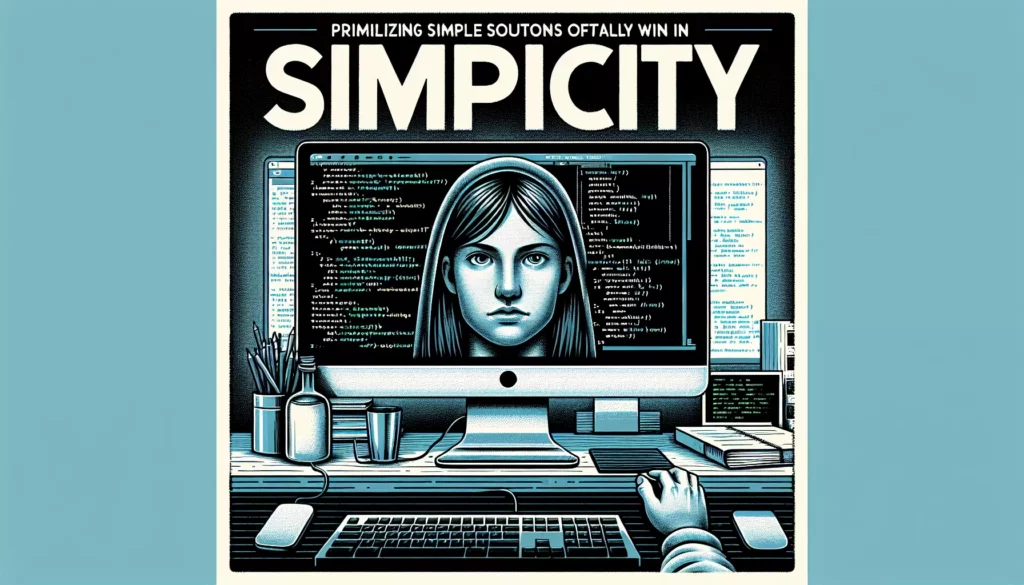
In the world of coding interviews, particularly those conducted by major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), there’s a common misconception that the most complex or clever solution is always the best. However, experienced interviewers often value simplicity and clarity over unnecessary complexity. This article will explore why simple solutions are often preferred in coding interviews and provide practical examples of how to refactor code for simplicity while maintaining functionality.
The Value of Simplicity in Coding Interviews
Before diving into specific examples and techniques, it’s crucial to understand why simplicity is highly valued in coding interviews:
- Readability: Simple code is easier to read and understand, both for the interviewer and for potential team members who might work with the code in the future.
- Maintainability: Simpler solutions are often easier to maintain, debug, and extend as requirements change.
- Efficiency: Contrary to popular belief, simpler solutions can often be more efficient in terms of time and space complexity.
- Error Reduction: Complex code is more prone to bugs and edge cases, while simpler code tends to be more robust.
- Time Management: In an interview setting, a simpler solution can be implemented and explained more quickly, allowing for better time management.
- Problem-Solving Skills: The ability to simplify complex problems demonstrates strong problem-solving and analytical skills.
Examples of Simplifying Code
Let’s look at some concrete examples of how to refactor code for simplicity while maintaining functionality. We’ll start with common scenarios and progressively move to more complex situations.
1. Simplifying Conditional Statements
Complex conditional statements can often be simplified, making the code more readable and maintainable. Consider the following example:
// Complex conditional
if (age > 18 && age < 65) {
if (income > 30000) {
if (creditScore > 700) {
approveLoad();
} else {
rejectLoan();
}
} else {
rejectLoan();
}
} else {
rejectLoan();
}
// Simplified version
if (age > 18 && age < 65 && income > 30000 && creditScore > 700) {
approveLoad();
} else {
rejectLoan();
}
In this example, we’ve reduced nested if statements into a single condition, making the code more readable and reducing the cognitive load required to understand the logic.
2. Leveraging Built-in Functions and Libraries
Sometimes, developers try to reinvent the wheel when there are built-in functions or libraries that can simplify the code. Here’s an example using JavaScript:
// Complex custom implementation
function isPalindrome(str) {
str = str.toLowerCase().replace(/[^a-z0-9]/g, '');
let left = 0;
let right = str.length - 1;
while (left < right) {
if (str[left] !== str[right]) {
return false;
}
left++;
right--;
}
return true;
}
// Simplified version using built-in methods
function isPalindrome(str) {
str = str.toLowerCase().replace(/[^a-z0-9]/g, '');
return str === str.split('').reverse().join('');
}
The simplified version leverages JavaScript’s built-in methods to achieve the same result with less code and improved readability.
3. Simplifying Loops and Iterations
Complex loop structures can often be simplified using higher-order functions or more appropriate loop types. Here’s an example in Python:
// Complex loop structure
result = []
for i in range(len(numbers)):
if numbers[i] % 2 == 0:
result.append(numbers[i] * 2)
else:
result.append(numbers[i])
// Simplified version using list comprehension
result = [num * 2 if num % 2 == 0 else num for num in numbers]
The simplified version uses a list comprehension, which is not only more concise but also more Pythonic and often more efficient.
4. Simplifying Data Structures
Choosing the right data structure can significantly simplify your code. Consider this example where we’re counting the frequency of words in a sentence:
// Complex implementation using arrays
function wordFrequency(sentence) {
const words = sentence.toLowerCase().split(' ');
const result = [];
for (let word of words) {
let found = false;
for (let i = 0; i < result.length; i++) {
if (result[i][0] === word) {
result[i][1]++;
found = true;
break;
}
}
if (!found) {
result.push([word, 1]);
}
}
return result;
}
// Simplified version using an object
function wordFrequency(sentence) {
const words = sentence.toLowerCase().split(' ');
const frequency = {};
for (let word of words) {
frequency[word] = (frequency[word] || 0) + 1;
}
return frequency;
}
The simplified version uses an object (which acts like a hash map in JavaScript) to count word frequencies, resulting in cleaner and more efficient code.
5. Simplifying Recursive Solutions
Recursive solutions can sometimes be simplified or replaced with iterative solutions. Here’s an example of simplifying the classic factorial function:
// Complex recursive solution
function factorial(n) {
if (n === 0 || n === 1) {
return 1;
}
return n * factorial(n - 1);
}
// Simplified iterative solution
function factorial(n) {
let result = 1;
for (let i = 2; i <= n; i++) {
result *= i;
}
return result;
}
While the recursive solution is elegant, the iterative solution is often more efficient and easier to understand, especially for larger values of n.
Strategies for Simplifying Your Code
Now that we’ve seen some examples, let’s discuss some general strategies you can apply to simplify your code:
1. Use Descriptive Variable Names
Clear, descriptive variable names can make your code self-documenting and easier to understand. For example:
// Complex
const x = getData();
const y = processData(x);
const z = y.filter(item => item.v > 10);
// Simplified
const rawData = getData();
const processedData = processData(rawData);
const filteredData = processedData.filter(item => item.value > 10);
2. Break Down Complex Functions
If a function is doing too much, break it down into smaller, more focused functions. This not only simplifies the code but also improves reusability and testability.
// Complex function
function processUserData(userData) {
// Validate user data
if (!userData.name || !userData.email || !userData.age) {
throw new Error('Invalid user data');
}
// Normalize data
userData.name = userData.name.trim().toLowerCase();
userData.email = userData.email.trim().toLowerCase();
// Calculate user category
let category;
if (userData.age < 18) {
category = 'junior';
} else if (userData.age < 65) {
category = 'adult';
} else {
category = 'senior';
}
// Save to database
saveToDatabase(userData, category);
}
// Simplified version with separate functions
function validateUserData(userData) {
if (!userData.name || !userData.email || !userData.age) {
throw new Error('Invalid user data');
}
}
function normalizeUserData(userData) {
return {
...userData,
name: userData.name.trim().toLowerCase(),
email: userData.email.trim().toLowerCase()
};
}
function calculateUserCategory(age) {
if (age < 18) return 'junior';
if (age < 65) return 'adult';
return 'senior';
}
function processUserData(userData) {
validateUserData(userData);
const normalizedData = normalizeUserData(userData);
const category = calculateUserCategory(userData.age);
saveToDatabase(normalizedData, category);
}
3. Use Appropriate Design Patterns
Design patterns can help simplify complex code structures. For example, the Strategy pattern can simplify code with multiple conditional statements:
// Complex code with multiple conditionals
function calculateShipping(method, weight) {
if (method === 'ground') {
return weight * 1.5;
} else if (method === 'air') {
return weight * 3;
} else if (method === 'sea') {
return weight * 0.5;
}
throw new Error('Invalid shipping method');
}
// Simplified version using the Strategy pattern
const shippingStrategies = {
ground: weight => weight * 1.5,
air: weight => weight * 3,
sea: weight => weight * 0.5
};
function calculateShipping(method, weight) {
const strategy = shippingStrategies[method];
if (!strategy) {
throw new Error('Invalid shipping method');
}
return strategy(weight);
}
4. Leverage Functional Programming Concepts
Functional programming concepts like map, filter, and reduce can often simplify complex loop structures:
// Complex imperative code
function processNumbers(numbers) {
const result = [];
for (let i = 0; i < numbers.length; i++) {
if (numbers[i] % 2 === 0) {
result.push(numbers[i] * 2);
}
}
return result;
}
// Simplified functional version
function processNumbers(numbers) {
return numbers.filter(n => n % 2 === 0).map(n => n * 2);
}
5. Use Modern Language Features
Modern programming languages often introduce features that can simplify common patterns. For example, in JavaScript, object destructuring and the spread operator can simplify object manipulation:
// Complex object manipulation
function updateUser(user, newProperties) {
const updatedUser = {};
for (let key in user) {
updatedUser[key] = user[key];
}
for (let key in newProperties) {
updatedUser[key] = newProperties[key];
}
return updatedUser;
}
// Simplified version using spread operator
function updateUser(user, newProperties) {
return { ...user, ...newProperties };
}
The Balance Between Simplicity and Optimization
While simplicity is crucial, it’s important to note that there can sometimes be a trade-off between simplicity and performance optimization. In coding interviews, it’s often best to start with a simple, clear solution and then discuss potential optimizations if time allows.
Here’s an example of how you might approach this:
// Simple solution for finding the maximum subarray sum
function maxSubarraySum(arr) {
let maxSum = arr[0];
let currentSum = arr[0];
for (let i = 1; i < arr.length; i++) {
currentSum = Math.max(arr[i], currentSum + arr[i]);
maxSum = Math.max(maxSum, currentSum);
}
return maxSum;
}
// More optimized but complex solution (Kadane's algorithm)
function optimizedMaxSubarraySum(arr) {
let maxSoFar = arr[0];
let maxEndingHere = arr[0];
for (let i = 1; i < arr.length; i++) {
maxEndingHere = maxEndingHere + arr[i];
if (maxEndingHere < arr[i]) {
maxEndingHere = arr[i];
}
if (maxSoFar < maxEndingHere) {
maxSoFar = maxEndingHere;
}
}
return maxSoFar;
}
In an interview, you might start with the simpler solution, explain its logic, and then mention that there’s a more optimized version (Kadane’s algorithm) if the interviewer is interested in discussing further optimizations.
Conclusion
Prioritizing simplicity in coding interviews demonstrates not just your coding skills, but also your ability to write maintainable, readable, and efficient code. By focusing on clear, concise solutions, you show that you understand the importance of code that can be easily understood and modified by others – a crucial skill in any professional development environment.
Remember, the goal in a coding interview is not just to solve the problem, but to demonstrate your problem-solving process and your ability to write production-quality code. By prioritizing simplicity, you’re showing that you can balance theoretical knowledge with practical application, a skill highly valued by companies like FAANG.
As you prepare for coding interviews, practice refactoring complex solutions into simpler ones. Look for opportunities to use built-in functions, appropriate data structures, and modern language features to streamline your code. And always be ready to explain your thought process – why you chose a particular approach and how it balances simplicity with functionality and performance.
In the end, the ability to write simple, elegant solutions to complex problems is what separates good programmers from great ones. By mastering this skill, you’ll not only perform better in coding interviews but also become a more effective and valuable software developer in your career.