Coding Like an Entrepreneur: How to Think About Problems Like a Startup Founder
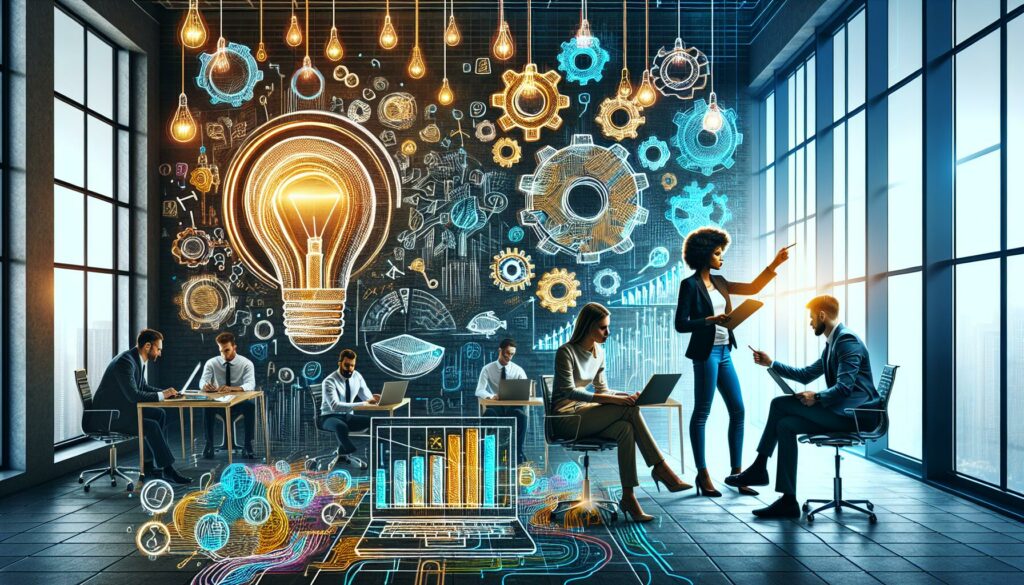
In the fast-paced world of technology, the ability to code is no longer just a technical skill—it’s a mindset. As the lines between software development and entrepreneurship continue to blur, it’s becoming increasingly important for programmers to think like startup founders. This approach not only enhances problem-solving abilities but also opens up new avenues for innovation and career growth. In this comprehensive guide, we’ll explore how to cultivate an entrepreneurial mindset in your coding journey, drawing parallels between coding challenges and the hurdles faced by startup founders.
The Entrepreneurial Coder’s Mindset
Before diving into specific strategies, it’s crucial to understand what it means to think like an entrepreneur. Startup founders are known for their:
- Ability to identify and solve real-world problems
- Resilience in the face of setbacks
- Creativity in finding innovative solutions
- Adaptability to changing circumstances
- Customer-centric approach to product development
As a coder, adopting these traits can significantly enhance your approach to programming challenges and career development.
1. Identify Real-World Problems
Entrepreneurs are constantly on the lookout for problems that need solving. As a coder, you should cultivate this same awareness. Instead of just completing coding exercises or building projects for the sake of learning, ask yourself:
- What real-world issues can my coding skills address?
- Are there inefficiencies in existing systems that I can improve?
- What pain points do people in my community or industry face that software could alleviate?
By focusing on actual problems, you’ll not only improve your coding skills but also develop solutions that have genuine value. This approach can lead to personal projects that showcase your abilities to potential employers or even form the basis of your own startup.
Practical Exercise:
Spend a week observing your daily routines and those of people around you. Note down at least three inefficiencies or annoyances that you encounter. Then, brainstorm how you could potentially solve these issues with a software solution. This exercise will train you to spot opportunities for innovation in everyday life.
2. Embrace the MVP (Minimum Viable Product) Concept
In the startup world, the MVP is a crucial concept. It’s about creating a product with just enough features to satisfy early customers and provide feedback for future development. As a coder, you can apply this principle to your projects:
- Start with the core functionality
- Release early and iterate based on feedback
- Don’t aim for perfection in the first version
This approach allows you to validate your ideas quickly and learn from real user interactions. It’s also an excellent way to manage large projects without getting overwhelmed.
Code Example: Building an MVP Todo App
Let’s look at how you might approach building a simple todo app using the MVP concept:
<!-- HTML -->
<input id="taskInput" type="text" placeholder="Enter a task">
<button onclick="addTask()">Add Task</button>
<ul id="taskList"></ul>
<!-- JavaScript -->
<script>
function addTask() {
var input = document.getElementById("taskInput");
var task = input.value;
if (task) {
var li = document.createElement("li");
li.textContent = task;
document.getElementById("taskList").appendChild(li);
input.value = "";
}
}
</script>
This basic implementation focuses on the core functionality of adding tasks. From here, you can iterate by adding features like task deletion, marking tasks as complete, or saving tasks to local storage.
3. Develop a Customer-Centric Approach
Successful entrepreneurs always keep their users in mind. As a coder, you should adopt this user-first mentality:
- Consider the end-user experience in every line of code you write
- Prioritize usability and accessibility in your projects
- Seek and incorporate user feedback regularly
This approach will not only make your code more valuable but also help you develop empathy—a crucial skill for both programmers and entrepreneurs.
Practical Tips:
- Conduct user testing sessions for your projects
- Create user personas to guide your development decisions
- Implement analytics to understand how users interact with your software
4. Learn to Pivot
Startup founders often need to pivot their business model or product direction based on market feedback. As a coder, you should be prepared to:
- Abandon code or projects that aren’t working
- Refactor your codebase when new requirements emerge
- Be open to learning new technologies as the industry evolves
This flexibility will serve you well in your coding career, whether you’re working on personal projects or as part of a team.
Code Example: Pivoting a Function
Consider this scenario: You’ve written a function to calculate the area of a circle, but now you need to expand it to handle multiple shapes.
// Original function
function calculateArea(radius) {
return Math.PI * radius * radius;
}
// Pivoted function
function calculateArea(shape, ...dimensions) {
switch(shape.toLowerCase()) {
case 'circle':
return Math.PI * dimensions[0] * dimensions[0];
case 'rectangle':
return dimensions[0] * dimensions[1];
case 'triangle':
return 0.5 * dimensions[0] * dimensions[1];
default:
throw new Error("Unsupported shape");
}
}
This pivot demonstrates adaptability in code, allowing for future expansion while maintaining the original functionality.
5. Embrace Continuous Learning
The tech landscape is constantly evolving, and successful entrepreneurs stay ahead by continuously educating themselves. As a coder, you should:
- Stay updated with the latest programming languages and frameworks
- Attend coding bootcamps, workshops, and conferences
- Engage in online coding communities and forums
- Contribute to open-source projects
Platforms like AlgoCademy can be invaluable in this journey, offering structured learning paths and interactive coding challenges that simulate real-world problems.
Learning Strategy:
Create a personal learning roadmap. Identify key skills you want to acquire in the next 6-12 months and break them down into weekly learning goals. Use a mix of resources like online courses, coding challenges, and project-based learning to achieve these goals.
6. Network and Collaborate
Entrepreneurs understand the power of networking and collaboration. As a coder, you can benefit from:
- Participating in hackathons and coding competitions
- Joining or creating coding meetups
- Collaborating on open-source projects
- Building a strong online presence (e.g., GitHub profile, tech blog)
These activities not only improve your coding skills but also open up opportunities for mentorship, job prospects, and potential startup partnerships.
Networking Tip:
Create a “value-first” approach to networking. Instead of focusing on what you can gain, think about what you can offer to others. Share your knowledge through blog posts, help others debug their code, or offer to speak at local coding events. This approach will naturally attract valuable connections and opportunities.
7. Develop a Growth Mindset
Entrepreneurs often face failures and setbacks, but they view these as opportunities for growth. As a coder, cultivate a growth mindset by:
- Embracing challenges as learning opportunities
- Viewing criticism as constructive feedback
- Celebrating small wins and progress
- Learning from your coding mistakes and bugs
This mindset will help you persevere through difficult coding challenges and continually improve your skills.
Practical Exercise: Bug Journal
Start keeping a “bug journal.” Whenever you encounter a challenging bug or make a significant mistake, document:
- The nature of the bug/mistake
- How you discovered it
- The steps you took to solve it
- What you learned from the experience
This practice will help you turn frustrating moments into valuable learning experiences and develop problem-solving resilience.
8. Think in Systems and Scalability
Successful entrepreneurs think beyond individual products to entire ecosystems and scalable business models. As a coder, you can adopt this mindset by:
- Designing modular and reusable code
- Considering how your solutions can scale to handle increased load or functionality
- Thinking about the broader impact of your code on the entire system or application
Code Example: Scalable API Design
Consider this example of a scalable API design using Node.js and Express:
const express = require('express');
const app = express();
// Middleware for parsing JSON bodies
app.use(express.json());
// Versioning your API
app.use('/api/v1', require('./routes/v1'));
// Error handling middleware
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something broke!');
});
// Environment-based configuration
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
This structure allows for easy versioning, modular routing, and environment-based configuration, making it scalable and maintainable as the application grows.
9. Prioritize and Manage Time Effectively
Entrepreneurs often juggle multiple responsibilities and must prioritize effectively. As a coder, you can improve your productivity by:
- Using time management techniques like the Pomodoro Technique
- Prioritizing tasks based on impact and urgency
- Setting clear goals and deadlines for your coding projects
- Learning to say no to low-priority tasks or distractions
Time Management Tip: The Eisenhower Matrix
Use the Eisenhower Matrix to prioritize your coding tasks:
- Urgent and Important: Do these tasks immediately
- Important but Not Urgent: Schedule these tasks
- Urgent but Not Important: Delegate these tasks if possible
- Neither Urgent nor Important: Eliminate these tasks
This method can help you focus on high-impact coding activities and avoid getting bogged down in less critical tasks.
10. Develop a Prototype Mindset
Entrepreneurs often create prototypes to test ideas quickly. As a coder, you can adopt this approach by:
- Building quick proof-of-concept applications to validate ideas
- Using rapid prototyping tools and frameworks
- Focusing on core functionality before adding bells and whistles
Prototyping Exercise:
Challenge yourself to build a working prototype of an app idea in just 24 hours. This time constraint will force you to focus on the essential features and help you develop the skill of quickly turning ideas into tangible products.
Conclusion: The Entrepreneurial Coder’s Edge
By thinking like an entrepreneur, you’ll not only become a better coder but also position yourself for exciting opportunities in the tech world. Whether you aspire to launch your own startup or want to be an invaluable asset to your development team, these entrepreneurial thinking skills will set you apart.
Remember, the journey of an entrepreneurial coder is ongoing. Continuously challenge yourself, stay curious, and always be on the lookout for problems to solve. With this mindset, you’ll be well-equipped to navigate the ever-changing landscape of technology and create meaningful impact through your code.
As you continue your coding journey, consider leveraging platforms like AlgoCademy to hone your algorithmic thinking and problem-solving skills. These abilities are crucial not just for technical interviews, but for approaching complex challenges with an entrepreneurial mindset.
Embrace the entrepreneurial spirit in your coding practice, and watch as new doors of opportunity open before you. Happy coding, and may your entrepreneurial journey in the world of technology be filled with innovation, growth, and success!