How to Use the Socratic Method to Teach Yourself to Code
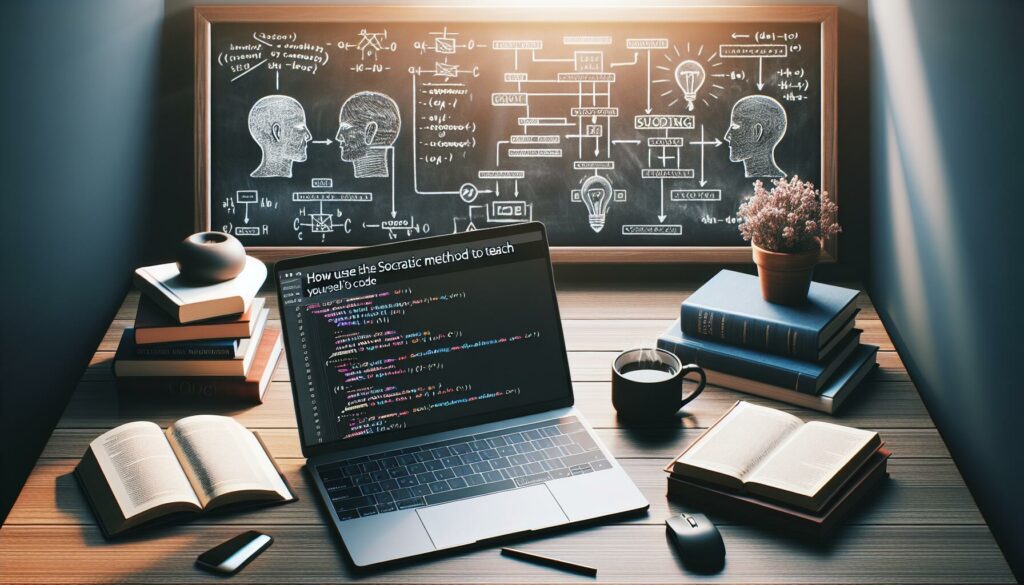
In the ever-evolving world of technology, learning to code has become an essential skill for many. Whether you’re aspiring to join the ranks of software developers at major tech companies or simply want to enhance your problem-solving abilities, mastering programming can open up a world of opportunities. But how can you effectively teach yourself to code? Enter the Socratic method – a powerful teaching technique that can revolutionize your self-learning journey.
Understanding the Socratic Method
Before we dive into how to apply the Socratic method to coding education, let’s first understand what it is. The Socratic method, named after the ancient Greek philosopher Socrates, is a form of cooperative argumentative dialogue between individuals. It’s based on asking and answering questions to stimulate critical thinking and to draw out ideas and underlying presuppositions.
In essence, the Socratic method involves:
- Asking probing questions
- Challenging assumptions
- Encouraging deep analysis
- Fostering critical thinking
When applied to learning, this method can help you gain a deeper understanding of complex concepts by breaking them down and examining them from different angles.
Why Use the Socratic Method for Coding?
You might wonder, “Why should I use the Socratic method to learn coding?” The answer lies in the nature of programming itself. Coding is not just about memorizing syntax or following a set of rigid rules. It’s about problem-solving, logical thinking, and creativity. The Socratic method aligns perfectly with these aspects of coding:
- Encourages Critical Thinking: By questioning your assumptions and approaches, you develop a more analytical mindset – crucial for debugging and optimizing code.
- Promotes Deep Understanding: Instead of surface-level knowledge, you gain insights into the underlying principles of programming languages and algorithms.
- Enhances Problem-Solving Skills: The method trains you to break down complex problems into manageable parts – a key skill in coding.
- Fosters Creativity: By challenging conventional thinking, you can come up with innovative solutions to coding challenges.
- Improves Self-Reflection: Regular self-questioning helps you identify your strengths and areas for improvement in your coding journey.
Applying the Socratic Method to Coding Education
Now that we understand the benefits, let’s explore how you can practically apply the Socratic method to teach yourself coding. Here’s a step-by-step guide:
1. Start with a Coding Concept or Problem
Begin by selecting a specific coding concept you want to learn or a problem you want to solve. For example, let’s say you want to understand how to implement a binary search algorithm.
2. Ask Fundamental Questions
Start by asking yourself basic questions about the concept:
- What is a binary search?
- Why is it useful?
- What are the prerequisites for using a binary search?
These questions help you establish a foundation and identify any gaps in your knowledge.
3. Break Down the Problem
Use questions to dissect the problem or concept:
- What are the key steps in a binary search?
- How does it differ from a linear search?
- What data structures are best suited for binary search?
This process helps you understand the components of the problem and how they relate to each other.
4. Challenge Your Assumptions
Question your initial understanding:
- Is binary search always the most efficient method?
- What are its limitations?
- Are there scenarios where it might fail?
This step prevents you from accepting information at face value and encourages critical analysis.
5. Explore Alternative Approaches
Ask yourself about different ways to solve the problem:
- Can I implement binary search iteratively and recursively?
- How would the implementation differ in various programming languages?
- Are there any optimizations I can make to the basic algorithm?
This fosters creativity and helps you understand the concept from multiple perspectives.
6. Implement and Test
As you code, continue to question your implementation:
- Why did I choose this particular approach?
- Is my code efficient and readable?
- How can I test edge cases?
This self-questioning during the coding process helps you write better, more thoughtful code.
7. Reflect and Iterate
After implementation, reflect on your learning:
- What did I learn from this process?
- How can I apply this knowledge to other coding problems?
- What areas do I need to explore further?
This reflection solidifies your learning and guides your future studies.
Practical Example: Implementing Binary Search
Let’s walk through a practical example of using the Socratic method to implement a binary search algorithm in Python. We’ll go through each step, asking questions and exploring the concept deeply.
Step 1: Understanding the Concept
Q: What is a binary search?
A: Binary search is an efficient algorithm for finding an item from a sorted list of items. It works by repeatedly dividing in half the portion of the list that could contain the item, until you’ve narrowed down the possible locations to just one.
Q: Why is it useful?
A: Binary search is much faster than linear search for large datasets. It has a time complexity of O(log n), which means it’s very efficient even for large amounts of data.
Q: What are the prerequisites for using a binary search?
A: The main prerequisite is that the list must be sorted. Binary search only works on sorted lists.
Step 2: Breaking Down the Problem
Q: What are the key steps in a binary search?
A: The key steps are:
1. Find the middle element of the list.
2. If the target value is equal to the middle element, we’re done.
3. If the target value is less than the middle element, repeat the search on the left half of the list.
4. If the target value is greater than the middle element, repeat the search on the right half of the list.
5. If the search space is empty, the target is not in the list.
Q: How does it differ from a linear search?
A: Linear search checks every element in the list sequentially, while binary search eliminates half of the remaining elements at each step.
Step 3: Challenging Assumptions
Q: Is binary search always the most efficient method?
A: Not always. For very small lists, linear search might be faster due to less overhead. Binary search is most efficient for large, sorted datasets.
Q: What are its limitations?
A: Binary search requires a sorted list, which can be a limitation if the list changes frequently. It also requires random access to elements, so it’s not suitable for certain data structures like linked lists.
Step 4: Exploring Alternative Approaches
Q: Can I implement binary search iteratively and recursively?
A: Yes, binary search can be implemented both iteratively and recursively. Let’s explore both approaches.
Step 5: Implementation
Let’s implement both iterative and recursive versions of binary search:
Iterative Implementation:
def binary_search_iterative(arr, x):
low = 0
high = len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == x:
return mid
elif arr[mid] < x:
low = mid + 1
else:
high = mid - 1
return -1 # Element not found
Recursive Implementation:
def binary_search_recursive(arr, x, low, high):
if low <= high:
mid = (low + high) // 2
if arr[mid] == x:
return mid
elif arr[mid] < x:
return binary_search_recursive(arr, x, mid + 1, high)
else:
return binary_search_recursive(arr, x, low, mid - 1)
else:
return -1 # Element not found
Step 6: Testing and Questioning
Now that we have our implementations, let’s ask some questions:
Q: How can we test these implementations?
A: We should test with various scenarios, including:
- A sorted list where the target is present
- A sorted list where the target is not present
- Edge cases like an empty list, a list with one element, and targets at the beginning or end of the list
Let’s write a test function:
def test_binary_search():
# Test case 1: Target in the middle
arr1 = [1, 3, 5, 7, 9, 11, 13]
assert binary_search_iterative(arr1, 7) == 3
assert binary_search_recursive(arr1, 7, 0, len(arr1)-1) == 3
# Test case 2: Target not in array
assert binary_search_iterative(arr1, 6) == -1
assert binary_search_recursive(arr1, 6, 0, len(arr1)-1) == -1
# Test case 3: Target at the beginning
assert binary_search_iterative(arr1, 1) == 0
assert binary_search_recursive(arr1, 1, 0, len(arr1)-1) == 0
# Test case 4: Target at the end
assert binary_search_iterative(arr1, 13) == 6
assert binary_search_recursive(arr1, 13, 0, len(arr1)-1) == 6
# Test case 5: Empty array
arr2 = []
assert binary_search_iterative(arr2, 1) == -1
assert binary_search_recursive(arr2, 1, 0, len(arr2)-1) == -1
# Test case 6: Array with one element
arr3 = [5]
assert binary_search_iterative(arr3, 5) == 0
assert binary_search_recursive(arr3, 5, 0, len(arr3)-1) == 0
print("All test cases passed!")
test_binary_search()
Step 7: Reflection and Iteration
After implementing and testing our binary search algorithms, let’s reflect on the process:
Q: What did we learn from this process?
A: We learned how to implement binary search both iteratively and recursively. We also learned the importance of thorough testing, including edge cases.
Q: How can we apply this knowledge to other coding problems?
A: The divide-and-conquer approach used in binary search can be applied to many other algorithms. The process of breaking down the problem, implementing a solution, and thoroughly testing it is applicable to virtually all coding tasks.
Q: What areas do we need to explore further?
A: We could explore the time and space complexity of our implementations in more detail. We could also look into variations of binary search, such as finding the first or last occurrence of an element in a sorted array with duplicates.
Benefits of Using the Socratic Method in Coding Education
By using the Socratic method to learn binary search, we’ve gained several benefits:
- Deep Understanding: We didn’t just memorize an algorithm; we understood why it works and its limitations.
- Critical Thinking: We questioned our assumptions and explored alternative implementations.
- Problem-Solving Skills: We broke down the problem into manageable steps and solved each part systematically.
- Self-Reflection: We constantly questioned our understanding and implementation, leading to more robust code.
- Comprehensive Learning: By exploring both iterative and recursive approaches, we gained a more complete understanding of the concept.
Integrating the Socratic Method with Other Learning Resources
While the Socratic method is powerful on its own, it can be even more effective when combined with other learning resources and techniques. Here are some ways to integrate the Socratic method into your broader coding education:
1. Online Coding Platforms
Platforms like AlgoCademy offer interactive coding tutorials and problem sets. As you work through these resources, apply the Socratic method by questioning each step of the process. Ask yourself why certain approaches are used and how they relate to concepts you’ve learned previously.
2. Textbooks and Documentation
When reading coding textbooks or language documentation, don’t just passively absorb information. Actively engage with the material by asking questions. For example, when learning about a new programming concept, ask yourself:
- How does this concept relate to what I already know?
- In what scenarios would this be particularly useful?
- What are the potential drawbacks or limitations?
3. Video Tutorials
While watching coding tutorials, pause regularly to ask yourself questions about the content. Don’t just follow along blindly – challenge yourself to predict what the instructor will do next or question why they’re using a particular approach.
4. Coding Projects
When working on personal coding projects, use the Socratic method to guide your development process. Regularly question your design decisions, implementation choices, and problem-solving approaches. This can lead to more thoughtful and robust code.
5. Peer Learning
If you’re learning with peers, use Socratic questioning in your discussions. Ask each other probing questions about your code and problem-solving approaches. This can lead to valuable insights and deeper understanding for everyone involved.
Overcoming Challenges in Self-Teaching with the Socratic Method
While the Socratic method is a powerful tool for self-teaching, it can present some challenges. Here are some common obstacles and how to overcome them:
1. Knowing What Questions to Ask
Challenge: You might struggle to come up with relevant, probing questions, especially when you’re new to a topic.
Solution: Start with basic questions like “What?”, “Why?”, and “How?”. As you gain more knowledge, your questions will naturally become more sophisticated. You can also look at example questions in coding tutorials or textbooks for inspiration.
2. Dealing with Uncertainty
Challenge: The Socratic method often leads to more questions than answers, which can be frustrating.
Solution: Embrace uncertainty as a part of the learning process. Remember that even experienced programmers don’t have all the answers. Use your uncertainties as motivation to dig deeper and do more research.
3. Maintaining Motivation
Challenge: Constantly questioning yourself can be mentally taxing and might affect your motivation.
Solution: Balance your questioning with periods of implementation and practice. Celebrate small victories and remind yourself that this process is making you a better programmer in the long run.
4. Lack of Immediate Feedback
Challenge: Unlike in a classroom setting, you don’t have a teacher to confirm if your questions or conclusions are on the right track.
Solution: Utilize online coding communities, forums, or AI-powered coding assistants for feedback. Platforms like Stack Overflow or GitHub Discussions can be great places to pose your questions and get input from experienced developers.
5. Depth vs. Breadth
Challenge: The Socratic method encourages deep dives into topics, which might slow down your overall progress through a curriculum.
Solution: Strike a balance between depth and breadth. Use the Socratic method to explore core concepts in depth, but be willing to move on once you’ve gained a solid understanding. You can always return to topics later for a deeper dive.
Preparing for Technical Interviews with the Socratic Method
The Socratic method can be particularly valuable when preparing for technical interviews, especially for positions at major tech companies. Here’s how you can leverage this approach:
1. Analyzing Problem Statements
When faced with a coding problem in an interview, use Socratic questioning to break down the problem statement:
- What are the key components of this problem?
- What assumptions am I making about the input or constraints?
- How does this problem relate to concepts I’ve studied before?
2. Exploring Multiple Solutions
Before diving into coding, question yourself about different approaches:
- What are the possible ways to solve this problem?
- What are the trade-offs between these approaches?
- How would the choice of data structure affect the solution?
3. Optimizing Your Solution
After implementing a solution, use Socratic questioning to optimize it:
- Can I improve the time or space complexity?
- Are there any edge cases I haven’t considered?
- How would this solution scale with larger inputs?
4. Explaining Your Thought Process
Interviewers often want to hear your thought process. The Socratic method trains you to articulate your thinking clearly:
- Why did I choose this particular approach?
- What were the key decisions I made in my implementation?
- How would I adapt this solution for different constraints?
Conclusion: Embracing the Socratic Method in Your Coding Journey
The Socratic method is a powerful tool for self-directed learning in coding. By constantly questioning, challenging assumptions, and exploring alternatives, you develop a deeper understanding of programming concepts and enhance your problem-solving skills. This approach not only helps you write better code but also prepares you for the kind of analytical thinking required in technical interviews and real-world software development.
Remember, the goal of the Socratic method is not to find easy answers, but to develop a mindset of curiosity and critical thinking. Embrace the questions, enjoy the process of discovery, and watch as your coding skills grow exponentially.
As you continue your coding education, whether through platforms like AlgoCademy, personal projects, or interview preparation, make the Socratic method a core part of your learning strategy. Question everything, challenge your understanding, and never stop exploring. In the dynamic world of programming, this inquiring mindset will be your greatest asset, driving continuous learning and growth throughout your career.