Why You Should Spend Time Learning ‘Hard’ Languages Like C++
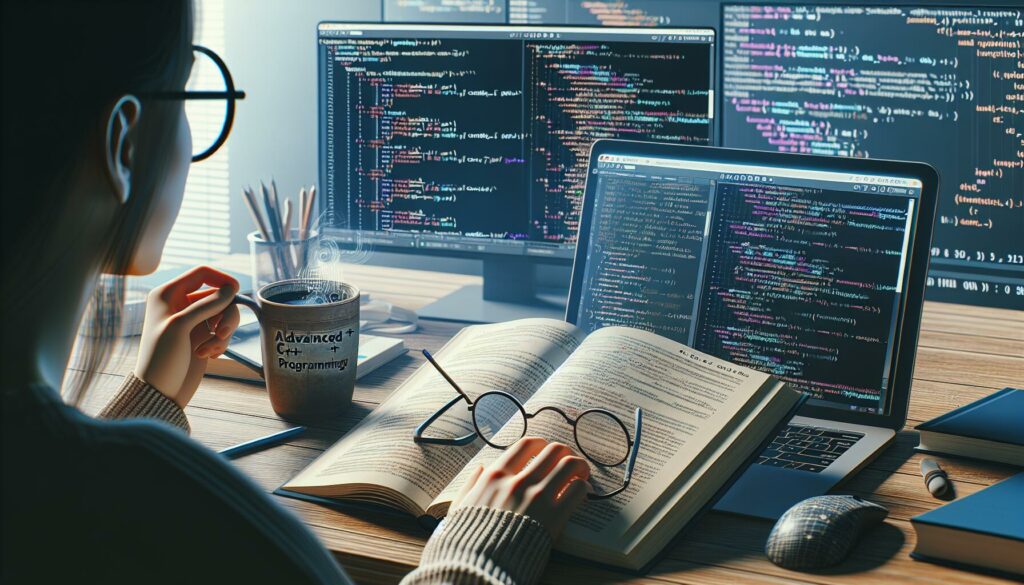
In the ever-evolving world of programming, there’s a constant debate about which languages are worth learning. While popular languages like Python and JavaScript often take the spotlight for their ease of use and widespread adoption, there’s a compelling case to be made for investing time in learning “hard” languages like C++. In this comprehensive guide, we’ll explore why dedicating time to mastering C++ can be a game-changer for your programming career, especially if you’re aiming for top-tier tech companies or looking to deepen your understanding of computer science fundamentals.
The Power and Complexity of C++
C++ is often regarded as one of the most powerful and versatile programming languages in existence. Created by Bjarne Stroustrup in 1979 as an extension of C, C++ has since evolved into a multi-paradigm language that supports procedural, object-oriented, and generic programming. Its complexity is both a blessing and a curse – while it can be challenging to learn, it offers unparalleled control over system resources and performance.
Key Features of C++
- Low-level memory manipulation
- High performance and efficiency
- Object-oriented programming support
- Extensive standard library
- Template metaprogramming
- Multi-paradigm approach
These features make C++ an excellent choice for system programming, game development, embedded systems, and high-performance applications. But why should you invest time in learning such a complex language? Let’s dive into the reasons.
1. Deep Understanding of Computer Architecture
Learning C++ forces you to understand how computers work at a fundamental level. Unlike higher-level languages that abstract away many details, C++ requires you to manage memory manually, understand pointers, and deal with low-level system interactions. This knowledge is invaluable for several reasons:
- Better problem-solving skills: Understanding memory management helps you write more efficient code in any language.
- Debugging prowess: Knowledge of low-level operations makes it easier to identify and fix complex bugs.
- Performance optimization: You’ll gain insights into how to write faster, more efficient code across all languages.
For example, consider this simple C++ code that demonstrates manual memory management:
int* createArray(int size) {
int* arr = new int[size]; // Allocate memory
for(int i = 0; i < size; i++) {
arr[i] = i;
}
return arr;
}
int main() {
int* myArray = createArray(5);
// Use the array
delete[] myArray; // Free the allocated memory
return 0;
}
This example illustrates how C++ gives you direct control over memory allocation and deallocation, a concept that’s hidden in many higher-level languages but crucial for understanding efficient resource management.
2. Enhanced Problem-Solving Skills
C++ is known for its steep learning curve, which can be frustrating at first but ultimately leads to improved problem-solving abilities. The challenges you face when working with C++ often require you to think more critically about your code structure and algorithm design. This mental exercise translates well to other programming tasks and languages.
Key areas where C++ enhances problem-solving skills include:
- Algorithm implementation: C++ forces you to think about the details of algorithm implementation, improving your overall algorithmic thinking.
- Data structure design: Creating custom data structures in C++ deepens your understanding of how they work under the hood.
- Performance optimization: The need to write efficient C++ code hones your ability to optimize algorithms and data structures.
Consider this example of implementing a simple linked list in C++:
struct Node {
int data;
Node* next;
Node(int d) : data(d), next(nullptr) {}
};
class LinkedList {
private:
Node* head;
public:
LinkedList() : head(nullptr) {}
void insert(int data) {
Node* newNode = new Node(data);
newNode->next = head;
head = newNode;
}
// Other methods like delete, display, etc.
};
Implementing data structures like this from scratch helps you understand their inner workings, which is crucial for efficient algorithm design and problem-solving in any programming context.
3. Preparation for High-Performance Computing
In an era where processing power is at a premium and efficiency is key, C++ remains a go-to language for high-performance computing. Learning C++ prepares you for scenarios where every CPU cycle and byte of memory counts. This is particularly relevant in fields such as:
- Game development
- Financial modeling and trading systems
- Scientific simulations
- Real-time systems
- Embedded programming
The performance benefits of C++ come from its close-to-the-metal nature and features like inline assembly, which allows for fine-grained optimizations. Here’s a simple example demonstrating the use of inline assembly in C++:
#include <iostream>
int addNumbers(int a, int b) {
int result;
__asm__ (
"movl %1, %%eax;"
"addl %2, %%eax;"
"movl %%eax, %0;"
: "=r" (result)
: "r" (a), "r" (b)
);
return result;
}
int main() {
std::cout << "Sum: " << addNumbers(5, 3) << std::endl;
return 0;
}
While this level of optimization is rarely necessary in everyday programming, understanding these capabilities gives you a significant edge in performance-critical applications.
4. Improved Coding Practices
C++ enforces stricter coding practices compared to many other languages. This strictness, while sometimes frustrating, ultimately leads to better coding habits that carry over to other languages. Some key areas where C++ promotes good practices include:
- Strong typing: C++ requires explicit type declarations, reducing runtime errors and promoting clearer code.
- Resource management: Concepts like RAII (Resource Acquisition Is Initialization) teach efficient and safe resource handling.
- Code organization: C++’s separation of interface and implementation (header and source files) encourages modular design.
Here’s an example demonstrating RAII in C++:
#include <iostream>
#include <memory>
class Resource {
public:
Resource() { std::cout << "Resource acquired\n"; }
~Resource() { std::cout << "Resource released\n"; }
void use() { std::cout << "Resource used\n"; }
};
int main() {
{
std::unique_ptr<Resource> res = std::make_unique<Resource>();
res->use();
} // Resource automatically released here
return 0;
}
This pattern of automatic resource management is a powerful concept that can be applied to many programming scenarios, leading to more robust and maintainable code.
5. Versatility in Career Opportunities
Proficiency in C++ opens doors to a wide range of career opportunities. Many high-profile tech companies, including those in the FAANG (Facebook, Amazon, Apple, Netflix, Google) group, use C++ extensively in their core systems. Some areas where C++ skills are highly valued include:
- Systems programming
- Game development
- Financial technology
- Embedded systems
- Automotive software
- Aerospace and defense
Moreover, the problem-solving skills and deep understanding of computer systems gained from learning C++ make you a more versatile programmer, capable of adapting to various programming challenges and languages.
6. Foundation for Learning Other Languages
Once you’ve mastered C++, learning other programming languages becomes significantly easier. The concepts you learn in C++, such as memory management, pointers, and object-oriented programming, provide a solid foundation for understanding how other languages work under the hood. This knowledge allows you to:
- Pick up new languages more quickly
- Understand the trade-offs between different programming paradigms
- Appreciate the design decisions behind various language features
For instance, understanding how C++ manages memory manually can help you appreciate garbage collection in languages like Java or Python, making you more aware of potential performance implications.
7. Preparation for Technical Interviews
Many top tech companies, especially those focusing on system-level programming or high-performance applications, often include C++ questions in their technical interviews. Even if the role you’re applying for doesn’t specifically require C++, demonstrating proficiency in such a complex language can set you apart from other candidates. It shows:
- Your ability to handle complex programming concepts
- Your dedication to mastering challenging skills
- Your deep understanding of computer science fundamentals
Here’s an example of a C++ interview question you might encounter:
// Implement a function to reverse a linked list
ListNode* reverseList(ListNode* head) {
ListNode* prev = nullptr;
ListNode* current = head;
ListNode* next = nullptr;
while (current != nullptr) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
return prev;
}
Being able to implement such algorithms efficiently in C++ demonstrates not only your coding skills but also your understanding of data structures and memory management.
8. Continuous Learning and Community
The C++ community is known for its dedication to continuous improvement and learning. By engaging with this community, you’ll benefit from:
- Regular language updates: C++ undergoes standardization processes, with new features added periodically.
- Rich ecosystem of libraries and tools
- Active community forums and resources
- Opportunities to contribute to open-source projects
Staying active in the C++ community keeps your skills sharp and exposes you to cutting-edge programming techniques and best practices.
9. Cross-Platform Development
C++ offers excellent support for cross-platform development. With proper design and use of platform-independent libraries, you can write code that runs on multiple operating systems and hardware architectures. This capability is particularly valuable in today’s diverse computing environment, where applications need to run on various platforms.
Here’s a simple example of cross-platform code using the standard C++ library:
#include <iostream>
#include <fstream>
int main() {
std::ofstream file("example.txt");
if (file.is_open()) {
file << "Hello, cross-platform world!" << std::endl;
file.close();
std::cout << "File written successfully." << std::endl;
} else {
std::cerr << "Unable to open file." << std::endl;
}
return 0;
}
This code will work on any platform that supports standard C++, demonstrating the language’s portability.
10. Future-Proofing Your Skills
Despite being an older language, C++ continues to evolve and remain relevant. Its performance, control, and versatility ensure its place in the programming world for years to come. Learning C++ is an investment in future-proof skills that will likely remain valuable throughout your career.
Recent updates to the language, such as C++20, have introduced features like concepts and modules, further enhancing its capabilities:
// C++20 concept example
#include <concepts>
template<typename T>
concept Numeric = std::is_arithmetic_v<T>;
template<Numeric T>
T add(T a, T b) {
return a + b;
}
int main() {
std::cout << add(5, 3) << std::endl; // Works
// std::cout << add("Hello", "World") << std::endl; // Compilation error
return 0;
}
This example demonstrates how modern C++ features can lead to more robust and expressive code, showcasing the language’s ongoing evolution.
Conclusion
While learning C++ can be challenging, the benefits far outweigh the initial difficulties. From deepening your understanding of computer systems to enhancing your problem-solving skills and opening up diverse career opportunities, C++ offers a wealth of advantages for the dedicated programmer.
As you embark on your journey to master C++, remember that the skills you gain will not only make you a better C++ programmer but a better programmer overall. The deep understanding of computer science principles, the ability to write efficient and performant code, and the problem-solving skills you develop will serve you well throughout your programming career, regardless of the languages or technologies you work with in the future.
So, embrace the challenge, dive into the complexities of C++, and watch as your programming skills reach new heights. The journey may be tough, but the rewards are immeasurable. Happy coding!