Using Machine Learning to Improve Your Coding Interview Prep
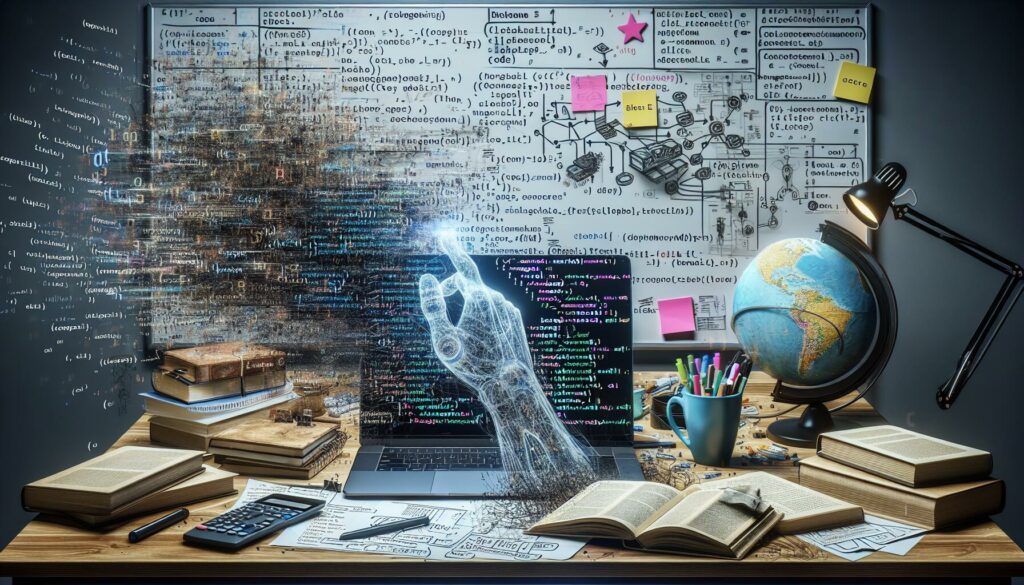
In today’s competitive tech landscape, mastering coding interviews is crucial for landing your dream job at top companies like Google, Amazon, or Facebook. As the complexity of interview questions continues to evolve, traditional preparation methods may fall short. This is where machine learning (ML) comes into play, offering innovative ways to enhance your coding interview preparation. In this comprehensive guide, we’ll explore how you can leverage ML techniques to supercharge your interview prep and increase your chances of success.
Understanding the Coding Interview Landscape
Before diving into how machine learning can help, it’s essential to understand the current state of coding interviews. Top tech companies often use algorithmic problems to assess candidates’ problem-solving skills, coding proficiency, and ability to think on their feet. These interviews typically cover a range of topics, including:
- Data Structures (arrays, linked lists, trees, graphs)
- Algorithms (sorting, searching, dynamic programming)
- System Design
- Object-Oriented Programming
- Time and Space Complexity Analysis
The challenge lies not only in mastering these topics but also in applying this knowledge under pressure. This is where machine learning can provide a significant advantage.
How Machine Learning Can Enhance Your Prep
Machine learning offers several ways to improve your coding interview preparation:
1. Personalized Problem Recommendations
ML algorithms can analyze your performance on practice problems and recommend new challenges tailored to your skill level and areas for improvement. This personalized approach ensures that you’re always working on relevant problems, maximizing the efficiency of your study time.
2. Intelligent Progress Tracking
By leveraging ML, you can gain insights into your learning patterns and progress over time. These algorithms can identify trends in your performance, highlighting areas where you’re improving and those that need more attention.
3. Automated Code Review
ML-powered code review tools can provide instant feedback on your solutions, pointing out potential optimizations, style improvements, and common pitfalls. This immediate feedback loop accelerates your learning and helps you develop better coding habits.
4. Predictive Interview Simulation
Advanced ML models can simulate realistic interview scenarios, adapting the difficulty and types of questions based on your responses. This helps you get comfortable with the pressure and pacing of actual interviews.
5. Natural Language Processing for Concept Understanding
NLP techniques can help you better understand and remember complex algorithmic concepts by breaking them down into more digestible explanations tailored to your learning style.
Implementing ML in Your Prep Routine
Now that we’ve covered the potential benefits, let’s explore how you can practically incorporate ML into your coding interview preparation:
1. Utilize ML-Powered Platforms
Several online platforms leverage ML to enhance coding practice. For example, AlgoCademy uses AI to provide personalized learning paths and real-time feedback on your code. Here’s how you might interact with such a platform:
// Example interaction with an ML-powered coding platform
// User submits a solution to a binary search problem
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
// ML system analyzes the code and provides feedback
ML_FEEDBACK = {
"efficiency": "Good use of binary search algorithm. Time complexity: O(log n)",
"style": "Consider using const for variables that don't change",
"suggestion": "Try implementing this recursively for practice",
"next_challenge": "Based on your performance, try 'Search in Rotated Sorted Array'"
}
2. Implement Your Own ML Models
For those with ML experience, creating your own models can be an excellent way to tailor your prep and simultaneously showcase your ML skills. Here’s a simple example of how you might use Python and scikit-learn to build a model that predicts the difficulty of coding problems:
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.naive_bayes import MultinomialNB
from sklearn.metrics import accuracy_score
# Load and prepare your data
data = pd.read_csv('coding_problems.csv')
X = data['problem_description']
y = data['difficulty_level']
# Split the data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Vectorize the text data
vectorizer = TfidfVectorizer()
X_train_vectorized = vectorizer.fit_transform(X_train)
X_test_vectorized = vectorizer.transform(X_test)
# Train a Naive Bayes classifier
clf = MultinomialNB()
clf.fit(X_train_vectorized, y_train)
# Make predictions and evaluate
y_pred = clf.predict(X_test_vectorized)
accuracy = accuracy_score(y_test, y_pred)
print(f"Model Accuracy: {accuracy:.2f}")
# Use the model to predict difficulty of a new problem
new_problem = ["Implement a function to find the longest palindromic substring in a given string."]
new_problem_vectorized = vectorizer.transform(new_problem)
predicted_difficulty = clf.predict(new_problem_vectorized)
print(f"Predicted Difficulty: {predicted_difficulty[0]}")
This example demonstrates how you can use ML to gain insights into problem difficulty, helping you structure your practice more effectively.
3. Leverage Natural Language Processing (NLP)
NLP can be a powerful tool for understanding and generating coding problem descriptions. Here’s an example of how you might use the transformers library to generate coding problem descriptions:
from transformers import GPT2LMHeadModel, GPT2Tokenizer
# Load pre-trained model and tokenizer
model = GPT2LMHeadModel.from_pretrained("gpt2")
tokenizer = GPT2Tokenizer.from_pretrained("gpt2")
# Generate a coding problem description
prompt = "Create a coding problem that involves"
input_ids = tokenizer.encode(prompt, return_tensors='pt')
output = model.generate(input_ids, max_length=100, num_return_sequences=1, no_repeat_ngram_size=2)
generated_text = tokenizer.decode(output[0], skip_special_tokens=True)
print(generated_text)
This script can help you generate unique problem descriptions to practice with, expanding your exposure to different problem types.
Advanced ML Techniques for Interview Prep
As you become more comfortable with basic ML applications, you can explore more advanced techniques to further enhance your preparation:
1. Reinforcement Learning for Problem-Solving Strategies
Reinforcement Learning (RL) can be used to develop and refine problem-solving strategies. By framing the coding process as a series of decisions, an RL agent can learn optimal approaches to tackling different types of problems.
import gym
import numpy as np
from gym import spaces
from stable_baselines3 import PPO
class CodingEnvironment(gym.Env):
def __init__(self):
super(CodingEnvironment, self).__init__()
self.action_space = spaces.Discrete(4) # Example: 4 possible actions
self.observation_space = spaces.Box(low=0, high=1, shape=(10,), dtype=np.float32)
def step(self, action):
# Implement the logic for taking an action and returning the new state, reward, etc.
pass
def reset(self):
# Reset the environment to an initial state
pass
# Create the environment
env = CodingEnvironment()
# Initialize the agent
model = PPO("MlpPolicy", env, verbose=1)
# Train the agent
model.learn(total_timesteps=10000)
# Use the trained agent
obs = env.reset()
for _ in range(1000):
action, _states = model.predict(obs, deterministic=True)
obs, reward, done, info = env.step(action)
if done:
obs = env.reset()
This example sets up a basic framework for using RL to learn coding strategies. You’d need to flesh out the environment details based on your specific coding challenges.
2. Time Series Analysis for Performance Tracking
Time series analysis can help you understand trends in your performance over time. Here’s an example using the Prophet library:
from fbprophet import Prophet
import pandas as pd
import matplotlib.pyplot as plt
# Assume you have a DataFrame 'df' with columns 'ds' (date) and 'y' (performance metric)
df = pd.DataFrame({
'ds': pd.date_range(start='2023-01-01', periods=100),
'y': np.random.randn(100).cumsum() # Example performance data
})
model = Prophet()
model.fit(df)
future = model.make_future_dataframe(periods=30) # Forecast 30 days ahead
forecast = model.predict(future)
fig1 = model.plot(forecast)
fig2 = model.plot_components(forecast)
plt.show()
This script helps you visualize your performance trends and make predictions about your future progress.
3. Clustering for Problem Categorization
Clustering algorithms can help you identify patterns in coding problems, grouping similar challenges together. This can be useful for understanding the landscape of potential interview questions:
from sklearn.cluster import KMeans
from sklearn.feature_extraction.text import TfidfVectorizer
# Assume 'problems' is a list of problem descriptions
vectorizer = TfidfVectorizer(stop_words='english')
X = vectorizer.fit_transform(problems)
kmeans = KMeans(n_clusters=5, random_state=42)
kmeans.fit(X)
# Get the most common words in each cluster
order_centroids = kmeans.cluster_centers_.argsort()[:, ::-1]
terms = vectorizer.get_feature_names()
for i in range(5):
print(f"Cluster {i}:")
for ind in order_centroids[i, :10]:
print(f" {terms[ind]}")
print()
This clustering approach can help you identify common themes or categories in coding interview questions, allowing you to focus your studies more effectively.
Ethical Considerations and Limitations
While ML can significantly enhance your coding interview preparation, it’s important to consider some ethical implications and limitations:
- Over-reliance on AI: Don’t let ML tools replace your own critical thinking and problem-solving skills. They should supplement, not substitute, your learning.
- Data Privacy: If you’re using platforms that collect your coding data, ensure they have robust privacy policies in place.
- Bias in ML Models: Be aware that ML models can perpetuate biases present in their training data. This could potentially lead to skewed recommendations or assessments.
- The Human Element: Remember that coding interviews also assess communication skills and cultural fit, which ML can’t fully prepare you for.
Conclusion
Machine learning offers powerful tools to enhance your coding interview preparation, from personalized problem recommendations to advanced performance analysis. By incorporating ML techniques into your study routine, you can gain valuable insights, optimize your learning path, and potentially increase your chances of success in technical interviews.
However, it’s crucial to use these tools thoughtfully and in conjunction with traditional studying methods. The goal is to leverage ML to become a better problem solver and coder, not to find shortcuts or cheat the system.
As you embark on your journey to master coding interviews, remember that the ultimate aim is not just to pass the interview, but to become a skilled and confident software engineer. Use ML as a means to that end, always focusing on deepening your understanding and honing your problem-solving abilities.
Happy coding, and best of luck with your interviews!