How to Automate Your Learning Process as a Developer
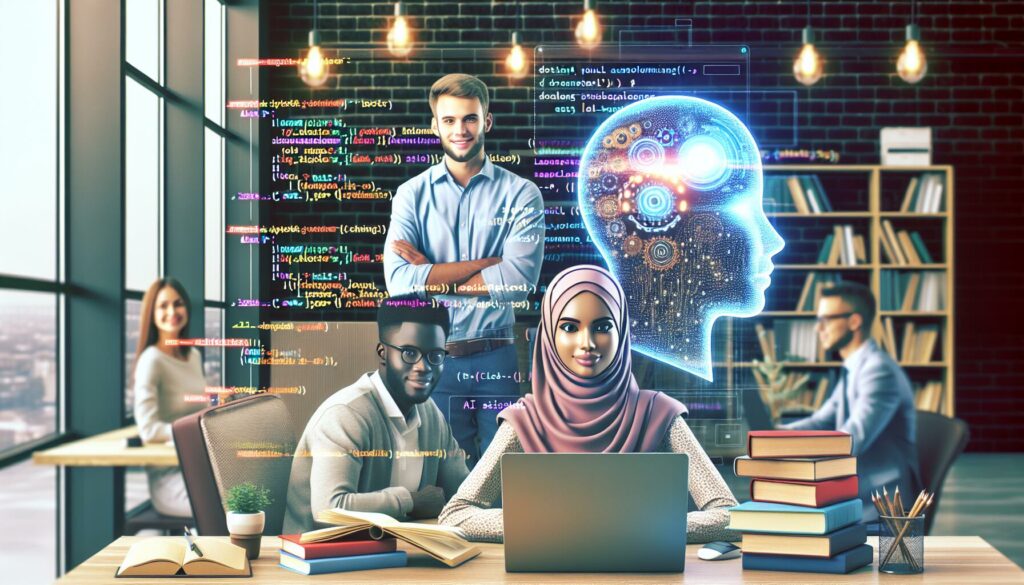
In the ever-evolving world of software development, staying up-to-date with the latest technologies, frameworks, and best practices is crucial. As a developer, continuous learning is not just a choice; it’s a necessity. However, with the vast amount of information available and the rapid pace of change in the tech industry, it can be overwhelming to manage your learning process effectively. This is where automation comes in handy. By automating various aspects of your learning journey, you can streamline your education, save time, and ensure that you’re always on top of the latest trends in the field.
In this comprehensive guide, we’ll explore various strategies and tools to automate your learning process as a developer. We’ll cover everything from setting up automated news feeds to leveraging AI-powered learning assistants. By the end of this article, you’ll have a toolkit of techniques to supercharge your learning and stay ahead in the competitive world of software development.
1. Set Up Automated News Feeds
One of the first steps in automating your learning process is to set up automated news feeds. This ensures that you’re always informed about the latest developments in your areas of interest without having to manually search for information.
1.1. RSS Feeds
RSS (Really Simple Syndication) feeds are an excellent way to aggregate content from multiple sources into a single, easily digestible format. Here’s how to set up RSS feeds:
- Choose an RSS reader: Popular options include Feedly, Inoreader, and NewsBlur.
- Identify relevant tech blogs, news sites, and official documentation pages that offer RSS feeds.
- Add these feeds to your chosen RSS reader.
- Set up categories or folders to organize your feeds (e.g., “JavaScript”, “Python”, “DevOps”).
By dedicating just 15-20 minutes a day to scanning your RSS feeds, you can stay informed about the latest trends, updates, and best practices in your field.
1.2. Email Newsletters
Many tech publications and influential developers offer curated email newsletters. These can be an excellent source of information delivered directly to your inbox. Some popular newsletters for developers include:
- JavaScript Weekly
- Python Weekly
- DevOps’ish
- Hacker Newsletter
To avoid cluttering your primary inbox, consider creating a separate email address specifically for these newsletters. You can then set up filters to automatically categorize and prioritize these emails.
2. Leverage AI-Powered Learning Assistants
Artificial Intelligence has made significant strides in recent years, and there are now several AI-powered tools that can assist in your learning process. These tools can provide personalized recommendations, answer questions, and even help you practice coding.
2.1. ChatGPT and GPT-4
OpenAI’s language models, such as ChatGPT and GPT-4, can be powerful allies in your learning journey. You can use these AI assistants to:
- Explain complex programming concepts
- Debug code snippets
- Suggest best practices and design patterns
- Generate practice problems
- Provide code reviews
To make the most of these AI assistants, try to ask specific, well-formulated questions. For example, instead of asking “How do I use Python?”, you could ask “Can you explain the difference between list comprehension and traditional for loops in Python, and provide examples of when to use each?”
2.2. GitHub Copilot
GitHub Copilot is an AI-powered code completion tool that can significantly speed up your coding process and help you learn new programming patterns. While it’s primarily designed to assist with coding, it can also be a valuable learning tool:
- Observe how Copilot completes your code to learn new syntax and patterns
- Use Copilot’s suggestions as a starting point, then research and understand why it made those suggestions
- Experiment with different ways of writing comments to see how Copilot interprets your intent
Remember, while AI tools can be incredibly helpful, they should be used as aids to your learning, not replacements for understanding core concepts.
3. Automate Your Coding Practice
Regular coding practice is essential for improving your skills. By automating aspects of your practice routine, you can ensure consistency and track your progress more effectively.
3.1. Automated Coding Challenges
Platforms like LeetCode, HackerRank, and CodeWars offer daily coding challenges. Many of these platforms allow you to set up email reminders or integrate with your calendar to ensure you never miss a day of practice.
To automate your practice routine:
- Choose a platform and create an account
- Set up daily reminders or calendar integrations
- Commit to solving at least one problem each day
- Use the platform’s tracking features to monitor your progress
3.2. Spaced Repetition for Concept Review
Spaced repetition is a learning technique that involves reviewing information at increasing intervals. This method can be particularly effective for retaining complex programming concepts. You can automate this process using apps like Anki or RemNote:
- Create flashcards for key programming concepts, algorithms, and design patterns
- Set up a daily review schedule in your chosen app
- Let the app’s algorithm determine when you need to review each concept based on your performance
By consistently reviewing concepts using spaced repetition, you can significantly improve your long-term retention of important programming knowledge.
4. Set Up Automated Project Ideas Generator
Working on projects is one of the best ways to apply your learning and build a portfolio. However, coming up with project ideas can sometimes be challenging. You can automate this process to ensure you always have fresh ideas to work on.
4.1. Use AI for Project Idea Generation
You can use AI tools like ChatGPT to generate project ideas based on your skill level and interests. Here’s a simple prompt you can use:
"I'm a [your skill level] developer interested in [your areas of interest]. Can you suggest 5 project ideas that would help me improve my skills and would be impressive additions to my portfolio?"
You can then save these ideas in a project management tool or a simple text file for future reference.
4.2. Automated GitHub Repository Creation
Once you have a project idea, you can automate the process of setting up a new GitHub repository. Here’s a simple bash script that creates a new GitHub repository and sets it up locally:
#!/bin/bash
# Replace YOUR_GITHUB_USERNAME with your actual GitHub username
GITHUB_USERNAME="YOUR_GITHUB_USERNAME"
# Get the repository name from the user
echo "Enter the name of your new repository:"
read REPO_NAME
# Create the repository on GitHub
curl -u $GITHUB_USERNAME https://api.github.com/user/repos -d "{\"name\":\"$REPO_NAME\"}"
# Create a new directory with the repository name
mkdir $REPO_NAME
cd $REPO_NAME
# Initialize the local repository
git init
echo "# $REPO_NAME" > README.md
git add README.md
git commit -m "Initial commit"
# Add the remote repository
git remote add origin https://github.com/$GITHUB_USERNAME/$REPO_NAME.git
# Push to the remote repository
git push -u origin master
echo "Repository $REPO_NAME created and initialized!"
Save this script as a .sh file, make it executable with chmod +x script_name.sh
, and run it whenever you want to start a new project.
5. Automate Your Learning Environment Setup
Having a consistent and efficient learning environment is crucial for productive learning. You can automate the setup of your development environment to ensure you always have the tools you need at your fingertips.
5.1. Dotfiles Management
Dotfiles are configuration files for various tools and applications, typically hidden in your home directory (hence the name, as they often start with a dot). By managing your dotfiles in a Git repository, you can easily set up your preferred environment on any machine:
- Create a GitHub repository for your dotfiles
- Add your configuration files (e.g., .vimrc, .bashrc, .gitconfig) to this repository
- Create a script that symlinks these files to your home directory
Here’s a simple script to manage your dotfiles:
#!/bin/bash
DOTFILES_DIR="$HOME/dotfiles"
# Create symlinks
ln -sf $DOTFILES_DIR/.vimrc $HOME/.vimrc
ln -sf $DOTFILES_DIR/.bashrc $HOME/.bashrc
ln -sf $DOTFILES_DIR/.gitconfig $HOME/.gitconfig
echo "Dotfiles linked successfully!"
5.2. Automated Software Installation
You can create scripts to automatically install and configure the software you commonly use for development. Here’s an example script for macOS using Homebrew:
#!/bin/bash
# Install Homebrew if not already installed
if ! command -v brew &>/dev/null; then
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
fi
# Update Homebrew
brew update
# Install common development tools
brew install git
brew install node
brew install python
brew install vscode
# Install your favorite IDEs or text editors
brew install --cask visual-studio-code
brew install --cask intellij-idea
echo "Development environment setup complete!"
You can customize this script based on your preferred tools and languages.
6. Implement a Learning Management System
A Learning Management System (LMS) can help you organize your learning goals, track your progress, and manage your learning resources. While there are many commercial LMS options available, you can create a simple automated system using tools you likely already use.
6.1. Use Trello for Learning Management
Trello is a flexible project management tool that can be adapted for learning management. Here’s how you can set it up:
- Create a new Trello board for your learning journey
- Set up lists for different stages of learning (e.g., “To Learn”, “In Progress”, “Completed”)
- Create cards for each topic or skill you want to learn
- Use labels to categorize topics (e.g., “Frontend”, “Backend”, “DevOps”)
- Attach relevant resources (articles, videos, courses) to each card
- Set due dates for learning goals
You can automate certain aspects of your Trello board using Trello’s built-in automation features or by integrating with tools like Zapier or IFTTT.
6.2. Automate Progress Tracking
To automate progress tracking, you can use Google Sheets and the Google Apps Script. Here’s a simple script that logs your daily learning activities:
function logLearningActivity() {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
var date = new Date();
var ui = SpreadsheetApp.getUi();
var response = ui.prompt('Log Learning Activity', 'What did you learn today?', ui.ButtonSet.OK_CANCEL);
if (response.getSelectedButton() == ui.Button.OK) {
var activity = response.getResponseText();
sheet.appendRow([date, activity]);
ui.alert('Activity logged successfully!');
}
}
function onOpen() {
var ui = SpreadsheetApp.getUi();
ui.createMenu('Learning Log')
.addItem('Log Activity', 'logLearningActivity')
.addToUi();
}
This script adds a custom menu to your Google Sheet that allows you to quickly log your learning activities. You can then use Google Sheets’ built-in features to visualize your progress over time.
7. Automate Your Note-Taking Process
Effective note-taking is crucial for retaining information and referring back to what you’ve learned. You can automate aspects of your note-taking process to make it more efficient and organized.
7.1. Use Templates for Consistent Note Structure
Create templates for different types of notes (e.g., concept explanations, code snippets, project ideas) and automate their creation. If you’re using a note-taking app like Notion, you can create template buttons that automatically generate new pages with your predefined structure.
Here’s an example of a simple note template for learning a new programming concept:
# [Concept Name]
## Definition
[Brief definition of the concept]
## Key Points
- [Point 1]
- [Point 2]
- [Point 3]
## Code Example
```[language]
[code snippet]
```
## Use Cases
- [Use case 1]
- [Use case 2]
## Resources
- [Link to resource 1]
- [Link to resource 2]
## Review Questions
1. [Question 1]
2. [Question 2]
## Personal Notes
[Any additional thoughts or connections]
7.2. Automated Code Snippet Management
For managing code snippets, you can use tools like GitHub Gists or specialized apps like SnippetsLab. To automate the process of saving code snippets, you can create a simple script that allows you to quickly save snippets from your clipboard.
Here’s a Python script that saves clipboard content as a GitHub Gist:
import requests
import pyperclip
import os
GITHUB_TOKEN = os.environ.get('GITHUB_TOKEN')
def create_gist(content):
url = 'https://api.github.com/gists'
headers = {
'Authorization': f'token {GITHUB_TOKEN}'
}
data = {
'description': 'Code snippet',
'public': False,
'files': {
'snippet.txt': {
'content': content
}
}
}
response = requests.post(url, json=data, headers=headers)
if response.status_code == 201:
print(f"Gist created successfully: {response.json()['html_url']}")
else:
print(f"Failed to create Gist: {response.text}")
if __name__ == '__main__':
snippet = pyperclip.paste()
create_gist(snippet)
To use this script, you’ll need to set up a GitHub personal access token and store it as an environment variable. You can then run this script whenever you want to save a code snippet from your clipboard as a Gist.
8. Set Up Automated Code Reviews
Code reviews are an excellent way to improve your coding skills and learn best practices. While human code reviews are invaluable, you can automate certain aspects of the code review process to catch common issues and enforce coding standards.
8.1. Use Linters and Static Analysis Tools
Linters and static analysis tools can automatically check your code for style issues, potential bugs, and adherence to best practices. Here’s how to set up automated linting for a Python project using flake8:
- Install flake8:
pip install flake8
- Create a
.flake8
configuration file in your project root:
[flake8]
max-line-length = 88
extend-ignore = E203, E266, E501, W503
max-complexity = 18
select = B,C,E,F,W,T4,B9
- Set up a pre-commit hook to run flake8 before each commit. Create a file named
.pre-commit-config.yaml
in your project root:
repos:
- repo: https://github.com/pre-commit/pre-commit-hooks
rev: v3.2.0
hooks:
- id: trailing-whitespace
- id: end-of-file-fixer
- id: check-yaml
- id: check-added-large-files
- repo: https://github.com/pycqa/flake8
rev: 3.9.2
hooks:
- id: flake8
- Install pre-commit:
pip install pre-commit
- Set up the git hook scripts:
pre-commit install
Now, flake8 will automatically run before each commit, catching style issues and potential bugs.
8.2. Implement Continuous Integration (CI)
Setting up a CI pipeline can automate the process of running tests, linters, and other checks whenever you push code to your repository. Here’s an example of a simple GitHub Actions workflow for a Python project:
name: Python CI
on: [push, pull_request]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.x'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install flake8 pytest
if [ -f requirements.txt ]; then pip install -r requirements.txt; fi
- name: Lint with flake8
run: |
flake8 .
- name: Test with pytest
run: |
pytest
Save this as .github/workflows/python-ci.yml
in your repository. This workflow will run flake8 and your tests every time you push code or open a pull request.
9. Automate Your Learning Reflection Process
Reflection is a crucial part of the learning process. It helps you consolidate your knowledge, identify areas for improvement, and track your progress. You can automate aspects of your reflection process to make it a consistent part of your routine.
9.1. Set Up Automated Reflection Prompts
Use a tool like IFTTT (If This Then That) to send yourself daily or weekly reflection prompts. Here’s how to set it up:
- Sign up for an IFTTT account
- Create a new applet
- For the “If This” part, choose the “Date & Time” service and select “Every day at”
- For the “Then That” part, choose the “Email” service and select “Send me an email”
- In the email body, include reflection prompts such as:
- What did I learn today?
- What challenges did I face and how did I overcome them?
- How can I apply what I learned to my projects?
- What do I want to learn next?
9.2. Use Automated Mood and Productivity Tracking
Tracking your mood and productivity can provide valuable insights into your learning process. You can use apps like Exist.io or RescueTime to automatically track various aspects of your day and correlate them with your learning activities.
For a more customized solution, you can create a simple script that prompts you for your mood and productivity levels and logs them to a spreadsheet. Here’s a Python script that does this:
import gspread
from oauth2client.service_account import ServiceAccountCredentials
from datetime import datetime
# Set up Google Sheets API
scope = ['https://spreadsheets.google.com/feeds', 'https://www.googleapis.com/auth/drive']
creds = ServiceAccountCredentials.from_json_keyfile_name('path/to/your/credentials.json', scope)
client = gspread.authorize(creds)
# Open the spreadsheet
sheet = client.open("Learning Reflection Log").sheet1
# Get user input
date = datetime.now().strftime("%Y-%m-%d")
mood = input("Rate your mood today (1-10): ")
productivity = input("Rate your productivity today (1-10): ")
reflection = input("Brief reflection on today's learning: ")
# Append to spreadsheet
sheet.append_row([date, mood, productivity, reflection])
print("Reflection logged successfully!")
To use this script, you’ll need to set up a Google Sheets API credentials file and install the required Python packages (gspread
and oauth2client
).
10. Leverage Automated Learning Recommendations
As you progress in your learning journey, it can be helpful to receive personalized recommendations for what to learn next. While human mentorship is invaluable, you can also leverage automated systems to provide learning suggestions.
10.1. Use AI-Powered Learning Platforms
Platforms like Coursera and Udacity use AI algorithms to recommend courses based on your learning history and goals. To make the most of these recommendations:
- Keep your profile updated with your current skills and learning objectives
- Complete courses and provide feedback to improve the accuracy of recommendations
- Regularly check the recommended courses and add relevant ones to your learning plan
10.2. Create a Custom Recommendation System
You can create a simple recommendation system using your learning history and a predefined skill tree. Here’s a basic Python script that suggests skills to learn based on your current skillset:
import random
skill_tree = {
'Python': ['Django', 'Flask', 'FastAPI', 'Data Analysis', 'Machine Learning'],
'JavaScript': ['React', 'Vue', 'Angular', 'Node.js', 'Express'],
'Database': ['SQL', 'MongoDB', 'PostgreSQL', 'Redis', 'Cassandra'],
'DevOps': ['Docker', 'Kubernetes', 'Jenkins', 'Ansible', 'Terraform'],
'Cloud': ['AWS', 'Azure', 'Google Cloud', 'Heroku', 'DigitalOcean']
}
def get_recommendations(current_skills, num_recommendations=3):
recommendations = []
for category, skills in skill_tree.items():
new_skills = [skill for skill in skills if skill not in current_skills]
if new_skills:
recommendations.extend(new_skills)
return random.sample(recommendations, min(num_recommendations, len(recommendations)))
# Example usage
my_skills = ['Python', 'JavaScript', 'SQL', 'Docker']
recommendations = get_recommendations(my_skills)
print(f"Based on your current skills, you might want to learn: {', '.join(recommendations)}")
This script provides a starting point that you can expand upon. You could enhance it by adding difficulty levels to skills, incorporating your learning history, or integrating it with your learning management system.
Conclusion
Automating your learning process as a developer can significantly enhance your efficiency and ensure that you’re consistently improving your skills. From setting up automated news feeds to leveraging AI-powered learning assistants, there are numerous ways to streamline your education and stay ahead in the fast-paced world of software development.
Remember, automation should enhance your learning process, not replace critical thinking and hands-on practice. Use these tools and techniques as aids to support your learning journey, but always strive to deeply understand the concepts you’re studying.
By implementing even a few of these automation strategies, you can create a more structured, consistent, and effective learning routine. This will not only help you acquire new skills more quickly but also ensure that you’re always prepared for the next challenge in your development career.
Happy learning, and may your automated learning process lead you to new heights in your development journey!