The Importance of Learning Version Control (Git) Early in Your Coding Journey
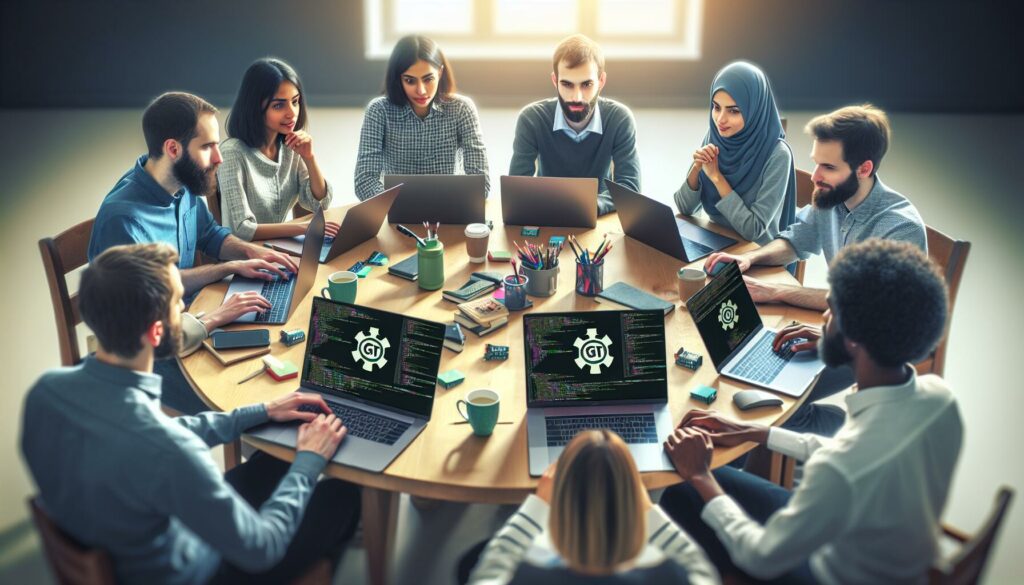
When embarking on your coding journey, it’s easy to get caught up in learning programming languages, algorithms, and data structures. While these are undoubtedly crucial skills, there’s one tool that often gets overlooked by beginners but is absolutely essential in the professional world of software development: version control, specifically Git. In this comprehensive guide, we’ll explore why learning Git early in your coding journey is not just beneficial, but crucial for your success as a programmer.
What is Version Control?
Before diving into the importance of Git, let’s first understand what version control is. Version control is a system that helps track and manage changes to files over time. It allows you to recall specific versions later, revert files to a previous state, compare changes over time, see who last modified something that might be causing a problem, and more.
While version control systems are used in various fields, they are particularly crucial in software development. The most popular version control system today is Git, created by Linus Torvalds in 2005 for development of the Linux kernel.
Why Git is Essential for Beginners
Now that we understand what version control is, let’s explore why Git, in particular, is so important for beginners to learn early in their coding journey.
1. Industry Standard
Git is the de facto standard for version control in the software industry. Whether you’re aiming to work for a tech giant like Google or Facebook, or a small startup, chances are they’re using Git. Learning Git early gives you a valuable skill that’s in high demand across the industry.
2. Project History and Collaboration
Git allows you to maintain a complete history of your project. This means you can track changes, revert to previous versions if needed, and collaborate with others seamlessly. As a beginner, this might not seem important when you’re working on small personal projects, but as you progress to larger, more complex projects, especially those involving team collaboration, Git becomes indispensable.
3. Backup and Recovery
Git acts as a distributed backup system for your code. By pushing your code to remote repositories like GitHub or GitLab, you ensure that your work is safe even if your local machine fails. This peace of mind is invaluable, especially when you’re working on important projects.
4. Experimentation Without Fear
One of the best ways to learn coding is through experimentation. Git allows you to create separate branches where you can try out new ideas without affecting your main codebase. If the experiment works, you can merge it back in. If it doesn’t, you can simply discard the branch. This encourages a fearless approach to coding and learning.
5. Open Source Contribution
The open-source community is a fantastic resource for learning and improving your coding skills. Most open-source projects use Git for version control. By learning Git early, you open up opportunities to contribute to open-source projects, which can significantly boost your learning and your coding resume.
6. Code Review and Quality
Git facilitates code reviews through features like pull requests. This allows other developers to review your code before it’s merged into the main project. As a beginner, getting your code reviewed by more experienced developers is an excellent way to improve your coding skills and learn best practices.
Getting Started with Git
Now that we’ve established the importance of Git, let’s look at how you can get started with it.
1. Installation
The first step is to install Git on your machine. You can download it from the official Git website (https://git-scm.com/). The installation process is straightforward for all major operating systems.
2. Basic Configuration
After installation, you’ll need to set up your Git configuration. Open your terminal or command prompt and enter the following commands, replacing the email and name with your own:
git config --global user.email "you@example.com"
git config --global user.name "Your Name"
3. Creating a Repository
To start using Git in a project, you need to initialize a repository. Navigate to your project directory in the terminal and run:
git init
4. Basic Git Commands
Here are some basic Git commands to get you started:
git add <file>
: Adds a file to the staging areagit commit -m "Commit message"
: Commits the staged changesgit status
: Shows the status of changes as untracked, modified, or stagedgit log
: Displays a log of all commitsgit branch
: Lists all local branchesgit checkout -b <branch-name>
: Creates and switches to a new branchgit merge <branch-name>
: Merges the specified branch into the current branch
5. Remote Repositories
To collaborate with others or back up your code, you’ll need to use remote repositories. GitHub is the most popular platform for this. After creating a GitHub account and a new repository, you can add it as a remote to your local repository:
git remote add origin https://github.com/username/repository.git
git push -u origin master
Integrating Git into Your Learning Process
Now that you have a basic understanding of Git, let’s explore how you can integrate it into your learning process as a beginner programmer.
1. Version Your Practice Projects
Start using Git for all your practice projects, no matter how small. This will help you build the habit of committing changes regularly and maintaining a clean project history.
2. Use Branches for Features
When working on a new feature or trying out a new concept, create a new branch. This allows you to experiment freely without affecting your main codebase. It’s a great way to learn how branching works in larger projects.
3. Write Meaningful Commit Messages
Get into the habit of writing clear, concise commit messages. A good commit message briefly describes what changes were made and why. This skill will be invaluable when working on larger projects or in a team setting.
4. Explore Open Source Projects
Once you’re comfortable with basic Git operations, start exploring open-source projects on GitHub. You don’t need to contribute right away; just observing how professional developers use Git can be incredibly educational.
5. Practice Collaborative Workflows
If possible, find a coding buddy or join a study group where you can practice collaborative Git workflows. This includes creating pull requests, reviewing code, and resolving merge conflicts.
6. Use Git for Non-Code Projects
Git isn’t just for code. You can use it to version control your study notes, documentation, or even your resume. This gives you more opportunities to practice Git commands and concepts.
Common Git Challenges for Beginners
As you start using Git, you might encounter some challenges. Here are a few common ones and how to address them:
1. Merge Conflicts
Merge conflicts occur when Git can’t automatically merge changes from different branches. They can be intimidating at first, but they’re a normal part of collaborative development. When you encounter a merge conflict, Git will mark the conflicting areas in your files. You’ll need to manually resolve these conflicts by editing the files, then stage and commit the resolved files.
2. Detached HEAD State
You might sometimes find yourself in a “detached HEAD” state, which means you’re not on any branch. This usually happens when you checkout a specific commit. To fix this, you can create a new branch from your current state:
git checkout -b new-branch-name
3. Accidental Commits
If you accidentally commit changes you didn’t mean to, you can undo the last commit while keeping the changes:
git reset --soft HEAD~1
4. Pushing to Wrong Remote
If you accidentally push to the wrong remote repository, you can usually undo this by force pushing to the correct remote. However, be very careful with force pushing as it can overwrite remote changes:
git push origin +master
Advanced Git Concepts
As you become more comfortable with Git, you might want to explore some more advanced concepts:
1. Rebasing
Rebasing is an alternative to merging that can create a cleaner project history. It moves or combines a sequence of commits to a new base commit. However, it should be used with caution, especially on shared branches.
2. Interactive Rebasing
Interactive rebasing allows you to modify commits in various ways as they’re moved to the new base. This is useful for cleaning up your commit history before merging a feature branch.
3. Cherry-Picking
Cherry-picking allows you to pick individual commits from one branch and apply them to another. This can be useful when you want to pull in a specific change without merging entire branches.
4. Submodules
Submodules allow you to keep a Git repository as a subdirectory of another Git repository. This can be useful for incorporating third-party code into your project.
5. Git Hooks
Git hooks are scripts that Git executes before or after events such as commit, push, and receive. They can be used to enforce certain workflows or coding standards.
Git in Professional Development
As you progress in your coding journey and start working on professional projects, you’ll encounter more advanced Git workflows:
1. Gitflow Workflow
Gitflow is a branching model that involves using feature branches and multiple primary branches. It’s particularly well-suited for projects that have a scheduled release cycle.
2. Trunk-Based Development
This is a branching strategy where developers collaborate on code in a single branch called ‘trunk’, resisting any pressure to create other long-lived development branches.
3. Forking Workflow
In this workflow, common in open-source projects, developers ‘fork’ a central repository, make changes in their fork, and then submit a pull request to have their changes incorporated into the main project.
Git and Continuous Integration/Continuous Deployment (CI/CD)
In professional settings, Git is often integrated with CI/CD pipelines. These pipelines automatically build, test, and deploy code changes. Understanding how Git interacts with these systems is crucial for modern software development.
Git Best Practices
As you become more proficient with Git, it’s important to adopt best practices:
1. Commit Often
Make small, frequent commits rather than large, infrequent ones. This makes it easier to track changes and roll back if necessary.
2. Use Meaningful Branch Names
Use descriptive, meaningful names for your branches. For example, “feature/user-authentication” is more informative than “new-feature”.
3. Keep Your Repository Clean
Regularly delete merged branches and use .gitignore to exclude files that shouldn’t be tracked (like build artifacts or IDE-specific files).
4. Don’t Commit Sensitive Information
Never commit sensitive information like passwords or API keys. Use environment variables or secure vaults for such data.
5. Write Good Commit Messages
A good commit message should complete the sentence “If applied, this commit will…”. Keep the subject line under 50 characters and provide more detail in the body if necessary.
Conclusion
Learning Git early in your coding journey is an investment that will pay dividends throughout your career. It’s not just a tool for version control; it’s a fundamental skill that will enhance your ability to manage projects, collaborate with others, and contribute to the wider programming community.
As you continue your learning journey with platforms like AlgoCademy, remember to integrate Git into your practice. Use it for your coding exercises, algorithm implementations, and project work. The more you use Git, the more comfortable you’ll become with its concepts and commands.
Remember, becoming proficient with Git is a gradual process. Don’t be discouraged if you find it challenging at first. Like any skill, it takes practice and patience to master. But the effort you put into learning Git will be well worth it as you progress in your programming career.
So, as you dive into learning data structures, algorithms, and preparing for technical interviews, make sure to give Git the attention it deserves. It’s not just a tool; it’s a critical skill that will set you apart as a well-rounded, professional developer.