Music Theory and Coding: How Learning One Can Improve the Other
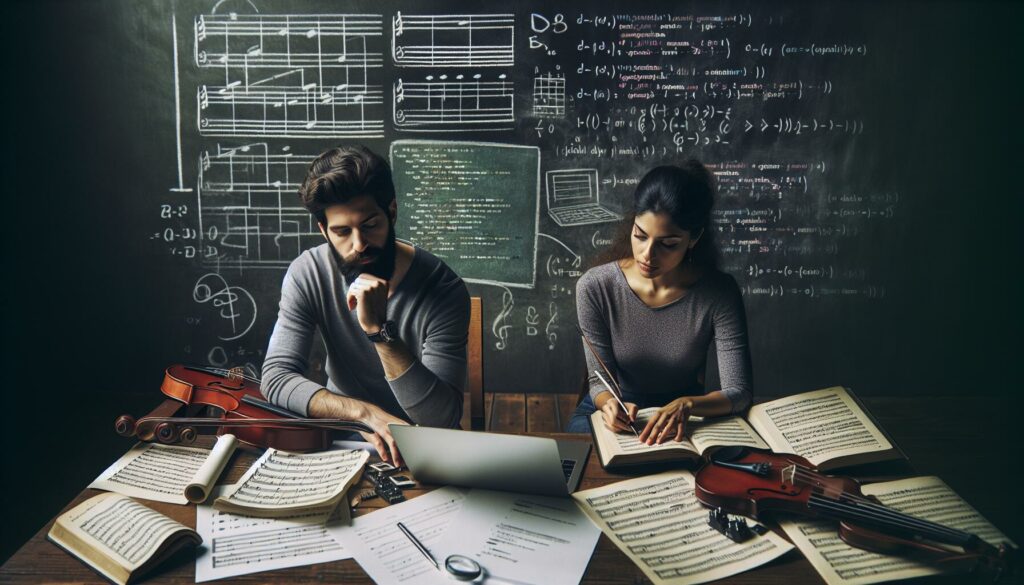
In the seemingly disparate worlds of music theory and coding, there lies a fascinating intersection of skills, concepts, and cognitive processes. As we delve into this intriguing relationship, we’ll explore how learning one discipline can significantly enhance proficiency in the other. This synergy is particularly relevant in the context of coding education platforms like AlgoCademy, which focuses on developing programming skills and preparing learners for technical interviews at major tech companies.
The Harmony of Music Theory and Coding
At first glance, music theory and coding might appear to be worlds apart. One deals with the intricacies of sound, harmony, and rhythm, while the other involves writing instructions for computers. However, upon closer examination, we find that these two fields share numerous fundamental principles and cognitive approaches.
1. Pattern Recognition
Both music theory and coding rely heavily on pattern recognition. In music, recognizing patterns in chord progressions, melodic structures, and rhythmic sequences is crucial for understanding and creating compositions. Similarly, in coding, identifying patterns in algorithms, data structures, and problem-solving approaches is essential for efficient programming.
For instance, consider the concept of a “loop” in programming:
for (int i = 0; i < 10; i++) {
System.out.println("Iteration: " + i);
}
This loop structure is analogous to repetitive patterns in music, such as a recurring chorus or a repeated bass line. Both require the ability to recognize and manipulate patterns to create a desired outcome.
2. Logical Thinking
Music theory and coding both demand strong logical thinking skills. In music, this manifests in understanding the relationships between notes, chords, and scales, and how they fit together to create harmonious compositions. In coding, logical thinking is crucial for designing algorithms, debugging code, and solving complex problems.
Consider the process of harmonizing a melody in music theory. This requires a logical approach to selecting chords that complement the melody notes while adhering to the rules of harmony. Similarly, in coding, creating a function to solve a specific problem requires a logical sequence of steps:
function calculateFactorial(n) {
if (n === 0 || n === 1) {
return 1;
} else {
return n * calculateFactorial(n - 1);
}
}
Both scenarios demonstrate the application of logical thinking to achieve a desired result.
3. Abstract Thinking
Abstract thinking is a cornerstone of both music theory and coding. In music, abstract concepts like tonality, modulation, and counterpoint require the ability to think beyond concrete notes and rhythms. In coding, abstraction is fundamental to creating reusable code, designing efficient algorithms, and understanding complex systems.
For example, the concept of a “function” in programming is an abstraction that encapsulates a set of instructions:
function greet(name) {
return "Hello, " + name + "!";
}
console.log(greet("Alice")); // Outputs: Hello, Alice!
This abstraction is similar to how a musical motif can be reused and transformed throughout a composition, representing an abstract idea rather than a specific set of notes.
How Music Theory Enhances Coding Skills
Learning music theory can significantly boost one’s coding abilities in several ways:
1. Improved Pattern Recognition
Studying music theory hones one’s ability to recognize and manipulate patterns. This skill directly translates to coding, where pattern recognition is crucial for:
- Identifying common algorithmic structures
- Recognizing design patterns in software architecture
- Spotting recurring bugs or inefficiencies in code
For instance, a programmer familiar with musical patterns might more easily recognize the structure of a recursive algorithm, seeing it as analogous to a musical theme that references itself:
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
2. Enhanced Problem-Solving Skills
Music theory often involves problem-solving, such as figuring out how to resolve a dissonant chord or how to modulate between keys smoothly. This problem-solving mindset is invaluable in coding, where developers constantly face challenges like:
- Optimizing algorithms for better performance
- Debugging complex issues in large codebases
- Designing scalable and maintainable software architectures
The ability to approach problems from multiple angles, a skill honed in music theory, can lead to more creative and efficient solutions in coding.
3. Improved Focus and Attention to Detail
Studying music theory requires meticulous attention to detail, from analyzing chord voicings to understanding the nuances of rhythmic notation. This level of focus and precision is equally crucial in coding, where a single misplaced character can lead to errors or unexpected behavior.
For example, consider the following JavaScript code:
let x = 5;
let y = "5";
if (x == y) {
console.log("Equal");
} else {
console.log("Not equal");
}
A keen eye for detail, developed through music theory study, might help a programmer quickly spot the potential issue with type coercion in this comparison.
4. Creativity and Innovation
Music theory not only teaches rules but also how to creatively bend or break them for artistic effect. This mindset of creative exploration within a structured system is highly valuable in coding, encouraging developers to think outside the box and come up with innovative solutions to complex problems.
How Coding Enhances Music Theory Skills
The relationship between music theory and coding is reciprocal. Just as music theory can improve coding skills, learning to code can significantly enhance one’s understanding and application of music theory:
1. Algorithmic Thinking in Composition
Coding teaches algorithmic thinking, which can be applied to music composition and analysis. For example, understanding loops and conditionals in programming can help in creating complex rhythmic patterns or generative music:
function generateRhythm(beats, probability) {
let rhythm = [];
for (let i = 0; i < beats; i++) {
if (Math.random() < probability) {
rhythm.push(1);
} else {
rhythm.push(0);
}
}
return rhythm;
}
let myRhythm = generateRhythm(16, 0.5);
console.log(myRhythm); // Outputs a random rhythm pattern
This algorithmic approach to rhythm generation can inspire new ideas in musical composition and improvisation.
2. Data Structures for Musical Analysis
Understanding data structures from coding can provide new ways to analyze and represent musical structures. For instance, a tree data structure can be used to represent the hierarchical nature of musical form:
class MusicalSection {
constructor(name) {
this.name = name;
this.subsections = [];
}
addSubsection(subsection) {
this.subsections.push(subsection);
}
}
let sonata = new MusicalSection("Sonata");
let exposition = new MusicalSection("Exposition");
let development = new MusicalSection("Development");
let recapitulation = new MusicalSection("Recapitulation");
sonata.addSubsection(exposition);
sonata.addSubsection(development);
sonata.addSubsection(recapitulation);
This structured approach to musical analysis can lead to deeper insights into compositional techniques and form.
3. Logical Thinking in Harmonic Analysis
The logical thinking developed through coding can enhance one’s ability to analyze complex harmonic progressions. For example, a programmer might approach chord progression analysis as a series of logical steps, similar to a flowchart or decision tree in programming.
4. Automation of Music Theory Tasks
Coding skills allow musicians and music theorists to automate repetitive tasks, such as generating chord progressions, transposing melodies, or analyzing large datasets of musical pieces. This can lead to more efficient learning and research in music theory.
function transposeNote(note, semitones) {
const notes = ['C', 'C#', 'D', 'D#', 'E', 'F', 'F#', 'G', 'G#', 'A', 'A#', 'B'];
let index = notes.indexOf(note);
return notes[(index + semitones + 12) % 12];
}
console.log(transposeNote('C', 7)); // Outputs: G
Practical Applications in Learning Platforms
The synergy between music theory and coding can be leveraged in learning platforms like AlgoCademy to create more engaging and effective learning experiences:
1. Interdisciplinary Exercises
Coding platforms could incorporate music theory concepts into programming exercises. For example, creating algorithms to generate chord progressions or analyze the structure of a musical piece. This interdisciplinary approach can make learning more engaging and help students see the broader applications of their coding skills.
2. Visualization Tools
Coding skills can be used to create interactive visualizations of music theory concepts. For instance, a web-based tool that visually represents the circle of fifths and allows users to interact with it through code:
class CircleOfFifths {
constructor() {
this.notes = ['C', 'G', 'D', 'A', 'E', 'B', 'F#', 'C#', 'G#', 'D#', 'A#', 'F'];
}
getRelativeMajor(index) {
return this.notes[index];
}
getRelativeMinor(index) {
return this.notes[(index + 9) % 12].toLowerCase();
}
}
let circle = new CircleOfFifths();
console.log(circle.getRelativeMajor(0)); // Outputs: C
console.log(circle.getRelativeMinor(0)); // Outputs: a
3. AI-Powered Learning Assistance
Platforms like AlgoCademy could integrate AI algorithms that recognize patterns in a student’s coding style and suggest improvements based on musical analogies. For example, if a student struggles with nested loops, the AI could draw parallels to complex rhythmic patterns in music to provide a different perspective on the concept.
4. Gamification Using Musical Elements
Coding challenges could be gamified using musical elements. For instance, successfully completing a coding task could unlock a musical achievement, such as adding a new instrument to a virtual ensemble or progressing through different musical eras as coding skills improve.
Challenges and Considerations
While the connection between music theory and coding offers numerous benefits, there are also challenges to consider:
1. Learning Curve
Both music theory and coding have steep learning curves. Integrating both disciplines may be overwhelming for some learners, especially beginners. It’s important to introduce interdisciplinary concepts gradually and provide ample support.
2. Individual Learning Styles
Not all learners may resonate with the connection between music and coding. Learning platforms should offer alternative approaches to cater to different learning styles and interests.
3. Balancing Depth and Breadth
While exploring the connections between music theory and coding can be enriching, it’s crucial to ensure that core programming concepts are not overshadowed. The integration should enhance, not detract from, the primary goal of developing strong coding skills.
4. Keeping Content Relevant
As both music technology and programming languages evolve, learning platforms must continuously update their content to remain relevant and align with current industry practices.
Conclusion
The intersection of music theory and coding presents a unique opportunity to enhance learning in both fields. By recognizing and leveraging the cognitive skills shared between these disciplines, learners can develop a more holistic and creative approach to problem-solving, pattern recognition, and abstract thinking.
For platforms like AlgoCademy, integrating elements of music theory into coding education can create a more engaging, interdisciplinary learning experience. This approach not only broadens students’ perspectives but also prepares them for the increasingly diverse and creative challenges in the tech industry.
As we continue to explore the synergies between different fields of study, the combination of music theory and coding stands out as a particularly harmonious and promising avenue for educational innovation. By embracing this interdisciplinary approach, we can cultivate a new generation of programmers who are not only technically proficient but also creatively inspired and cognitively versatile.