What Chess Can Teach You About Learning Algorithms and Data Structures
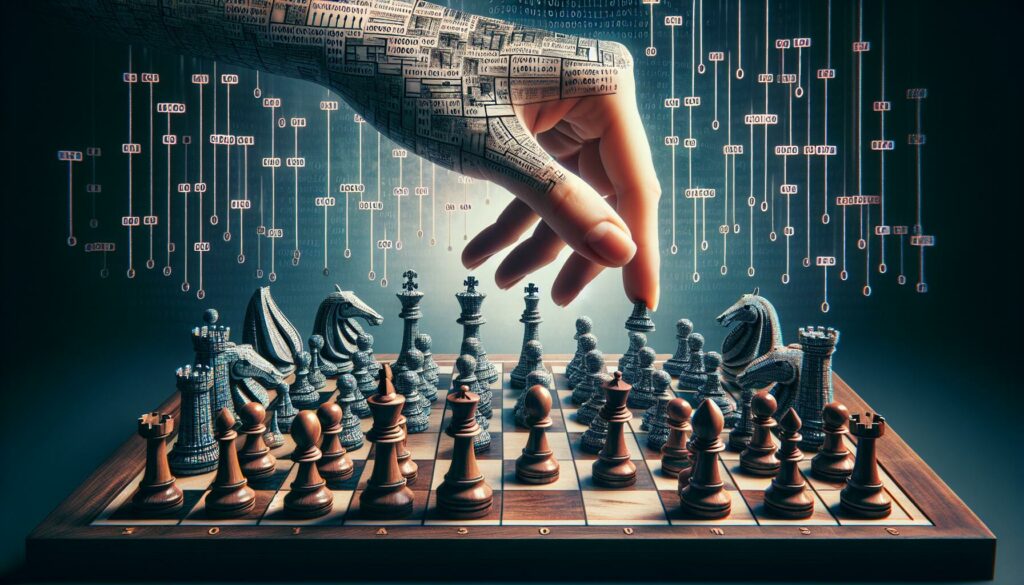
In the world of programming, mastering algorithms and data structures is akin to becoming a grandmaster in chess. Both disciplines require strategic thinking, pattern recognition, and the ability to plan several moves ahead. As we delve into this comparison, we’ll explore how the ancient game of chess can provide valuable insights into learning some of the most crucial concepts in computer science.
The Opening: Setting the Foundation
In chess, the opening moves set the tone for the entire game. Similarly, when learning algorithms and data structures, it’s crucial to start with a solid foundation. Just as chess players memorize common openings, programmers should familiarize themselves with basic data structures like arrays, linked lists, and hash tables.
Consider the following code snippet that demonstrates a simple array in Python:
# Basic array in Python
chess_pieces = ["Pawn", "Knight", "Bishop", "Rook", "Queen", "King"]
# Accessing elements
print(chess_pieces[0]) # Output: Pawn
print(chess_pieces[-1]) # Output: King
# Adding an element
chess_pieces.append("Extra Queen")
# Removing an element
chess_pieces.pop()
This basic structure allows for quick access and manipulation, much like how knowing standard chess openings provides a strategic advantage from the start of the game.
The Middlegame: Developing Strategies
As a chess game progresses, players must develop and execute complex strategies. This phase mirrors the process of learning more advanced algorithms. In chess, you might sacrifice a pawn to gain positional advantage; in programming, you might trade off space complexity for time efficiency.
Let’s look at the classic example of a binary search algorithm, which sacrifices the simplicity of linear search for logarithmic time complexity:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
result = binary_search(sorted_array, 7)
print(f"Element found at index: {result}") # Output: Element found at index: 3
This algorithm, like a well-executed chess strategy, efficiently narrows down the search space with each iteration, demonstrating the power of divide-and-conquer approaches in both chess and programming.
Tactical Combinations: Algorithmic Patterns
Chess players often study tactical combinations – sequences of moves that lead to a concrete advantage. In the realm of algorithms, design patterns serve a similar purpose. These are proven solutions to common programming problems that can be applied across various scenarios.
One such pattern is the sliding window technique, useful for solving substring problems efficiently. Here’s an example of finding the longest substring without repeating characters:
def longest_substring_without_repeats(s):
char_set = set()
max_length = 0
left = right = 0
while right < len(s):
if s[right] not in char_set:
char_set.add(s[right])
max_length = max(max_length, right - left + 1)
right += 1
else:
char_set.remove(s[left])
left += 1
return max_length
# Example usage
input_string = "abcabcbb"
result = longest_substring_without_repeats(input_string)
print(f"Length of longest substring: {result}") # Output: Length of longest substring: 3
This algorithm, like a well-executed tactical combination in chess, efficiently solves a complex problem by maintaining a sliding window and adjusting it based on the current state.
Endgame: Optimization and Refinement
In the endgame of chess, every move is crucial, and efficiency is paramount. This phase is comparable to optimizing algorithms and fine-tuning data structures for peak performance. Just as chess players study endgame positions to make the most of their remaining pieces, programmers analyze time and space complexity to squeeze out every bit of efficiency.
Consider the process of optimizing a recursive algorithm using dynamic programming. The classic example is the Fibonacci sequence:
# Naive recursive approach
def fibonacci_recursive(n):
if n <= 1:
return n
return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
# Optimized dynamic programming approach
def fibonacci_dp(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Example usage
n = 10
print(f"Recursive: {fibonacci_recursive(n)}") # Slower for large n
print(f"Dynamic Programming: {fibonacci_dp(n)}") # Much faster for large n
The dynamic programming approach, like a well-studied endgame position, allows for much faster computation by storing and reusing intermediate results.
Pattern Recognition: From Chess to Code
One of the most valuable skills in both chess and programming is pattern recognition. Chess players learn to recognize common pawn structures, piece configurations, and tactical motifs. Similarly, programmers develop an eye for code patterns, algorithmic structures, and data manipulation techniques.
For instance, recognizing the pattern of a binary tree can lead to efficient solutions for many problems. Here’s an example of a binary tree implementation and a depth-first search traversal:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
result = []
def dfs(node):
if not node:
return
dfs(node.left)
result.append(node.val)
dfs(node.right)
dfs(root)
return result
# Example usage
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
print(inorder_traversal(root)) # Output: [4, 2, 5, 1, 3]
Recognizing the recursive nature of tree structures allows for elegant and efficient solutions, much like how recognizing familiar patterns in chess positions can guide strategic decision-making.
Time Management: Balancing Depth and Breadth
In chess tournaments, players must manage their time wisely, deciding when to spend more time on a critical position and when to make quicker moves. This skill translates directly to learning algorithms and data structures. Knowing when to dive deep into a particular topic and when to gain a broader understanding is crucial for effective learning.
For example, while it’s important to understand the intricacies of advanced algorithms like Red-Black trees, it’s equally valuable to have a working knowledge of a wide range of sorting algorithms. Here’s a quick implementation of the quicksort algorithm, which demonstrates a good balance of efficiency and conceptual depth:
def quicksort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
# Example usage
unsorted_array = [3, 6, 8, 10, 1, 2, 1]
sorted_array = quicksort(unsorted_array)
print(f"Sorted array: {sorted_array}") # Output: Sorted array: [1, 1, 2, 3, 6, 8, 10]
Understanding quicksort provides insights into divide-and-conquer strategies and partitioning, concepts that are broadly applicable in algorithm design.
Analyzing Positions: Complexity Analysis
Chess players spend considerable time analyzing positions, calculating variations, and evaluating the strength of different moves. This analytical approach is mirrored in the way programmers perform complexity analysis on algorithms. Understanding the time and space complexity of different approaches is crucial for writing efficient code.
Let’s consider the problem of finding the nth Fibonacci number and analyze different approaches:
import time
def fib_recursive(n):
if n <= 1:
return n
return fib_recursive(n-1) + fib_recursive(n-2)
def fib_dynamic(n):
if n <= 1:
return n
dp = [0] * (n+1)
dp[1] = 1
for i in range(2, n+1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
def fib_iterative(n):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n+1):
a, b = b, a + b
return b
# Time comparison
n = 30
start = time.time()
print(f"Recursive: {fib_recursive(n)}")
print(f"Time taken: {time.time() - start} seconds")
start = time.time()
print(f"Dynamic: {fib_dynamic(n)}")
print(f"Time taken: {time.time() - start} seconds")
start = time.time()
print(f"Iterative: {fib_iterative(n)}")
print(f"Time taken: {time.time() - start} seconds")
This comparison demonstrates how different approaches to the same problem can have vastly different time complexities. The recursive solution has an exponential time complexity, while the dynamic programming and iterative solutions have linear time complexity. This analysis, like evaluating chess positions, helps in choosing the most appropriate algorithm for a given situation.
Learning from Masters: Studying Classic Algorithms
Chess players often study games played by grandmasters to learn advanced strategies and techniques. Similarly, programmers can gain invaluable insights by studying classic algorithms developed by computer science pioneers. These algorithms often demonstrate elegant solutions to complex problems and showcase important problem-solving techniques.
Let’s look at the implementation of Dijkstra’s algorithm, a classic solution for finding the shortest path in a weighted graph:
import heapq
def dijkstra(graph, start):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return distances
# Example usage
graph = {
'A': {'B': 4, 'C': 2},
'B': {'D': 3, 'E': 1},
'C': {'B': 1, 'D': 5},
'D': {'E': 2},
'E': {}
}
start_node = 'A'
shortest_distances = dijkstra(graph, start_node)
print(f"Shortest distances from {start_node}: {shortest_distances}")
Studying this algorithm provides insights into graph traversal, priority queues, and greedy algorithms – concepts that are fundamental to many advanced programming problems.
Continuous Improvement: Practice and Review
Both chess players and programmers understand the importance of continuous practice and review. In chess, players often analyze their games to identify mistakes and areas for improvement. Similarly, reviewing and refactoring code is an essential practice for improving programming skills.
Here’s an example of how one might refactor a simple function to make it more efficient and readable:
# Original function
def find_max(numbers):
max_num = numbers[0]
for num in numbers:
if num > max_num:
max_num = num
return max_num
# Refactored function
def find_max_refactored(numbers):
return max(numbers) if numbers else None
# Example usage
number_list = [3, 7, 2, 9, 1, 5]
print(f"Original: {find_max(number_list)}")
print(f"Refactored: {find_max_refactored(number_list)}")
This refactoring demonstrates how built-in functions can often provide more efficient and concise solutions. Regularly reviewing and improving code, like analyzing chess games, leads to better overall skill and understanding.
Adaptability: Handling Different Problem Types
Chess players must be able to adapt their strategy based on their opponent’s style and the specific position on the board. Similarly, programmers need to be adaptable, choosing the right data structure or algorithm based on the specific requirements of each problem.
Consider the following example where we use different data structures to solve the same problem of finding duplicate elements:
def find_duplicates_list(nums):
seen = []
duplicates = []
for num in nums:
if num in seen:
duplicates.append(num)
else:
seen.append(num)
return duplicates
def find_duplicates_set(nums):
seen = set()
return {num for num in nums if num in seen or seen.add(num)}
# Example usage
numbers = [1, 2, 3, 4, 2, 5, 6, 4, 7, 8, 8]
print(f"Using list: {find_duplicates_list(numbers)}")
print(f"Using set: {find_duplicates_set(numbers)}")
This example shows how using a set can lead to a more efficient solution for this particular problem, demonstrating the importance of choosing the right tool for the job.
Visualization: Mental Models in Chess and Coding
Chess players develop strong visualization skills, able to picture potential board positions several moves ahead. Programmers benefit from similar mental modeling, visualizing data structures and the flow of algorithms. This skill is particularly useful when working with complex data structures like trees and graphs.
Here’s an example of how we might visualize a binary search tree insertion process:
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def insert(root, val):
if not root:
return TreeNode(val)
if val < root.val:
root.left = insert(root.left, val)
else:
root.right = insert(root.right, val)
return root
def inorder_traversal(root):
if not root:
return []
return inorder_traversal(root.left) + [root.val] + inorder_traversal(root.right)
# Building a BST
root = None
values = [5, 3, 7, 1, 4, 6, 8]
for value in values:
root = insert(root, value)
print(f"Inorder traversal: {inorder_traversal(root)}")
Visualizing this process helps in understanding how the tree structure evolves with each insertion, similar to how chess players visualize the board changing with each move.
Problem Decomposition: Breaking Down Complex Challenges
In chess, complex positions are often broken down into smaller, more manageable sub-problems. This approach is directly applicable to algorithmic problem-solving, where breaking a large problem into smaller, solvable components is a key strategy.
Let’s look at how we might break down the problem of finding the longest palindromic substring:
def longest_palindrome_substring(s):
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return s[left+1:right]
longest = ""
for i in range(len(s)):
# Odd length palindromes
palindrome1 = expand_around_center(i, i)
if len(palindrome1) > len(longest):
longest = palindrome1
# Even length palindromes
palindrome2 = expand_around_center(i, i+1)
if len(palindrome2) > len(longest):
longest = palindrome2
return longest
# Example usage
s = "babad"
print(f"Longest palindromic substring: {longest_palindrome_substring(s)}")
This solution breaks down the problem into checking for palindromes centered at each character (odd-length) and between each pair of characters (even-length), demonstrating how complex problems can be solved by addressing simpler sub-problems.
Conclusion: The Synergy Between Chess and Coding
The parallels between chess and learning algorithms and data structures are striking. Both disciplines require strategic thinking, pattern recognition, and the ability to plan ahead. By approaching the study of algorithms and data structures with the same rigor and analytical mindset as a chess player, programmers can significantly enhance their problem-solving skills and coding abilities.
Remember, just as becoming a chess master takes years of dedicated practice and study, mastering algorithms and data structures is a journey that requires patience, persistence, and continuous learning. By embracing the strategies and mindsets common to both chess and coding, you can accelerate your growth as a programmer and develop a deeper understanding of computational thinking.
Whether you’re navigating the complexities of a chess endgame or optimizing a challenging algorithm, the skills you develop will serve you well in both arenas. So, the next time you sit down to code, think like a chess player: analyze the problem, consider your options, plan your strategy, and make your move. With practice and perseverance, you’ll find yourself becoming a grandmaster of algorithms and data structures, ready to tackle any coding challenge that comes your way.