Coding Through Mindfulness: How Staying Present Can Boost Your Learning
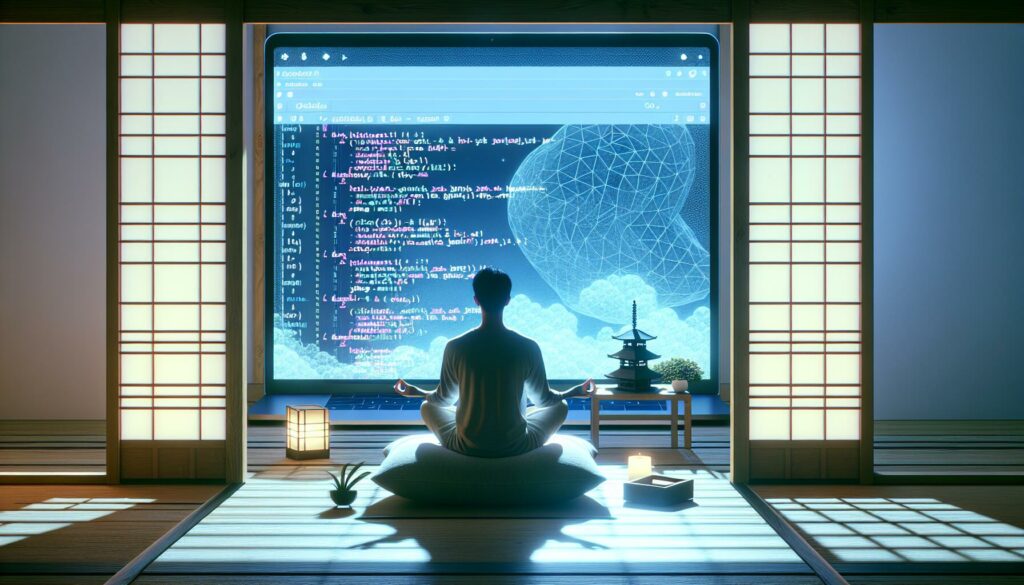
In the fast-paced world of coding and technology, it’s easy to get caught up in the rush to learn new languages, master complex algorithms, and stay ahead of the curve. However, an often-overlooked aspect of effective learning and problem-solving is the practice of mindfulness. This article explores how incorporating mindfulness techniques into your coding journey can significantly enhance your learning experience, boost productivity, and improve your overall skills as a programmer.
Understanding Mindfulness in the Context of Coding
Mindfulness, at its core, is the practice of being fully present and engaged in the current moment. When applied to coding, it means giving your complete attention to the task at hand, whether you’re debugging a tricky piece of code, learning a new programming concept, or working through a complex algorithm.
For many developers, especially those just starting their journey or preparing for technical interviews with top tech companies, the coding process can be overwhelming. The pressure to perform, coupled with the vast amount of information to absorb, can lead to stress, anxiety, and decreased productivity. This is where mindfulness comes into play, offering a way to navigate these challenges with greater ease and efficiency.
The Benefits of Mindful Coding
1. Enhanced Focus and Concentration
One of the primary benefits of practicing mindfulness while coding is improved focus. By training your mind to stay present, you can better concentrate on the code in front of you, reducing distractions and increasing your ability to spot errors or identify optimal solutions.
For instance, when working on a complex algorithm problem on platforms like AlgoCademy, mindful coding can help you maintain focus during long problem-solving sessions. This sustained attention is crucial when tackling the types of challenging questions often asked in technical interviews at major tech companies.
2. Reduced Stress and Anxiety
Learning to code, especially when preparing for high-stakes interviews, can be stressful. Mindfulness techniques, such as deep breathing or brief meditation sessions, can help manage this stress. By staying present and avoiding worry about future outcomes, you can approach your coding practice with a calmer, more focused mind.
3. Improved Problem-Solving Skills
Mindfulness encourages a state of open awareness, which can lead to more creative problem-solving. When you’re fully present with a coding challenge, you’re more likely to notice subtle details or make unexpected connections that could lead to innovative solutions.
4. Better Code Quality
By practicing mindful coding, you’re more likely to write cleaner, more efficient code. Being present allows you to pay closer attention to best practices, code structure, and potential optimizations, resulting in higher quality output.
5. Enhanced Learning Retention
When you’re fully engaged in the learning process, you’re more likely to retain information. This is particularly beneficial when working through tutorials or practicing new concepts on platforms like AlgoCademy, where each lesson builds on previous knowledge.
Practical Mindfulness Techniques for Coders
Now that we understand the benefits, let’s explore some practical ways to incorporate mindfulness into your coding practice:
1. Mindful Breathing
Before starting a coding session or when feeling overwhelmed, take a few minutes to focus on your breath. This simple practice can help center your mind and prepare you for focused work.
// A simple mindful breathing exercise
function mindfulBreathing() {
for (let i = 0; i < 5; i++) {
console.log("Breathe in deeply for 4 seconds");
console.log("Hold for 4 seconds");
console.log("Exhale slowly for 6 seconds");
}
console.log("Ready to code with a clear mind!");
}
mindfulBreathing();
2. The Pomodoro Technique with Mindfulness
Combine the Pomodoro Technique (working in focused 25-minute intervals) with mindfulness. During each Pomodoro, commit to being fully present with your code. Use the short breaks to practice brief mindfulness exercises.
3. Mindful Code Review
When reviewing your code or studying solutions on AlgoCademy, do so mindfully. Read each line slowly and intentionally, fully understanding its purpose and function within the larger context of the program.
4. Gratitude Practice
At the end of each coding session, take a moment to reflect on what you’ve learned or accomplished. This practice of gratitude can help maintain a positive mindset and motivation in your coding journey.
5. Mindful Debugging
When faced with a bug, approach the debugging process mindfully. Instead of becoming frustrated, view it as an opportunity to learn. Pay close attention to each step of your debugging process, staying curious and open to what the code is telling you.
// Example of mindful debugging
function mindfulDebugging(code) {
console.log("Taking a deep breath and approaching the bug with curiosity.");
let steps = [
"Identify the problem",
"Reproduce the error",
"Isolate the source",
"Fix the bug",
"Test the solution"
];
steps.forEach((step, index) => {
console.log(`Step ${index + 1}: ${step}`);
// Implement each step mindfully
// ...
});
console.log("Bug fixed. Reflecting on what I've learned from this process.");
}
Integrating Mindfulness into Your Coding Education
When using platforms like AlgoCademy to learn and practice coding, there are several ways to incorporate mindfulness into your study routine:
1. Set Intentional Learning Goals
Before starting a new lesson or coding challenge, take a moment to set clear, intentional goals for what you want to achieve. This mindful approach helps focus your learning and makes the process more purposeful.
2. Practice Active Listening
When watching video tutorials or reading explanations, practice active, mindful listening. Fully engage with the content, avoiding the temptation to multitask or let your mind wander.
3. Reflect on Your Progress
Regularly take time to mindfully reflect on your coding progress. Celebrate your achievements, no matter how small, and consider areas where you can improve without judgment.
4. Mindful Problem-Solving
When tackling coding challenges, approach each problem with a mindful attitude. Read the problem statement carefully, consider different approaches without rushing, and implement your solution with full attention to detail.
// Example of mindful problem-solving approach
function solveProblemMindfully(problem) {
console.log("Taking a moment to center myself before starting.");
let steps = [
"Read and understand the problem statement",
"Identify the inputs and expected outputs",
"Consider multiple approaches",
"Choose the most suitable approach",
"Implement the solution step by step",
"Test with various inputs",
"Optimize if necessary"
];
steps.forEach((step, index) => {
console.log(`Step ${index + 1}: ${step}`);
// Implement each step with full attention
// ...
});
console.log("Solution complete. Reflecting on the process and what I've learned.");
}
Overcoming Challenges in Mindful Coding
While the benefits of mindful coding are clear, implementing these practices can come with its own set of challenges. Here are some common obstacles you might face and strategies to overcome them:
1. Dealing with Distractions
In our hyper-connected world, distractions are constant. Notifications, emails, and the temptation to multitask can pull your attention away from your code.
Strategy: Create a dedicated coding environment. Turn off notifications, use website blockers if necessary, and communicate your focus time to others. Treat your coding sessions as important appointments with yourself.
2. Handling Frustration
Coding can be frustrating, especially when dealing with persistent bugs or struggling to grasp a new concept. This frustration can make it difficult to maintain a mindful approach.
Strategy: Acknowledge your feelings without judgment. Take short breaks to reset your mind, perhaps using a quick mindfulness exercise. Remember that challenges are part of the learning process and approach them with curiosity rather than frustration.
3. Maintaining Consistency
Like any new habit, consistently practicing mindful coding can be challenging, especially when under pressure or tight deadlines.
Strategy: Start small. Begin with just a few minutes of mindful coding practice each day and gradually increase. Use reminders or integrate mindfulness into your existing coding routine, such as taking a mindful breath before each new function you write.
4. Balancing Mindfulness with Productivity
There might be concerns that taking time for mindfulness practices could slow down your coding or learning progress.
Strategy: Remember that mindfulness is about quality, not just quantity. The improved focus and reduced errors that come with mindful coding often lead to better overall productivity. Track your progress and efficiency to see the benefits for yourself.
Mindfulness and Technical Interviews
For many coders, especially those using platforms like AlgoCademy, a major goal is to prepare for technical interviews at top tech companies. Mindfulness can be a powerful tool in this preparation process and during the interviews themselves.
Mindful Interview Preparation
- Focused Practice: Apply mindfulness techniques when working through practice problems. This helps in deeply understanding each problem and solution, rather than rushing through to solve as many as possible.
- Stress Management: Use mindfulness to manage the stress and anxiety that often come with interview preparation. Regular mindfulness practice can help you stay calm and focused during your study sessions.
- Efficient Learning: Mindful learning can help you retain information more effectively, making your preparation more efficient.
During the Interview
- Staying Present: Mindfulness techniques can help you stay present and focused during the interview, rather than getting caught up in anxiety about the outcome.
- Clear Communication: Being mindful can improve your ability to listen carefully to the interviewer and communicate your thoughts clearly.
- Problem-Solving Under Pressure: Mindful approaches to problem-solving can help you think more clearly and creatively when faced with challenging questions.
// Mindful approach to a technical interview question
function solveTechnicalInterviewQuestion(question) {
console.log("Taking a deep breath and centering myself.");
let steps = [
"Listen carefully to the question",
"Clarify any uncertainties",
"Think through the problem out loud",
"Consider edge cases",
"Implement the solution step by step",
"Test the solution",
"Discuss potential optimizations"
];
steps.forEach((step, index) => {
console.log(`Step ${index + 1}: ${step}`);
// Implement each step mindfully
// ...
});
console.log("Solution presented. Reflecting on the process regardless of the outcome.");
}
The Long-Term Benefits of Mindful Coding
Incorporating mindfulness into your coding practice isn’t just about short-term gains in focus and productivity. It can have significant long-term benefits for your career and personal development as a programmer:
1. Sustainable Learning
Mindful coding practices promote a more sustainable approach to learning. Instead of burning out from intense cramming sessions, you develop a steady, consistent practice that can be maintained over the long term. This is particularly valuable in the ever-evolving field of technology, where continuous learning is essential.
2. Improved Problem-Solving Skills
Regular mindfulness practice can enhance your overall problem-solving abilities. By training your mind to stay present and approach challenges with curiosity, you develop a more flexible and creative approach to coding problems. This skill is invaluable not just in coding, but in all aspects of a tech career.
3. Better Work-Life Balance
The mindfulness skills you develop through mindful coding can extend to other areas of your life, helping you maintain a better work-life balance. This can lead to reduced stress, improved overall well-being, and a more sustainable career in the long run.
4. Enhanced Collaboration Skills
Mindfulness can improve your ability to listen actively and communicate clearly, which are crucial skills in collaborative coding environments. This can lead to better teamwork, more effective code reviews, and improved relationships with colleagues.
5. Adaptability in a Changing Industry
The tech industry is known for its rapid changes. The adaptability and open-mindedness fostered by mindfulness practices can help you navigate these changes more effectively, staying current with new technologies and methodologies.
Conclusion: Embracing Mindfulness in Your Coding Journey
As we’ve explored throughout this article, integrating mindfulness into your coding practice can have profound effects on your learning, productivity, and overall experience as a programmer. Whether you’re just starting out, preparing for technical interviews, or looking to enhance your existing skills, mindfulness offers a powerful tool to support your growth.
Remember, the journey of mindful coding is a personal one. What works best for you may be different from others. Experiment with different techniques, be patient with yourself, and observe how these practices impact your coding over time. As you continue to use platforms like AlgoCademy to develop your programming skills, let mindfulness be a constant companion in your learning journey.
By cultivating a mindful approach to coding, you’re not just becoming a better programmer – you’re developing skills that will serve you well throughout your career and life. So take a deep breath, center yourself, and approach your next coding challenge with renewed focus and presence. Happy coding!