From Cooking to Coding: Lessons in Precision, Timing, and Patience
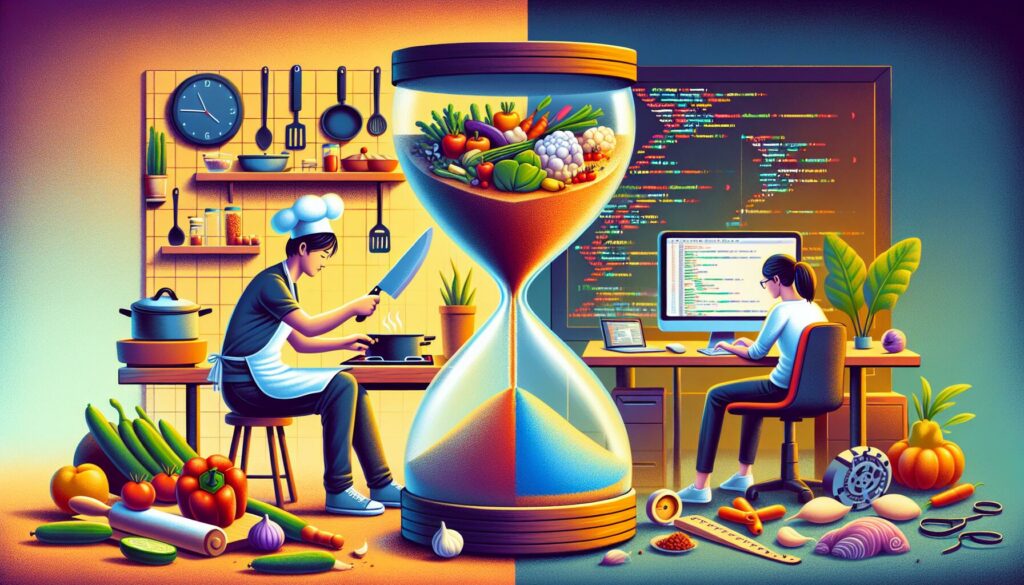
At first glance, the worlds of cooking and coding might seem worlds apart. One involves pots, pans, and culinary creativity, while the other deals with computers, algorithms, and digital innovation. However, upon closer inspection, these two disciplines share surprising similarities that can offer valuable insights for aspiring programmers. In this article, we’ll explore how the skills and mindset cultivated in the kitchen can translate into success in the world of coding, and how platforms like AlgoCademy can help bridge this gap.
The Art of Precision
In both cooking and coding, precision is key. Just as a chef must measure ingredients carefully to achieve the perfect flavor balance, a programmer must pay close attention to syntax and structure to create functional code. Let’s break down this parallel:
Cooking Precision
In the culinary world, precision can make or break a dish. Consider baking, where even small variations in ingredient measurements can lead to drastically different results. A few extra grams of baking powder might turn a fluffy cake into a dense, unpalatable mess. Similarly, the timing of when ingredients are added can significantly impact the final product.
Coding Precision
In programming, precision is equally crucial. A misplaced semicolon, an incorrect variable name, or a mismatched parenthesis can cause an entire program to fail. Just as in cooking, the order in which operations are performed (known as the order of operations in programming) can dramatically affect the outcome.
For example, consider this simple JavaScript code snippet:
let x = 5;
let y = 3;
let result = x + y * 2;
The result here will be 11, not 16, because multiplication takes precedence over addition in the order of operations. A programmer needs to be aware of these rules, just as a chef needs to understand the impact of adding ingredients in a specific order.
The Importance of Timing
Timing is another critical factor that links cooking and coding. In both fields, understanding when to perform certain actions can be the difference between success and failure.
Timing in the Kitchen
Any experienced cook knows that timing is everything. Vegetables need to be added to a stir-fry at different times based on their cooking rates. Pasta must be cooked for just the right amount of time to achieve the perfect al dente texture. Even the timing of seasoning can impact the final flavor profile of a dish.
Timing in Code
In programming, timing is equally important, especially when dealing with asynchronous operations, multi-threaded programs, or real-time systems. Consider this example of asynchronous JavaScript using Promises:
function fetchData() {
return new Promise((resolve) => {
setTimeout(() => {
resolve("Data fetched!");
}, 2000);
});
}
async function processData() {
console.log("Starting data fetch...");
const data = await fetchData();
console.log(data);
console.log("Data processing complete.");
}
processData();
In this code, understanding the timing of asynchronous operations is crucial. The await
keyword ensures that the program waits for the data to be fetched before moving on to the next line, much like a chef waiting for water to boil before adding pasta.
The Virtue of Patience
Both cooking and coding require a significant amount of patience. Rome wasn’t built in a day, and neither are complex recipes or sophisticated programs.
Patience in Cooking
Many dishes require slow cooking, marination, or resting periods to develop their full flavors. A rushed chef might be tempted to increase heat to speed up cooking, often resulting in burnt exteriors and raw interiors. Patience allows flavors to meld, meats to tenderize, and bread to rise properly.
Patience in Coding
Similarly, in programming, patience is a virtue. Complex problems often require time to solve, and rushing can lead to suboptimal solutions or overlooked bugs. Debugging, in particular, demands patience as programmers methodically trace through code to identify and fix issues.
Consider this recursive function to calculate the nth Fibonacci number:
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
console.log(fibonacci(40));
While this function works, it’s incredibly inefficient for large values of n. A patient programmer might take the time to optimize this using dynamic programming or memoization, significantly improving its performance.
The Joy of Experimentation
Both cooking and coding encourage experimentation and creativity. This willingness to try new things often leads to innovation and discovery.
Culinary Experiments
In the kitchen, chefs often experiment with new flavor combinations, cooking techniques, or ingredient substitutions. These experiments can lead to exciting new dishes or improvements on classic recipes. Even failures can be valuable learning experiences, teaching what works and what doesn’t.
Coding Experiments
Programmers, too, benefit from experimentation. Trying out new algorithms, exploring different programming paradigms, or testing various libraries can lead to more efficient or elegant solutions. Platforms like AlgoCademy encourage this kind of experimentation by providing a safe environment to test and refine coding skills.
For instance, a programmer might experiment with different sorting algorithms to find the most efficient one for a specific dataset:
function bubbleSort(arr) {
const n = arr.length;
for (let i = 0; i < n - 1; i++) {
for (let j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
[arr[j], arr[j + 1]] = [arr[j + 1], arr[j]];
}
}
}
return arr;
}
function quickSort(arr) {
if (arr.length <= 1) return arr;
const pivot = arr[arr.length - 1];
const left = [], right = [];
for (let i = 0; i < arr.length - 1; i++) {
if (arr[i] < pivot) left.push(arr[i]);
else right.push(arr[i]);
}
return [...quickSort(left), pivot, ...quickSort(right)];
}
const arr = [64, 34, 25, 12, 22, 11, 90];
console.log(bubbleSort([...arr]));
console.log(quickSort([...arr]));
By implementing and comparing different sorting algorithms, a programmer can gain insights into their efficiency and suitability for various scenarios.
The Importance of Planning
Both cooking and coding benefit greatly from careful planning and preparation.
Mise en Place in Cooking
In professional kitchens, chefs use a concept called “mise en place,” which means “everything in its place.” This involves preparing and organizing all ingredients before cooking begins. This preparation ensures smooth execution and helps prevent mistakes or forgotten ingredients.
Planning in Coding
In programming, planning is equally important. Before writing any code, experienced developers often create flowcharts, write pseudocode, or sketch out system architectures. This planning phase helps identify potential issues early and ensures a more structured approach to problem-solving.
For example, before implementing a complex algorithm, a programmer might write pseudocode like this:
// Function to find the longest palindromic substring
function longestPalindromicSubstring(s):
if length of s is less than 2, return s
Initialize start and maxLength to 0
For each character i in s:
Check for odd-length palindromes centered at i
Update start and maxLength if a longer palindrome is found
Check for even-length palindromes centered at i and i+1
Update start and maxLength if a longer palindrome is found
Return substring of s from start to start + maxLength
This pseudocode outlines the logic of the algorithm without getting bogged down in syntax details, making it easier to plan and implement the actual code.
The Power of Iteration
Both cooking and coding often involve a process of continuous improvement and refinement.
Iterative Cooking
Chefs rarely perfect a recipe on the first try. Instead, they cook a dish multiple times, tweaking ingredients, adjusting cooking times, and refining techniques with each iteration. This process of continuous improvement leads to better, more refined dishes over time.
Iterative Coding
Similarly, in programming, the first version of a program is rarely the best. Developers often write an initial implementation, then refactor and optimize their code over multiple iterations. This iterative approach is fundamental to agile development methodologies and is crucial for creating efficient, maintainable software.
Consider this example of iterative improvement in code:
// Initial implementation
function isPrime(n) {
if (n <= 1) return false;
for (let i = 2; i < n; i++) {
if (n % i === 0) return false;
}
return true;
}
// Improved implementation
function isPrime(n) {
if (n <= 1) return false;
if (n <= 3) return true;
if (n % 2 === 0 || n % 3 === 0) return false;
for (let i = 5; i * i <= n; i += 6) {
if (n % i === 0 || n % (i + 2) === 0) return false;
}
return true;
}
console.log(isPrime(997)); // A large prime number
The improved version is much more efficient, especially for large numbers, demonstrating how iteration can lead to better solutions.
The Importance of Learning from Others
Both cooking and coding benefit immensely from learning from others and building upon existing knowledge.
Culinary Traditions
In cooking, chefs often draw inspiration from traditional recipes and techniques passed down through generations. They learn from master chefs, cookbooks, and culinary schools, building upon this foundation to create their own unique dishes.
Coding Communities
Similarly, in programming, developers rarely work in isolation. They learn from tutorials, documentation, and online communities. Platforms like Stack Overflow and GitHub allow programmers to share knowledge, collaborate on projects, and learn from each other’s code.
AlgoCademy exemplifies this collaborative learning approach in the coding world. It provides a structured environment where learners can access curated resources, interactive tutorials, and AI-powered assistance. This guided learning experience helps bridge the gap between novice and expert, much like how an apprentice chef might learn under the guidance of a seasoned professional.
The Role of Tools
Both cooking and coding rely heavily on the right tools to achieve the best results.
Kitchen Tools
In the kitchen, having the right tools can make a significant difference. A sharp knife, a reliable oven, or a high-quality food processor can greatly enhance a chef’s ability to create delicious meals efficiently.
Coding Tools
In programming, tools are equally important. Integrated Development Environments (IDEs), version control systems, debugging tools, and libraries all contribute to a developer’s productivity and code quality.
AlgoCademy serves as a comprehensive toolset for aspiring programmers. It provides an integrated environment where learners can write, test, and debug code, all while receiving real-time feedback and guidance. This is similar to how a well-equipped kitchen allows a chef to focus on creativity and execution rather than struggling with inadequate tools.
The Satisfaction of Creating
Perhaps the most significant similarity between cooking and coding is the profound satisfaction that comes from creating something from scratch.
Culinary Creation
There’s a unique joy in taking raw ingredients and transforming them into a delicious meal. The process of cooking allows for personal expression and creativity, resulting in a tangible product that can be shared and enjoyed with others.
Code Creation
Similarly, programming offers the satisfaction of building something from nothing but logic and creativity. Whether it’s a simple script that automates a task or a complex application that solves real-world problems, the act of coding allows developers to bring their ideas to life in a tangible, functional form.
AlgoCademy taps into this creative satisfaction by providing challenges and projects that allow learners to apply their skills to real-world scenarios. This hands-on approach not only reinforces learning but also gives students the joy of creating functional programs, much like how a cooking class might culminate in students preparing a full meal.
Conclusion: The Recipe for Success
The parallels between cooking and coding are numerous and profound. Both disciplines require precision, timing, patience, creativity, planning, iteration, learning from others, the right tools, and offer the satisfaction of creation. By recognizing these similarities, aspiring programmers can apply lessons from the culinary world to their coding journey.
Platforms like AlgoCademy serve as the perfect “cooking school” for code, providing a structured environment where learners can develop their skills, experiment with new “recipes” (algorithms and coding patterns), and gradually progress from simple “dishes” (basic programs) to complex “gourmet meals” (sophisticated applications).
Whether you’re sautéing vegetables or sorting arrays, remember that mastery comes with practice, patience, and a willingness to learn. So, the next time you find yourself stuck on a coding problem, take a step back, think about how you might approach a challenging recipe, and apply those same principles to your code. You might just find that the solution is as satisfying as a perfectly cooked meal.
Happy coding, and bon appétit!