How to Practice Explaining Your Code as a Non-Technical Person Would Understand It
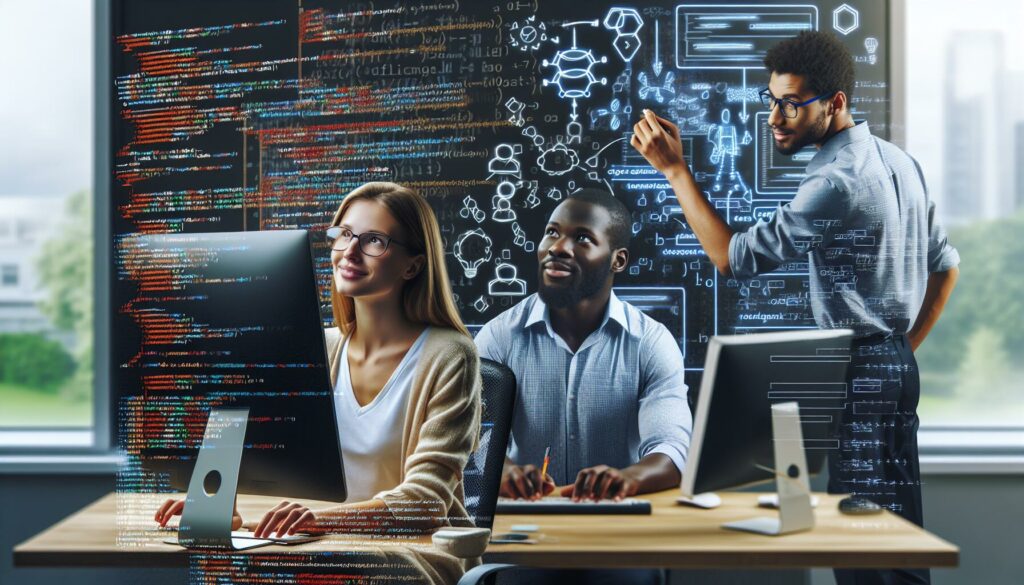
As a programmer, one of the most valuable skills you can develop is the ability to explain your code to non-technical individuals. This skill not only enhances your communication abilities but also deepens your understanding of the code you write. In this comprehensive guide, we’ll explore various techniques and strategies to help you practice explaining your code in a way that anyone can understand, regardless of their technical background.
Why Is This Skill Important?
Before diving into the methods, let’s understand why being able to explain code to non-technical people is crucial:
- Improved collaboration: In most work environments, you’ll need to communicate with non-technical team members, stakeholders, or clients.
- Better problem-solving: Explaining complex concepts in simple terms often leads to new insights and solutions.
- Career advancement: The ability to bridge the gap between technical and non-technical worlds is highly valued in leadership roles.
- Enhanced learning: Teaching others reinforces your own understanding and helps identify gaps in your knowledge.
1. Start with the Big Picture
When explaining your code, it’s tempting to dive straight into the details. However, starting with the overall purpose and context can make your explanation much more accessible.
Practice Exercise:
Take a piece of code you’ve written and try to explain its purpose in one sentence, without using any technical terms. For example:
“This code helps a store keep track of how many items are left in stock and alerts the manager when it’s time to order more.”
2. Use Analogies and Real-World Examples
Analogies are powerful tools for explaining complex concepts. They help bridge the gap between the abstract world of code and familiar, everyday experiences.
Practice Exercise:
Choose a programming concept (e.g., a loop, a function, or a data structure) and come up with three different analogies to explain it. For instance, explaining a function:
- “A function is like a recipe. It takes ingredients (inputs), follows a set of instructions, and produces a dish (output).”
- “Think of a function as a vending machine. You put in money (input), select an item (process), and receive your snack (output).”
- “A function is similar to a factory assembly line. Raw materials go in, a specific process is followed, and a finished product comes out.”
3. Visualize the Code
Visual representations can greatly enhance understanding, especially for non-technical individuals. Practice creating simple diagrams or flowcharts to illustrate your code’s logic.
Practice Exercise:
Take a simple algorithm you’ve implemented (e.g., a sorting algorithm or a search function) and create a flowchart representing its steps. Use basic shapes and minimal text. Share this with a non-technical friend and see if they can follow the logic.
4. Break It Down into Steps
Complex processes become more digestible when broken down into smaller, manageable steps. This approach helps non-technical individuals follow the logic more easily.
Practice Exercise:
Choose a function from your code and break down its operation into 5-7 simple steps. Avoid using technical jargon. For example, explaining a function that calculates the average of a list of numbers:
- The function receives a list of numbers.
- It counts how many numbers are in the list.
- It adds up all the numbers in the list.
- It divides the total sum by the count of numbers.
- It returns this final result as the average.
5. Use Metaphors
Metaphors, like analogies, can help translate abstract concepts into more familiar territory. They can make complex ideas more relatable and easier to grasp.
Practice Exercise:
Create metaphors for different programming concepts. For example:
- “Variables are like labeled boxes where you can store different types of information.”
- “A database is like a giant filing cabinet where all the important information is organized and stored.”
- “An API is like a waiter in a restaurant, taking your order (request) to the kitchen (server) and bringing back your food (data).”
6. Explain the ‘Why’ Not Just the ‘How’
Often, understanding why certain code exists or why a particular approach was chosen is more valuable than knowing the exact implementation details.
Practice Exercise:
Select a piece of code you’ve written and explain:
- What problem it solves
- Why this solution was chosen over alternatives
- What benefits this approach provides
Try to do this without mentioning any specific programming concepts or syntax.
7. Use Everyday Language
Replacing technical terms with everyday language can make your explanations more accessible. This doesn’t mean dumbing down the content, but rather finding more relatable ways to express complex ideas.
Practice Exercise:
Create a “translation guide” for common programming terms. For example:
- “Iterate” → “Go through each item one by one”
- “Concatenate” → “Join or combine”
- “Boolean” → “Yes/No or True/False value”
- “Parameter” → “Information the function needs to do its job”
8. Interactive Explanations
Engaging your audience in the explanation process can significantly improve understanding. Interactive elements make the learning process more dynamic and memorable.
Practice Exercise:
Design a simple “unplugged” activity (no computers involved) that demonstrates a programming concept. For instance, to explain sorting algorithms:
- Write numbers on index cards.
- Give the cards to a group of people.
- Guide them through the steps of a sorting algorithm (e.g., bubble sort) to arrange themselves in order.
9. Relate to Familiar Software or Apps
Drawing parallels between your code and widely-used software or apps can provide context and make the explanation more relatable.
Practice Exercise:
Think of popular apps or software (e.g., social media platforms, productivity tools) and explain how certain features might work behind the scenes. For example:
- “The way a social media feed updates is similar to how our notification system works. It constantly checks for new information and displays it when available.”
- “Our search function works a bit like Google. It looks through all the available information and tries to find the most relevant matches based on what you typed.”
10. Practice Progressive Disclosure
Start with the basics and gradually introduce more complex concepts as needed. This approach prevents overwhelming the listener and allows for a more natural learning progression.
Practice Exercise:
Take a complex feature in your code and create three levels of explanation:
- Basic: A one-sentence description of what it does.
- Intermediate: A paragraph explaining the main components and how they interact.
- Advanced: A detailed walkthrough of the implementation, including potential challenges and solutions.
11. Use Storytelling Techniques
Framing your explanation as a story can make it more engaging and easier to follow. People naturally connect with narratives and often remember information better when it’s presented in a story format.
Practice Exercise:
Create a short story that explains how a piece of your code works. Include characters (which could represent different parts of the code), a setting (the context or environment), and a plot (the flow of execution). For example:
“Imagine a busy restaurant kitchen. The head chef (main function) receives orders (input) from the waitstaff. She then delegates tasks to different station chefs (helper functions). The salad chef prepares appetizers, the grill master cooks the main course, and the pastry chef handles desserts. Each chef follows their recipes (algorithms) to prepare their part of the meal. Finally, the head chef reviews all the dishes (output) before they’re sent out to the diners.”
12. Leverage Comparisons and Contrasts
Comparing and contrasting your code or its concepts with familiar ideas can highlight key features and improve understanding.
Practice Exercise:
Choose two related programming concepts (e.g., arrays and linked lists, or for loops and while loops) and create a comparison table. Use non-technical language to describe their similarities and differences. For instance:
For Loop | While Loop |
---|---|
Like a countdown timer with a set number of beeps | Like an alarm clock that keeps ringing until you turn it off |
You know exactly how many times it will run | Keeps going until a certain condition is met |
Good for when you have a specific list to go through | Useful when you’re not sure how many times you need to do something |
13. Use Simplified Code Examples
While explaining code to non-technical people, it can be helpful to use extremely simplified code examples that focus on the concept rather than syntax.
Practice Exercise:
Create “pseudo-code” versions of your actual code that use plain English instead of programming syntax. For example, a function to calculate the area of a rectangle might look like this:
Function: Calculate Rectangle Area
Inputs: length, width
Steps:
1. Multiply length by width
2. Store the result as area
3. Give back the area
End Function
14. Incorporate Feedback and Iteration
Improving your explanation skills is an iterative process. Regularly seek feedback and refine your approach based on what works best for your audience.
Practice Exercise:
After explaining a concept:
- Ask the listener to summarize what they understood.
- Identify any points of confusion or misunderstanding.
- Revise your explanation to address these issues.
- Keep a log of successful explanations and techniques for future reference.
15. Use Analogies from Different Fields
Drawing analogies from various fields can help cater to different backgrounds and interests, making your explanations more universally accessible.
Practice Exercise:
Take a programming concept and create analogies from at least three different fields. For example, explaining “debugging”:
- Medical: “Debugging is like diagnosing an illness. You observe the symptoms (errors), run tests (add print statements or use a debugger), and then prescribe a treatment (fix the code).”
- Detective Work: “Debugging is similar to solving a mystery. You gather clues (error messages), interview witnesses (check logs), and piece together the evidence to solve the case (fix the bug).”
- Gardening: “Debugging is like weeding a garden. You identify unwanted elements (bugs), carefully remove them without damaging the surrounding plants (code), and ensure the garden (program) can grow healthily.”
16. Practice Active Listening
Effective explanation isn’t just about talking; it’s also about listening and adapting to your audience’s needs and level of understanding.
Practice Exercise:
When explaining a concept:
- Pause regularly and ask if there are any questions.
- Encourage the listener to rephrase what you’ve said in their own words.
- Pay attention to non-verbal cues that might indicate confusion or understanding.
- Be prepared to revisit or rephrase parts of your explanation based on feedback.
17. Use Physical Props or Demonstrations
Sometimes, abstract concepts become clearer when represented by physical objects or actions.
Practice Exercise:
Create a “code explanation kit” with everyday items that can represent different programming concepts. For example:
- Use building blocks to represent modular code structure
- Demonstrate sorting algorithms with a deck of cards
- Use a series of cups filled with water to explain variables and data types
18. Relate to Problem-Solving in Daily Life
Connecting coding concepts to everyday problem-solving can make them more relatable and understandable.
Practice Exercise:
Take a common algorithm or programming pattern and explain how it relates to a daily task. For instance:
- Explain how finding the shortest route on a map app is similar to pathfinding algorithms in programming.
- Compare the process of following a recipe to executing a function with parameters.
- Relate the concept of caching to keeping frequently used items within easy reach in a kitchen.
19. Create Mini-Challenges
Engaging your audience with small, manageable challenges can reinforce understanding and make the learning process more interactive.
Practice Exercise:
Design simple, non-technical challenges that demonstrate programming concepts. For example:
- To explain loops: Ask the person to write down steps for brushing teeth, but they can only use 10 words total (encouraging them to use repetition, like “Repeat 10 times: Brush up and down”).
- To explain conditionals: Create a simple flowchart for deciding what to wear based on weather conditions.
20. Use Humor and Memorable Phrases
Incorporating humor and crafting memorable phrases or mnemonics can make your explanations more engaging and easier to remember.
Practice Exercise:
Create humorous analogies or memorable phrases for common programming terms or concepts. For example:
- “A bug is just the computer’s way of saying ‘You’re not the boss of me!'”
- “Remember, in programming, DRY means ‘Don’t Repeat Yourself’, not ‘Do Repeat Yourself’.”
- “Recursion is like a Russian nesting doll – it’s dolls all the way down until you hit the base case!”
Conclusion
Mastering the art of explaining code to non-technical individuals is a valuable skill that enhances your effectiveness as a programmer and team member. By consistently practicing these techniques, you’ll not only improve your communication skills but also deepen your own understanding of the code you write.
Remember, the key to successful explanation lies in empathy, patience, and the ability to bridge the gap between technical concepts and everyday experiences. As you continue to refine your explanation skills, you’ll find that you become not just a better communicator, but a more thoughtful and effective programmer overall.
Keep practicing, stay curious about different ways to express ideas, and always be open to feedback. With time and effort, you’ll develop the ability to make even the most complex code understandable to anyone, regardless of their technical background.