How to Break Down Large Problems in Coding Interviews (Even When They Seem Impossible)
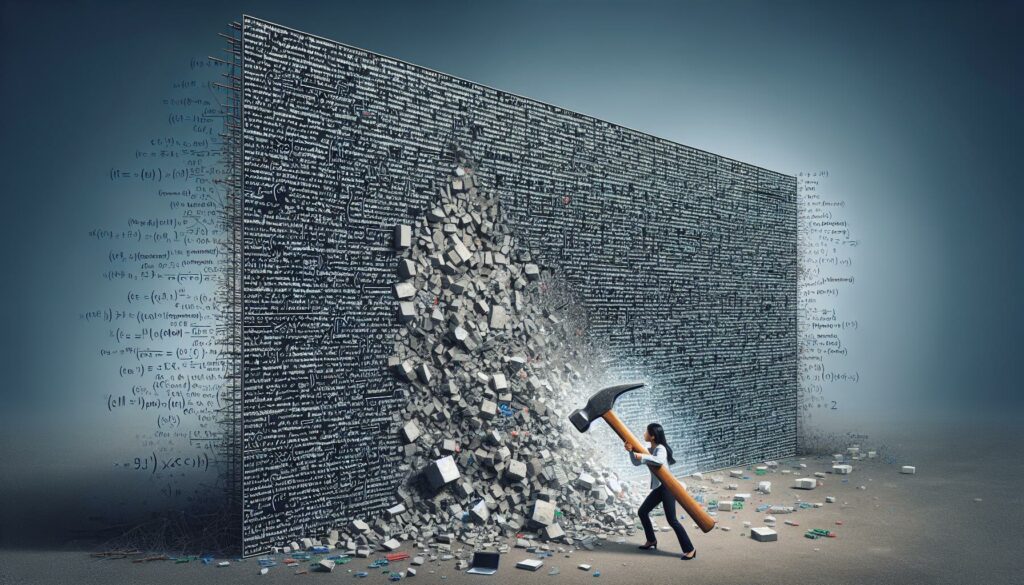
Coding interviews can be daunting, especially when you’re faced with a complex problem that seems impossible to solve at first glance. However, the ability to break down large problems into manageable pieces is a crucial skill that can make all the difference in your interview performance. In this comprehensive guide, we’ll explore effective strategies to tackle even the most challenging coding problems, helping you approach your next technical interview with confidence.
1. Understanding the Importance of Problem Breakdown
Before we dive into specific techniques, it’s essential to understand why breaking down large problems is so crucial in coding interviews:
- Manageable chunks: Dividing a complex problem into smaller parts makes it less overwhelming and more approachable.
- Structured thinking: It demonstrates your ability to think systematically and organize your thoughts.
- Progress tracking: Solving smaller components gives you a sense of progress and keeps you motivated.
- Easier debugging: When issues arise, it’s simpler to isolate and fix problems in smaller sections of code.
- Impressive to interviewers: Showcasing your problem-solving approach can be just as important as reaching the final solution.
2. The UMPIRE Method: A Systematic Approach
One effective framework for breaking down coding problems is the UMPIRE method. This acronym stands for:
- Understand the problem
- Match the problem to potential algorithms
- Plan the approach
- Implement the solution
- Review the implementation
- Evaluate the solution
Let’s explore each step in detail:
2.1. Understand the Problem
Before you start coding, make sure you fully grasp the problem at hand:
- Read the problem statement carefully, multiple times if necessary.
- Identify the input and expected output.
- Ask clarifying questions about edge cases or ambiguous aspects.
- Restate the problem in your own words to confirm understanding.
2.2. Match the Problem to Potential Algorithms
Once you understand the problem, consider which algorithms or data structures might be applicable:
- Identify patterns that resemble known algorithmic problems.
- Consider common data structures (arrays, linked lists, trees, graphs, etc.) that might be useful.
- Think about algorithmic paradigms like divide-and-conquer, dynamic programming, or greedy algorithms.
2.3. Plan the Approach
Before diving into code, outline your solution:
- Break the problem into smaller, manageable sub-problems.
- Sketch out a high-level algorithm or pseudocode.
- Consider time and space complexity requirements.
- Discuss your approach with the interviewer to get early feedback.
2.4. Implement the Solution
Now it’s time to write the actual code:
- Start with a basic implementation, focusing on correctness rather than optimization.
- Use clear variable names and add comments to explain your thought process.
- Implement one sub-problem at a time, testing as you go.
- Don’t hesitate to use helper functions to keep your main logic clean and modular.
2.5. Review the Implementation
After coding, take a step back and review your work:
- Walk through your code with a simple example to ensure it works as expected.
- Check for any obvious bugs or edge cases you might have missed.
- Consider if there are any parts that could be optimized or simplified.
2.6. Evaluate the Solution
Finally, analyze your solution critically:
- Discuss the time and space complexity of your implementation.
- Consider alternative approaches and their trade-offs.
- Reflect on what you learned from solving this problem.
3. Practical Techniques for Breaking Down Complex Problems
Now that we’ve covered the UMPIRE framework, let’s explore some specific techniques you can use to break down large coding problems:
3.1. Divide and Conquer
The divide and conquer approach involves breaking a problem into smaller, similar sub-problems, solving them independently, and then combining the results.
Example: Merge Sort
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
3.2. Start with a Brute Force Solution
Begin with the simplest, most straightforward solution, even if it’s inefficient. This helps you understand the problem better and provides a baseline for optimization.
Example: Finding the maximum subarray sum
def max_subarray_sum_brute_force(arr):
max_sum = float('-inf')
for i in range(len(arr)):
for j in range(i, len(arr)):
current_sum = sum(arr[i:j+1])
max_sum = max(max_sum, current_sum)
return max_sum
3.3. Solve a Simpler Version First
If the problem seems too complex, try solving a simplified version first, then gradually add complexity.
Example: Instead of finding the kth largest element in an unsorted array, start by finding the largest element, then the second largest, and so on.
3.4. Use Concrete Examples
Work through specific examples to gain insights into the problem’s patterns and edge cases.
Example: When solving a string manipulation problem, try it with short strings, long strings, strings with repeated characters, empty strings, etc.
3.5. Look for Patterns or Symmetry
Many problems have underlying patterns or symmetries that can simplify the solution.
Example: In dynamic programming problems, identifying overlapping subproblems can lead to more efficient solutions.
3.6. Work Backwards
Sometimes, starting from the desired output and working backwards can provide insights into the solution.
Example: In graph problems, consider the properties of the final state and how you might reach it from the initial state.
4. Common Pitfalls to Avoid
While breaking down problems, be aware of these common mistakes:
- Jumping to code too quickly: Take time to plan and understand the problem thoroughly before coding.
- Ignoring edge cases: Consider empty inputs, negative numbers, overflow scenarios, etc.
- Overcomplicating the solution: Sometimes, a simple approach is best. Don’t add unnecessary complexity.
- Failing to communicate: Keep the interviewer informed about your thought process throughout.
- Getting stuck on one approach: Be willing to pivot if your initial strategy isn’t working.
5. Practice Makes Perfect
Improving your ability to break down complex problems requires consistent practice. Here are some ways to hone your skills:
- Solve diverse problems: Practice with a variety of problem types to broaden your problem-solving toolkit.
- Time yourself: Work on solving problems within realistic time constraints to simulate interview conditions.
- Explain your solutions: Practice articulating your thought process, either to a study partner or by writing it down.
- Review and reflect: After solving a problem, consider alternative approaches and areas for improvement.
- Use platforms like AlgoCademy: Take advantage of interactive coding tutorials and AI-powered assistance to guide your learning.
6. Real-World Example: Breaking Down a Complex Problem
Let’s walk through an example of breaking down a complex problem using the techniques we’ve discussed. Consider the following interview question:
“Design a data structure that supports inserting, removing, and getting a random element, all in O(1) time complexity.”
Here’s how we might approach this problem using the UMPIRE method:
6.1. Understand the Problem
- We need a data structure that supports three operations: insert, remove, and getRandom.
- All operations should have O(1) time complexity.
- Clarifying question: Should duplicate elements be allowed?
6.2. Match to Potential Algorithms/Data Structures
- Hash table for O(1) insert and remove.
- Array for O(1) random access.
- We might need to combine these data structures.
6.3. Plan the Approach
- Use a hash table to store elements for O(1) insert and remove.
- Use an array to support O(1) random access.
- Maintain a mapping between the hash table and array indices.
- For removal, swap the element with the last element in the array before deleting.
6.4. Implement the Solution
Here’s a Python implementation of our planned approach:
import random
class RandomizedSet:
def __init__(self):
self.elements = []
self.element_to_index = {}
def insert(self, val: int) -> bool:
if val in self.element_to_index:
return False
self.elements.append(val)
self.element_to_index[val] = len(self.elements) - 1
return True
def remove(self, val: int) -> bool:
if val not in self.element_to_index:
return False
index = self.element_to_index[val]
last_element = self.elements[-1]
# Swap with the last element
self.elements[index] = last_element
self.element_to_index[last_element] = index
# Remove the last element
self.elements.pop()
del self.element_to_index[val]
return True
def getRandom(self) -> int:
return random.choice(self.elements)
6.5. Review the Implementation
- The solution uses a list (self.elements) and a dictionary (self.element_to_index) to achieve O(1) time complexity for all operations.
- Insert: Appends to the list and updates the dictionary.
- Remove: Swaps with the last element before removing to maintain O(1) time complexity.
- GetRandom: Uses Python’s random.choice() for O(1) random selection.
6.6. Evaluate the Solution
- Time Complexity: O(1) for all operations as required.
- Space Complexity: O(n) where n is the number of elements.
- Trade-offs: The solution uses extra space to achieve the required time complexity.
- Potential improvement: We could discuss handling edge cases like empty sets more explicitly.
7. Conclusion
Breaking down large problems in coding interviews is a skill that can be developed with practice and the right approach. By using frameworks like UMPIRE and employing techniques such as divide and conquer, starting with brute force solutions, and working with concrete examples, you can tackle even the most challenging interview questions with confidence.
Remember, the goal in a coding interview is not just to arrive at the correct solution, but to demonstrate your problem-solving process. By effectively breaking down problems, you showcase your ability to think critically, communicate clearly, and approach complex challenges methodically – all valuable skills in the eyes of potential employers.
As you continue to practice and refine your problem-solving skills, consider leveraging resources like AlgoCademy, which offers interactive coding tutorials and AI-powered assistance to help you progress from beginner-level coding to mastering technical interviews. With dedication and the right strategies, you’ll be well-prepared to tackle any coding challenge that comes your way in your next interview.