The Hidden Benefits of Teaching Others While Preparing for Coding Interviews
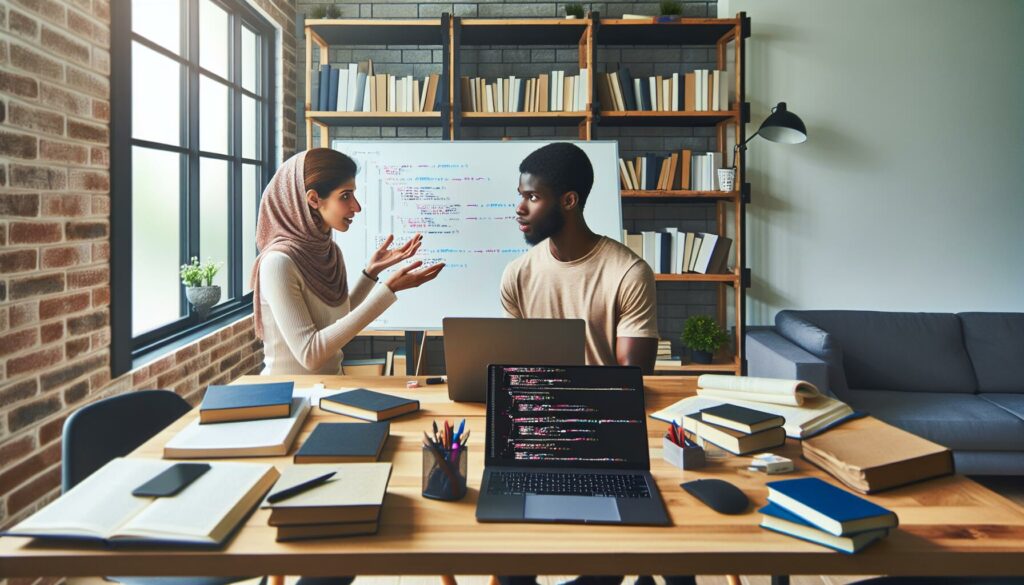
When it comes to preparing for coding interviews, especially for prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), most developers focus on solving problems, memorizing algorithms, and practicing data structures. While these are undoubtedly crucial aspects of interview preparation, there’s a powerful yet often overlooked strategy that can significantly boost your chances of success: teaching others.
In this comprehensive guide, we’ll explore the hidden benefits of teaching others while preparing for coding interviews. We’ll delve into how this approach can enhance your understanding, improve your communication skills, and ultimately make you a more attractive candidate to potential employers.
The Power of Teaching in Learning
Before we dive into the specific benefits of teaching in the context of coding interviews, let’s first understand why teaching is such a powerful learning tool in general.
The Protégé Effect
The “protégé effect” is a psychological phenomenon where teaching others helps the teacher learn and retain information better. When you teach a concept to someone else, you’re forced to organize your thoughts, break down complex ideas into simpler terms, and find ways to explain things clearly. This process of reformulating and articulating knowledge reinforces your own understanding and helps identify gaps in your knowledge.
Active Recall
Teaching involves active recall, which is one of the most effective learning techniques. When you teach, you’re not just passively reviewing information; you’re actively retrieving it from your memory and applying it in real-time. This strengthens the neural pathways associated with that knowledge, making it easier to recall in the future.
Deeper Understanding
To teach effectively, you need to understand a concept deeply enough to explain it in multiple ways and answer questions about it. This pursuit of deeper understanding can lead you to explore aspects of a topic you might have overlooked when studying alone.
Benefits Specific to Coding Interview Preparation
Now that we’ve established the general benefits of teaching, let’s explore how these advantages specifically apply to preparing for coding interviews.
1. Mastery of Fundamental Concepts
Coding interviews often test your understanding of fundamental computer science concepts. By teaching these concepts to others, you’re forced to revisit and solidify your own understanding. For example, explaining how a binary search tree works to a beginner will ensure that you truly grasp the concept inside and out.
Example: Teaching Binary Search
Let’s say you’re teaching someone how to implement a binary search algorithm. You might start by explaining the concept:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
result = binary_search(sorted_array, 7)
print(f"Element found at index: {result}")
As you explain this algorithm, you’ll naturally delve into topics like time complexity, the importance of the array being sorted, and how to handle edge cases. This process reinforces your own understanding and prepares you to discuss these concepts confidently in an interview setting.
2. Improved Problem-Solving Skills
When you teach problem-solving techniques to others, you’re not just sharing knowledge; you’re also honing your own problem-solving skills. You’ll learn to approach problems from different angles and develop a more structured thought process.
Example: Teaching Two-Pointer Technique
Suppose you’re explaining the two-pointer technique for solving array problems. You might use an example like finding a pair of numbers in a sorted array that sum up to a target value:
def find_pair_with_sum(arr, target):
left, right = 0, len(arr) - 1
while left < right:
current_sum = arr[left] + arr[right]
if current_sum == target:
return [arr[left], arr[right]]
elif current_sum < target:
left += 1
else:
right -= 1
return None
# Example usage
sorted_array = [1, 2, 3, 4, 5, 6, 7, 8, 9]
target_sum = 10
result = find_pair_with_sum(sorted_array, target_sum)
print(f"Pair found: {result}")
By walking someone through this problem and its solution, you’ll reinforce your understanding of when and how to apply the two-pointer technique, making you better prepared to tackle similar problems in interviews.
3. Enhanced Communication Skills
One of the most valuable skills in coding interviews is the ability to clearly communicate your thought process. When you teach others, you’re constantly practicing this skill. You learn to explain complex concepts in simple terms, which is exactly what interviewers look for.
Tips for Improving Communication While Teaching:
- Use analogies to relate coding concepts to real-world scenarios
- Break down complex problems into smaller, manageable steps
- Encourage questions and practice answering them concisely
- Use visual aids like diagrams or pseudocode to illustrate concepts
4. Exposure to Different Perspectives
When you teach, you’re not just imparting knowledge; you’re also learning from your students. Their questions and alternative approaches can provide new insights and perspectives that you might not have considered. This exposure to different ways of thinking can be invaluable in coding interviews, where creative problem-solving is often rewarded.
5. Building a Support Network
Teaching others in the coding community can help you build a network of fellow learners and professionals. This network can provide emotional support during your job search, offer additional learning resources, and even lead to job opportunities through referrals.
6. Reinforcing Your Own Knowledge
The act of teaching forces you to revisit and reinforce your own knowledge. You might discover gaps in your understanding that you weren’t aware of, giving you the opportunity to fill those gaps before your interviews.
Example: Reinforcing Knowledge of Time Complexity
Let’s say you’re teaching time complexity analysis. You might create an exercise comparing different sorting algorithms:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Example usage
unsorted_array = [64, 34, 25, 12, 22, 11, 90]
print(f"Bubble Sort: {bubble_sort(unsorted_array.copy())}")
print(f"Merge Sort: {merge_sort(unsorted_array.copy())}")
As you explain the time complexity of these algorithms (O(n^2) for Bubble Sort and O(n log n) for Merge Sort), you’ll reinforce your own understanding of algorithmic efficiency, a crucial topic in coding interviews.
7. Developing Leadership and Mentorship Skills
Teaching others demonstrates leadership and mentorship abilities, which are highly valued in the tech industry. Many companies look for developers who can not only code well but also guide and support their teammates.
How to Incorporate Teaching into Your Interview Preparation
Now that we’ve explored the benefits, let’s look at some practical ways to incorporate teaching into your coding interview preparation:
1. Start a Study Group
Organize a regular study group with fellow developers preparing for interviews. Take turns leading sessions on different topics. This provides a structured environment for teaching and learning.
2. Contribute to Online Forums
Participate in coding forums like Stack Overflow or Reddit’s programming communities. Answer questions from beginners and explain concepts clearly. This asynchronous form of teaching can fit easily into your schedule.
3. Create Content
Start a blog or YouTube channel where you explain coding concepts and solve interview-style problems. The process of creating content will force you to deeply understand and articulate complex ideas.
4. Mentor a Junior Developer
If you have the opportunity, mentor a junior developer at work or through a mentorship program. This one-on-one interaction can provide invaluable teaching experience.
5. Pair Programming
Engage in pair programming sessions where you alternate between “driver” (writing code) and “navigator” (guiding and explaining). This mimics the collaborative nature of many coding interviews.
6. Teach a Coding Workshop
Organize a coding workshop at a local meetup or online. This larger-scale teaching experience can significantly boost your confidence and communication skills.
Overcoming Challenges in Teaching While Preparing for Interviews
While teaching others offers numerous benefits, it’s not without its challenges. Here are some common obstacles you might face and how to overcome them:
1. Time Management
Challenge: Teaching takes time, which you might feel could be better spent on personal study.
Solution: View teaching as an integral part of your preparation, not a separate activity. Set specific time blocks for teaching and personal study to ensure a balance.
2. Imposter Syndrome
Challenge: You might feel you’re not qualified enough to teach others, especially when preparing for high-stakes interviews.
Solution: Remember that you don’t need to be an expert to teach. Focus on topics you’re comfortable with and be honest about what you’re still learning. Your journey can inspire others.
3. Dealing with Difficult Questions
Challenge: You might encounter questions you can’t answer immediately.
Solution: Use these moments as learning opportunities. Research the answer together or promise to follow up. This demonstrates humility and a commitment to continuous learning, both valuable traits in the tech industry.
4. Maintaining Focus on Interview Preparation
Challenge: It’s easy to get sidetracked into teaching topics that aren’t directly relevant to coding interviews.
Solution: Create a curriculum aligned with common interview topics. Use resources like LeetCode or HackerRank to guide your teaching content.
Real-world Success Stories
To illustrate the power of teaching in interview preparation, let’s look at a couple of real-world success stories:
Case Study 1: Sarah’s Journey
Sarah, a self-taught developer, was preparing for her first technical interviews. She started a small study group with three other aspiring developers. By taking turns explaining concepts and solving problems together, Sarah found that her understanding of data structures and algorithms improved dramatically. The practice of articulating her thought process while coding helped her shine in her interviews, ultimately landing her a job at a top tech company.
Case Study 2: Mike’s Blog
Mike, an experienced developer looking to transition to a FAANG company, started a technical blog as part of his interview preparation. He committed to writing one article per week explaining a complex algorithm or system design concept. The process of researching, writing, and responding to comments forced Mike to develop a deep understanding of these topics. During his interviews, he was able to discuss these concepts with confidence and clarity, impressing his interviewers and securing multiple job offers.
Conclusion
Preparing for coding interviews, especially for top tech companies, can be a daunting task. However, by incorporating teaching into your preparation strategy, you can gain a significant advantage. Not only will you reinforce your own knowledge and improve your problem-solving skills, but you’ll also develop crucial soft skills that are highly valued in the tech industry.
Remember, the goal of a coding interview is not just to solve problems, but to demonstrate your ability to think critically, communicate effectively, and collaborate with others. By teaching, you’re honing all of these skills simultaneously.
So, as you embark on your journey to ace those coding interviews, don’t just study in isolation. Seek out opportunities to teach, explain, and mentor others. You might be surprised at how much you learn in the process, and how much it enhances your performance when it’s time to shine in those all-important interviews.
Happy teaching, and best of luck with your coding interviews!