Learning from Every Wrong Answer: How Mistakes Shape Coding Interview Success
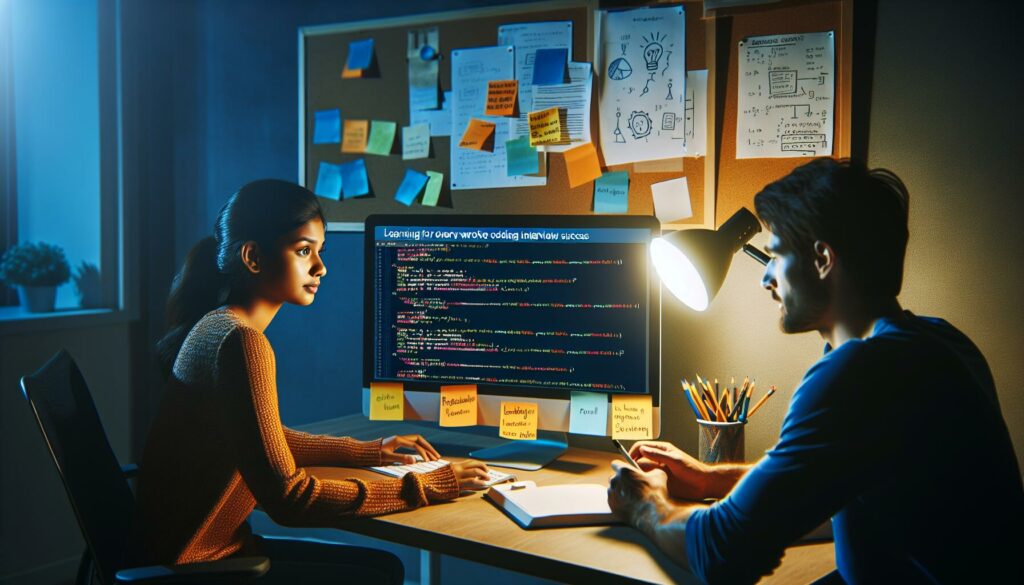
In the world of coding interviews, particularly for prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), the path to success is often paved with numerous challenges and, inevitably, mistakes. However, it’s crucial to understand that these errors are not setbacks but stepping stones to mastery. This article delves into how embracing and learning from mistakes can significantly enhance your coding interview preparation and ultimately lead to success.
The Importance of Mistakes in the Learning Process
Before we dive into specific strategies, it’s essential to recognize the value of mistakes in the learning process. Cognitive science research has consistently shown that making errors, followed by correction and reflection, leads to deeper understanding and better long-term retention of information. This concept, known as “productive failure,” is particularly relevant in the context of coding interviews.
When you encounter a problem you can’t immediately solve or make a mistake in your approach, your brain is forced to engage more deeply with the material. This struggle creates stronger neural connections, making it more likely that you’ll remember the correct solution in the future. In essence, mistakes are not just inevitable; they’re invaluable.
Common Mistakes in Coding Interviews
To effectively learn from mistakes, it’s helpful to categorize the types of errors commonly made in coding interviews. Here are some of the most frequent:
1. Algorithmic Errors
- Choosing the wrong algorithm for the problem
- Implementing an algorithm incorrectly
- Failing to optimize an algorithm for efficiency
2. Data Structure Misuse
- Selecting an inappropriate data structure
- Incorrectly implementing a data structure
- Not leveraging the full capabilities of a chosen data structure
3. Time and Space Complexity Misjudgments
- Underestimating the time complexity of a solution
- Overlooking space complexity considerations
- Failing to recognize trade-offs between time and space
4. Edge Case Oversights
- Not considering empty or null inputs
- Overlooking boundary conditions
- Failing to handle unexpected input types
5. Coding Style and Best Practices
- Writing unclear or poorly organized code
- Not following naming conventions
- Lack of comments or documentation
Strategies for Learning from Mistakes
Now that we’ve identified common mistake categories, let’s explore strategies to turn these errors into learning opportunities:
1. Maintain an Error Log
Keep a detailed record of the mistakes you make during your practice sessions. For each error, note:
- The problem you were attempting to solve
- The mistake you made
- Why you think you made the mistake
- The correct approach or solution
Regularly review this log to identify patterns in your errors and track your progress over time.
2. Implement a Post-Problem Review Process
After solving each practice problem, whether correctly or incorrectly, take time to reflect:
- What went well in your approach?
- What could be improved?
- Are there alternative solutions you didn’t consider?
- How does this problem relate to others you’ve encountered?
This reflection process helps solidify your understanding and broadens your problem-solving toolkit.
3. Embrace the “Explain It to Me” Technique
When you make a mistake, try explaining the problem, your approach, and where you went wrong to an imaginary student or a rubber duck (a technique known as “rubber duck debugging”). This process of verbalization often helps clarify your thinking and can reveal gaps in your understanding.
4. Utilize AI-Powered Assistance
Platforms like AlgoCademy offer AI-powered assistance that can provide targeted feedback on your code. Use these tools to:
- Identify logical errors in your algorithms
- Suggest optimizations for time and space complexity
- Highlight best practices and coding style improvements
Remember, the goal is not just to get the right answer but to understand why it’s right and how to arrive at it efficiently.
5. Implement Spaced Repetition
When you make a mistake on a problem, don’t just move on. Schedule to revisit that problem type after a set interval (e.g., 1 day, 1 week, 1 month). This spaced repetition helps reinforce your learning and ensures that you’re truly mastering the concept, not just memorizing a specific solution.
6. Analyze Official Solutions and Other Approaches
After attempting a problem, regardless of whether you solved it correctly, always review the official solution and other approaches shared by the community. This practice helps you:
- Identify more efficient algorithms or data structures
- Learn new coding techniques and best practices
- Understand different ways of thinking about the same problem
Turning Specific Mistakes into Learning Opportunities
Let’s look at how to learn from specific types of mistakes:
Algorithmic Errors
When you choose the wrong algorithm or implement one incorrectly:
- Review the problem constraints and requirements carefully.
- Create a list of algorithms that could potentially solve the problem.
- Analyze the trade-offs between different algorithmic approaches.
- Implement the chosen algorithm step-by-step, testing each component.
Example: If you mistakenly use a bubble sort for a large dataset, research more efficient sorting algorithms like quicksort or mergesort. Implement each algorithm and compare their performance on various input sizes.
Data Structure Misuse
To improve your data structure selection and implementation:
- Create a cheat sheet of common data structures and their use cases.
- Practice implementing data structures from scratch to understand their internals.
- Analyze the time complexity of operations on different data structures.
Example: If you used an array when a hash table would have been more efficient, implement both solutions and compare their time complexities. This hands-on comparison will help you internalize when to use each data structure.
Time and Space Complexity Misjudgments
To better assess complexity:
- Practice calculating time and space complexity for every solution you write.
- Create worst-case scenarios for your algorithms and analyze their performance.
- Study common complexity classes (e.g., O(n), O(log n), O(n^2)) and their characteristics.
Example: If you underestimated the time complexity of a nested loop solution, implement it and test with increasingly large inputs to observe the performance degradation. Then, try to optimize it and compare the results.
Edge Case Oversights
To improve your handling of edge cases:
- Develop a checklist of common edge cases (e.g., empty input, single element, maximum values).
- Before coding, write down test cases that cover these edge scenarios.
- Implement robust input validation in your solutions.
Example: If you forgot to handle null inputs, create a set of test cases that include null, empty, and valid inputs. Modify your solution to gracefully handle all these cases.
Coding Style and Best Practices
To enhance your code quality:
- Study style guides for your preferred programming language.
- Use linters and code formatters to enforce consistent style.
- Practice writing self-documenting code with clear variable and function names.
- Regularly review and refactor your old code.
Example: If your code was difficult to read due to poor organization, refactor it into smaller, well-named functions. Compare the before and after versions to see how readability improves.
Leveraging Tools and Resources
To maximize your learning from mistakes, take advantage of the following tools and resources:
1. Online Coding Platforms
Websites like LeetCode, HackerRank, and AlgoCademy offer a wealth of coding problems with immediate feedback. Use these platforms to:
- Practice a wide variety of problem types
- Compare your solutions with others
- Analyze the efficiency of your code
Example usage:
<!-- Python code example -->
def two_sum(nums, target):
seen = {}
for i, num in enumerate(nums):
complement = target - num
if complement in seen:
return [seen[complement], i]
seen[num] = i
return []
# Test the function
print(two_sum([2, 7, 11, 15], 9)) # Should output [0, 1]
After implementing your solution, compare it with top-rated solutions on the platform to identify potential improvements in efficiency or readability.
2. Version Control Systems
Use Git or another version control system to track your progress and mistakes over time. This allows you to:
- Review your coding history
- Easily revert to previous versions if needed
- Maintain different branches for various problem-solving approaches
Example Git workflow:
git init
git add solution.py
git commit -m "Initial solution for Two Sum problem"
# After improving the solution
git add solution.py
git commit -m "Optimized Two Sum solution using hash table"
3. Code Profilers
Use profiling tools to analyze the performance of your code. This helps you:
- Identify bottlenecks in your algorithms
- Understand the real-world impact of different data structures
- Verify your time complexity assumptions
Example using Python’s cProfile:
import cProfile
def slow_function():
return sum(i * i for i in range(10000))
cProfile.run('slow_function()')
Analyze the output to identify which parts of your code are consuming the most time and optimize accordingly.
4. Peer Code Reviews
Engage in code reviews with peers or mentors. This practice:
- Exposes you to different problem-solving approaches
- Helps identify blind spots in your coding style
- Improves your ability to read and understand others’ code
Consider joining coding communities or study groups to facilitate regular code reviews.
Psychological Aspects of Learning from Mistakes
While the technical aspects of learning from coding mistakes are crucial, it’s equally important to cultivate the right mindset:
1. Embrace a Growth Mindset
Adopt the belief that your abilities can be developed through dedication and hard work. This mindset helps you:
- View challenges as opportunities for growth
- Persist in the face of setbacks
- Learn from criticism and the success of others
2. Practice Self-Compassion
Be kind to yourself when you make mistakes. Remember that errors are a natural and necessary part of the learning process. This approach helps:
- Reduce stress and anxiety associated with coding interviews
- Maintain motivation during challenging periods
- Foster a positive relationship with the learning process
3. Celebrate Small Wins
Acknowledge and celebrate your progress, no matter how small. This practice:
- Boosts confidence and motivation
- Helps maintain a positive outlook on your coding journey
- Reinforces the habit of continuous improvement
Applying Lessons Learned to Real Interviews
As you accumulate insights from your mistakes, it’s crucial to apply these lessons in actual interview scenarios:
1. Mock Interviews
Regularly participate in mock interviews to:
- Simulate the pressure of real interviews
- Practice articulating your thought process
- Identify areas where you tend to make mistakes under pressure
2. Time Management
Use your experience with mistakes to improve your time management during interviews:
- Allocate time for understanding the problem thoroughly
- Budget time for testing and debugging
- Know when to ask for hints or clarification
3. Communication Skills
Learn to effectively communicate about your mistakes and problem-solving process:
- Practice explaining your approach, including potential pitfalls
- Be transparent about challenges you’re facing during the interview
- Demonstrate your ability to learn and adapt quickly
Conclusion
In the journey towards mastering coding interviews, especially for prestigious companies like FAANG, mistakes are not just inevitable—they’re invaluable. By systematically learning from each error, you transform challenges into opportunities for growth. Remember that every wrong answer brings you one step closer to the right one, and every mistake is a chance to deepen your understanding.
As you continue your preparation, embrace the learning process, stay persistent, and maintain a growth mindset. Utilize the strategies, tools, and resources discussed in this article to turn your mistakes into stepping stones for success. With dedication and the right approach to learning from errors, you’ll not only improve your coding skills but also develop the resilience and problem-solving abilities that are crucial for excelling in technical interviews and your future career in software development.
Remember, the most successful developers aren’t those who never make mistakes—they’re the ones who learn the most from them. So, code on, make mistakes, learn, and grow. Your future self will thank you for every error you encounter and overcome on this challenging but rewarding journey.