Why Learning Dynamic Programming Is Easier Than You Think: Breaking Down the Complexity
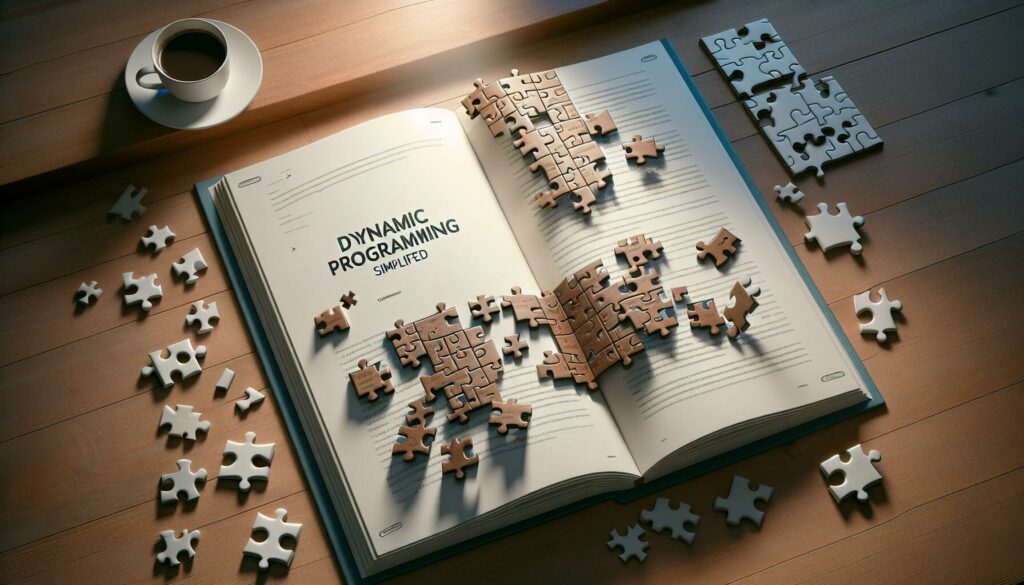
Dynamic Programming (DP) is often considered one of the most challenging concepts in computer science and algorithm design. Many aspiring programmers and even experienced developers shy away from it, thinking it’s too complex or abstract. However, I’m here to tell you that learning dynamic programming is easier than you might think. In this comprehensive guide, we’ll break down the complexity of DP, explore its core principles, and show you how to approach DP problems with confidence.
Understanding Dynamic Programming
Before we dive into why learning dynamic programming is more accessible than you might believe, let’s start with a clear definition of what DP actually is.
Dynamic Programming is an algorithmic paradigm that solves complex problems by breaking them down into simpler subproblems. It is a method for solving optimization problems by caching solutions to overlapping subproblems, thus avoiding redundant computations.
The key characteristics of dynamic programming include:
- Optimal substructure: The optimal solution to the problem can be constructed from optimal solutions of its subproblems.
- Overlapping subproblems: The problem can be broken down into subproblems which are reused several times.
- Memoization: Storing the results of expensive function calls and returning the cached result when the same inputs occur again.
Why Dynamic Programming Seems Intimidating
There are several reasons why dynamic programming might appear daunting at first:
- Abstract concept: DP requires a shift in thinking from straightforward, step-by-step problem-solving to a more recursive and optimized approach.
- Pattern recognition: Identifying when and how to apply DP can be challenging for beginners.
- Mathematical foundation: Some DP problems have a strong mathematical basis, which can be intimidating for those less comfortable with math.
- Complex implementations: DP solutions often involve multi-dimensional arrays or complex data structures, which can be difficult to visualize and implement.
However, these challenges are not insurmountable. With the right approach and mindset, anyone can learn and master dynamic programming.
Breaking Down the Complexity: A Step-by-Step Approach
Let’s break down the process of learning dynamic programming into manageable steps:
1. Understand the Basics
Start by grasping the fundamental concepts of DP:
- Recursion: Many DP problems can be initially solved using recursion.
- Memoization: Learn how to store and reuse previously calculated results.
- Tabulation: Understand how to build solutions iteratively using tables.
2. Recognize Common Patterns
DP problems often fall into certain categories. Familiarize yourself with these common patterns:
- 0/1 Knapsack
- Unbounded Knapsack
- Longest Common Subsequence
- Longest Increasing Subsequence
- Matrix Chain Multiplication
- Coin Change
3. Start with Simple Problems
Begin your DP journey with straightforward problems like:
- Fibonacci sequence
- Climbing stairs
- Maximum subarray sum
4. Practice Regularly
Consistent practice is key to mastering DP. Solve problems regularly and revisit concepts to reinforce your understanding.
5. Analyze and Optimize
For each problem you solve, analyze your solution and look for ways to optimize it. This will help you develop a deeper understanding of DP principles.
The AlgoCademy Approach to Learning Dynamic Programming
At AlgoCademy, we understand the challenges of learning dynamic programming. That’s why we’ve developed a comprehensive approach to make the learning process more accessible and enjoyable:
1. Interactive Tutorials
Our platform offers step-by-step, interactive tutorials that guide you through the process of solving DP problems. These tutorials break down complex concepts into digestible chunks, making it easier to understand and apply DP principles.
2. Visualization Tools
We provide visualization tools that help you see how DP algorithms work in real-time. This visual approach can be particularly helpful for understanding concepts like memoization and tabulation.
3. AI-Powered Assistance
Our AI-powered coding assistant can provide hints, explain concepts, and even help debug your code as you work through DP problems. This personalized support can significantly accelerate your learning process.
4. Curated Problem Sets
We’ve carefully curated a set of DP problems that progress from beginner to advanced levels. This structured approach ensures that you build a strong foundation before tackling more complex challenges.
5. Real-World Applications
To make DP more relatable, we include examples of how these algorithms are used in real-world scenarios. This helps bridge the gap between theory and practical application.
Practical Examples: Solving DP Problems
Let’s look at a couple of examples to illustrate how dynamic programming can be applied to solve problems efficiently.
Example 1: Fibonacci Sequence
The Fibonacci sequence is a classic example used to introduce DP. Let’s see how we can optimize the solution using memoization:
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
print(fibonacci(10)) # Output: 55
In this example, we use a dictionary to store previously calculated Fibonacci numbers. This memoization technique dramatically improves the efficiency of the algorithm from O(2^n) to O(n).
Example 2: Climbing Stairs
Consider the problem of climbing stairs where you can take either 1 or 2 steps at a time. How many distinct ways can you climb n stairs?
def climbStairs(n):
if n <= 1:
return 1
dp = [0] * (n + 1)
dp[0] = dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
print(climbStairs(5)) # Output: 8
This solution uses tabulation to build up the solution iteratively. The time complexity is O(n), and it efficiently solves the problem without recursion.
Common Misconceptions About Dynamic Programming
Let’s address some common misconceptions that might be holding you back from embracing dynamic programming:
1. “DP is only for geniuses”
This is far from the truth. While DP requires practice and patience, it’s a skill that anyone can learn with dedication and the right resources.
2. “DP is all about memorization”
While memorizing common patterns can be helpful, understanding the underlying principles is far more important. Focus on grasping the concepts rather than memorizing solutions.
3. “DP is not used in real-world applications”
Dynamic programming has numerous real-world applications, including:
- Shortest path algorithms in GPS systems
- Resource allocation in economics
- Bioinformatics for DNA sequence alignment
- Natural language processing for speech recognition
4. “Once you learn DP, you can solve any problem”
While DP is a powerful tool, it’s not a one-size-fits-all solution. It’s important to recognize when DP is appropriate and when other algorithms might be more suitable.
Tips for Mastering Dynamic Programming
Here are some additional tips to help you on your journey to mastering dynamic programming:
1. Develop a Problem-Solving Framework
When approaching a DP problem, follow these steps:
- Identify if the problem has optimal substructure and overlapping subproblems.
- Define the recurrence relation.
- Decide between top-down (memoization) or bottom-up (tabulation) approach.
- Implement the solution.
- Optimize for space and time complexity.
2. Learn to Recognize DP Opportunities
Look for problems that involve:
- Optimization (finding maximum or minimum values)
- Counting the number of ways to do something
- Problems with recursive solutions that have repeated subproblems
3. Practice State Transition
Understanding how to transition from one state to another is crucial in DP. Practice defining and manipulating state variables in your solutions.
4. Study Time and Space Complexity
Always analyze the time and space complexity of your DP solutions. Look for opportunities to optimize, such as using rolling arrays to reduce space complexity.
5. Implement Both Recursive and Iterative Solutions
For each problem, try implementing both the top-down (recursive with memoization) and bottom-up (iterative with tabulation) approaches. This will deepen your understanding of DP concepts.
The Road to DP Mastery: A Learning Path
To help you structure your learning journey, here’s a suggested path to DP mastery:
- Start with basic recursion problems
- Move on to simple DP problems (like Fibonacci and Climbing Stairs)
- Tackle 1D DP problems (e.g., Maximum Subarray Sum)
- Progress to 2D DP problems (e.g., Longest Common Subsequence)
- Explore more complex DP patterns (e.g., Knapsack problems)
- Study advanced DP techniques (e.g., Bitmask DP, Digit DP)
- Apply DP to real-world problems and optimization scenarios
Leveraging AlgoCademy for Your DP Journey
AlgoCademy offers a range of features to support your dynamic programming learning journey:
1. Structured Curriculum
Our DP course is designed to take you from beginner to advanced level, with carefully curated problems and explanations at each stage.
2. Interactive Coding Environment
Practice DP problems in our browser-based IDE, which supports multiple programming languages and provides instant feedback.
3. Solution Discussions
After solving a problem, engage with our community in solution discussions. Learn from others and share your own insights.
4. Performance Tracking
Monitor your progress with our performance tracking tools. Identify areas for improvement and celebrate your achievements.
5. Mock Interviews
Once you’ve built your DP skills, put them to the test with our mock interview feature, which simulates technical interviews from top tech companies.
Conclusion: Embracing the DP Challenge
Dynamic Programming may seem daunting at first, but with the right approach and resources, it’s a skill that’s well within your reach. By breaking down the complexity, focusing on core principles, and practicing regularly, you can master DP and add a powerful tool to your problem-solving arsenal.
Remember, every expert was once a beginner. The key is to start, persist, and enjoy the learning process. With platforms like AlgoCademy providing structured learning paths, interactive tools, and community support, you have everything you need to succeed in your DP journey.
So, take that first step. Dive into a DP problem today, and discover for yourself why learning dynamic programming is easier than you think. Your future self will thank you for the valuable skills you’re about to acquire.
Happy coding, and may your DP solutions always be optimal!