How to Think Like a Computer Scientist During a Coding Interview
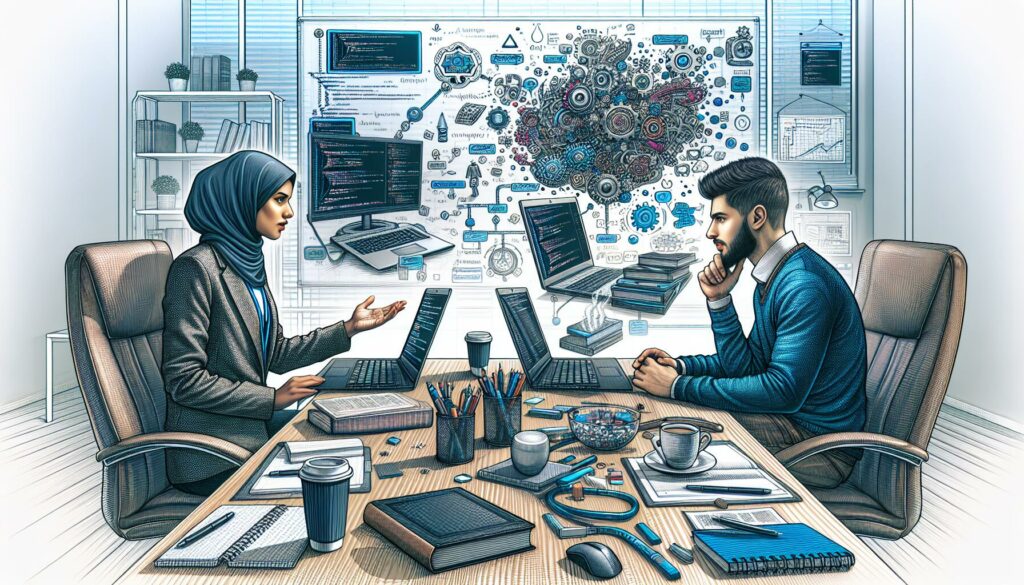
Coding interviews can be daunting, especially when you’re aiming for positions at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). However, the key to success lies not just in memorizing algorithms and data structures, but in developing the ability to think like a computer scientist. This mindset can help you approach problems systematically, communicate your thoughts effectively, and ultimately impress your interviewers. In this comprehensive guide, we’ll explore strategies to cultivate this thinking pattern and excel in your next coding interview.
1. Understand the Problem: Break It Down
The first step in thinking like a computer scientist is to thoroughly understand the problem at hand. This involves breaking down complex issues into smaller, manageable components.
Key Strategies:
- Read the problem statement carefully, multiple times if necessary.
- Identify the input and expected output.
- List any constraints or special conditions mentioned.
- Ask clarifying questions to ensure you have all the necessary information.
For example, if given a problem to find the longest palindromic substring in a string, you might break it down like this:
- Input: A string of characters
- Output: The longest substring that reads the same forward and backward
- Constraints: Case-sensitive? Empty string allowed? Maximum string length?
2. Visualize the Problem: Use Diagrams and Examples
Computer scientists often use visual aids to better understand and solve problems. This can be particularly helpful in interviews to communicate your thought process clearly.
Techniques:
- Draw diagrams to represent data structures or algorithms.
- Use flowcharts to illustrate the flow of your solution.
- Provide concrete examples to test your understanding.
For instance, when solving a graph problem, sketch out the nodes and edges. If working on a dynamic programming solution, create a table to show how you’re building up the solution.
3. Think Algorithmically: Develop a Step-by-Step Approach
Algorithmic thinking involves developing a clear, step-by-step approach to solving problems. This is crucial in demonstrating your problem-solving skills during an interview.
Steps to Follow:
- Start with a naive solution, even if it’s not optimal.
- Identify the core operations needed to solve the problem.
- Consider different algorithmic paradigms (e.g., divide and conquer, dynamic programming).
- Refine your approach iteratively, optimizing for time and space complexity.
Let’s consider an example of finding the two numbers in an array that sum up to a target value:
def find_two_sum(nums, target):
# Naive approach: O(n^2) time complexity
for i in range(len(nums)):
for j in range(i+1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return []
# Optimized approach: O(n) time complexity
def find_two_sum_optimized(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
By starting with a naive solution and then optimizing, you demonstrate your ability to think critically and improve upon initial ideas.
4. Analyze Complexity: Time and Space
A hallmark of computer science thinking is the ability to analyze the efficiency of algorithms in terms of time and space complexity. This is often expressed using Big O notation.
Key Points:
- Identify the worst-case, average-case, and best-case scenarios.
- Consider how the algorithm scales with input size.
- Be prepared to discuss trade-offs between time and space complexity.
For example, in the two sum problem above:
- Naive approach: O(n^2) time complexity, O(1) space complexity
- Optimized approach: O(n) time complexity, O(n) space complexity
Being able to articulate these differences shows a deep understanding of algorithmic efficiency.
5. Consider Edge Cases: Robust Problem Solving
Computer scientists are meticulous in considering all possible scenarios, including edge cases that might break a solution.
Strategies:
- Identify potential edge cases (e.g., empty inputs, extremely large inputs, negative numbers).
- Test your solution against these cases.
- Implement error handling and input validation where necessary.
For instance, in a string manipulation problem, consider:
- Empty string
- String with only one character
- String with all identical characters
- Very long string (to test for time limit exceeded errors)
6. Optimize and Refactor: Continuous Improvement
The ability to optimize and refactor code is a crucial skill for computer scientists. Even after arriving at a working solution, consider ways to improve it.
Approaches:
- Look for redundant operations that can be eliminated.
- Consider using more efficient data structures.
- Explore ways to reduce the space complexity without significantly impacting time complexity.
Here’s an example of optimizing a function that checks if a number is prime:
def is_prime(n):
# Initial implementation
if n < 2:
return False
for i in range(2, n):
if n % i == 0:
return False
return True
# Optimized version
def is_prime_optimized(n):
if n < 2:
return False
if n == 2:
return True
if n % 2 == 0:
return False
for i in range(3, int(n**0.5) + 1, 2):
if n % i == 0:
return False
return True
The optimized version reduces the number of iterations and checks, demonstrating an understanding of mathematical properties and algorithmic optimization.
7. Communicate Clearly: Explain Your Thought Process
Effective communication is a vital skill for computer scientists, especially during interviews. It’s not just about solving the problem, but also about articulating your thought process clearly.
Tips for Clear Communication:
- Think out loud as you work through the problem.
- Explain your reasoning for choosing specific approaches.
- Use precise terminology and avoid ambiguity.
- Be open to feedback and willing to incorporate suggestions.
Practice explaining your solutions to others or even to yourself in a mirror. This can help you identify areas where your explanations might be unclear or where you might be making assumptions that need clarification.
8. Use Appropriate Data Structures: The Right Tool for the Job
A key aspect of thinking like a computer scientist is choosing the most appropriate data structures for a given problem. This decision can significantly impact the efficiency and elegance of your solution.
Common Data Structures and Their Use Cases:
- Arrays: For sequential data with constant-time access by index.
- Linked Lists: For dynamic data with efficient insertions and deletions.
- Hash Tables: For fast lookups, insertions, and deletions based on keys.
- Trees: For hierarchical data and efficient searching (e.g., Binary Search Trees).
- Graphs: For representing complex relationships between entities.
- Stacks and Queues: For managing data with specific access patterns (LIFO/FIFO).
Consider a problem where you need to find the first non-repeating character in a string. While you could use nested loops to check each character against every other character, a more efficient approach would be to use a hash table:
def first_non_repeating_char(s):
char_count = {}
for char in s:
char_count[char] = char_count.get(char, 0) + 1
for char in s:
if char_count[char] == 1:
return char
return None
This solution demonstrates the use of a hash table to achieve O(n) time complexity, showcasing the ability to select and utilize appropriate data structures.
9. Apply Problem-Solving Patterns: Recognize Common Approaches
Experienced computer scientists often recognize patterns in problems that allow them to apply well-known solution strategies. Familiarizing yourself with these patterns can help you approach new problems more efficiently.
Common Problem-Solving Patterns:
- Two Pointers: Often used for array or string problems.
- Sliding Window: Useful for contiguous sequence problems.
- Divide and Conquer: Breaking down problems into smaller subproblems.
- Dynamic Programming: Solving complex problems by breaking them down into simpler subproblems.
- Backtracking: Used for problems where you need to find all (or some) solutions to a computational problem.
- Depth-First Search (DFS) and Breadth-First Search (BFS): For traversing or searching tree or graph data structures.
For example, consider the problem of finding the longest substring without repeating characters. This can be efficiently solved using the sliding window technique:
def longest_substring_without_repeats(s):
char_index = {}
max_length = start = 0
for i, char in enumerate(s):
if char in char_index and start <= char_index[char]:
start = char_index[char] + 1
else:
max_length = max(max_length, i - start + 1)
char_index[char] = i
return max_length
This solution demonstrates the application of the sliding window pattern to efficiently solve a string manipulation problem.
10. Test and Debug: Ensure Correctness
A crucial aspect of thinking like a computer scientist is the ability to test your code thoroughly and debug effectively when issues arise.
Testing and Debugging Strategies:
- Write test cases that cover various scenarios, including edge cases.
- Use print statements or a debugger to track variable values and program flow.
- Implement assertions to catch unexpected behavior.
- Break down complex functions into smaller, testable units.
Here’s an example of how you might approach testing a function that reverses a linked list:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_linked_list(head):
prev = None
current = head
while current:
next_temp = current.next
current.next = prev
prev = current
current = next_temp
return prev
# Test cases
def test_reverse_linked_list():
# Test case 1: Normal case
head = ListNode(1, ListNode(2, ListNode(3)))
reversed_head = reverse_linked_list(head)
assert reversed_head.val == 3
assert reversed_head.next.val == 2
assert reversed_head.next.next.val == 1
assert reversed_head.next.next.next == None
# Test case 2: Single node
single_node = ListNode(1)
reversed_single = reverse_linked_list(single_node)
assert reversed_single.val == 1
assert reversed_single.next == None
# Test case 3: Empty list
empty_list = None
reversed_empty = reverse_linked_list(empty_list)
assert reversed_empty == None
print("All test cases passed!")
test_reverse_linked_list()
This example shows how to create test cases that cover normal scenarios, edge cases, and potential pitfalls, demonstrating a thorough approach to ensuring code correctness.
Conclusion: Putting It All Together
Thinking like a computer scientist during a coding interview is about more than just writing code. It’s about approaching problems systematically, communicating clearly, and demonstrating a deep understanding of computer science principles. By breaking down problems, visualizing solutions, thinking algorithmically, analyzing complexity, considering edge cases, optimizing code, using appropriate data structures, recognizing patterns, and thoroughly testing your solutions, you can showcase your skills and stand out in technical interviews.
Remember, the goal is not just to solve the problem, but to demonstrate your problem-solving process and your ability to think critically about computational challenges. With practice and the right mindset, you can approach coding interviews with confidence and showcase your abilities as a computer scientist.
As you prepare for your next coding interview, consider using platforms like AlgoCademy to hone your skills. With interactive coding tutorials, AI-powered assistance, and a focus on algorithmic thinking and problem-solving, AlgoCademy can help you develop the mindset and skills needed to excel in technical interviews at top tech companies.
By internalizing these strategies and practicing regularly, you’ll be well-equipped to tackle any coding challenge that comes your way. Remember, becoming a great computer scientist is a journey of continuous learning and improvement. Embrace the process, stay curious, and keep pushing yourself to think more deeply about the problems you encounter. With dedication and the right approach, you’ll be well on your way to mastering the art of thinking like a computer scientist during coding interviews and beyond.