Coding Interview Prep: Learning to Thrive in Ambiguity (When You Don’t Know the Answer)
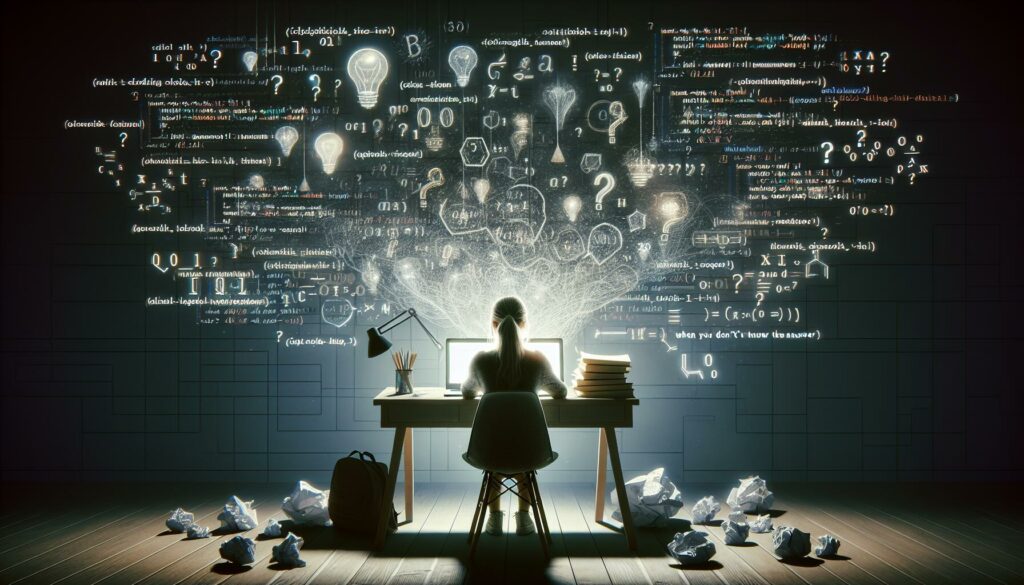
In the world of coding interviews, especially those conducted by major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), candidates often find themselves facing challenging questions that push the boundaries of their knowledge and problem-solving abilities. While thorough preparation is crucial, it’s equally important to develop strategies for handling situations where you don’t immediately know the answer. This article will explore techniques to help you navigate uncertainty and thrive in ambiguous coding interview scenarios.
1. Embrace the Unknown: A Mindset Shift
The first step in thriving during ambiguous coding interview situations is to adopt the right mindset. Remember that interviewers are not just testing your knowledge, but also your ability to think critically and approach unfamiliar problems.
- Stay calm and composed: Take a deep breath and remind yourself that it’s okay not to have an immediate answer.
- View challenges as opportunities: Instead of feeling discouraged, see these moments as chances to showcase your problem-solving skills.
- Be honest: If you’re unsure about something, it’s better to admit it than to bluff your way through. Interviewers appreciate honesty and the ability to acknowledge limitations.
2. Break Down the Problem
When faced with a complex or unfamiliar problem, breaking it down into smaller, more manageable parts can help you gain clarity and find a starting point.
- Identify the core components of the problem.
- Look for familiar elements or patterns that you can relate to known concepts.
- Create a high-level outline of potential steps or approaches.
For example, if asked to implement a complex data structure you’re not familiar with, start by considering its basic operations and how they might be implemented using simpler structures you know well.
3. Think Aloud and Collaborate
Interviewers are interested in your thought process, not just the final answer. Verbalizing your thoughts can help you organize your ideas and allow the interviewer to provide guidance if needed.
- Explain your understanding: Restate the problem in your own words to ensure you’ve grasped it correctly.
- Share your approach: Discuss potential solutions, even if you’re not sure they’re correct.
- Ask clarifying questions: Don’t hesitate to seek additional information or clarification about the problem.
Remember, the interview is a collaborative process. Engaging with the interviewer can lead to valuable insights and demonstrate your communication skills.
4. Start with a Brute Force Solution
When you’re unsure how to approach a problem optimally, begin with a simple, brute force solution. This strategy serves multiple purposes:
- It shows that you can at least solve the problem, even if not efficiently.
- It provides a starting point for optimization discussions.
- It helps you understand the problem better as you work through it.
Here’s an example of how you might approach a brute force solution for finding the two numbers in an array that sum to a target value:
def find_two_sum_brute_force(nums, target):
for i in range(len(nums)):
for j in range(i + 1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return [] # No solution found
# Example usage
nums = [2, 7, 11, 15]
target = 9
result = find_two_sum_brute_force(nums, target)
print(f"Indices of the two numbers: {result}")
After implementing this solution, you can discuss its time complexity (O(n^2)) and potential optimizations, such as using a hash table to improve efficiency.
5. Draw from Related Concepts
Even if you don’t know the exact solution, you can often apply principles from related problems or data structures you’re familiar with. This demonstrates your ability to make connections and adapt your knowledge.
For instance, if asked about implementing a Trie (prefix tree) and you’re not entirely familiar with it, you could start by relating it to concepts you know:
- It’s a tree-like structure, similar to a binary tree.
- Each node represents a character in a string.
- It’s efficient for prefix matching, similar to how a binary search tree is efficient for range queries.
From there, you can begin to sketch out a basic implementation:
class TrieNode:
def __init__(self):
self.children = {}
self.is_end_of_word = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end_of_word = True
def search(self, word):
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end_of_word
# Example usage
trie = Trie()
trie.insert("apple")
print(trie.search("apple")) # True
print(trie.search("app")) # False
6. Use Pseudocode and High-Level Descriptions
When you’re unsure about the exact implementation details, using pseudocode or high-level descriptions can help you communicate your ideas effectively. This approach allows you to outline your thought process without getting bogged down in syntax details.
For example, if asked to implement a graph traversal algorithm you’re not entirely familiar with, you might start with:
function graphTraversal(graph, startNode):
initialize a queue or stack (depending on BFS or DFS)
add startNode to the queue/stack
initialize a set to keep track of visited nodes
while queue/stack is not empty:
currentNode = remove node from queue/stack
if currentNode is not in visited:
process currentNode
add currentNode to visited
for each neighbor of currentNode:
if neighbor is not in visited:
add neighbor to queue/stack
return visited nodes or other required information
This pseudocode demonstrates your understanding of key concepts like graph representation, traversal methods, and handling visited nodes, even if you’re not sure about the specific implementation details.
7. Leverage Your Problem-Solving Framework
Having a structured approach to problem-solving can be invaluable when facing unfamiliar challenges. A common framework used in coding interviews is the UMPIRE method:
- Understand: Clarify the problem and requirements.
- Match: Identify similar problems or patterns you’ve encountered before.
- Plan: Outline your approach before coding.
- Implement: Write the code, starting with a basic solution if needed.
- Review: Check your solution for correctness and efficiency.
- Evaluate: Discuss potential improvements or alternative approaches.
By systematically working through these steps, you can approach even unfamiliar problems with confidence and structure.
8. Practice with Ambiguous Problems
To become more comfortable with ambiguity, incorporate open-ended or vague problems into your practice routine. Platforms like AlgoCademy often include such challenges to help you develop this skill. Here are some ways to practice:
- System design questions: These often have no single correct answer and require you to make assumptions and trade-offs.
- Optimization problems: Start with a basic solution and gradually improve it, discussing the pros and cons of each approach.
- Real-world scenarios: Practice designing solutions for complex, real-world problems that may not have clear-cut answers.
For example, consider this open-ended problem:
“Design a system to manage traffic lights in a smart city.”
This problem requires you to make assumptions, consider various factors, and propose a flexible solution. You might start by:
- Defining the scope (e.g., number of intersections, types of sensors)
- Outlining the system architecture (e.g., central control system, local controllers)
- Discussing data flow and decision-making processes
- Considering scalability and fault tolerance
Practicing with such problems helps you develop the skills to navigate ambiguity effectively.
9. Learn to Estimate and Make Reasonable Assumptions
In many coding interviews, especially for system design questions, you’ll need to make estimates and assumptions. Developing this skill can help you navigate uncertain scenarios more confidently.
- Use round numbers: When estimating, use powers of 10 for easier calculations.
- Provide ranges: Instead of exact figures, give reasonable upper and lower bounds.
- Explain your reasoning: Always clarify the basis for your estimates or assumptions.
For example, if asked to estimate the storage requirements for a social media platform:
Assumptions:
- 1 million active users
- Average 100 posts per user per year
- Average post size: 1 KB (text) + 200 KB (image) = ~200 KB
Calculation:
1,000,000 users * 100 posts/year * 200 KB/post = 20 TB/year
Estimate:
We might need 20-30 TB of storage per year, accounting for metadata and potential growth.
This approach shows your ability to break down complex problems and make reasonable estimates, even without exact information.
10. Reflect and Learn from Each Experience
After each interview or practice session where you encounter ambiguity, take time to reflect on your performance and identify areas for improvement:
- Review your approach: Consider what worked well and what could be improved in your problem-solving process.
- Research unfamiliar concepts: Look up any topics or algorithms that came up during the interview that you weren’t familiar with.
- Practice similar problems: Find and solve related problems to reinforce your learning and expand your problem-solving toolkit.
Remember, every challenging interview question is an opportunity to learn and grow as a developer.
Conclusion
Thriving in ambiguity during coding interviews is a skill that can be developed with practice and the right mindset. By embracing uncertainty, breaking down problems, thinking aloud, starting with brute force solutions, drawing from related concepts, using pseudocode, leveraging problem-solving frameworks, practicing with open-ended questions, making reasonable estimates, and reflecting on each experience, you can navigate even the most challenging interview scenarios with confidence.
Remember that interviewers are often more interested in your problem-solving approach and ability to handle uncertainty than in your immediate knowledge of every possible algorithm or data structure. By demonstrating your ability to think critically, communicate effectively, and approach unfamiliar problems systematically, you’ll showcase the skills that top tech companies value most.
As you continue your interview preparation journey, platforms like AlgoCademy can provide valuable resources, practice problems, and AI-powered assistance to help you develop these crucial skills. Embrace the challenges, learn from each experience, and approach your coding interviews with confidence, knowing that you have the tools to thrive even when faced with ambiguity.