The Best Algorithms to Learn for a Coding Interview â and Why They Keep Repeating
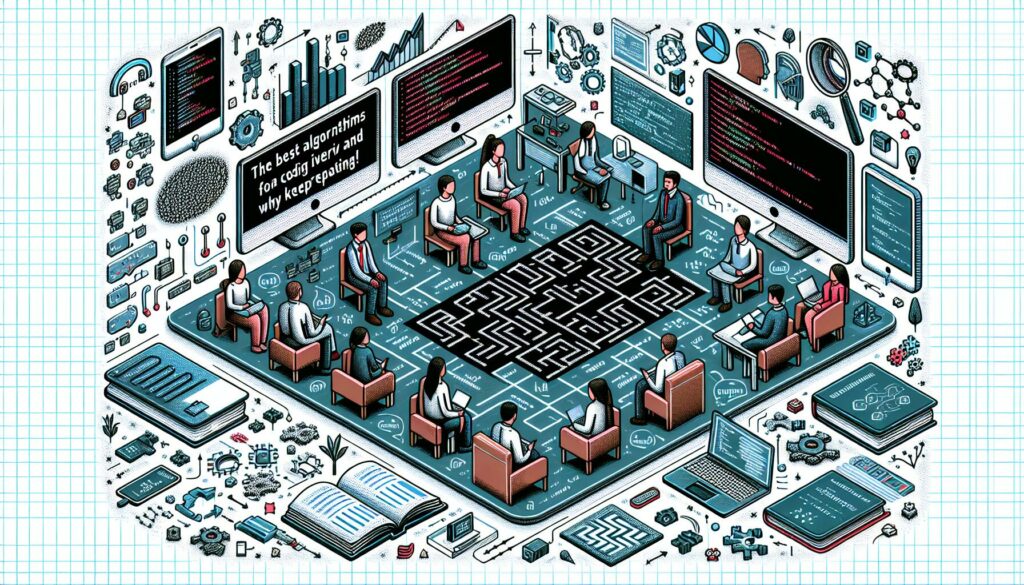
When preparing for a coding interview, especially with top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), mastering key algorithms is crucial. These algorithms not only form the foundation of computer science but also frequently appear in technical interviews. In this comprehensive guide, we’ll explore the best algorithms to learn, why they’re important, and why they keep showing up in interviews time and time again.
Why Algorithms Matter in Coding Interviews
Before diving into specific algorithms, it’s essential to understand why they play such a significant role in the interview process:
- Problem-Solving Skills: Algorithms demonstrate your ability to break down complex problems into manageable steps.
- Efficiency: They showcase your understanding of time and space complexity, crucial for writing performant code.
- Fundamental Knowledge: Algorithms form the building blocks of computer science and software development.
- Adaptability: Mastering core algorithms helps you tackle unfamiliar problems by applying known patterns.
The Top Algorithms to Learn for Coding Interviews
1. Binary Search
Binary search is a fundamental algorithm used to efficiently search a sorted array by repeatedly dividing the search interval in half.
Why it’s important:
- Demonstrates understanding of divide-and-conquer techniques
- Efficient for large datasets with O(log n) time complexity
- Frequently used in various applications and as a building block for more complex algorithms
Example implementation in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
2. Depth-First Search (DFS) and Breadth-First Search (BFS)
DFS and BFS are graph traversal algorithms used to explore nodes in a graph or tree data structure.
Why they’re important:
- Essential for solving problems related to trees, graphs, and networks
- Demonstrate understanding of recursive (DFS) and iterative (BFS) approaches
- Applicable in various real-world scenarios, such as social network analysis and web crawling
Example implementation of DFS in Python:
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start) # Process the node
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
3. Quick Sort
Quick Sort is an efficient, in-place sorting algorithm that uses a divide-and-conquer strategy.
Why it’s important:
- Demonstrates understanding of divide-and-conquer algorithms and partitioning
- Efficient average-case time complexity of O(n log n)
- Widely used in practice and forms the basis for many optimized sorting implementations
Example implementation in Python:
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
4. Dynamic Programming
Dynamic Programming is not a specific algorithm but a technique for solving complex problems by breaking them down into simpler subproblems.
Why it’s important:
- Solves optimization problems efficiently
- Demonstrates ability to identify overlapping subproblems and optimal substructure
- Applicable in various fields, including economics, bioinformatics, and computer science
Example: Fibonacci sequence using dynamic programming
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
5. Dijkstra’s Algorithm
Dijkstra’s algorithm is used to find the shortest path between nodes in a graph with non-negative edge weights.
Why it’s important:
- Fundamental for solving shortest path problems in weighted graphs
- Demonstrates understanding of greedy algorithms and graph theory
- Widely used in network routing protocols and GPS navigation systems
Example implementation in Python (simplified):
import heapq
def dijkstra(graph, start):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return distances
6. Binary Tree Traversals
Binary tree traversals (in-order, pre-order, and post-order) are fundamental algorithms for processing tree-based data structures.
Why they’re important:
- Essential for understanding and manipulating tree structures
- Demonstrate recursive thinking and ability to work with hierarchical data
- Form the basis for more complex tree-based algorithms
Example implementation of in-order traversal in Python:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
result = []
if root:
result.extend(inorder_traversal(root.left))
result.append(root.val)
result.extend(inorder_traversal(root.right))
return result
7. Sliding Window Technique
The sliding window technique is used to perform operations on a specific window of elements in an array or string.
Why it’s important:
- Efficient for solving substring or subarray problems
- Demonstrates ability to optimize brute force approaches
- Applicable in various scenarios, such as finding longest substring with certain properties
Example: Finding the maximum sum subarray of size k
def max_sum_subarray(arr, k):
n = len(arr)
if n < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(n - k):
window_sum = window_sum - arr[i] + arr[i + k]
max_sum = max(max_sum, window_sum)
return max_sum
Why These Algorithms Keep Repeating in Interviews
Now that we’ve explored some of the best algorithms to learn for coding interviews, let’s discuss why these particular algorithms keep appearing in technical interviews:
1. Foundational Knowledge
These algorithms represent core computer science concepts. Mastery of these algorithms demonstrates a solid understanding of fundamental principles, which is crucial for any software engineer.
2. Problem-Solving Patterns
Many of these algorithms embody common problem-solving patterns. Once you understand these patterns, you can apply them to a wide range of problems, even those you haven’t encountered before.
3. Efficiency and Optimization
Algorithms like Quick Sort and Binary Search showcase an understanding of efficiency and the importance of optimizing solutions. This is critical in real-world software development where performance matters.
4. Versatility
These algorithms are versatile and can be applied to various domains and problem types. For example, graph algorithms like DFS and BFS can be used in network analysis, web crawling, and social network applications.
5. Industry Relevance
Many of these algorithms have direct applications in industry. For instance, Dijkstra’s algorithm is used in network routing, while dynamic programming is applied in resource allocation and optimization problems.
6. Cognitive Assessment
Implementing these algorithms requires a combination of logical thinking, code organization, and attention to detail. These skills are highly valued in software development.
7. Historical Significance
Some of these algorithms, like Quick Sort and Binary Search, have stood the test of time. Their continued relevance demonstrates their importance in computer science.
How to Prepare for Algorithm-Based Interview Questions
Now that you understand the importance of these algorithms, here are some tips to help you prepare for algorithm-based interview questions:
1. Practice Regularly
Consistent practice is key. Solve algorithm problems regularly on platforms like LeetCode, HackerRank, or AlgoCademy. Aim to solve at least one problem a day.
2. Understand the Fundamentals
Don’t just memorize solutions. Understand the underlying principles and why certain approaches work better than others.
3. Analyze Time and Space Complexity
For each solution you develop, analyze its time and space complexity. This will help you understand the efficiency of your algorithms and how they might scale with larger inputs.
4. Learn to Communicate Your Thought Process
Practice explaining your approach out loud. In an interview, your thought process is often as important as the final solution.
5. Study Multiple Approaches
For each problem, try to come up with multiple solutions. This will help you understand trade-offs between different approaches and demonstrate flexibility in your thinking.
6. Implement from Scratch
While it’s important to understand library functions, practice implementing algorithms from scratch. This deepens your understanding and prepares you for interviews where you might need to write code without relying on built-in functions.
7. Review and Reflect
After solving a problem, take time to review your solution and reflect on what you learned. Look at other people’s solutions to gain new perspectives.
Conclusion
Mastering these key algorithms is essential for success in coding interviews, especially when aiming for positions at top tech companies. They provide a solid foundation in computer science principles, demonstrate problem-solving skills, and showcase your ability to write efficient code.
Remember, the goal isn’t just to memorize these algorithms, but to understand the underlying concepts and problem-solving patterns they represent. With consistent practice and a deep understanding of these fundamental algorithms, you’ll be well-prepared to tackle even the most challenging coding interview questions.
As you continue your journey in software development, these algorithms will serve as valuable tools in your problem-solving toolkit, helping you design efficient solutions to complex problems throughout your career. Happy coding, and best of luck in your interviews!