Why Whiteboard Coding Is Outdated and How to Prepare for Real-World Problems Instead
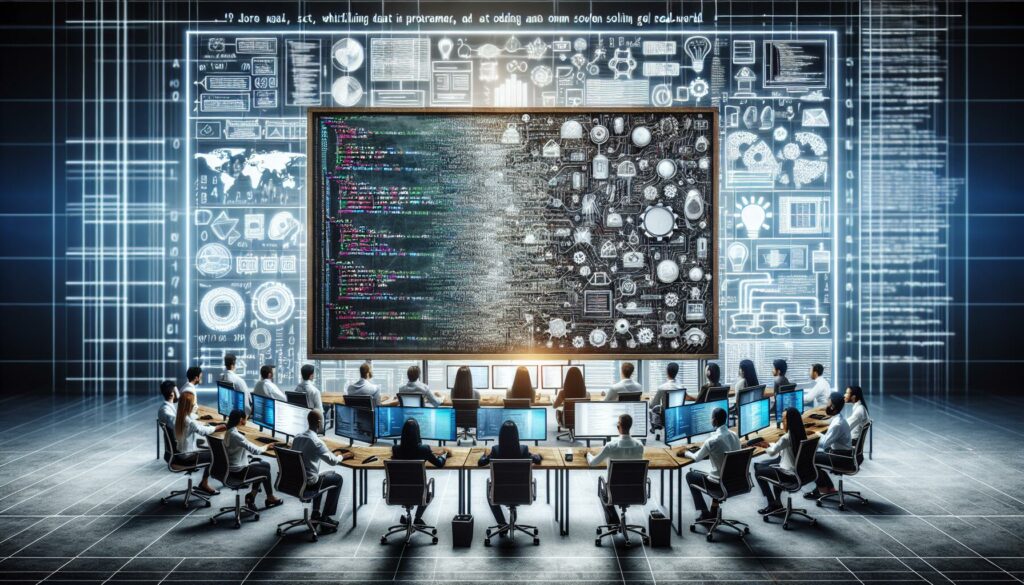
In the ever-evolving landscape of software development and tech hiring, the traditional whiteboard coding interview has long been a staple. However, as the industry progresses and the nature of software engineering work changes, many are questioning whether this approach is still relevant or effective. This article delves into why whiteboard coding is becoming outdated and explores more practical alternatives that better prepare candidates for real-world programming challenges.
The Rise and Fall of Whiteboard Coding
Whiteboard coding interviews gained popularity in the early days of tech hiring as a way to assess a candidate’s problem-solving skills and ability to think on their feet. The process typically involves a candidate solving algorithmic problems or writing code on a whiteboard while explaining their thought process to the interviewer.
Why It Became Popular
- Simplicity: It required minimal setup – just a whiteboard and markers.
- Focus on fundamentals: It emphasized core computer science concepts and algorithmic thinking.
- Stress testing: It allowed interviewers to observe how candidates perform under pressure.
The Problems with Whiteboard Coding
Despite its widespread use, whiteboard coding has several significant drawbacks:
- Artificial environment: Writing code on a whiteboard is far removed from the actual day-to-day work of a software developer.
- Limited scope: It often focuses on algorithmic puzzles that may not reflect the skills needed for the job.
- High stress, low relevance: The high-pressure situation can cause even skilled developers to underperform.
- Bias towards recent graduates: Those fresh out of computer science programs may perform better due to recent academic training.
- Lack of real tools: Developers rely on IDEs, documentation, and other tools that are absent in whiteboard scenarios.
The Shift Towards Real-World Problem Solving
As the tech industry matures, there’s a growing recognition that interview processes should more closely mirror the actual work environment and challenges that developers face. This shift is driven by several factors:
1. Emphasis on Practical Skills
Modern software development requires a diverse set of skills beyond algorithmic problem-solving. These include:
- Understanding and working with existing codebases
- Debugging and troubleshooting
- Writing maintainable and scalable code
- Collaborating with team members
- Using version control systems
- Working with APIs and third-party libraries
2. Recognition of Diverse Problem-Solving Approaches
Not all developers think or work in the same way. Real-world coding allows for different approaches and styles, which can lead to innovative solutions.
3. Importance of Tools and Resources
Modern development relies heavily on tools like IDEs, debuggers, and online resources. Evaluating a candidate’s ability to effectively use these tools is crucial.
4. Focus on Project-Based Assessment
Many companies are moving towards project-based interviews or take-home assignments that better reflect the actual work environment.
Alternatives to Whiteboard Coding
As the industry moves away from whiteboard coding, several alternative interview methods have gained traction:
1. Pair Programming Sessions
In pair programming interviews, the candidate works alongside an interviewer to solve a problem or implement a feature. This approach offers several benefits:
- Mimics real collaborative environments
- Allows interviewers to observe problem-solving in action
- Provides insight into communication skills and teamwork
2. Take-Home Projects
Take-home projects involve giving candidates a small project to complete on their own time. This method:
- Allows candidates to work in a comfortable environment
- Provides a more realistic assessment of coding skills
- Evaluates time management and project planning abilities
3. Code Review Exercises
In this approach, candidates review existing code and provide feedback. This assesses:
- Ability to understand and work with existing codebases
- Code quality assessment skills
- Communication of technical concepts
4. System Design Discussions
For more senior roles, system design discussions focus on high-level architecture and decision-making. This evaluates:
- Understanding of large-scale systems
- Ability to make trade-offs and justify decisions
- Knowledge of best practices and design patterns
5. Live Coding with Real Tools
This approach involves coding in a real development environment, complete with an IDE and access to documentation. Benefits include:
- A more realistic coding experience
- Assessment of tool proficiency
- Observation of actual coding practices
How to Prepare for Real-World Coding Interviews
As the interview landscape changes, so too should your preparation strategies. Here are some tips to help you prepare for modern coding interviews:
1. Build Projects
Nothing beats hands-on experience. Build personal projects that:
- Demonstrate your ability to create end-to-end solutions
- Showcase your proficiency with relevant technologies
- Highlight your problem-solving skills in real-world scenarios
2. Practice Collaborative Coding
Improve your pair programming skills by:
- Participating in open-source projects
- Joining coding communities and hackathons
- Practicing with friends or mentors
3. Enhance Your Debugging Skills
Debugging is a crucial skill in real-world development. Improve by:
- Working on complex projects with multiple components
- Practicing with deliberate bug-hunting exercises
- Learning to use debugging tools effectively
4. Study System Design
For more advanced roles, understanding system design is crucial:
- Read books and articles on system architecture
- Practice designing scalable systems
- Understand trade-offs in different architectural decisions
5. Improve Code Quality and Best Practices
Focus on writing clean, maintainable code:
- Study design patterns and when to apply them
- Practice refactoring complex codebases
- Learn and apply SOLID principles
6. Familiarize Yourself with Common Tools
Be proficient with tools commonly used in development:
- Version control systems (e.g., Git)
- IDEs and their advanced features
- Debugging tools and techniques
Real-World Coding Examples
To illustrate the difference between whiteboard coding and real-world problems, let’s look at a couple of examples:
Whiteboard Coding Example: Reverse a String
A typical whiteboard coding question might ask you to reverse a string without using built-in functions. Here’s how you might solve it:
function reverseString(str) {
let reversed = '';
for (let i = str.length - 1; i >= 0; i--) {
reversed += str[i];
}
return reversed;
}
console.log(reverseString('hello')); // Outputs: 'olleh'
While this demonstrates basic string manipulation and loop control, it’s far from a real-world scenario.
Real-World Coding Example: Implement a Caching Mechanism
In contrast, a real-world problem might involve implementing a caching mechanism to improve the performance of a web application. Here’s a simplified example using Node.js and Redis:
const express = require('express');
const redis = require('redis');
const util = require('util');
const app = express();
const client = redis.createClient();
// Promisify Redis get and set operations
const getAsync = util.promisify(client.get).bind(client);
const setAsync = util.promisify(client.set).bind(client);
// Simulated expensive operation
async function fetchDataFromDatabase(key) {
// Imagine this is a complex database query
await new Promise(resolve => setTimeout(resolve, 2000));
return `Data for ${key}`;
}
app.get('/data/:key', async (req, res) => {
const { key } = req.params;
try {
// Try to get data from cache
let data = await getAsync(key);
if (data === null) {
// If not in cache, fetch from database
console.log('Cache miss. Fetching from database...');
data = await fetchDataFromDatabase(key);
// Store in cache for future requests
await setAsync(key, data, 'EX', 60); // Expire after 60 seconds
} else {
console.log('Cache hit!');
}
res.json({ data });
} catch (error) {
res.status(500).json({ error: 'An error occurred' });
}
});
app.listen(3000, () => console.log('Server running on port 3000'));
This example demonstrates several real-world concepts:
- Working with external libraries (Express, Redis)
- Implementing caching to improve performance
- Handling asynchronous operations
- Error handling and appropriate HTTP responses
- Basic API design
This type of problem better reflects the challenges a developer might face in their day-to-day work and allows candidates to showcase a broader range of skills.
The Role of Platforms Like AlgoCademy
As the industry shifts towards more practical and real-world focused interviews, platforms like AlgoCademy play a crucial role in helping developers prepare. Here’s how AlgoCademy and similar platforms are adapting to this change:
1. Diverse Problem Sets
While still offering algorithmic challenges, these platforms are increasingly incorporating problems that mirror real-world scenarios, such as:
- API design and implementation
- Database query optimization
- Refactoring exercises
- System design challenges
2. Interactive Coding Environments
Instead of simple text editors, these platforms often provide fully-featured IDEs that closely resemble real development environments, including:
- Syntax highlighting and auto-completion
- Debugging tools
- Access to documentation and external resources
3. Project-Based Learning
Recognizing the importance of building complete applications, platforms like AlgoCademy are introducing project-based learning modules that guide users through creating full-stack applications, covering aspects like:
- Front-end and back-end development
- Database design and implementation
- API integration
- Deployment and DevOps basics
4. Collaborative Features
To prepare users for pair programming interviews and collaborative work environments, these platforms are incorporating features such as:
- Peer code review systems
- Virtual pair programming sessions
- Team-based coding challenges
5. AI-Powered Assistance
Leveraging AI technology, platforms like AlgoCademy can provide:
- Personalized learning paths based on individual strengths and weaknesses
- Intelligent code analysis and feedback
- Simulated code review experiences
6. Industry-Aligned Curriculum
By constantly updating their content based on industry trends and feedback from tech companies, these platforms ensure that their curriculum remains relevant to current hiring practices, focusing on:
- In-demand technologies and frameworks
- Best practices in software development
- Emerging areas like cloud computing and machine learning
Conclusion
The shift away from whiteboard coding towards more practical, real-world problem-solving in tech interviews reflects the evolving nature of software development. This change benefits both companies and candidates by providing a more accurate assessment of the skills needed for success in modern development roles.
For aspiring developers and those looking to advance their careers, this shift presents both challenges and opportunities. While it may require adjusting your preparation strategies, it also allows you to showcase a broader range of skills and demonstrate your ability to tackle real-world problems.
Platforms like AlgoCademy are evolving to meet these new demands, offering resources and tools that align closely with industry needs. By leveraging these platforms and focusing on building practical skills through projects and collaborative coding, you can better prepare yourself for the realities of modern software development and increase your chances of success in today’s tech interviews.
Remember, the goal of interview preparation is not just to land a job, but to develop skills that will serve you throughout your career. Embrace this shift towards real-world problem-solving as an opportunity to become a more well-rounded and effective developer.