Coding Interviews as Storytelling: How to Turn Your Problem-Solving Process into a Narrative
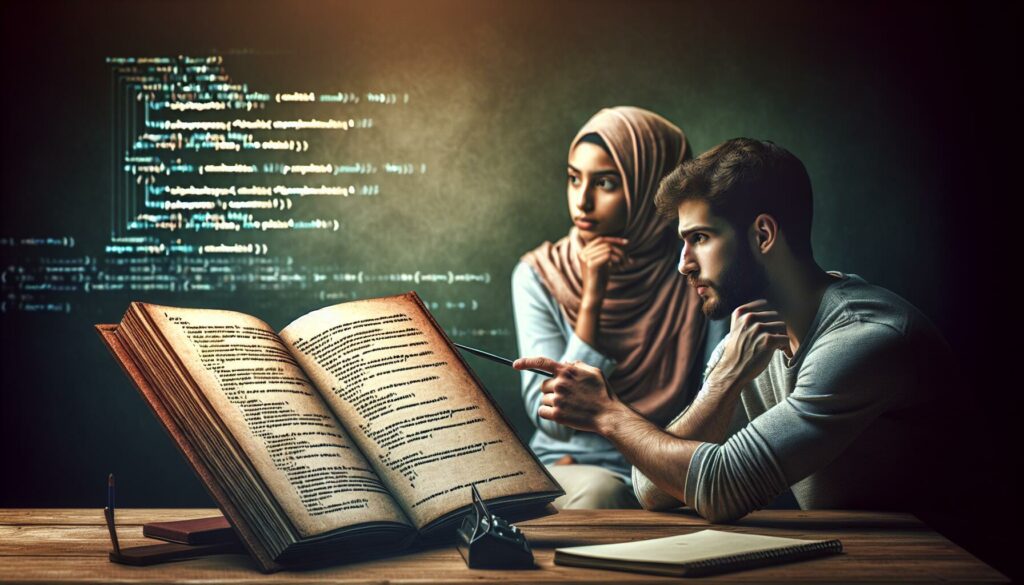
When it comes to coding interviews, especially those at prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), many candidates focus solely on solving the problem at hand. While arriving at the correct solution is crucial, how you communicate your thought process can be equally important. This is where the art of storytelling comes into play. By framing your problem-solving approach as a narrative, you can not only showcase your technical skills but also demonstrate your ability to think critically, communicate effectively, and collaborate with others. In this comprehensive guide, we’ll explore how to transform your coding interview into a compelling story that will captivate your interviewers and set you apart from other candidates.
Why Storytelling Matters in Coding Interviews
Before we dive into the specifics of crafting your narrative, let’s understand why storytelling is so important in the context of coding interviews:
- Engagement: A well-told story keeps your interviewer engaged and interested in your thought process.
- Clarity: Narratives help organize your thoughts and make your approach more coherent and easy to follow.
- Memorability: Interviewers are more likely to remember candidates who present their solutions as interesting stories.
- Demonstration of Soft Skills: Storytelling showcases your communication skills, an essential quality for any developer working in a team.
- Insight into Your Problem-Solving Style: A narrative approach gives interviewers a deeper understanding of how you tackle challenges.
The Elements of a Coding Interview Story
Just like any good story, your coding interview narrative should have a clear structure. Here are the key elements to include:
1. Setting the Scene
Begin by clearly stating the problem and any constraints or assumptions. This is your opportunity to show that you’ve understood the question and are ready to tackle it.
Example:
Interviewer: "Given an array of integers, find two numbers such that they add up to a specific target number."
You: "Alright, so we're dealing with an array of integers and a target sum. Before we dive in, let me make sure I understand the problem correctly. We need to find a pair of numbers in the array that add up to the given target, correct? And I assume we're looking for the indices of these numbers, not the numbers themselves?"
2. Introducing the Characters (Data Structures and Algorithms)
Discuss the potential approaches and data structures you might use. This is where you can showcase your knowledge of various algorithms and their trade-offs.
Example:
You: "For this problem, a few approaches come to mind. We could use a brute force method with nested loops, which would be simple but potentially inefficient. Alternatively, we could use a hash table to optimize our search. Let's explore these options and see which one fits best for our scenario."
3. The Plot (Your Approach)
Explain your chosen approach step-by-step. This is the meat of your story, where you detail how you’re going to solve the problem.
Example:
You: "I think the hash table approach would be most efficient here. We'll iterate through the array once, and for each element, we'll check if its complement (target - current element) exists in our hash table. If it does, we've found our pair. If not, we'll add the current element to the hash table and move on. This way, we're building our solution as we go, which is both time and space efficient."
4. The Climax (Implementation)
This is where you actually code your solution. As you write, explain each step, just as a narrator would describe the action in a story.
Example:
You: "Let's implement this solution in Python. We'll start by defining our function:"
def two_sum(nums, target):
seen = {} # Our hash table to store numbers we've seen
for i, num in enumerate(nums):
complement = target - num
if complement in seen:
return [seen[complement], i] # Found the pair!
seen[num] = i # Add current number to hash table
return [] # No solution found
"As we iterate through the array, we're constantly checking if we've seen the complement before. If we have, we've found our pair and can return their indices. If not, we add the current number to our hash table and continue. This gives us a time complexity of O(n) and a space complexity of O(n) in the worst case."
5. The Resolution (Testing and Edge Cases)
Discuss how you would test your solution and handle any edge cases. This shows your attention to detail and your ability to think critically about your own code.
Example:
You: "Now that we have our implementation, let's consider some test cases:
# Test case 1: Normal case
print(two_sum([2, 7, 11, 15], 9)) # Should return [0, 1]
# Test case 2: No solution
print(two_sum([2, 7, 11, 15], 30)) # Should return []
# Test case 3: Duplicate numbers
print(two_sum([3, 3], 6)) # Should return [0, 1]
We should also consider edge cases like an empty array or an array with only one element. In these cases, our function would correctly return an empty list, as there's no valid solution."
6. The Moral of the Story (Time and Space Complexity Analysis)
Conclude by analyzing the time and space complexity of your solution. This demonstrates your understanding of algorithmic efficiency.
Example:
You: "To wrap up, let's analyze the time and space complexity of our solution. We're iterating through the array once, which gives us a time complexity of O(n). For each element, we're performing constant time operations (hash table lookups and insertions), so this doesn't increase our overall time complexity.
In terms of space, in the worst case, we might need to store almost all the elements in our hash table before finding a solution, giving us a space complexity of O(n).
This solution offers a good balance between time and space efficiency, especially when compared to the brute force approach which would have a time complexity of O(n^2) and a space complexity of O(1)."
Tips for Effective Storytelling in Coding Interviews
Now that we’ve covered the structure of your coding interview story, let’s look at some tips to make your narrative more engaging and effective:
1. Use Analogies
Analogies can help make complex concepts more relatable and easier to understand. For example, you might compare a hash table to a library catalog, where each book (element) has a unique call number (hash) for quick lookup.
2. Think Out Loud
Don’t just silently write code. Verbalize your thought process as you go. This gives the interviewer insight into how you approach problems and allows them to provide hints if you’re heading in the wrong direction.
3. Use Visual Aids
If you’re in a whiteboard interview, don’t hesitate to draw diagrams or flowcharts to illustrate your approach. This can make your explanation clearer and show your ability to communicate complex ideas visually.
4. Maintain a Logical Flow
Ensure your explanation follows a logical progression. Start with the problem statement, move on to potential approaches, explain your chosen method, implement the solution, and conclude with testing and analysis.
5. Be Open to Feedback
If the interviewer suggests an alternative approach or points out a flaw in your reasoning, be receptive. Show that you can incorporate feedback and adjust your approach accordingly.
6. Practice Active Listening
Pay close attention to the interviewer’s questions and comments. They may provide valuable hints or ask you to consider certain aspects of the problem that you might have overlooked.
7. Use Transitions
Use transitional phrases to move smoothly between different parts of your explanation. For example, “Now that we’ve identified our approach, let’s move on to the implementation” or “Having implemented our solution, let’s consider some test cases.”
8. Show Enthusiasm
Demonstrate your passion for problem-solving. Use phrases like “That’s an interesting challenge” or “I’m excited to tackle this problem” to show your genuine interest in the task at hand.
Common Pitfalls to Avoid
While crafting your coding interview narrative, be aware of these common mistakes:
1. Jumping to Implementation Too Quickly
Resist the urge to start coding immediately. Take the time to understand the problem, consider different approaches, and explain your thought process before diving into the implementation.
2. Ignoring Time and Space Complexity
Always discuss the efficiency of your solution. Even if you don’t immediately come up with the most optimal approach, showing that you’re thinking about complexity demonstrates your understanding of algorithmic efficiency.
3. Neglecting Edge Cases
Don’t forget to consider and discuss edge cases. This shows attention to detail and thoroughness in your problem-solving approach.
4. Being Too Verbose or Too Quiet
Strike a balance in your communication. Provide enough detail to clearly explain your approach, but avoid rambling or getting sidetracked.
5. Giving Up Too Easily
If you’re stuck, don’t give up. Instead, verbalize your thought process and the roadblocks you’re encountering. The interviewer may provide hints or ask guiding questions to help you move forward.
Practice Makes Perfect
Like any skill, turning your problem-solving process into a compelling narrative takes practice. Here are some ways to hone this skill:
1. Mock Interviews
Conduct mock interviews with friends, mentors, or through online platforms. Practice explaining your thought process out loud as you solve problems.
2. Solve Problems on Coding Platforms
Use platforms like LeetCode, HackerRank, or AlgoCademy to practice solving algorithmic problems. After solving each problem, write out or record yourself explaining your solution as if you were in an interview.
3. Teach Others
Try explaining coding concepts or solutions to others, even if they’re not programmers. This will help you develop the skill of breaking down complex ideas into understandable narratives.
4. Record Yourself
Record video or audio of yourself solving and explaining coding problems. Review these recordings to identify areas for improvement in your communication and problem-solving approach.
5. Join Coding Communities
Participate in coding forums or local meetups where you can discuss problem-solving approaches with others. This exposure to different perspectives can enrich your storytelling abilities.
Conclusion
Mastering the art of storytelling in coding interviews is a powerful way to showcase not just your technical skills, but also your ability to think critically, communicate effectively, and approach problems methodically. By framing your problem-solving process as a narrative, you create a more engaging and memorable interview experience.
Remember, the goal is not just to solve the problem, but to take the interviewer on a journey through your thought process. Start by setting the scene with a clear understanding of the problem, introduce the characters (your chosen data structures and algorithms), develop the plot (your approach and implementation), reach the climax (testing and edge cases), and conclude with the moral of the story (complexity analysis).
With practice and mindful application of the tips we’ve discussed, you can transform your coding interviews from mere technical assessments into compelling stories of problem-solving prowess. This narrative approach will not only make you stand out as a candidate but also demonstrate the kind of clear, structured thinking that is invaluable in real-world software development scenarios.
So, the next time you face a coding interview, remember: you’re not just writing code, you’re telling the story of how you conquer challenges, one algorithm at a time. Good luck, and may your coding tales always have happy endings!