How to Master Algorithms Like a Mathematician: Solving Coding Problems Step-by-Step
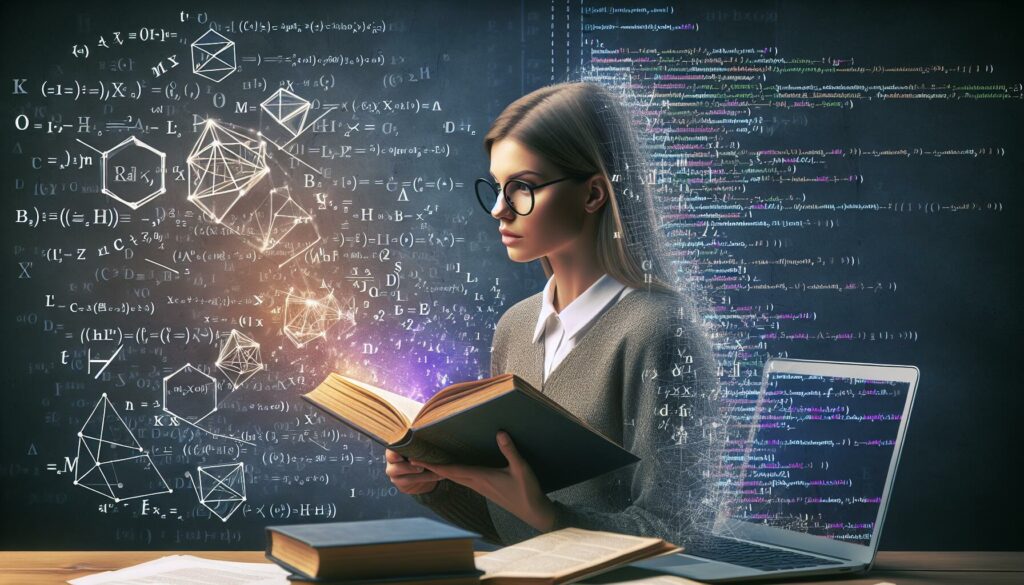
In the world of computer science and software engineering, mastering algorithms is akin to a mathematician wielding their most powerful tools. Algorithms are the backbone of efficient problem-solving in programming, and understanding them deeply can significantly enhance your coding skills. Whether you’re a beginner looking to improve or an experienced developer preparing for technical interviews at top tech companies, this guide will walk you through the process of mastering algorithms with a mathematician’s precision.
1. Understand the Fundamentals
Before diving into complex algorithms, it’s crucial to have a solid grasp of the fundamentals. This includes:
- Basic data structures (arrays, linked lists, stacks, queues)
- Time and space complexity analysis
- Basic algorithmic paradigms (brute force, divide and conquer, greedy algorithms)
- Mathematical concepts (logarithms, exponents, modular arithmetic)
These foundational elements will serve as building blocks for more advanced concepts. Platforms like AlgoCademy offer interactive tutorials that can help you solidify these basics through hands-on coding exercises.
2. Develop a Problem-Solving Mindset
Mathematicians approach problems systematically, and programmers should do the same. Here’s how to cultivate a problem-solving mindset:
- Break down the problem: Divide complex problems into smaller, manageable parts.
- Identify patterns: Look for similarities with problems you’ve solved before.
- Think abstractly: Consider the essence of the problem, not just its surface details.
- Visualize: Use diagrams or flowcharts to represent the problem and potential solutions.
Practice these techniques regularly, and you’ll find yourself approaching coding challenges with increased confidence and clarity.
3. Master Common Algorithmic Techniques
As you progress, focus on mastering these essential algorithmic techniques:
3.1 Dynamic Programming
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It’s particularly useful for optimization problems.
Key concepts:
- Memoization
- Tabulation
- Optimal substructure
Example problem: Fibonacci sequence calculation
def fibonacci(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
3.2 Depth-First Search (DFS) and Breadth-First Search (BFS)
These graph traversal algorithms are fundamental for solving problems involving trees, networks, and other graph structures.
Key concepts:
- Stack vs. Queue usage
- Recursive vs. Iterative implementations
- Visited node tracking
Example DFS implementation:
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start)
for next in graph[start] - visited:
dfs(graph, next, visited)
return visited
3.3 Binary Search
Binary search is an efficient algorithm for finding an item in a sorted list of items.
Key concepts:
- Divide and conquer
- Logarithmic time complexity
- Sorted input requirement
Example implementation:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
4. Practice, Practice, Practice
Like mathematicians who solve countless problems to hone their skills, programmers must practice regularly to master algorithms. Here are some effective ways to practice:
4.1 Solve Coding Challenges
Platforms like AlgoCademy, LeetCode, and HackerRank offer a wide range of coding challenges. Start with easier problems and gradually increase the difficulty. Aim to solve at least one problem daily.
4.2 Implement Algorithms from Scratch
Don’t just memorize algorithms; implement them yourself. This deepens your understanding and helps you internalize the logic behind each algorithm.
4.3 Participate in Coding Competitions
Join online coding contests or local hackathons. These events push you to solve problems under time pressure, simulating the conditions of technical interviews.
4.4 Review and Optimize Your Solutions
After solving a problem, don’t stop there. Look for ways to optimize your solution. Can you reduce the time complexity? Can you use less memory? This process of refinement is crucial for mastery.
5. Analyze Time and Space Complexity
A mathematician’s approach to algorithms involves rigorous analysis. Learn to analyze the time and space complexity of your solutions using Big O notation. This skill is crucial for optimizing your code and is often a focus in technical interviews.
5.1 Common Time Complexities
- O(1) – Constant time
- O(log n) – Logarithmic time
- O(n) – Linear time
- O(n log n) – Linearithmic time
- O(n^2) – Quadratic time
- O(2^n) – Exponential time
Practice identifying these complexities in your code and in standard algorithms. Understanding complexity helps you choose the right algorithm for a given problem and optimize your solutions effectively.
6. Learn from Others
Mathematicians often collaborate and learn from each other’s work. Similarly, programmers can benefit greatly from studying others’ solutions and approaches.
6.1 Read Code
Study well-written, efficient code. Platforms like GitHub allow you to explore open-source projects and see how experienced developers implement algorithms in real-world applications.
6.2 Participate in Code Reviews
If possible, participate in code reviews at work or in open-source projects. Reviewing others’ code and having your code reviewed provides valuable insights and exposes you to different problem-solving approaches.
6.3 Attend Coding Workshops and Webinars
Many organizations and online platforms offer workshops and webinars on advanced algorithmic concepts. These can provide structured learning experiences and the opportunity to learn from experts in the field.
7. Develop a Systematic Approach to Problem-Solving
Mathematicians often follow a structured approach when tackling complex problems. Adopt a similar methodology for coding challenges:
- Understand the problem: Read the problem statement carefully. Identify the input, expected output, and any constraints.
- Analyze examples: Look at the given examples and try to understand the pattern or logic behind them.
- Break it down: Divide the problem into smaller subproblems or steps.
- Plan your approach: Decide on the algorithm or data structure you’ll use to solve the problem.
- Implement the solution: Write clean, readable code to implement your planned approach.
- Test and debug: Run your code with the given examples and additional test cases. Debug any issues.
- Optimize: Look for ways to improve the efficiency of your solution.
This systematic approach helps ensure that you don’t miss important details and increases your chances of arriving at an optimal solution.
8. Understand the Theoretical Foundations
While practical coding skills are crucial, understanding the theoretical foundations of algorithms can significantly enhance your problem-solving abilities. Delve into topics such as:
- Graph theory
- Computational complexity theory
- Automata theory
- Formal languages
These theoretical concepts often provide insights that can lead to more elegant and efficient solutions to complex problems.
9. Implement Advanced Data Structures
As you progress in your algorithmic journey, familiarize yourself with and implement more advanced data structures. These can often be the key to solving complex problems efficiently:
- Trie
- Segment Tree
- Fenwick Tree (Binary Indexed Tree)
- Disjoint Set Union (Union-Find)
- Red-Black Tree
Understanding when and how to use these data structures can give you a significant advantage in solving algorithmic problems.
10. Simulate Interview Conditions
If you’re preparing for technical interviews at top tech companies, it’s important to simulate interview conditions:
- Practice coding on a whiteboard or using a simple text editor without auto-completion.
- Set time limits for solving problems.
- Explain your thought process out loud as you solve problems.
- Have a friend or mentor conduct mock interviews.
Platforms like AlgoCademy often provide features that simulate interview conditions, helping you prepare more effectively for real interviews.
11. Stay Updated with Current Trends
The field of algorithms and computer science is constantly evolving. Stay updated with the latest developments:
- Follow relevant blogs and publications.
- Attend conferences or watch conference talks online.
- Explore new algorithms and their applications in emerging fields like machine learning and blockchain.
12. Teach Others
One of the best ways to solidify your understanding of algorithms is to teach others. Consider:
- Starting a blog to explain algorithms and problem-solving techniques.
- Mentoring junior developers or students.
- Contributing to online forums and helping others solve algorithmic problems.
Teaching not only reinforces your own knowledge but also helps you gain new perspectives on familiar concepts.
Conclusion
Mastering algorithms like a mathematician is a journey that requires dedication, practice, and a systematic approach. By following the steps outlined in this guide and consistently challenging yourself with new problems, you can develop a deep understanding of algorithms and enhance your problem-solving skills.
Remember that becoming proficient in algorithms is not just about memorizing solutions, but about developing a mindset that allows you to approach new problems with confidence and creativity. Whether you’re aiming to excel in coding interviews at top tech companies or simply want to become a better programmer, the skills you develop through algorithmic mastery will serve you well throughout your career in software development.
Platforms like AlgoCademy can be invaluable resources on this journey, providing structured learning paths, interactive coding environments, and AI-powered assistance to help you progress from beginner to expert level. Embrace the challenge, stay curious, and keep pushing your boundaries. With persistence and the right approach, you can indeed master algorithms like a mathematician and unlock new levels of problem-solving prowess in your coding endeavors.