Coding Like a Chef: Using Recipes (Design Patterns) to Write Reusable Code
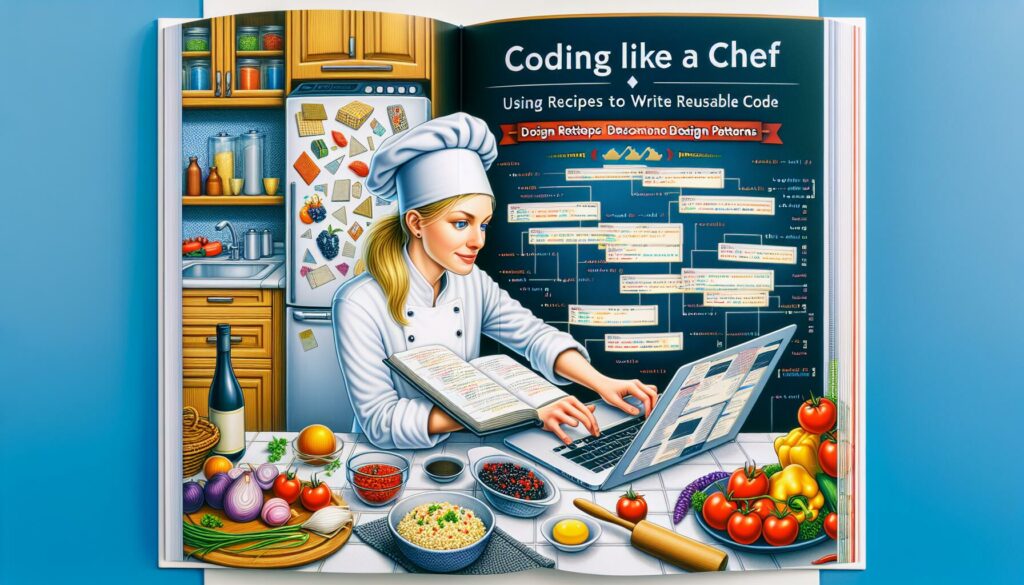
In the world of programming, writing efficient and reusable code is akin to being a master chef in the kitchen. Just as chefs use tried-and-tested recipes to create delicious dishes, programmers can leverage design patterns to craft elegant and maintainable software solutions. This article will explore how you can elevate your coding skills by understanding and implementing design patterns, ultimately helping you become a more proficient developer ready to tackle complex problems and excel in technical interviews.
What Are Design Patterns?
Design patterns are reusable solutions to common problems that occur in software design. They are not finished designs or code that can be directly copied into your program. Instead, they are templates or best practices that can be adapted to solve specific issues in your software architecture.
Think of design patterns as recipes in a cookbook. A recipe provides you with a list of ingredients and steps to follow, but you can still adjust it based on your preferences or available resources. Similarly, design patterns offer a blueprint for solving a particular problem, but you need to implement them in a way that fits your specific programming context.
Why Are Design Patterns Important?
Understanding and utilizing design patterns can significantly improve your coding skills and make you a more valuable developer. Here’s why:
- Reusability: Design patterns promote code reuse, reducing the need to reinvent the wheel for common programming challenges.
- Scalability: They provide proven solutions that can scale well as your project grows.
- Maintainability: By following established patterns, your code becomes more organized and easier to maintain.
- Communication: Design patterns create a common language among developers, making it easier to discuss and collaborate on software architecture.
- Problem-solving: They offer tested solutions to recurring problems, saving time and reducing the likelihood of introducing bugs.
Categories of Design Patterns
Design patterns are typically categorized into three main groups:
1. Creational Patterns
These patterns deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. Some examples include:
- Singleton
- Factory Method
- Abstract Factory
- Builder
- Prototype
2. Structural Patterns
Structural patterns focus on how classes and objects are composed to form larger structures. Examples include:
- Adapter
- Bridge
- Composite
- Decorator
- Facade
3. Behavioral Patterns
These patterns are concerned with algorithms and the assignment of responsibilities between objects. Some examples are:
- Observer
- Strategy
- Command
- State
- Iterator
Implementing Design Patterns: A Practical Approach
Let’s dive into some practical examples of how to implement design patterns in your code. We’ll look at three popular patterns from each category.
Creational Pattern: Singleton
The Singleton pattern ensures a class has only one instance and provides a global point of access to it. This is useful for managing shared resources, such as a database connection or a configuration manager.
Here’s an example of a Singleton pattern implementation in Python:
class DatabaseConnection:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super(DatabaseConnection, cls).__new__(cls)
cls._instance.connect()
return cls._instance
def connect(self):
# Simulate database connection
print("Connecting to the database...")
def query(self, sql):
# Simulate database query
print(f"Executing query: {sql}")
# Usage
db1 = DatabaseConnection()
db2 = DatabaseConnection()
print(db1 is db2) # Output: True
db1.query("SELECT * FROM users")
db2.query("INSERT INTO users (name) VALUES ('John')")
In this example, no matter how many times you instantiate DatabaseConnection
, you’ll always get the same instance, ensuring a single connection to the database.
Structural Pattern: Adapter
The Adapter pattern allows incompatible interfaces to work together. It’s like using a power adapter when traveling to a country with different electrical outlets.
Here’s an example of the Adapter pattern in Python:
class OldPrinter:
def print_old(self, text):
print(f"[Old Printer] {text}")
class NewPrinter:
def print_new(self, text):
print(f"[New Printer] {text}")
class PrinterAdapter:
def __init__(self, printer):
self.printer = printer
def print(self, text):
if isinstance(self.printer, OldPrinter):
self.printer.print_old(text)
elif isinstance(self.printer, NewPrinter):
self.printer.print_new(text)
# Usage
old_printer = OldPrinter()
new_printer = NewPrinter()
adapter1 = PrinterAdapter(old_printer)
adapter2 = PrinterAdapter(new_printer)
adapter1.print("Hello, World!") # Output: [Old Printer] Hello, World!
adapter2.print("Hello, World!") # Output: [New Printer] Hello, World!
This Adapter pattern allows us to use both old and new printer interfaces through a common print()
method.
Behavioral Pattern: Observer
The Observer pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. This is commonly used in event-driven programming.
Here’s an example of the Observer pattern in Python:
class Subject:
def __init__(self):
self._observers = []
self._state = None
def attach(self, observer):
self._observers.append(observer)
def detach(self, observer):
self._observers.remove(observer)
def notify(self):
for observer in self._observers:
observer.update(self._state)
def set_state(self, state):
self._state = state
self.notify()
class Observer:
def update(self, state):
pass
class ConcreteObserverA(Observer):
def update(self, state):
print(f"Observer A: Reacted to the event. New state: {state}")
class ConcreteObserverB(Observer):
def update(self, state):
print(f"Observer B: Reacted to the event. New state: {state}")
# Usage
subject = Subject()
observer_a = ConcreteObserverA()
observer_b = ConcreteObserverB()
subject.attach(observer_a)
subject.attach(observer_b)
subject.set_state("New State")
# Output:
# Observer A: Reacted to the event. New state: New State
# Observer B: Reacted to the event. New state: New State
In this example, the observers (A and B) are automatically notified when the subject’s state changes.
Benefits of Using Design Patterns in Your Code
Incorporating design patterns into your coding practice offers numerous advantages:
- Improved Code Quality: Design patterns are tried-and-tested solutions, which can lead to more robust and efficient code.
- Faster Development: By using established patterns, you can solve complex problems more quickly without having to reinvent solutions.
- Better Collaboration: Design patterns provide a common vocabulary for developers, making it easier to communicate ideas and collaborate on projects.
- Easier Maintenance: Code that follows well-known patterns is often easier to understand and maintain, even for developers who didn’t write the original code.
- Scalability: Many design patterns are created with scalability in mind, making it easier to expand your application as needed.
- Flexibility: Design patterns often make your code more flexible, allowing for easier modifications and extensions in the future.
Common Pitfalls to Avoid
While design patterns are powerful tools, it’s important to use them judiciously. Here are some common pitfalls to avoid:
- Overuse: Don’t try to force a design pattern into every part of your code. Use them only when they provide a clear benefit.
- Premature Optimization: Avoid implementing complex patterns early in development when simpler solutions might suffice.
- Ignoring Context: Remember that design patterns are guidelines, not strict rules. Always consider your specific context and requirements.
- Complexity for Complexity’s Sake: Don’t use a design pattern just to make your code look more sophisticated. The goal is to solve problems efficiently, not to showcase your knowledge of patterns.
- Neglecting Performance: Some design patterns can introduce performance overhead. Always consider the performance implications of the patterns you choose to implement.
Design Patterns and Technical Interviews
Understanding design patterns can be a significant advantage in technical interviews, especially for positions at major tech companies. Here’s how knowledge of design patterns can help you:
- Problem-Solving Skills: Interviewers often present complex scenarios that can be efficiently solved using design patterns. Recognizing when and how to apply these patterns demonstrates strong problem-solving skills.
- Code Organization: Using design patterns in your solutions shows that you can write well-organized, maintainable code – a crucial skill for any professional developer.
- System Design Questions: Many system design interview questions can be addressed using various design patterns. For example, the Observer pattern could be useful in designing a notification system.
- Demonstrating Best Practices: By incorporating design patterns, you show that you’re familiar with industry best practices and can write production-quality code.
- Handling Edge Cases: Many design patterns are created to handle specific edge cases or common problems. Showing awareness of these can impress interviewers.
Practical Exercises to Master Design Patterns
To truly internalize design patterns and be able to use them effectively, practice is key. Here are some exercises you can try:
- Refactor Existing Code: Take a piece of code you’ve written before and try to refactor it using appropriate design patterns. This will help you see how patterns can improve existing code.
- Build a Mini-Project: Create a small project that incorporates multiple design patterns. For example, you could build a simple game engine using the Observer pattern for event handling, the Factory pattern for creating game objects, and the State pattern for managing game states.
- Solve LeetCode Problems: Try solving LeetCode problems with design patterns in mind. While many algorithmic problems don’t require design patterns, some more complex ones can benefit from their application.
- Implement Each Pattern: Go through each of the major design patterns and implement them in your preferred programming language. This will give you hands-on experience with how each pattern works.
- Code Review: If possible, participate in code reviews or review open-source projects. Try to identify where design patterns are used (or could be used) in the code.
Conclusion: Cooking Up Great Code with Design Patterns
Just as a chef combines ingredients and techniques to create delicious meals, a skilled programmer uses design patterns to craft elegant, efficient, and maintainable code. By understanding and applying these “recipes” for software design, you can elevate your coding skills, solve complex problems more effectively, and stand out in technical interviews.
Remember, the key to mastering design patterns is practice and application. Start by identifying common patterns in code you encounter, then gradually incorporate them into your own projects. As you become more comfortable with these patterns, you’ll find yourself naturally reaching for the right “ingredient” to solve each programming challenge you face.
Whether you’re preparing for a technical interview at a major tech company or simply aiming to improve your coding skills, a solid understanding of design patterns is an invaluable asset. So, put on your chef’s hat, fire up your IDE, and start cooking up some fantastic code!
Additional Resources
To further your understanding of design patterns, consider exploring these resources:
- “Design Patterns: Elements of Reusable Object-Oriented Software” by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides (also known as the “Gang of Four” book) – This is the seminal work on design patterns and a must-read for any serious developer.
- “Head First Design Patterns” by Eric Freeman and Elisabeth Robson – A more accessible introduction to design patterns with lots of visual aids and examples.
- Refactoring.Guru (https://refactoring.guru/design-patterns) – An excellent online resource with clear explanations and examples of various design patterns.
- GitHub repositories with design pattern implementations in various languages – These can provide practical examples of how patterns are used in real-world code.
Remember, the journey to mastering design patterns is ongoing. Keep practicing, stay curious, and always look for opportunities to apply these powerful tools in your coding projects. Happy coding!