From Zero to Hero in Python: Why It’s the Best Language for Self-Taught Programmers
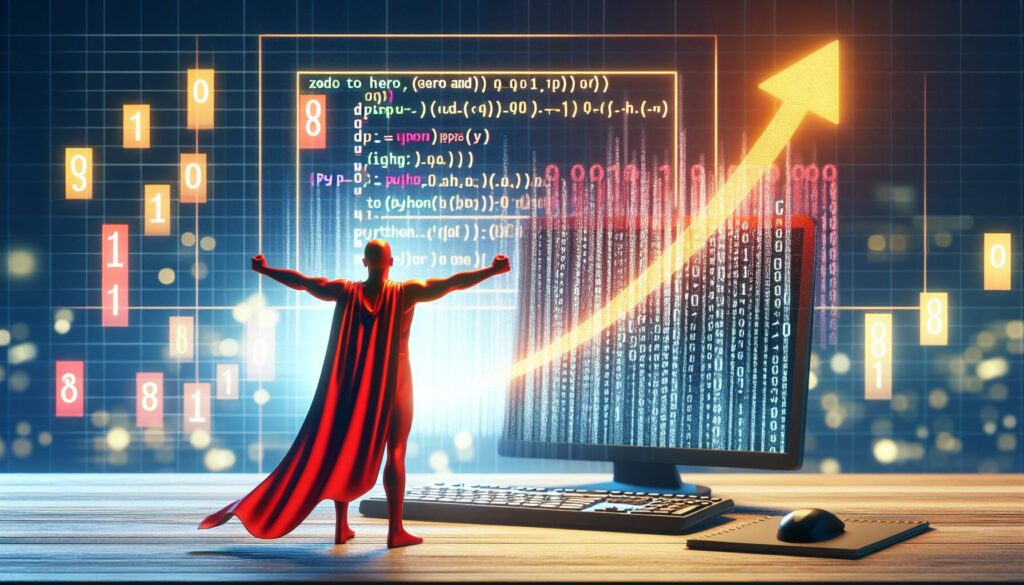
In the ever-evolving world of technology, programming skills have become increasingly valuable. Whether you’re looking to switch careers, enhance your current job prospects, or simply explore a new hobby, learning to code can open up a world of opportunities. Among the myriad of programming languages available, Python stands out as an excellent choice for self-taught programmers. In this comprehensive guide, we’ll explore why Python is the ideal language for beginners and how you can go from zero to hero in your coding journey.
Why Python is Perfect for Self-Taught Programmers
1. Easy to Learn and Read
Python’s syntax is clean, simple, and resembles natural language, making it incredibly easy for beginners to grasp. Unlike some other languages that use complex symbols and structures, Python uses indentation and simple keywords, which makes the code more readable and intuitive.
For example, here’s a simple “Hello, World!” program in Python:
print("Hello, World!")
Compare this to the same program in Java:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
The simplicity of Python’s syntax allows beginners to focus on learning programming concepts rather than getting bogged down by complex language rules.
2. Versatility
Python is a versatile language that can be used for a wide range of applications, including:
- Web development (with frameworks like Django and Flask)
- Data analysis and visualization
- Machine learning and artificial intelligence
- Scientific computing
- Game development
- Automation and scripting
This versatility means that as you progress in your learning journey, you can apply your Python skills to various domains and projects, keeping your learning experience engaging and relevant.
3. Large and Supportive Community
Python boasts a vast and active community of developers, which is invaluable for self-taught programmers. This community contributes to:
- Extensive documentation and tutorials
- A wide range of third-party libraries and frameworks
- Active forums and Q&A platforms (like Stack Overflow) where you can get help
- Regular updates and improvements to the language
Having access to such a supportive community can significantly accelerate your learning process and help you overcome challenges along the way.
4. Abundance of Learning Resources
Due to its popularity, there is no shortage of learning resources for Python. From free online courses and tutorials to comprehensive books and video series, you’ll find materials suited to every learning style and level. Some popular resources include:
- Codecademy’s Python courses
- Python.org’s official tutorials
- Coursera and edX Python specializations
- “Automate the Boring Stuff with Python” by Al Sweigart
- YouTube channels like Corey Schafer’s Python tutorials
This abundance of resources ensures that you can always find the right material to support your learning journey, regardless of your current skill level.
Getting Started with Python: A Step-by-Step Guide
Now that we’ve established why Python is an excellent choice for self-taught programmers, let’s dive into how you can get started on your journey from zero to hero.
Step 1: Install Python
The first step is to install Python on your computer. Visit the official Python website (python.org) and download the latest version for your operating system. Follow the installation instructions, and make sure to add Python to your system’s PATH during installation.
Step 2: Choose an Integrated Development Environment (IDE)
While you can write Python code in any text editor, using an IDE can significantly enhance your coding experience. Some popular IDEs for Python include:
- PyCharm: A powerful IDE with a free community edition
- Visual Studio Code: A lightweight, customizable editor with excellent Python support
- IDLE: Python’s built-in IDE, suitable for beginners
Step 3: Learn the Basics
Start by learning the fundamental concepts of Python programming. This includes:
- Variables and data types
- Basic operators
- Conditional statements (if, else, elif)
- Loops (for and while)
- Functions
- Lists, tuples, and dictionaries
Here’s a simple example that incorporates some of these basics:
def greet(name):
return f"Hello, {name}!"
names = ["Alice", "Bob", "Charlie"]
for name in names:
greeting = greet(name)
print(greeting)
This code defines a function, uses a list, and implements a loop to greet multiple people.
Step 4: Practice, Practice, Practice
The key to mastering any programming language is practice. Start with small projects and coding exercises. Websites like HackerRank, LeetCode, and Project Euler offer coding challenges that can help you hone your skills.
Step 5: Explore Python Libraries
One of Python’s strengths is its extensive collection of libraries. As you become more comfortable with the basics, start exploring libraries relevant to your interests. Some popular libraries include:
- NumPy and Pandas for data analysis
- Matplotlib and Seaborn for data visualization
- Scikit-learn for machine learning
- Django and Flask for web development
Step 6: Build Projects
Apply your knowledge by building real-world projects. This could be a simple web scraper, a data analysis tool, or a basic web application. Building projects will help you understand how different components of the language work together and give you practical experience.
Step 7: Learn Object-Oriented Programming (OOP)
Once you’re comfortable with the basics, dive into object-oriented programming. Python is an object-oriented language, and understanding OOP concepts will take your skills to the next level. Here’s a simple example of a class in Python:
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def bark(self):
return f"{self.name} says Woof!"
my_dog = Dog("Buddy", 3)
print(my_dog.bark()) # Output: Buddy says Woof!
Step 8: Contribute to Open Source
Contributing to open-source projects is a great way to improve your skills, learn from experienced developers, and give back to the community. Platforms like GitHub have many Python projects looking for contributors at all skill levels.
Advanced Topics in Python
As you progress from beginner to intermediate and advanced levels, you’ll encounter more complex topics in Python. Here are some areas to explore:
1. Decorators
Decorators are a powerful feature in Python that allow you to modify or enhance functions without changing their source code. Here’s a simple example:
def uppercase_decorator(function):
def wrapper():
result = function()
return result.upper()
return wrapper
@uppercase_decorator
def greet():
return "hello, world!"
print(greet()) # Output: HELLO, WORLD!
2. Generators
Generators are functions that can be paused and resumed, allowing you to generate a sequence of values over time. They are memory-efficient and useful for working with large datasets:
def fibonacci_generator():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
fib = fibonacci_generator()
for _ in range(10):
print(next(fib))
3. Context Managers
Context managers allow you to allocate and release resources precisely. The most common use is with file handling:
with open("example.txt", "w") as file:
file.write("Hello, World!")
4. Multithreading and Multiprocessing
For concurrent programming, Python offers both multithreading and multiprocessing capabilities. These are crucial for writing efficient programs that can utilize modern multi-core processors.
5. Asynchronous Programming
Python’s asyncio library allows for asynchronous programming, which is particularly useful for I/O-bound operations. Here’s a simple example:
import asyncio
async def main():
print("Hello")
await asyncio.sleep(1)
print("World")
asyncio.run(main())
Python in the Real World: Applications and Career Opportunities
As you progress in your Python journey, you’ll find that the language opens up numerous career opportunities across various industries. Here are some areas where Python is widely used:
1. Data Science and Machine Learning
Python is the go-to language for data scientists and machine learning engineers. Libraries like NumPy, Pandas, and Scikit-learn make it easy to perform complex data analysis and build machine learning models.
2. Web Development
With frameworks like Django and Flask, Python is a popular choice for backend web development. Many companies, including Instagram and Mozilla, use Python for their web applications.
3. Artificial Intelligence
Python’s simplicity and powerful libraries make it an excellent choice for AI development. Libraries like TensorFlow and PyTorch are widely used in the AI community.
4. Game Development
While not as common as C++ for game development, Python is used in game development, particularly for scripting and prototyping. The Pygame library is popular for creating 2D games.
5. Finance
Many financial institutions use Python for quantitative and qualitative analysis. Its data processing capabilities make it valuable for tasks like risk management and trade automation.
6. Scientific Computing
Python’s scientific computing libraries, such as SciPy and SymPy, make it a valuable tool in fields like physics, chemistry, and biology.
Continuing Your Python Journey
Becoming proficient in Python is a journey that requires dedication and continuous learning. Here are some tips to help you continue growing as a Python developer:
1. Stay Updated
Python is constantly evolving. Stay updated with the latest features and best practices by following Python blogs, attending conferences, or joining local Python user groups.
2. Read Other People’s Code
Reading well-written Python code can teach you new techniques and best practices. Explore open-source projects on platforms like GitHub to see how experienced developers structure their code.
3. Teach Others
Teaching is an excellent way to solidify your understanding. Consider starting a blog, creating tutorials, or mentoring beginners in your community.
4. Specialize
While it’s good to have broad knowledge, consider specializing in a particular area of Python development that aligns with your interests and career goals.
5. Participate in Code Reviews
If possible, participate in code reviews. Reviewing others’ code and having your code reviewed can provide valuable insights and help you improve your coding skills.
Conclusion
Python’s simplicity, versatility, and robust community support make it an ideal language for self-taught programmers. By following the steps outlined in this guide and consistently practicing, you can go from a complete beginner to a proficient Python developer. Remember, the journey from zero to hero in programming is a marathon, not a sprint. Embrace the learning process, stay curious, and don’t be afraid to make mistakes along the way.
As you progress in your Python journey, you’ll discover that the language opens up a world of possibilities. Whether you’re interested in web development, data science, artificial intelligence, or any other field of technology, Python provides you with the tools and flexibility to bring your ideas to life.
So, take that first step, write your first “Hello, World!” program, and embark on your exciting journey into the world of Python programming. With dedication and persistence, you’ll be amazed at how quickly you can progress from a coding novice to a Python hero. Happy coding!