How to Avoid ‘Tutorial Hell’: Break Free from Following and Start Building
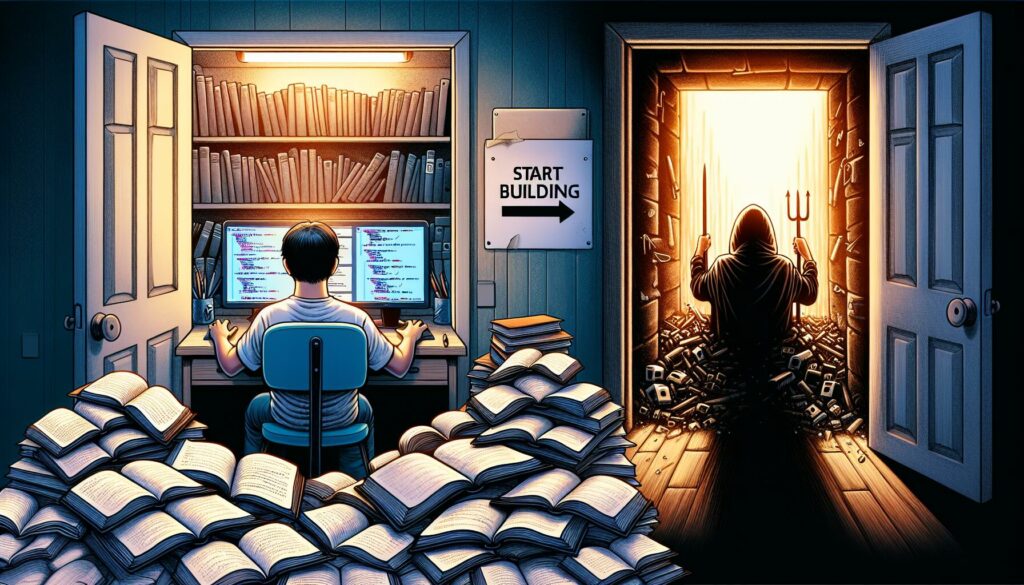
In the world of coding and programming, there’s a common pitfall that many aspiring developers fall into: ‘Tutorial Hell’. This phenomenon occurs when learners become stuck in an endless cycle of following tutorials without ever applying their knowledge to real-world projects. While tutorials are an essential part of the learning process, relying on them exclusively can hinder your growth as a programmer. In this comprehensive guide, we’ll explore what tutorial hell is, why it’s problematic, and most importantly, how to break free from it and start building your own projects.
Understanding Tutorial Hell
Tutorial hell is a state where a learner continuously consumes educational content without taking the crucial step of applying that knowledge independently. It’s characterized by:
- Constantly jumping from one tutorial to another
- Feeling confident while following along, but lost when trying to code independently
- Lack of progress in personal projects
- Dependency on step-by-step instructions
- Fear of making mistakes or facing errors
While tutorials are valuable resources, especially for beginners, they can become a crutch that prevents true learning and skill development if relied upon too heavily.
The Dangers of Staying in Tutorial Hell
Remaining in tutorial hell can have several negative consequences for your programming journey:
- Limited problem-solving skills: You may struggle to tackle real-world problems that don’t have a specific tutorial.
- Lack of creativity: Following tutorials step-by-step doesn’t encourage creative thinking or innovative solutions.
- Imposter syndrome: You might feel like you’re not a “real” programmer because you can’t code without guidance.
- Slow progress: Your skills may not develop as quickly as they could if you were working on independent projects.
- Difficulty in interviews: Technical interviews often require you to solve problems on the spot, which can be challenging if you’re used to following tutorials.
Signs You’re Stuck in Tutorial Hell
Recognizing that you’re in tutorial hell is the first step to breaking free. Here are some signs to watch out for:
- You’ve completed numerous tutorials but haven’t built any projects of your own
- You feel lost when trying to start a project from scratch
- You’re constantly searching for tutorials on basic concepts you’ve already covered
- You avoid coding challenges or exercises that don’t provide step-by-step solutions
- You feel anxious about making mistakes or encountering errors in your code
Strategies to Break Free from Tutorial Hell
Now that we’ve identified the problem, let’s explore effective strategies to escape tutorial hell and start building your own projects:
1. Set Clear Goals
Before diving into any tutorial or project, define what you want to achieve. Having clear, specific goals will help you focus on learning what’s necessary to accomplish them, rather than aimlessly following tutorials.
Example goal: “Build a functional weather app using React and a weather API within the next month.”
2. Practice Active Learning
Instead of passively following tutorials, engage with the content actively:
- Take notes on key concepts
- Pause videos to try coding solutions yourself before seeing the answer
- Experiment with changing variables or functions to see how it affects the outcome
- Try to explain the concepts you’re learning in your own words
3. Build Projects Alongside Tutorials
As you work through tutorials, apply the concepts to a personal project. This helps reinforce your learning and gives you practical experience.
For example, if you’re following a tutorial on building a to-do list app, create your own version with additional features or a different theme.
4. Embrace the ‘Read, Search, Ask’ Method
When you encounter a problem:
- Read the error message or documentation carefully
- Search for solutions online (Stack Overflow, documentation, forums)
- Ask for help in coding communities if you’re still stuck
This approach encourages problem-solving skills and reduces dependency on tutorials.
5. Start with Small, Achievable Projects
Begin with simple projects that you can complete relatively quickly. This builds confidence and provides a sense of accomplishment. As you progress, gradually increase the complexity of your projects.
Examples of beginner-friendly projects:
- A personal portfolio website
- A calculator app
- A simple game like Tic-Tac-Toe or Rock-Paper-Scissors
6. Join Coding Challenges and Hackathons
Participate in coding challenges on platforms like LeetCode, HackerRank, or CodeWars. These challenges force you to think independently and solve problems without step-by-step guidance. Hackathons are also great opportunities to apply your skills in a time-constrained, collaborative environment.
7. Contribute to Open Source Projects
Contributing to open source projects exposes you to real-world codebases and collaborative development. Start with small contributions like fixing bugs or improving documentation, then gradually take on larger tasks.
8. Learn to Read and Understand Documentation
Develop the skill of reading and understanding official documentation. This is crucial for working with new technologies or APIs without relying on tutorials.
Practice by building small projects using only the official documentation as a reference.
9. Embrace Errors and Debugging
Instead of fearing errors, view them as learning opportunities. Develop your debugging skills by:
- Reading error messages carefully
- Using console.log() or debugger tools to understand what’s happening in your code
- Breaking down problems into smaller, manageable parts
10. Teach Others
Teaching is one of the best ways to solidify your understanding. Start a blog, create video tutorials, or mentor other learners. Explaining concepts to others will highlight gaps in your knowledge and deepen your understanding.
Building Your First Independent Project
Now that we’ve discussed strategies to break free from tutorial hell, let’s walk through the process of building your first independent project. We’ll use a simple weather app as an example.
Step 1: Plan Your Project
Before writing any code, outline your project:
- Define the features (e.g., display current weather, 5-day forecast, search by city)
- Choose your tech stack (e.g., HTML, CSS, JavaScript, and a weather API)
- Sketch a basic design or user interface
Step 2: Set Up Your Development Environment
Create a new directory for your project and set up version control:
mkdir weather-app
cd weather-app
git init
touch index.html style.css script.js
Step 3: Create the HTML Structure
Start with a basic HTML structure:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Weather App</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="app">
<h1>Weather App</h1>
<input type="text" id="city-input" placeholder="Enter city name">
<button id="search-btn">Search</button>
<div id="weather-info"></div>
</div>
<script src="script.js"></script>
</body>
</html>
Step 4: Style Your App
Add some basic CSS to style your app:
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f0f0f0;
}
#app {
background-color: white;
padding: 20px;
border-radius: 5px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.1);
}
input, button {
margin: 10px 0;
padding: 5px;
}
#weather-info {
margin-top: 20px;
}
Step 5: Implement the JavaScript Logic
Now, let’s add the JavaScript to fetch weather data and update the UI:
const apiKey = 'YOUR_API_KEY'; // Replace with your actual API key
const cityInput = document.getElementById('city-input');
const searchBtn = document.getElementById('search-btn');
const weatherInfo = document.getElementById('weather-info');
searchBtn.addEventListener('click', () => {
const city = cityInput.value;
if (city) {
fetchWeather(city);
}
});
async function fetchWeather(city) {
try {
const response = await fetch(`https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${apiKey}&units=metric`);
const data = await response.json();
displayWeather(data);
} catch (error) {
console.error('Error fetching weather data:', error);
weatherInfo.textContent = 'Failed to fetch weather data. Please try again.';
}
}
function displayWeather(data) {
const { name, main, weather } = data;
weatherInfo.innerHTML = `
<h2>Weather in ${name}</h2>
<p>Temperature: ${main.temp}°C</p>
<p>Feels like: ${main.feels_like}°C</p>
<p>Description: ${weather[0].description}</p>
`;
}
Remember to replace ‘YOUR_API_KEY’ with an actual API key from OpenWeatherMap or a similar service.
Step 6: Test and Debug
Open your index.html file in a browser and test your app. Enter a city name and click the search button. If you encounter any errors, use the browser’s developer tools to debug and fix issues.
Step 7: Enhance and Expand
Once your basic app is working, consider adding more features or improving the design. Some ideas:
- Add error handling for invalid city names
- Include weather icons
- Implement a 5-day forecast
- Add geolocation to automatically detect the user’s location
Conclusion: Embracing the Journey of Independent Learning
Breaking free from tutorial hell is a crucial step in your journey as a programmer. It’s about transitioning from passive consumption to active creation. Remember, the goal isn’t to never use tutorials again, but to use them as tools for learning rather than as crutches.
As you start building your own projects, you’ll face challenges and make mistakes. Embrace these experiences – they’re invaluable parts of the learning process. Each error you encounter and solve is a step towards becoming a more competent and confident developer.
Keep in mind that even experienced developers regularly consult documentation, search for solutions, and learn new things. The key is to approach these resources with a problem-solving mindset rather than looking for exact, step-by-step instructions.
Finally, remember that programming is a journey of continuous learning. As you break free from tutorial hell and start building, you’ll discover new technologies, techniques, and challenges. Embrace this ongoing process of growth and discovery. Your path may not always be smooth, but each project you complete and each problem you solve will bring you closer to your goals as a developer.
So, take that first step. Choose a project, start coding, and embrace the learning process. Your future as a skilled, independent developer begins now!