Coding for the Visual Learner: How Diagrams and Flowcharts Can Enhance Your Programming Skills
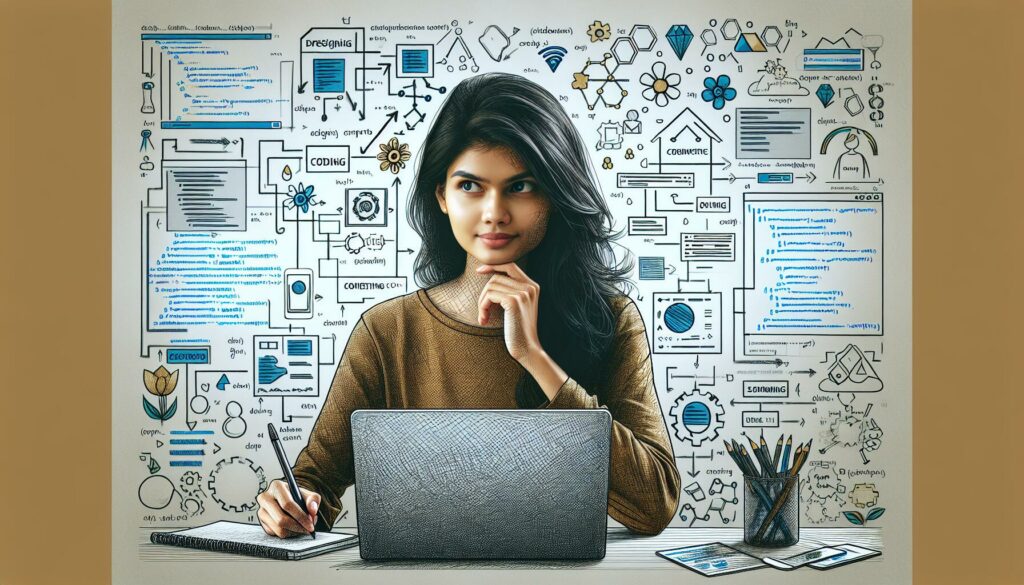
In the world of programming, where lines of code can stretch endlessly across a screen, visual learners often find themselves at a disadvantage. The abstract nature of coding can be challenging for those who prefer to see concepts laid out in a more tangible, visual format. However, there’s good news: diagrams and flowcharts can be powerful tools to enhance your programming skills, making complex algorithms and code structures more accessible and easier to understand.
In this comprehensive guide, we’ll explore how visual aids like diagrams and flowcharts can revolutionize your coding education and boost your programming prowess. Whether you’re a beginner just starting your coding journey or an experienced developer looking to refine your skills, incorporating visual elements into your learning process can lead to significant improvements in comprehension and problem-solving abilities.
The Power of Visual Learning in Programming
Before we dive into the specific benefits of diagrams and flowcharts, let’s understand why visual learning can be so effective in the context of programming:
- Simplification of Complex Concepts: Visual representations can break down intricate coding concepts into more digestible chunks, making them easier to grasp and remember.
- Enhanced Pattern Recognition: Diagrams help in identifying patterns and relationships between different components of a program, which is crucial for algorithmic thinking.
- Improved Problem-Solving: Visualizing a problem often leads to more creative and efficient solutions, as it allows you to see the big picture and potential roadblocks.
- Better Memory Retention: Visual information is typically easier to recall than text-based information, helping you retain programming concepts for longer periods.
- Facilitated Communication: Diagrams and flowcharts provide a universal language for discussing code structures and algorithms with other developers.
Types of Diagrams and Flowcharts for Programmers
There are several types of diagrams and flowcharts that can be particularly useful for programmers. Let’s explore some of the most common and effective ones:
1. Flowcharts
Flowcharts are perhaps the most well-known type of diagram in programming. They use shapes and arrows to represent the flow of a program or algorithm. Here’s why flowcharts are invaluable:
- They provide a clear, step-by-step visualization of program logic.
- They help identify decision points and potential branches in your code.
- They’re excellent for planning before you start coding, ensuring you have a solid structure in place.
Example of a simple flowchart for a login process:
<!-- Flowchart diagram would be inserted here -->
Start
|
V
[Input Username and Password]
|
V
<Is input valid?>
| |
Yes No
| |
V V
[Grant Access] [Display Error Message]
| |
V V
End [Return to Input]
2. UML Diagrams
Unified Modeling Language (UML) diagrams are a standardized set of visualization tools used in software engineering. They come in various types, each serving a different purpose:
- Class Diagrams: Illustrate the structure of a system by showing classes, their attributes, methods, and relationships between classes.
- Sequence Diagrams: Depict how objects interact with each other over time, which is particularly useful for understanding complex processes.
- Use Case Diagrams: Show the interactions between users (actors) and the system, helping to define system requirements.
Example of a simple UML Class Diagram:
<!-- UML Class Diagram would be inserted here -->
+-------------------+
| User |
+-------------------+
| - username: String|
| - password: String|
+-------------------+
| + login(): boolean|
| + logout(): void |
+-------------------+
3. Entity-Relationship Diagrams (ERDs)
ERDs are crucial for database design and management. They show:
- The structure of a database, including tables and their relationships.
- The attributes (columns) of each table.
- The types of relationships between tables (one-to-one, one-to-many, many-to-many).
Understanding ERDs is essential for anyone working with databases or developing data-driven applications.
4. Mind Maps
While not strictly a programming diagram, mind maps can be incredibly useful for:
- Brainstorming project ideas and features.
- Organizing thoughts when tackling a complex programming problem.
- Planning the structure of a large codebase or application.
Mind maps start with a central concept and branch out into related ideas, creating a visual representation of your thought process.
How Diagrams and Flowcharts Enhance Programming Skills
Now that we’ve covered the types of diagrams, let’s delve into how they can specifically enhance your programming skills:
1. Improved Algorithm Design
Designing efficient algorithms is at the heart of good programming. Flowcharts and diagrams can significantly improve your algorithm design skills by:
- Allowing you to visualize the steps of an algorithm before coding.
- Helping identify potential bottlenecks or inefficiencies in your logic.
- Making it easier to compare different approaches to solving a problem.
For example, when tackling a sorting algorithm like quicksort, a flowchart can help you understand the recursive nature of the algorithm and the partitioning process more intuitively than looking at code alone.
2. Better Code Structure and Organization
Using diagrams, particularly UML class diagrams, can lead to better-structured and more organized code. Here’s how:
- Class diagrams help you plan your object-oriented design before implementation.
- They encourage thinking about relationships between classes, promoting modularity and reusability.
- Visualizing the structure can help identify potential design patterns that could improve your code.
For instance, when designing a game engine, a class diagram can help you visualize how different components like rendering, physics, and input handling interact, leading to a more modular and maintainable codebase.
3. Enhanced Debugging Skills
Diagrams and flowcharts can be powerful allies in the debugging process:
- Flowcharts can help trace the execution path of a program, making it easier to identify where errors might occur.
- Sequence diagrams can illustrate the flow of method calls, helping pinpoint issues in object interactions.
- Visualizing the problem often leads to “aha!” moments in identifying bugs.
When debugging a complex multi-threaded application, for example, a sequence diagram can help you understand the order of operations and potential race conditions that might be causing unexpected behavior.
4. Improved Communication with Team Members
In a professional setting, the ability to communicate your ideas clearly is crucial. Diagrams serve as a universal language that can:
- Help explain complex algorithms or system designs to non-technical stakeholders.
- Facilitate discussions among team members about code structure and system architecture.
- Serve as documentation for future reference or for onboarding new team members.
For instance, when proposing a new feature for a web application, a combination of use case diagrams and sequence diagrams can effectively communicate both the user interaction and the underlying system processes to your team and stakeholders.
5. Accelerated Learning of New Concepts
When learning new programming concepts or frameworks, diagrams can significantly speed up the process:
- Flowcharts can help understand the lifecycle of processes or components in frameworks like React or Angular.
- ERDs can quickly convey the structure of a database system you’re learning to work with.
- Mind maps can organize information about a new programming language’s features and syntax.
For example, when learning about microservices architecture, a diagram showing the different services and their interactions can provide a much clearer understanding than text descriptions alone.
Incorporating Diagrams and Flowcharts into Your Coding Practice
Now that we’ve established the benefits, let’s look at how you can start incorporating these visual tools into your coding practice:
1. Start with Pen and Paper
Before diving into digital tools, start with the basics:
- Keep a notebook handy for quick sketches of ideas or problem-solving attempts.
- Use whiteboards for collaborative brainstorming sessions.
- Practice drawing common diagram elements like flowchart shapes or UML notations.
2. Utilize Digital Tools
There are numerous software options available for creating professional-looking diagrams:
- Draw.io: A free, web-based tool that’s great for creating various types of diagrams.
- Lucidchart: Offers a wide range of templates and is particularly good for collaborative diagramming.
- PlantUML: A text-based diagramming tool that’s excellent for version control and quick UML diagrams.
- Microsoft Visio: A powerful tool with a wide range of diagram types, popular in enterprise environments.
3. Integrate Diagramming into Your Workflow
Make creating diagrams a regular part of your coding process:
- Start projects by sketching out the overall structure and flow.
- Use diagrams to plan complex functions or methods before coding.
- Create ERDs before designing databases.
- Use mind maps for project planning and feature brainstorming.
4. Study and Recreate Diagrams
To improve your diagramming skills:
- Study diagrams in programming books and tutorials.
- Try to recreate diagrams from memory to reinforce your understanding.
- Practice creating diagrams for existing code or systems you’re familiar with.
5. Use Diagrams for Documentation
Incorporate diagrams into your code documentation:
- Add flowcharts to explain complex algorithms in comments or README files.
- Use class diagrams to document the structure of your object-oriented designs.
- Include sequence diagrams to explain intricate processes in your system.
Challenges and Solutions in Visual Learning for Programming
While visual learning through diagrams and flowcharts offers numerous benefits, it’s not without its challenges. Here are some common issues you might face and how to overcome them:
1. Oversimplification
Challenge: Diagrams can sometimes oversimplify complex concepts, leading to a false sense of understanding.
Solution: Use diagrams as a starting point, but always dive deeper into the code and documentation. Combine visual learning with hands-on coding practice to ensure a thorough understanding.
2. Time-Consuming
Challenge: Creating detailed diagrams can be time-consuming, especially when you’re under pressure to deliver code quickly.
Solution: Start with quick sketches and only elaborate on diagrams for complex parts of your system. Use tools that allow for rapid diagramming, and consider it an investment in code quality and maintainability.
3. Keeping Diagrams Updated
Challenge: As code evolves, diagrams can quickly become outdated, leading to confusion.
Solution: Integrate diagram updates into your code review process. Use version control for your diagrams, and consider tools that can generate diagrams directly from your code to keep them in sync.
4. Learning Curve for Diagramming Standards
Challenge: Standards like UML have their own syntax and rules, which can be intimidating for beginners.
Solution: Start with basic flowcharts and gradually introduce more complex diagram types. Focus on the essence of what you’re trying to communicate rather than getting bogged down in notation details initially.
5. Overreliance on Visual Representations
Challenge: Some learners might become too dependent on visual aids, struggling when they need to work with code directly.
Solution: Use diagrams as a complement to, not a replacement for, coding practice. Regularly challenge yourself to translate between visual representations and actual code.
Conclusion: Empowering Your Coding Journey with Visual Tools
Incorporating diagrams and flowcharts into your programming practice can significantly enhance your coding skills, particularly if you’re a visual learner. These tools offer a way to simplify complex concepts, improve problem-solving abilities, and communicate ideas more effectively.
Remember, the goal is not to replace coding with diagramming, but to use visual aids as a powerful complement to your programming toolkit. By leveraging the strengths of both visual and textual representations, you can develop a more comprehensive understanding of programming concepts and improve your ability to design, implement, and debug complex systems.
As you continue your coding journey, experiment with different types of diagrams and find the methods that work best for you. Whether you’re tackling algorithm design, planning a new project, or trying to understand a complex codebase, having these visual tools at your disposal will make you a more versatile and effective programmer.
Embrace the power of visual learning in your coding education, and watch as your programming skills reach new heights. Happy coding and diagramming!