Why Learning Data Structures is Like Packing for a Trip: How to Choose the Right Tools
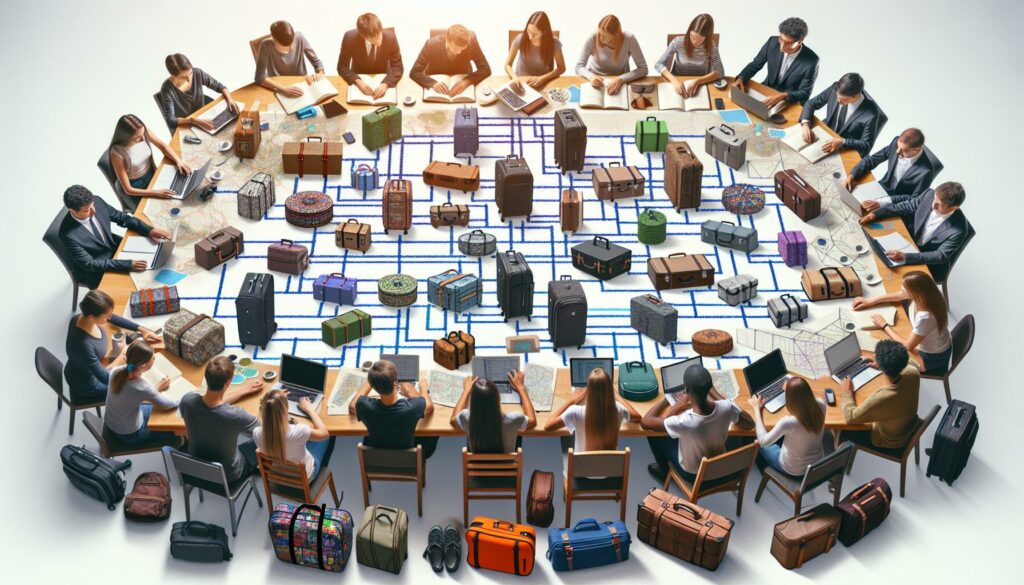
Embarking on a journey to master data structures is much like preparing for an exciting trip. Just as you carefully select the items to pack in your suitcase, choosing the right data structures for your programming tasks is crucial for efficient and effective code. In this comprehensive guide, we’ll explore the parallels between learning data structures and packing for a trip, providing you with the knowledge and tools you need to become a proficient programmer.
The Importance of Data Structures in Programming
Before we dive into our travel analogy, let’s first understand why data structures are so vital in the world of programming. Data structures are the building blocks of efficient algorithms and well-organized code. They provide a way to store, organize, and manage data, allowing for faster processing, easier maintenance, and more scalable solutions.
Just as experienced travelers know the value of packing the right items for their journey, seasoned programmers understand the importance of selecting the appropriate data structures for their projects. The right choice can lead to optimal performance, while the wrong one can result in slower execution times and increased complexity.
Packing Your Programming Suitcase: Essential Data Structures
Let’s start our journey by exploring some of the most common data structures you’ll want to pack in your programming suitcase:
1. Arrays: The Versatile T-Shirt
Arrays are like the versatile t-shirts you pack for any trip. They’re simple, flexible, and can be used in various situations. Arrays store elements of the same type in contiguous memory locations, allowing for quick access and manipulation of data.
When to pack arrays:
- When you need to store a fixed number of elements
- For quick access to elements using an index
- When implementing basic data structures like stacks or queues
Example of array declaration in Java:
int[] numbers = new int[5]; // Declares an array of 5 integers
String[] names = {"Alice", "Bob", "Charlie"}; // Declares and initializes an array of strings
2. Linked Lists: The Adaptable Jacket
Linked lists are like the adaptable jacket you bring on a trip. They can be easily adjusted (by adding or removing nodes) and come in different styles (singly-linked, doubly-linked, or circular). Linked lists consist of nodes, where each node contains data and a reference to the next node.
When to pack linked lists:
- When you need frequent insertions or deletions
- For implementing more complex data structures like stacks, queues, or graphs
- When memory usage needs to be more dynamic
Example of a simple linked list node in Python:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
3. Stacks: The Organized Suitcase
Stacks are like the way you pack your suitcase, placing items on top of each other. They follow the Last-In-First-Out (LIFO) principle, where the last item added is the first one to be removed. This structure is perfect for managing function calls, parsing expressions, or implementing undo functionality.
When to pack stacks:
- For managing function calls and recursion
- When implementing undo/redo features
- For parsing expressions or validating syntax
Example of a stack implementation using JavaScript:
class Stack {
constructor() {
this.items = [];
}
push(element) {
this.items.push(element);
}
pop() {
if (this.isEmpty()) return "Stack is empty";
return this.items.pop();
}
isEmpty() {
return this.items.length === 0;
}
}
4. Queues: The Airport Security Line
Queues are like the airport security line you encounter during your trip. They follow the First-In-First-Out (FIFO) principle, where the first item added is the first one to be removed. Queues are essential for managing tasks, scheduling processes, or implementing breadth-first search algorithms.
When to pack queues:
- For managing tasks or processes in order
- When implementing breadth-first search algorithms
- For handling asynchronous operations
Example of a queue implementation in C++:
#include <queue>
#include <iostream>
int main() {
std::queue<int> myQueue;
myQueue.push(10);
myQueue.push(20);
myQueue.push(30);
std::cout << "Front element: " << myQueue.front() << std::endl;
myQueue.pop();
std::cout << "New front element: " << myQueue.front() << std::endl;
return 0;
}
5. Trees: The Family Tree Photo Album
Trees are like the family tree photo album you might bring on your trip. They represent hierarchical structures with a root node and child nodes. Trees are crucial for organizing data in a hierarchical manner, such as file systems, HTML DOM, or decision trees in machine learning.
When to pack trees:
- For representing hierarchical data structures
- When implementing search algorithms like binary search trees
- For parsing expressions or implementing decision trees
Example of a basic binary tree node in Java:
class TreeNode {
int data;
TreeNode left;
TreeNode right;
public TreeNode(int data) {
this.data = data;
this.left = null;
this.right = null;
}
}
6. Graphs: The Travel Itinerary
Graphs are like your travel itinerary, showing connections between different locations. They consist of vertices (nodes) and edges (connections between nodes). Graphs are essential for representing complex relationships, such as social networks, map routing, or dependency analysis.
When to pack graphs:
- For representing networks or relationships
- When solving problems like shortest path or connectivity
- For modeling real-world scenarios with complex interactions
Example of a simple graph implementation in Python:
class Graph:
def __init__(self):
self.graph = {}
def add_edge(self, u, v):
if u not in self.graph:
self.graph[u] = []
self.graph[u].append(v)
def print_graph(self):
for vertex in self.graph:
print(f"{vertex}: {self.graph[vertex]}")
7. Hash Tables: The Travel Guidebook Index
Hash tables are like the index in your travel guidebook, allowing you to quickly look up information. They provide fast insertion, deletion, and retrieval of data using a key-value pair system. Hash tables are crucial for implementing dictionaries, caches, or symbol tables in compilers.
When to pack hash tables:
- For fast data retrieval based on unique keys
- When implementing caching mechanisms
- For removing duplicates from a dataset
Example of using a hash table (dictionary) in Python:
travel_info = {
"Paris": "Eiffel Tower",
"Rome": "Colosseum",
"London": "Big Ben"
}
print(travel_info["Paris"]) # Output: Eiffel Tower
travel_info["Tokyo"] = "Tokyo Tower" # Adding a new entry
Choosing the Right Data Structure: Factors to Consider
Now that we’ve packed our programming suitcase with essential data structures, let’s discuss how to choose the right one for your specific needs. Just as you consider various factors when packing for a trip, there are key aspects to keep in mind when selecting a data structure:
1. Time Complexity: How Fast Can You Access Your Items?
Time complexity is like considering how quickly you can access items in your luggage. Different data structures have varying time complexities for operations like insertion, deletion, and search. For example:
- Arrays offer O(1) access time for elements by index but O(n) for searching an unsorted array.
- Hash tables provide O(1) average-case time for insertion, deletion, and search operations.
- Binary search trees offer O(log n) time for search, insertion, and deletion in balanced trees.
Consider the most frequent operations in your program and choose a data structure that optimizes those operations.
2. Space Complexity: How Much Can You Fit in Your Suitcase?
Space complexity is akin to the amount of space available in your suitcase. Some data structures require more memory than others:
- Arrays have a fixed size and use contiguous memory, which can be efficient but inflexible.
- Linked lists use more memory due to the additional storage needed for node pointers.
- Hash tables may require extra space for handling collisions and maintaining a low load factor.
Consider the amount of data you need to store and the memory constraints of your system when choosing a data structure.
3. Functionality: What Activities Are You Planning?
The functionality of a data structure is like considering the activities you’ve planned for your trip. Different data structures excel at different tasks:
- If you need to maintain a sorted collection, consider using a binary search tree or a heap.
- For implementing a cache with fast access and expiration, a hash table combined with a linked list (LRU cache) might be ideal.
- If you need to model hierarchical relationships, a tree structure would be most appropriate.
Analyze the specific requirements of your program to determine which data structure best suits your needs.
4. Ease of Implementation: How Skilled Are You at Packing?
The ease of implementation is comparable to your packing skills. Some data structures are simpler to implement and use than others:
- Arrays and linked lists are relatively straightforward to implement and understand.
- More complex structures like balanced trees (e.g., AVL trees or Red-Black trees) require careful implementation to maintain their properties.
- Graph algorithms can be intricate and may require a solid understanding of graph theory.
Consider your skill level and the time available for implementation when choosing a data structure.
Practical Examples: Packing for Different Programming Scenarios
Let’s explore some common programming scenarios and discuss which data structures would be most suitable, just as we would pack differently for various types of trips:
Scenario 1: Building a Contact List Application
For a contact list application, you’ll want fast search, insertion, and deletion operations. Here’s how you might “pack” for this scenario:
- Primary data structure: Hash Table (for fast lookup by name or phone number)
- Secondary structure: Trie (for implementing auto-complete functionality)
- Auxiliary structure: Array or Linked List (for maintaining a sorted view of contacts)
Example of using a hash table for a contact list in Python:
class ContactList:
def __init__(self):
self.contacts = {}
def add_contact(self, name, phone):
self.contacts[name] = phone
def get_phone(self, name):
return self.contacts.get(name, "Contact not found")
def delete_contact(self, name):
if name in self.contacts:
del self.contacts[name]
return True
return False
# Usage
contact_list = ContactList()
contact_list.add_contact("Alice", "123-456-7890")
contact_list.add_contact("Bob", "987-654-3210")
print(contact_list.get_phone("Alice")) # Output: 123-456-7890
contact_list.delete_contact("Bob")
print(contact_list.get_phone("Bob")) # Output: Contact not found
Scenario 2: Implementing a File System
For implementing a file system, you’ll need to represent hierarchical relationships and support efficient navigation. Here’s how you might “pack” for this scenario:
- Primary data structure: Tree (to represent the directory structure)
- Secondary structure: Hash Table (for fast file lookup within directories)
- Auxiliary structure: Stack (for implementing directory traversal)
Example of a simple file system implementation in Java:
import java.util.*;
class FileSystemNode {
String name;
boolean isDirectory;
Map<String, FileSystemNode> children;
public FileSystemNode(String name, boolean isDirectory) {
this.name = name;
this.isDirectory = isDirectory;
this.children = isDirectory ? new HashMap<>() : null;
}
}
class FileSystem {
private FileSystemNode root;
public FileSystem() {
root = new FileSystemNode("/", true);
}
public void mkdir(String path) {
String[] parts = path.split("/");
FileSystemNode current = root;
for (String part : parts) {
if (part.isEmpty()) continue;
if (!current.children.containsKey(part)) {
current.children.put(part, new FileSystemNode(part, true));
}
current = current.children.get(part);
}
}
public void createFile(String path) {
String[] parts = path.split("/");
FileSystemNode current = root;
for (int i = 0; i < parts.length - 1; i++) {
String part = parts[i];
if (part.isEmpty()) continue;
if (!current.children.containsKey(part)) {
throw new IllegalArgumentException("Directory does not exist");
}
current = current.children.get(part);
}
String fileName = parts[parts.length - 1];
current.children.put(fileName, new FileSystemNode(fileName, false));
}
public void ls(String path) {
String[] parts = path.split("/");
FileSystemNode current = root;
for (String part : parts) {
if (part.isEmpty()) continue;
if (!current.children.containsKey(part)) {
throw new IllegalArgumentException("Path does not exist");
}
current = current.children.get(part);
}
if (current.isDirectory) {
for (String child : current.children.keySet()) {
System.out.println(child);
}
} else {
System.out.println(current.name);
}
}
}
// Usage
FileSystem fs = new FileSystem();
fs.mkdir("/home/user");
fs.createFile("/home/user/document.txt");
fs.ls("/home/user"); // Output: document.txt
Scenario 3: Implementing a Social Network
For a social network application, you’ll need to represent complex relationships and support efficient traversal. Here’s how you might “pack” for this scenario:
- Primary data structure: Graph (to represent user connections)
- Secondary structure: Hash Table (for fast user lookup)
- Auxiliary structures: Queue (for breadth-first search of connections) and Stack (for depth-first search)
Example of a simple social network implementation in Python:
from collections import deque
class SocialNetwork:
def __init__(self):
self.users = {}
self.connections = {}
def add_user(self, user_id, name):
self.users[user_id] = name
self.connections[user_id] = set()
def add_connection(self, user1, user2):
if user1 in self.users and user2 in self.users:
self.connections[user1].add(user2)
self.connections[user2].add(user1)
def get_friends(self, user_id):
return [self.users[friend] for friend in self.connections[user_id]]
def find_connection_bfs(self, start_user, end_user):
queue = deque([(start_user, [start_user])])
visited = set()
while queue:
(node, path) = queue.popleft()
if node not in visited:
visited.add(node)
if node == end_user:
return path
for neighbor in self.connections[node]:
if neighbor not in visited:
queue.append((neighbor, path + [neighbor]))
return None
# Usage
social_network = SocialNetwork()
social_network.add_user(1, "Alice")
social_network.add_user(2, "Bob")
social_network.add_user(3, "Charlie")
social_network.add_user(4, "David")
social_network.add_connection(1, 2)
social_network.add_connection(2, 3)
social_network.add_connection(3, 4)
print(social_network.get_friends(2)) # Output: ['Alice', 'Charlie']
print(social_network.find_connection_bfs(1, 4)) # Output: [1, 2, 3, 4]
Conclusion: Mastering the Art of Data Structure Selection
Learning to choose the right data structure is an essential skill for any programmer, much like knowing how to pack efficiently for different types of trips. As you gain experience, you’ll develop an intuition for which data structures best suit various scenarios, allowing you to write more efficient and elegant code.
Remember these key takeaways:
- Understand the characteristics and trade-offs of different data structures.
- Consider time and space complexity when making your selection.
- Analyze the specific requirements of your program and choose accordingly.
- Don’t be afraid to combine multiple data structures to solve complex problems.
- Practice implementing and using various data structures to build your skills.
By mastering the art of selecting and implementing the right data structures, you’ll be well-equipped to tackle a wide range of programming challenges. Just as a well-packed suitcase can make your trip more enjoyable, the right choice of data structures can make your coding journey smoother and more efficient.
So, the next time you embark on a new programming project, take a moment to consider which data structures you’ll need to pack in your coding suitcase. With the right tools at your disposal, you’ll be ready to face any challenge that comes your way in the exciting world of software development.