Why Coders Should Learn Computational Thinking Before Touching a Keyboard
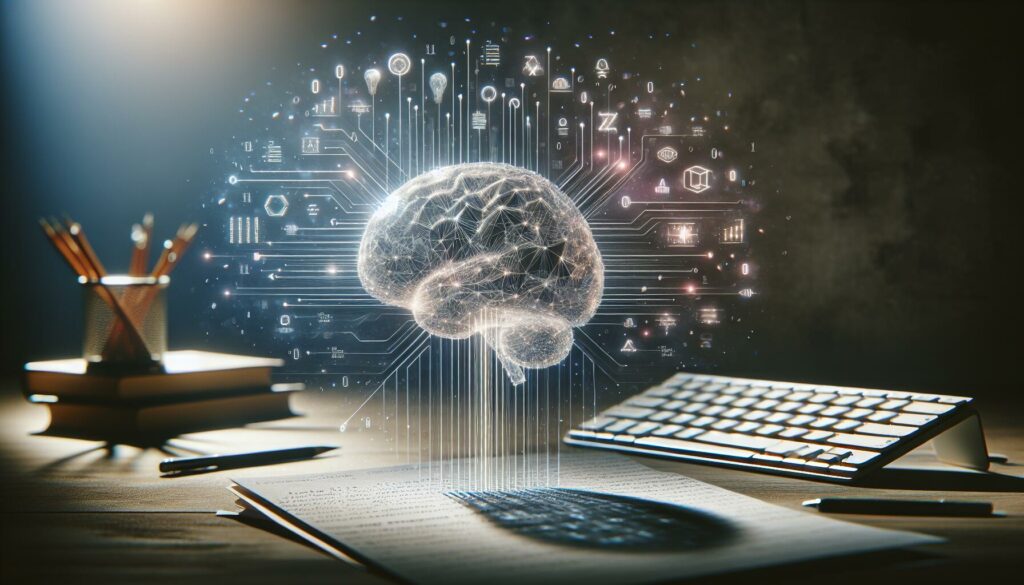
In the fast-paced world of technology, there’s often a rush to dive straight into coding. The allure of creating something tangible, be it a website or an app, can be irresistible. However, before aspiring coders touch a keyboard, there’s a crucial skill they should master: computational thinking. This foundational approach to problem-solving is not just beneficial; it’s essential for anyone serious about excelling in the field of programming. Let’s explore why computational thinking is the bedrock of coding success and how it can transform your approach to software development.
What is Computational Thinking?
Computational thinking is a problem-solving process that involves breaking down complex problems into manageable parts, recognizing patterns, and developing step-by-step solutions. It’s not about thinking like a computer, but rather about formulating problems and their solutions in a way that a computer can effectively execute.
The core components of computational thinking include:
- Decomposition: Breaking down a complex problem into smaller, more manageable parts.
- Pattern Recognition: Identifying similarities or patterns within and among problems.
- Abstraction: Focusing on the important information while ignoring irrelevant details.
- Algorithm Design: Developing a step-by-step solution to the problem.
These skills are not exclusive to computer science; they’re valuable in many areas of life and work. However, they are particularly crucial in programming, where complex problems need to be solved efficiently and logically.
The Benefits of Learning Computational Thinking First
Now that we understand what computational thinking is, let’s delve into why it’s so important to learn before jumping into coding:
1. It Builds a Strong Problem-Solving Foundation
Coding is essentially problem-solving. By learning computational thinking first, you’re training your brain to approach problems in a structured and logical manner. This skill is transferable across all programming languages and paradigms.
For example, when faced with a complex coding task, a developer with strong computational thinking skills will instinctively break down the problem, identify patterns, and create a step-by-step plan before writing a single line of code. This approach leads to more efficient and effective solutions.
2. It Enhances Algorithm Design Skills
Algorithms are at the heart of programming. Computational thinking teaches you how to design algorithms effectively, which is crucial for writing efficient code. By understanding how to create step-by-step solutions, you’ll be better equipped to tackle complex programming challenges.
Consider the classic problem of sorting a list of numbers. A developer with strong computational thinking skills might approach it like this:
- Decompose the problem: Understand that sorting involves comparing and swapping elements.
- Recognize patterns: Notice that the largest number “bubbles up” to the end in each pass.
- Abstract: Focus on the comparison and swapping mechanism, ignoring specific numbers.
- Design the algorithm: Create a step-by-step process for bubble sort.
This approach leads to a clear understanding of the sorting algorithm before any code is written.
3. It Improves Code Quality and Efficiency
When you start with computational thinking, you’re more likely to write clean, efficient, and well-structured code. You’ll have a clear mental model of what your code should do before you start typing, which helps prevent convoluted or inefficient solutions.
For instance, consider a task to find the most frequent element in an array. A programmer without computational thinking might jump straight into coding a nested loop solution, which could be inefficient for large datasets. In contrast, someone who applies computational thinking might realize that using a hash map could provide a more efficient solution, resulting in code like this:
function findMostFrequent(arr) {
const frequencyMap = {};
let maxFreq = 0;
let mostFrequent;
for (const num of arr) {
frequencyMap[num] = (frequencyMap[num] || 0) + 1;
if (frequencyMap[num] > maxFreq) {
maxFreq = frequencyMap[num];
mostFrequent = num;
}
}
return mostFrequent;
}
This solution, derived from computational thinking, is both efficient and elegant.
4. It Facilitates Language-Agnostic Learning
Programming languages come and go, but computational thinking remains constant. By focusing on this skill first, you’re learning principles that apply across all languages and platforms. This makes it easier to adapt to new technologies and programming paradigms in the future.
For example, the concept of recursion in computational thinking can be applied in various languages:
// JavaScript
function factorial(n) {
return n <= 1 ? 1 : n * factorial(n - 1);
}
# Python
def factorial(n):
return 1 if n <= 1 else n * factorial(n - 1)
// Java
public static int factorial(int n) {
return n <= 1 ? 1 : n * factorial(n - 1);
}
The underlying principle remains the same, regardless of the syntax.
5. It Enhances Debugging Skills
Debugging is an essential skill for any programmer. Computational thinking improves your ability to analyze problems systematically, making it easier to identify and fix bugs in your code. By understanding the logical flow and structure of your program, you can more easily pinpoint where things might be going wrong.
For instance, when debugging a recursive function that’s not producing the expected output, a developer with strong computational thinking skills might:
- Break down the function into its base case and recursive case.
- Analyze each part separately to ensure they’re logically correct.
- Trace the function’s execution step-by-step for a small input.
- Identify any discrepancies between the expected and actual behavior.
This systematic approach, rooted in computational thinking, makes debugging more efficient and effective.
How to Develop Computational Thinking Skills
Now that we’ve established the importance of computational thinking, let’s look at how you can develop these skills:
1. Practice Problem Decomposition
Start with complex problems and practice breaking them down into smaller, manageable parts. This could be as simple as planning your day or as complex as designing a new software system. The key is to identify the individual components that make up the larger problem.
For example, if you’re tasked with creating a to-do list application, you might break it down like this:
- User Interface: Design the layout and user interactions
- Data Management: How to store and retrieve tasks
- Task Operations: Add, delete, and mark tasks as complete
- Prioritization: Implement a system for task priority
- Notifications: Set up reminders for upcoming tasks
By breaking down the problem, you make it less overwhelming and more manageable.
2. Engage in Pattern Recognition Exercises
Look for patterns in everyday life and in coding problems. Sudoku puzzles, for instance, are excellent for developing pattern recognition skills. In programming, try to identify common patterns in different algorithms or data structures.
For example, you might notice that many sorting algorithms follow a similar pattern of comparing and swapping elements. Or you might recognize that tree-based data structures often use recursive algorithms for traversal and manipulation.
3. Practice Abstraction
Learn to focus on the essential details while ignoring the irrelevant ones. This skill is crucial in programming, where you often need to model real-world problems in code. Start by describing complex systems in simple terms, focusing only on the most important aspects.
For instance, when modeling a car in code, you might abstract it to:
class Car {
String make;
String model;
int year;
double fuelLevel;
void start() { /* ... */ }
void drive() { /* ... */ }
void refuel() { /* ... */ }
}
This abstraction captures the essential aspects of a car without getting bogged down in unnecessary details.
4. Design Algorithms for Everyday Tasks
Practice creating step-by-step instructions for common tasks, like making a sandwich or navigating to a destination. This helps you think in terms of clear, logical steps – a crucial skill in programming.
For example, an algorithm for making a peanut butter and jelly sandwich might look like this:
- Get two slices of bread
- Open the peanut butter jar
- Spread peanut butter on one slice of bread
- Open the jelly jar
- Spread jelly on the other slice of bread
- Put the two slices together, with the spreads facing inward
- Cut the sandwich diagonally (optional)
This exercise helps you think in terms of clear, sequential steps, which is essential in algorithm design.
5. Solve Puzzles and Brain Teasers
Engage with logic puzzles, riddles, and brain teasers. These challenges exercise your problem-solving skills and encourage you to think creatively and logically – key aspects of computational thinking.
For instance, consider this classic river-crossing puzzle:
A farmer needs to cross a river with a wolf, a goat, and a cabbage. The boat can only carry the farmer and one other item at a time. If left alone, the wolf would eat the goat, and the goat would eat the cabbage. How can the farmer get everything across safely?
Solving this puzzle requires breaking down the problem, recognizing patterns, and developing a step-by-step solution – all key components of computational thinking.
6. Learn Basic Flowcharting
Flowcharts are visual representations of algorithms or processes. Learning to create flowcharts can help you visualize the flow of logic in a program or system. This skill bridges the gap between abstract thinking and concrete implementation.
For example, a simple flowchart for determining if a number is even or odd might look like this:
[Start] → (Input number n) → <Is n % 2 == 0?> → [Yes] → (Print "Even")
↓ ↓
[No] [End]
↓
(Print "Odd")
↓
[End]
This visual representation helps in understanding the logical flow of the algorithm.
Integrating Computational Thinking into Coding Practice
Once you’ve developed a strong foundation in computational thinking, it’s time to apply these skills to your coding practice. Here are some strategies to integrate computational thinking into your programming workflow:
1. Plan Before You Code
Before writing any code, take the time to plan your approach. Use the decomposition and abstraction skills you’ve developed to break down the problem and identify the key components of your solution. Sketch out a high-level design or create pseudocode to outline your algorithm.
For example, if you’re building a function to calculate the Fibonacci sequence, your planning might look like this:
Function: fibonacci(n)
Input: Integer n (the position in the sequence)
Output: The nth Fibonacci number
Algorithm:
1. If n is 0 or 1, return n
2. Initialize variables for the two previous numbers
3. Iterate from 2 to n:
a. Calculate the new number by adding the two previous numbers
b. Update the previous numbers
4. Return the final calculated number
This planning stage helps you clarify your thoughts and catch potential issues before you start coding.
2. Implement Incrementally
Use the decomposition skills you’ve learned to implement your solution in small, manageable increments. This approach allows you to test and verify each part of your solution as you go, making it easier to identify and fix issues.
For instance, when implementing the Fibonacci function, you might:
- Start with the base cases (n = 0 and n = 1)
- Implement the iteration logic
- Add the calculation for each new number
- Implement the variable updates
Testing each step ensures that your implementation is correct before moving on to the next part.
3. Look for Patterns and Optimizations
As you code, use your pattern recognition skills to identify common structures or repeated logic. This can help you optimize your code and potentially refactor it into more reusable components.
For example, you might notice that many of your functions follow a similar error-checking pattern:
function doSomething(param) {
if (param === null || param === undefined) {
throw new Error("Invalid parameter");
}
// Rest of the function logic
}
Recognizing this pattern could lead you to create a utility function for parameter validation, improving code reusability and readability.
4. Use Abstraction in Your Code Design
Apply the abstraction skills you’ve developed to create clean, modular code. Encapsulate related functionality into classes or modules, and use interfaces to define clear contracts between different parts of your system.
For instance, if you’re building a game, you might create abstract classes for different types of game objects:
abstract class GameObject {
protected int x;
protected int y;
public abstract void update();
public abstract void render();
}
class Player extends GameObject {
public void update() { /* Player-specific update logic */ }
public void render() { /* Player-specific render logic */ }
}
class Enemy extends GameObject {
public void update() { /* Enemy-specific update logic */ }
public void render() { /* Enemy-specific render logic */ }
}
This abstraction allows you to treat different game objects uniformly while allowing for specific implementations.
5. Document Your Thought Process
As you code, document your thought process and the reasoning behind your decisions. This not only helps others understand your code but also reinforces your own computational thinking skills.
For example, when implementing a complex algorithm, you might add comments like this:
// Using a hash map for O(1) lookup time
const frequencyMap = {};
// Iterate through the array once, O(n) time complexity
for (const num of arr) {
// Update frequency count
frequencyMap[num] = (frequencyMap[num] || 0) + 1;
// Keep track of the most frequent element
if (frequencyMap[num] > maxFreq) {
maxFreq = frequencyMap[num];
mostFrequent = num;
}
}
These comments explain the reasoning behind the chosen approach and demonstrate computational thinking in action.
The Long-Term Benefits of Computational Thinking for Coders
Investing time in developing computational thinking skills before diving into coding pays dividends throughout your programming career. Here are some of the long-term benefits:
1. Improved Problem-Solving Abilities
Computational thinking enhances your overall problem-solving skills, making you more effective not just in coding, but in tackling complex challenges in any domain. You’ll find yourself approaching problems more systematically and creatively.
2. Enhanced Adaptability
As technology evolves rapidly, the ability to adapt to new languages, frameworks, and paradigms is crucial. Computational thinking provides a solid foundation that makes it easier to learn and adapt to new technologies throughout your career.
3. Better Communication with Non-Technical Stakeholders
The abstraction and decomposition skills you develop through computational thinking can help you communicate complex technical concepts to non-technical stakeholders more effectively. This is invaluable in roles that require collaboration with diverse teams.
4. Increased Efficiency in Coding
With strong computational thinking skills, you’ll likely find yourself writing more efficient code from the start. This can save time in the long run by reducing the need for extensive refactoring and optimization later in the development process.
5. Improved Debugging and Troubleshooting
The systematic approach fostered by computational thinking makes you more adept at identifying and fixing issues in your code. You’ll be able to approach debugging more methodically, potentially reducing the time spent on troubleshooting.
6. Enhanced Ability to Learn New Programming Concepts
As you encounter new programming concepts or paradigms, your foundation in computational thinking will help you grasp these ideas more quickly. You’ll be able to see how these new concepts relate to the fundamental principles of problem-solving and algorithm design.
Conclusion
In the exciting world of coding, it’s tempting to jump straight into writing code. However, taking the time to develop strong computational thinking skills before touching a keyboard can set you up for long-term success as a programmer. By mastering decomposition, pattern recognition, abstraction, and algorithm design, you’ll be better equipped to tackle complex coding challenges, write efficient and maintainable code, and adapt to new technologies throughout your career.
Remember, computational thinking is not just a skill for computer scientists – it’s a valuable approach to problem-solving that can benefit you in many areas of life. As you embark on your coding journey, invest time in developing these foundational skills. They will serve as a strong foundation upon which you can build your programming expertise, helping you become not just a coder, but a truly effective problem solver in the digital age.
So, before you dive into your next coding project, take a step back. Analyze the problem, break it down, look for patterns, and design your solution. Your future self – and your code – will thank you for it.