Why Learning the Fundamentals of Algorithms is More Important Than Knowing Frameworks
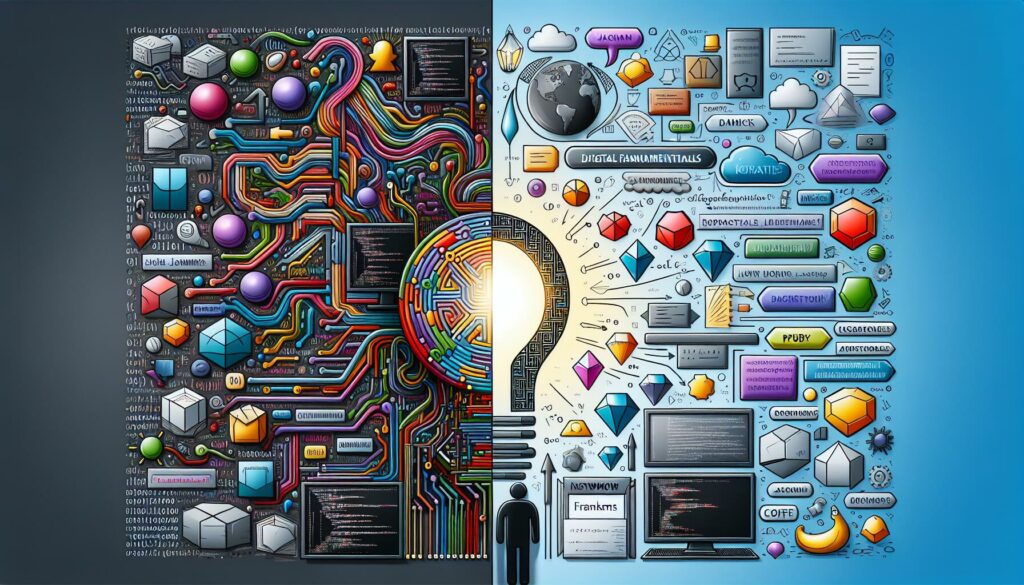
In the ever-evolving world of software development, there’s a constant debate about what skills are most crucial for programmers to master. While frameworks come and go, one aspect of programming remains timeless and invaluable: the fundamentals of algorithms. This article will explore why investing time in learning algorithmic thinking and problem-solving skills is more beneficial than solely focusing on the latest frameworks.
The Ephemeral Nature of Frameworks
Frameworks are undoubtedly useful tools in a developer’s arsenal. They provide pre-built components, streamline development processes, and can significantly speed up project completion. However, frameworks have a relatively short lifespan in the fast-paced tech industry.
Consider these facts:
- New frameworks emerge regularly, often replacing or overshadowing existing ones.
- Popular frameworks undergo frequent updates and changes, requiring developers to constantly adapt.
- What’s trendy today may become obsolete within a few years.
This rapid turnover means that investing too heavily in mastering a specific framework can lead to skills becoming outdated quickly. While it’s important to be familiar with current tools, relying solely on framework knowledge can be a risky career strategy.
The Timeless Value of Algorithmic Thinking
In contrast to the fleeting nature of frameworks, the fundamentals of algorithms and data structures have remained relevant since the inception of computer science. These core concepts form the bedrock of efficient and effective programming, regardless of the language or framework being used.
Here’s why algorithmic thinking is so valuable:
- Problem-Solving Skills: Algorithms teach you how to break down complex problems into manageable steps, a skill applicable across all areas of programming and beyond.
- Efficiency: Understanding algorithms helps you write more efficient code, optimizing performance and resource usage.
- Scalability: Algorithmic knowledge is crucial for building systems that can handle large amounts of data and users.
- Language Agnostic: Unlike framework-specific knowledge, algorithmic concepts can be applied across different programming languages.
- Foundation for Learning: A strong grasp of algorithms makes it easier to learn new languages and frameworks quickly.
Real-World Applications of Algorithmic Knowledge
To illustrate the practical importance of algorithmic thinking, let’s consider some real-world scenarios where this knowledge is crucial:
1. Optimizing Database Queries
Imagine you’re working on a social media application that needs to display a user’s feed. With millions of users and posts, an inefficient algorithm could lead to slow load times and a poor user experience. Knowledge of sorting and filtering algorithms can help you optimize these queries, ensuring the app remains responsive even as it scales.
2. Recommendation Systems
Companies like Netflix and Amazon rely heavily on recommendation algorithms to enhance user experience and drive sales. Understanding concepts like collaborative filtering and content-based filtering is essential for building effective recommendation systems.
3. Route Planning in Maps
Navigation apps use complex pathfinding algorithms to determine the best route between two points. Familiarity with algorithms like Dijkstra’s or A* is crucial for developing efficient route planning systems.
4. Data Compression
In an era of big data, efficient data storage and transmission are vital. Compression algorithms play a key role in reducing file sizes without losing important information. Whether you’re working on image processing or data storage solutions, understanding these algorithms is invaluable.
The Role of Algorithms in Technical Interviews
For those aspiring to work at major tech companies, often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google), a strong foundation in algorithms is not just beneficial—it’s essential. These companies are known for their rigorous technical interviews, which often focus heavily on algorithmic problem-solving.
Here’s why algorithms are crucial in these interviews:
- Problem-Solving Under Pressure: Interviewers want to see how you approach and solve complex problems in real-time.
- Efficiency Matters: It’s not just about finding a solution, but finding the most efficient one.
- Scalability Concerns: These companies deal with massive amounts of data, so they need developers who can write scalable code.
- Foundational Knowledge: Understanding of core algorithms demonstrates a deep knowledge of computer science principles.
Let’s look at a common interview question and how algorithmic knowledge can help:
Example: Two Sum Problem
Given an array of integers and a target sum, return indices of the two numbers such that they add up to the target.
A naive approach might use nested loops, checking every pair of numbers:
def two_sum_naive(nums, target):
for i in range(len(nums)):
for j in range(i + 1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return []
This solution works but has a time complexity of O(n^2), which is inefficient for large inputs.
With knowledge of hash tables, we can optimize this to O(n):
def two_sum_optimized(nums, target):
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return []
This optimized solution demonstrates how algorithmic knowledge can lead to more efficient solutions, a key factor in technical interviews.
Balancing Algorithms and Frameworks
While this article emphasizes the importance of algorithms, it’s not to say that frameworks are unimportant. In practice, a balanced approach is often the most beneficial:
- Start with Fundamentals: Build a strong foundation in algorithms and data structures.
- Learn Core Languages: Master one or two programming languages deeply.
- Explore Frameworks: Once you have a solid foundation, start learning frameworks relevant to your field.
- Continuous Learning: Keep updating your knowledge of both algorithms and current technologies.
How to Approach Learning Algorithms
Learning algorithms can seem daunting, but with the right approach, it can be an engaging and rewarding process:
- Start with the Basics: Begin with fundamental data structures (arrays, linked lists, trees, graphs) and basic algorithms (sorting, searching).
- Practice Regularly: Solve algorithmic problems on platforms like LeetCode, HackerRank, or AlgoCademy.
- Understand Time and Space Complexity: Learn to analyze the efficiency of your solutions.
- Implement from Scratch: Try implementing common data structures and algorithms yourself to deepen your understanding.
- Study Classic Algorithms: Familiarize yourself with well-known algorithms like Dijkstra’s, Dynamic Programming, and Divide and Conquer strategies.
- Read and Watch: Utilize books, online courses, and video tutorials to gain different perspectives on algorithmic concepts.
The Long-Term Benefits of Algorithmic Thinking
Investing time in learning algorithms pays off in numerous ways throughout a developer’s career:
- Career Longevity: While specific technologies may become obsolete, algorithmic skills remain valuable.
- Versatility: The ability to solve complex problems makes you adaptable to different roles and industries.
- Innovation: A deep understanding of algorithms can lead to creative solutions and novel approaches to problems.
- Leadership Opportunities: Strong problem-solving skills are crucial for technical leadership roles.
- Continuous Growth: The field of algorithms is vast, offering endless opportunities for learning and improvement.
Real-World Success Stories
To further illustrate the importance of algorithmic thinking, let’s look at some real-world success stories:
1. Google’s PageRank Algorithm
Google’s success is largely attributed to its PageRank algorithm, which revolutionized web search. This algorithm, based on graph theory and probability, demonstrates how fundamental algorithmic knowledge can lead to groundbreaking innovations.
2. Facebook’s News Feed Algorithm
Facebook’s ability to keep users engaged is largely due to its News Feed algorithm. This complex system, which determines what content to show each user, relies heavily on machine learning algorithms and data analysis techniques.
3. Uber’s Routing Algorithm
Uber’s success depends on efficiently matching drivers with riders and optimizing routes. Their routing algorithm, which considers factors like traffic, time of day, and user preferences, is a prime example of applied algorithmic thinking in solving real-world problems.
Overcoming the Challenges of Learning Algorithms
While the benefits of learning algorithms are clear, many developers find this area challenging. Here are some common obstacles and how to overcome them:
1. Perceived Difficulty
Challenge: Algorithms can seem abstract and complex at first.
Solution: Start with simpler problems and gradually increase difficulty. Use visualizations and real-world analogies to make concepts more tangible.
2. Time Investment
Challenge: Learning algorithms requires a significant time commitment.
Solution: Set aside regular, dedicated time for study. Even 30 minutes a day can lead to significant progress over time.
3. Lack of Immediate Application
Challenge: The benefits of algorithmic knowledge may not be immediately apparent in day-to-day coding tasks.
Solution: Look for opportunities to apply algorithmic thinking in your current projects. Even small optimizations can make a difference.
4. Overwhelm from Information Overload
Challenge: The field of algorithms is vast, which can be overwhelming.
Solution: Focus on mastering core concepts first. Create a structured learning plan and tackle one topic at a time.
Integrating Algorithmic Thinking into Daily Coding Practices
To truly benefit from algorithmic knowledge, it’s important to integrate this thinking into your daily coding practices:
- Code Reviews: When reviewing code (yours or others’), consider the algorithmic efficiency of the solutions.
- Optimization Challenges: Regularly challenge yourself to optimize existing code, even if it’s already working.
- Problem Decomposition: Practice breaking down complex tasks into smaller, algorithmic steps before starting to code.
- Performance Analysis: Get into the habit of analyzing the time and space complexity of your code.
- Algorithmic Discussions: Engage in discussions about algorithms with colleagues or in online communities.
The Future of Algorithms in Software Development
As we look to the future of software development, the importance of algorithmic thinking is only set to increase:
- Artificial Intelligence and Machine Learning: These fields rely heavily on advanced algorithms, and their importance in software development is growing rapidly.
- Big Data: As data volumes continue to explode, efficient algorithms for processing and analyzing this data become increasingly crucial.
- Internet of Things (IoT): With billions of connected devices, optimizing data flow and processing through smart algorithms is essential.
- Quantum Computing: As quantum computing evolves, it will bring new algorithmic challenges and opportunities.
Conclusion
While frameworks and technologies will continue to evolve and change, the fundamentals of algorithms remain a constant in the world of software development. By investing time in learning and mastering algorithmic thinking, developers equip themselves with a timeless skill set that enhances problem-solving abilities, improves code efficiency, and opens doors to exciting career opportunities.
Remember, the goal isn’t to memorize every algorithm but to develop a problem-solving mindset that can be applied to any coding challenge. Whether you’re a beginner just starting your coding journey or an experienced developer looking to enhance your skills, focusing on algorithmic fundamentals will always be a wise investment in your career.
As you continue your learning journey, platforms like AlgoCademy can be invaluable resources, offering interactive tutorials, AI-powered assistance, and a structured approach to mastering algorithms and data structures. Embrace the challenge of algorithmic thinking, and watch as it transforms not just your code, but your entire approach to software development.