The Art of Code Reading: How Reading Code Can Make You a Better Programmer
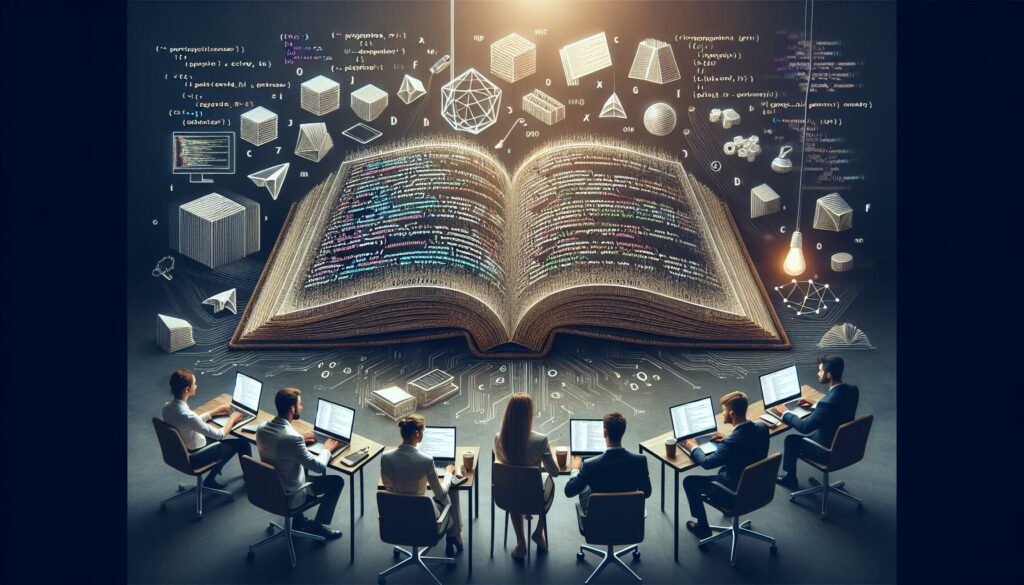
In the world of programming, writing code is often considered the primary skill. However, an equally important but often overlooked ability is reading code. As you progress in your coding journey, you’ll find that the ability to effectively read and understand code written by others is crucial for your growth as a programmer. This skill is particularly valuable when preparing for technical interviews at major tech companies, often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google).
In this comprehensive guide, we’ll explore the art of code reading, its importance in your programming career, and how it can significantly enhance your coding skills. We’ll also provide practical tips and strategies to improve your code reading abilities, making you a more well-rounded and efficient programmer.
Why is Code Reading Important?
Before we dive into the techniques of effective code reading, let’s understand why this skill is so crucial:
- Understanding Existing Codebases: In most professional settings, you’ll be working with existing codebases. The ability to quickly understand and navigate through this code is essential for productivity.
- Learning New Concepts: Reading code written by experienced developers exposes you to new programming concepts, design patterns, and best practices.
- Debugging Skills: Effective code reading is fundamental to debugging. It helps you trace issues and understand the flow of the program.
- Code Review: As you advance in your career, you’ll often be required to review code written by your peers. Strong code reading skills are crucial for providing meaningful feedback.
- Interview Preparation: Many technical interviews, especially at FAANG companies, involve reading and explaining code snippets or improving existing code.
The Process of Effective Code Reading
Reading code is not just about going through lines of text; it’s about understanding the logic, flow, and intent behind the code. Here’s a step-by-step process to approach code reading effectively:
1. Understand the Context
Before diving into the code, try to understand its context:
- What is the purpose of this code?
- What problem is it solving?
- What are the inputs and expected outputs?
This context will give you a framework to understand the code’s structure and logic.
2. Scan the Overall Structure
Start with a high-level overview of the code:
- Look at the file structure and organization
- Identify main functions or classes
- Note any import statements or dependencies
This step helps you understand the code’s architecture and how different parts interact.
3. Focus on the Main Logic
Once you have a general understanding, focus on the core logic:
- Identify the entry point of the program
- Follow the main execution path
- Pay attention to control structures (loops, conditionals)
This step helps you understand how the code achieves its purpose.
4. Dive into Details
Now, start examining the code in more detail:
- Look at individual functions and methods
- Understand data structures being used
- Examine variable names and their purposes
This detailed examination helps you understand the nuances of the implementation.
5. Make Notes and Diagrams
As you read, it’s helpful to:
- Take notes on key points
- Draw diagrams to visualize complex logic or data flow
- Write questions about parts you don’t understand
This active engagement with the code enhances your understanding and retention.
Strategies to Improve Your Code Reading Skills
Now that we understand the process, let’s look at some strategies to enhance your code reading abilities:
1. Read Code Regularly
Like any skill, code reading improves with practice. Make it a habit to read code regularly:
- Explore open-source projects on platforms like GitHub
- Read code written by experienced developers in your team
- Study implementations of standard algorithms and data structures
2. Use IDE Features
Modern Integrated Development Environments (IDEs) offer features that can aid in code reading:
- Use code navigation features to jump between definitions and references
- Utilize code folding to hide irrelevant details
- Use breakpoints and step-through debugging to understand code flow
3. Read Documentation and Comments
Good code often comes with documentation and comments. These can provide valuable insights:
- Start with README files and other documentation
- Pay attention to inline comments and docstrings
- Look for design documents or architectural overviews
4. Practice Code Explanation
Try explaining the code you’re reading to someone else (or even to yourself):
- Summarize what a function or class does
- Explain the overall architecture of the project
- Discuss the pros and cons of the implementation
This practice of verbalization helps solidify your understanding and identifies areas where your comprehension might be lacking.
5. Learn Common Design Patterns
Familiarize yourself with common design patterns and architectural styles:
- Study classic design patterns (e.g., Singleton, Factory, Observer)
- Learn about architectural patterns (e.g., MVC, Microservices)
- Understand language-specific idioms and best practices
Recognizing these patterns in code can greatly speed up your understanding of complex systems.
Advanced Code Reading Techniques
As you become more proficient, you can employ more advanced techniques:
1. Static Analysis
Use static analysis tools to gain insights into the code:
- Code complexity metrics
- Dependency analysis
- Code style and potential bug detection
These tools can highlight areas that might need closer examination.
2. Dynamic Analysis
Use runtime analysis to understand the code’s behavior:
- Profiling to identify performance bottlenecks
- Memory analysis to detect leaks or inefficient usage
- Code coverage to see which parts of the code are actually used
3. Comparative Reading
Compare different implementations of the same functionality:
- Look at how different languages solve the same problem
- Compare your implementation with a standard library implementation
- Examine different versions of the same codebase to understand evolution
Code Reading in Technical Interviews
Code reading is a crucial skill in technical interviews, especially at top tech companies. Here’s how it might be applied:
1. Code Explanation Questions
You might be asked to explain a piece of code:
def mystery_function(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
In this case, you’d need to recognize that this is an implementation of the bubble sort algorithm and explain its functionality and time complexity.
2. Code Improvement Questions
You might be given a piece of code and asked to improve it:
def find_duplicate(arr):
for i in range(len(arr)):
for j in range(i+1, len(arr)):
if arr[i] == arr[j]:
return arr[i]
return None
Here, you’d need to recognize that this is an inefficient way to find a duplicate in an array (O(n^2) time complexity) and suggest a more efficient solution, perhaps using a hash set for O(n) time complexity.
3. Debugging Questions
You might be presented with a buggy piece of code and asked to identify and fix the bug:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left < right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
In this case, you’d need to spot that the condition while left < right
should be while left <= right
to handle the case where the target is at the last element of the array.
Tools and Resources for Improving Code Reading Skills
To further enhance your code reading abilities, consider using these tools and resources:
1. Code Visualization Tools
- PythonTutor: Visualizes code execution step-by-step, great for understanding control flow.
- Sourcetrail: An open-source tool for exploring codebases visually.
2. Online Platforms
- LeetCode: Offers a wide range of coding problems with solution discussions.
- HackerRank: Provides coding challenges and competitions to practice both writing and reading code.
- CodeSignal: Offers coding assessments and interview practice problems.
3. Books
- “Clean Code” by Robert C. Martin: Teaches principles of writing readable code, which in turn helps in reading code.
- “Code Reading: The Open Source Perspective” by Diomidis Spinellis: Specifically focused on the skill of reading and understanding code.
4. Open Source Projects
Engaging with open source projects is an excellent way to practice code reading:
- Start with well-documented projects in your preferred language
- Try to understand the codebase enough to make small contributions
- Participate in code reviews to see how other developers read and critique code
Conclusion
The art of code reading is a fundamental skill for any programmer, especially those aiming for positions at top tech companies. It enhances your ability to understand complex systems, debug efficiently, and write better code yourself. By regularly practicing code reading, utilizing the right tools, and applying the strategies discussed in this article, you can significantly improve your programming skills and prepare yourself for success in technical interviews and your programming career.
Remember, becoming proficient at code reading is a gradual process. Start with simple codebases and progressively challenge yourself with more complex projects. As you improve, you’ll find that your overall programming skills, including problem-solving and algorithmic thinking, will also enhance significantly.
Happy coding, and may your journey in mastering the art of code reading be both enlightening and rewarding!