How to Fall in Love with Debugging and Make It Your Superpower
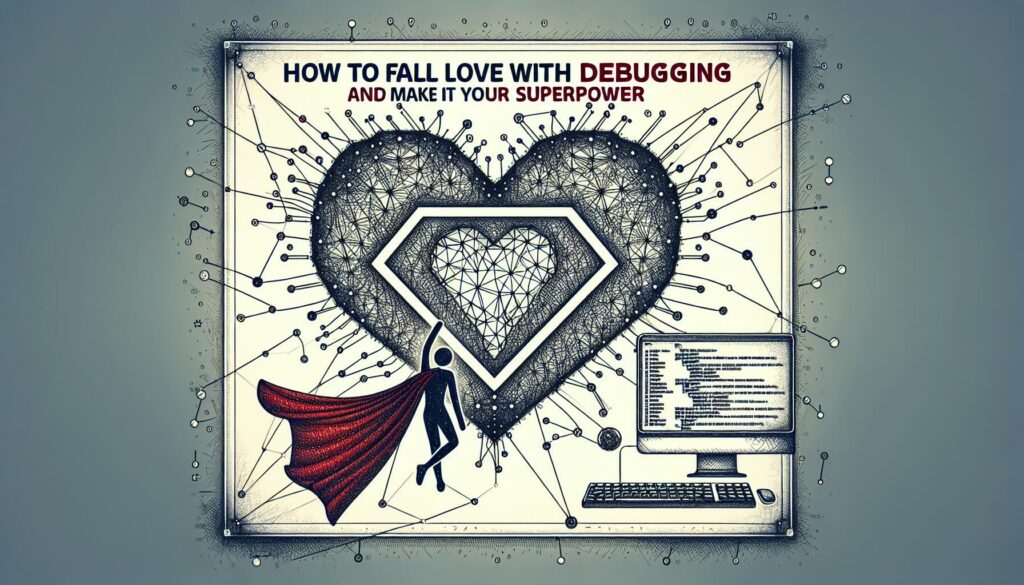
Debugging is often seen as a tedious and frustrating part of programming. Many developers view it as a necessary evil, something to be endured rather than enjoyed. But what if we told you that debugging could be not just bearable, but actually enjoyable? Even more, what if debugging could become your superpower, setting you apart as an exceptional programmer? In this comprehensive guide, we’ll explore how to change your perspective on debugging, embrace its challenges, and ultimately fall in love with the process.
Understanding the Importance of Debugging
Before we dive into the techniques and mindset shifts that can make debugging more enjoyable, let’s first understand why debugging is so crucial in the world of programming.
The Ubiquity of Bugs
Bugs are an inevitable part of software development. No matter how experienced or careful a programmer is, errors will occur. These can range from simple typos to complex logical errors that only manifest under specific conditions. Recognizing that bugs are a normal part of the development process is the first step in changing your relationship with debugging.
Debugging as a Learning Opportunity
Each bug you encounter is an opportunity to learn something new. Whether it’s a deeper understanding of the programming language you’re using, insights into system architecture, or a chance to improve your problem-solving skills, debugging is a constant teacher in the world of coding.
The Impact of Effective Debugging
Efficient debugging can significantly impact the overall quality and reliability of your code. It’s not just about fixing errors; it’s about understanding why they occurred and how to prevent similar issues in the future. This proactive approach can lead to more robust and maintainable software.
Changing Your Mindset: From Chore to Challenge
The first step in falling in love with debugging is to shift your perspective. Instead of viewing it as a tedious task, try to see it as an exciting challenge or puzzle to solve.
Embrace the Detective Mindset
Think of yourself as a detective solving a mystery. Each bug is a case to crack, and you’re the brilliant investigator piecing together the clues. This mindset can make the debugging process feel more like an engaging game than a chore.
Celebrate Small Victories
Every step towards solving a bug, no matter how small, is progress. Celebrate these small victories. Did you narrow down the problem area? Great! Did you eliminate a possible cause? Excellent! These small wins can keep you motivated throughout the debugging process.
View Bugs as Opportunities for Growth
Each bug you encounter and solve makes you a better programmer. It’s an opportunity to deepen your understanding of the code, the system, and programming concepts in general. Embrace these opportunities for growth and learning.
Developing a Systematic Approach to Debugging
Having a structured approach to debugging can make the process more efficient and less frustrating. Here’s a step-by-step method you can follow:
1. Reproduce the Bug
The first step in solving any bug is to consistently reproduce it. This helps you understand the conditions under which the bug occurs and provides a way to verify when you’ve successfully fixed it.
2. Isolate the Problem
Try to narrow down the area of the code where the bug is occurring. This might involve commenting out sections of code or using debugging tools to trace the execution path.
3. Form a Hypothesis
Based on the information you’ve gathered, form a hypothesis about what might be causing the bug. This is where your problem-solving skills and creativity come into play.
4. Test Your Hypothesis
Implement a potential fix based on your hypothesis and test it. If it doesn’t work, use the information you’ve gained to form a new hypothesis.
5. Fix and Verify
Once you’ve found a solution that works, implement it and verify that it solves the problem without introducing new issues.
6. Document and Learn
After fixing the bug, take some time to document what you learned. This can be invaluable for future debugging sessions and for improving your overall coding skills.
Leveraging Debugging Tools and Techniques
Mastering debugging tools can significantly enhance your debugging efficiency and make the process more enjoyable. Here are some essential tools and techniques to consider:
Integrated Development Environment (IDE) Debuggers
Most modern IDEs come with powerful built-in debuggers. These tools allow you to set breakpoints, step through code line by line, and inspect variables at runtime. Familiarize yourself with your IDE’s debugging features to streamline your debugging process.
Logging and Print Statements
While sometimes considered a primitive debugging technique, strategic use of logging or print statements can be incredibly effective. They allow you to track the flow of your program and the state of variables at different points in execution.
Version Control Systems
Tools like Git can be invaluable for debugging. They allow you to track changes over time, revert to previous working versions, and create separate branches for testing potential fixes without affecting the main codebase.
Browser Developer Tools
For web development, browser developer tools are essential. They provide features for inspecting HTML, CSS, and JavaScript, as well as network activity and performance metrics.
Specialized Debugging Tools
Depending on your programming language and environment, there may be specialized debugging tools available. For example, Python has pdb, JavaScript has Chrome DevTools, and Java has JConsole.
Advanced Debugging Techniques
As you become more comfortable with basic debugging, you can start exploring more advanced techniques to further enhance your skills:
Rubber Duck Debugging
This technique involves explaining your code and the problem you’re facing to an inanimate object (traditionally a rubber duck). The act of articulating the problem often leads to insights and solutions.
Binary Search Debugging
For bugs in large codebases, you can use a binary search approach. Comment out half of the code and see if the bug persists. Keep dividing the problematic section in half until you isolate the bug.
Time Travel Debugging
Some advanced debugging tools allow you to record the execution of your program and then “travel back in time” to examine the state at different points. This can be particularly useful for intermittent bugs.
Debugging by Induction
Start with a small, working piece of code and gradually add complexity, testing at each step. This can help you pinpoint exactly where and why a bug is introduced.
Cultivating Debugging-Friendly Coding Practices
Adopting certain coding practices can make your code easier to debug and maintain:
Write Clean, Readable Code
Clean, well-organized code is easier to debug. Use meaningful variable names, keep functions small and focused, and follow consistent formatting practices.
Implement Error Handling
Proper error handling can provide valuable information when bugs occur. Use try-catch blocks and custom error messages to make debugging easier.
Write Unit Tests
Unit tests can catch bugs early and make it easier to isolate problems. They also serve as documentation for how your code should behave.
Use Assertions
Assertions can help catch bugs early by validating assumptions in your code. They’re especially useful for catching edge cases and unexpected inputs.
The Psychology of Effective Debugging
Debugging isn’t just about technical skills; it also involves psychological factors. Understanding and managing these can make debugging more enjoyable and effective:
Manage Frustration
Debugging can be frustrating, especially when dealing with persistent or elusive bugs. Learn to recognize when you’re getting frustrated and take breaks when needed. A fresh perspective can often lead to breakthroughs.
Practice Patience
Some bugs take time to solve. Cultivate patience and persistence. Remember that even experienced programmers sometimes spend hours or days tracking down particularly tricky bugs.
Develop Curiosity
Approach bugs with curiosity rather than dread. Wonder about why the bug is occurring and what it might reveal about your code or system.
Build Confidence
Each bug you successfully debug builds your confidence and skills. Reflect on your successes and use them to bolster your belief in your ability to solve future problems.
Learning from Others: Debugging in a Team Environment
Debugging doesn’t have to be a solitary activity. Learning to debug effectively in a team environment can enhance your skills and make the process more enjoyable:
Pair Debugging
Working with a colleague to debug can provide fresh perspectives and insights. It’s also an opportunity to learn new techniques and approaches.
Code Reviews
Participating in code reviews, both as a reviewer and having your code reviewed, can help catch bugs early and improve your overall coding and debugging skills.
Share Your Debugging Stories
Discussing challenging bugs you’ve solved with your team can be both educational and motivating. It’s a chance to learn from others’ experiences and share your own insights.
Contribute to Open Source
Participating in open source projects can expose you to a wide variety of codebases and debugging challenges, accelerating your learning and skill development.
Continuous Improvement: Enhancing Your Debugging Skills
To truly make debugging your superpower, it’s important to continuously work on improving your skills:
Study Common Bug Patterns
Familiarize yourself with common types of bugs in your programming language or domain. This knowledge can help you spot and fix issues more quickly.
Read Post-Mortems
Many tech companies publish post-mortems of significant bugs or outages. Reading these can provide valuable insights into complex debugging scenarios.
Practice Deliberately
Set aside time to practice debugging skills. This could involve working on coding challenges, contributing to open source projects, or even intentionally introducing bugs into your own code to practice finding and fixing them.
Stay Updated
Keep up with new debugging tools and techniques in your field. The world of software development is constantly evolving, and new tools can significantly enhance your debugging capabilities.
Conclusion: Embracing Debugging as Your Superpower
Debugging is an essential skill for any programmer, but it doesn’t have to be a dreaded task. By changing your mindset, developing a systematic approach, mastering debugging tools, and continuously improving your skills, you can transform debugging from a necessary evil into a genuine superpower.
Remember, every bug you encounter is an opportunity to learn and grow as a programmer. Embrace these challenges, celebrate your successes, and don’t be afraid to dive deep into the intricacies of your code. With practice and persistence, you’ll find yourself not just tolerating debugging, but actually looking forward to it as an exciting and rewarding part of your programming journey.
So the next time you encounter a bug, smile. You’re not just fixing an error; you’re honing your superpower and becoming a better, more capable programmer with every line of code you debug.