From Hello World to Advanced Projects: How to Map Out Your Learning Journey
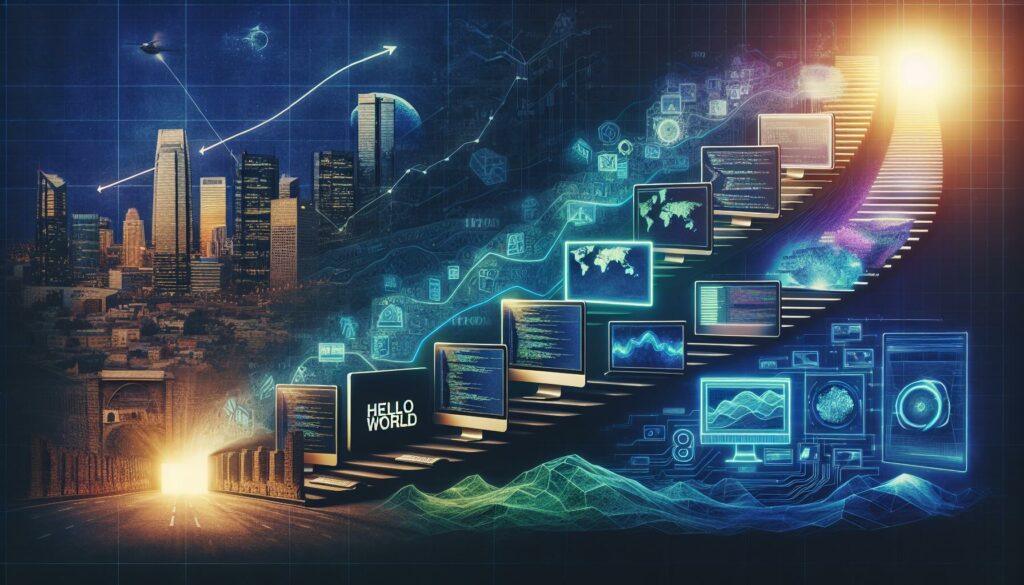
Embarking on a coding journey can feel like setting sail on a vast ocean of knowledge. Whether you’re a complete novice or looking to level up your skills, having a clear roadmap can make all the difference. In this comprehensive guide, we’ll explore how to chart your course from writing your first “Hello World” program to tackling advanced projects that could land you a job at a top tech company. Let’s dive into the world of programming and discover how to make your learning journey both effective and enjoyable.
1. Understanding the Basics: Your First Steps
Every coding journey begins with the basics. This foundation is crucial, regardless of which programming language you choose to start with.
1.1 Choose Your First Programming Language
Selecting your first programming language is an important decision. While there’s no universally “best” language to start with, some popular choices for beginners include:
- Python: Known for its readability and versatility
- JavaScript: Essential for web development
- Java: Widely used in enterprise environments
- C++: Powerful for system-level programming
For this guide, let’s use Python as our example language due to its beginner-friendly syntax and wide application range.
1.2 Set Up Your Development Environment
Before you start coding, you need to set up your development environment. For Python, this means:
- Installing Python from the official website
- Setting up a code editor (like Visual Studio Code, PyCharm, or even a simple text editor)
- Learning how to run Python scripts from the command line
1.3 Write Your First “Hello World” Program
The traditional first program in any language is the “Hello World” script. In Python, it looks like this:
print("Hello, World!")
This simple line introduces you to the print function and string literals in Python.
1.4 Understand Basic Programming Concepts
As you move beyond “Hello World,” focus on understanding these fundamental concepts:
- Variables and data types
- Control structures (if statements, loops)
- Functions and modules
- Basic input/output operations
Practice these concepts by writing small programs, such as a calculator or a simple game like “Guess the Number.”
2. Building a Strong Foundation: Intermediate Skills
Once you’re comfortable with the basics, it’s time to deepen your understanding and broaden your skill set.
2.1 Dive into Object-Oriented Programming (OOP)
OOP is a fundamental paradigm in modern programming. Key concepts to master include:
- Classes and objects
- Inheritance and polymorphism
- Encapsulation and abstraction
Try creating a simple project using OOP principles, such as a basic library management system or a pet shop inventory.
2.2 Learn About Data Structures and Algorithms
Understanding data structures and algorithms is crucial for writing efficient code and solving complex problems. Focus on:
- Arrays and linked lists
- Stacks and queues
- Trees and graphs
- Sorting and searching algorithms
Implement these data structures in your chosen language and practice solving algorithmic problems on platforms like LeetCode or HackerRank.
2.3 Explore File Handling and Database Basics
Most real-world applications involve working with files and databases. Learn about:
- Reading from and writing to files
- Working with CSV and JSON formats
- Basic SQL queries and database operations
Create a project that involves reading data from a file, processing it, and storing results in a database.
2.4 Introduction to Web Development
Even if you’re not planning to become a web developer, understanding web technologies is valuable. Learn the basics of:
- HTML and CSS
- JavaScript for front-end interactivity
- Basic server-side programming (e.g., using Flask for Python)
Build a simple personal website or a basic web application to apply these skills.
3. Advancing Your Skills: Tackling Complex Projects
As you become more proficient, it’s time to challenge yourself with more advanced concepts and larger projects.
3.1 Dive into Advanced Programming Concepts
Expand your knowledge with more advanced topics:
- Multithreading and concurrency
- Design patterns and software architecture
- Functional programming concepts
- Regular expressions and text processing
Apply these concepts by refactoring your earlier projects or creating new ones that specifically utilize these advanced features.
3.2 Explore Specialized Areas
Based on your interests, delve into specialized areas of programming such as:
- Machine Learning and Artificial Intelligence
- Data Science and Big Data
- Mobile App Development
- Game Development
- Cybersecurity
Each of these fields has its own set of technologies and best practices to learn. Choose one or two areas that align with your career goals or personal interests.
3.3 Contribute to Open Source Projects
Contributing to open source is an excellent way to gain real-world experience and collaborate with other developers. Start by:
- Finding projects that interest you on platforms like GitHub
- Reading the contribution guidelines
- Starting with small contributions like bug fixes or documentation improvements
- Gradually taking on larger features or improvements
3.4 Build a Substantial Personal Project
Develop a significant project that showcases your skills. This could be:
- A full-stack web application
- A mobile app with a backend service
- A machine learning project with practical applications
- A complex game or simulation
Ensure your project incorporates multiple technologies and demonstrates your problem-solving abilities.
4. Preparing for Professional Development
As you near the end of your learning journey, it’s time to focus on skills that will help you transition into a professional role.
4.1 Master Version Control
Version control is essential in professional development. Focus on:
- Git basics (commit, push, pull, branching)
- Collaborative workflows (pull requests, code reviews)
- Advanced Git features (rebasing, cherry-picking)
Practice these skills by version controlling all your projects and collaborating with others on GitHub or GitLab.
4.2 Learn About Software Development Methodologies
Familiarize yourself with common development methodologies:
- Agile and Scrum
- Test-Driven Development (TDD)
- Continuous Integration/Continuous Deployment (CI/CD)
Try implementing these methodologies in your personal projects to get a feel for how they work in practice.
4.3 Develop Soft Skills
Technical skills are crucial, but soft skills are equally important in a professional setting. Focus on improving:
- Communication skills (both written and verbal)
- Problem-solving and critical thinking
- Teamwork and collaboration
- Time management and organization
Participate in coding forums, attend local tech meetups, or join online communities to practice these skills.
4.4 Prepare for Technical Interviews
Many top tech companies have rigorous technical interviews. Prepare by:
- Practicing coding problems on platforms like LeetCode, HackerRank, or AlgoCademy
- Studying common algorithms and data structures
- Practicing system design questions
- Conducting mock interviews with peers or mentors
5. Continuous Learning and Specialization
The field of programming is constantly evolving, so your learning journey never truly ends. Here’s how to stay current and continue growing:
5.1 Stay Updated with New Technologies
Keep abreast of new developments in your field:
- Follow tech blogs and news sites
- Attend conferences and webinars
- Experiment with new tools and frameworks
5.2 Deepen Your Expertise
Choose areas where you want to become an expert:
- Read advanced books and research papers in your chosen field
- Take specialized courses or pursue certifications
- Mentor others to solidify your own understanding
5.3 Network and Build Professional Relationships
Networking can open up new opportunities and provide valuable insights:
- Join professional organizations related to your field
- Attend industry events and meetups
- Engage with other professionals on LinkedIn and other platforms
5.4 Consider Specialization or Broadening Your Skill Set
Depending on your career goals, you might choose to:
- Specialize deeply in a particular area (e.g., becoming a machine learning expert)
- Broaden your skills to become a full-stack developer or a technical lead
- Explore adjacent fields like DevOps or cloud computing
Conclusion: Your Unique Learning Journey
Remember, while this guide provides a structured approach to learning programming, your journey will be unique. Some key points to keep in mind:
- Pace yourself: Learning takes time, and it’s okay to progress at your own speed.
- Practice regularly: Consistent coding practice is key to improvement.
- Build projects: Apply your knowledge to real-world problems to solidify your understanding.
- Embrace challenges: Don’t shy away from difficult concepts or problems; they’re opportunities for growth.
- Seek help when needed: Utilize online resources, join coding communities, or find a mentor.
- Celebrate your progress: Acknowledge your achievements, no matter how small they may seem.
Your journey from “Hello World” to advanced projects is an exciting adventure filled with learning, challenges, and rewards. By following this roadmap and adapting it to your personal goals and interests, you’ll be well on your way to becoming a skilled programmer capable of tackling complex projects and potentially landing a job at a top tech company.
Remember, the key to success in programming is not just about accumulating knowledge, but also about developing problem-solving skills and a passion for continuous learning. As you progress through your journey, you’ll discover that each new concept you master opens up even more exciting possibilities in the world of coding.
So, take that first step, write your first line of code, and embark on this incredible journey. The world of programming is vast and full of opportunities, and with dedication and perseverance, you can achieve your coding goals and beyond. Happy coding!