Why Learning to Code Shouldn’t Start with Syntax: The Case for Problem-Solving First
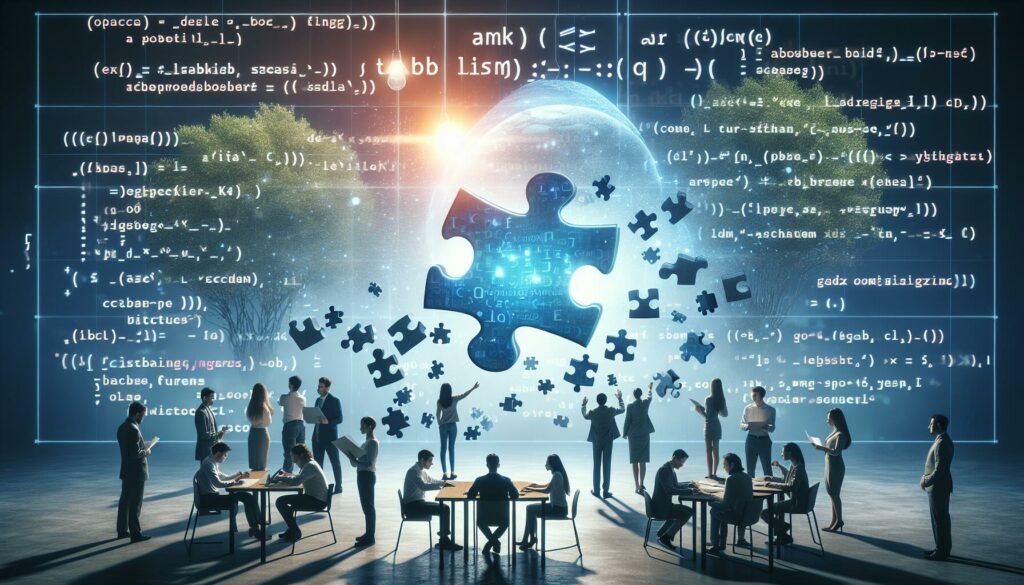
In the world of coding education, there’s a long-standing debate about the best approach to introduce newcomers to programming. Traditionally, many courses and bootcamps start by teaching syntax – the rules and structure of a programming language. However, at AlgoCademy, we believe there’s a more effective way to begin your coding journey: by focusing on problem-solving skills first. In this article, we’ll explore why this approach can lead to better outcomes for aspiring programmers and how it aligns with the broader goals of coding education.
The Traditional Approach: Syntax First
When you think about learning to code, what comes to mind? For many, it’s probably images of lines of code, curly braces, and semicolons. This is the syntax of programming languages, and it’s often where traditional coding courses begin. The rationale behind this approach is straightforward: you need to know the “language” before you can “speak” it.
A typical syntax-first lesson might look something like this:
print("Hello, World!")
if x > 5:
print("x is greater than 5")
else:
print("x is 5 or less")
Students are taught what each element means, how to structure their code, and the rules they need to follow. While this approach does have its merits – after all, you do need to learn syntax eventually – starting here can often lead to several challenges:
- Overwhelm: For complete beginners, being immediately confronted with unfamiliar symbols and rules can be intimidating.
- Lack of Context: Without understanding why they’re writing this code, students may struggle to see its relevance or application.
- Memorization Over Understanding: There’s a risk of students focusing on memorizing syntax rather than grasping underlying concepts.
- Difficulty in Transferring Skills: When faced with a new programming language, students might feel like they’re starting from scratch.
The AlgoCademy Approach: Problem-Solving First
At AlgoCademy, we advocate for a different approach: starting with problem-solving skills. This method focuses on developing computational thinking and algorithmic reasoning before diving into the specifics of any particular programming language. Here’s why we believe this approach is superior:
1. Developing a Problem-Solving Mindset
Programming, at its core, is about solving problems. By starting with problem-solving exercises, students learn to break down complex issues into smaller, manageable parts. They develop critical thinking skills that are applicable not just in coding, but in many areas of life and work.
For example, consider this problem-solving exercise:
You have a 5-liter jug and a 3-liter jug. How can you measure exactly 4 liters of water?
This puzzle doesn’t require any coding knowledge, but it does require the kind of logical thinking that’s essential in programming. Students learn to approach problems systematically, consider different solutions, and think creatively.
2. Understanding Algorithms
An algorithm is a step-by-step procedure for solving a problem or accomplishing a task. By focusing on algorithms before syntax, students learn to think about the logic and structure of their solutions independently of any specific programming language.
For instance, students might learn about sorting algorithms by physically sorting a deck of cards or a list of numbers. They can understand the concept of a “bubble sort” by seeing how larger elements “bubble up” to the top of the list with each pass.
3. Visualizing Code Execution
Another benefit of the problem-solving first approach is that it helps students visualize how code executes. Tools like flowcharts and pseudocode can be introduced to represent the flow of an algorithm without getting bogged down in syntax details.
Here’s an example of how a simple algorithm might be represented in pseudocode:
START
Input number N
Set sum = 0
For each number from 1 to N:
Add the number to sum
Print sum
END
This pseudocode describes an algorithm to calculate the sum of numbers from 1 to N. It’s easy to understand and can be translated into any programming language once the syntax is learned.
4. Building Confidence
Starting with problem-solving helps build confidence. Students see that they can devise solutions to complex problems even before they know how to write code. This confidence can carry them through the challenges of learning syntax and debugging code later on.
5. Preparing for Real-World Coding
In professional settings, programmers often start by planning their approach and designing algorithms before writing any code. By learning in this order, students are better prepared for real-world coding practices.
Integrating Syntax Learning
Of course, learning syntax is still a crucial part of becoming a proficient programmer. The key is in how and when it’s introduced. At AlgoCademy, we integrate syntax learning gradually, always in the context of problem-solving.
Here’s how this integration might look:
- Introduce a problem: Start with a real-world problem that needs solving.
- Develop an algorithm: Guide students in creating a step-by-step solution using pseudocode or flowcharts.
- Introduce relevant syntax: Show how the algorithm can be translated into actual code, introducing necessary syntax elements as needed.
- Practice and refine: Allow students to practice writing and refining the code, reinforcing both problem-solving skills and syntax knowledge.
This approach ensures that students understand why they’re using certain syntax elements, not just how to use them.
The Benefits of Problem-Solving First in Coding Education
The problem-solving first approach aligns closely with the broader goals of coding education. Let’s explore some of the key benefits:
1. Fostering Computational Thinking
Computational thinking is a set of problem-solving skills that are fundamental to computer science but applicable in many fields. It includes:
- Decomposition: Breaking down complex problems into smaller, manageable parts
- Pattern Recognition: Identifying similarities or patterns within and between problems
- Abstraction: Focusing on the important information while ignoring irrelevant details
- Algorithm Design: Developing step-by-step solutions to problems
By starting with problem-solving, we directly cultivate these skills, which form the foundation of strong programming abilities.
2. Enhancing Logical Reasoning
Programming requires strong logical reasoning skills. When students start by solving problems and designing algorithms, they’re exercising and developing these skills from the outset. This logical thinking becomes ingrained and carries over when they start writing actual code.
3. Improving Problem-Solving Transferability
One of the challenges in coding education is helping students transfer their skills from one programming language to another. When the focus is on problem-solving and algorithmic thinking, these skills become language-agnostic. Students who learn this way find it easier to pick up new programming languages because they understand the underlying concepts.
4. Preparing for Technical Interviews
At AlgoCademy, one of our focuses is preparing students for technical interviews, particularly for major tech companies. These interviews often emphasize problem-solving skills over syntax knowledge. By starting with problem-solving, we’re aligning our curriculum with what these companies are looking for in candidates.
5. Encouraging Creativity and Innovation
When students focus on problem-solving first, they’re encouraged to think creatively and come up with innovative solutions. This approach fosters a mindset of exploration and experimentation, which is crucial in the ever-evolving field of technology.
Implementing Problem-Solving First in Coding Education
So how can we effectively implement this problem-solving first approach in coding education? Here are some strategies we use at AlgoCademy:
1. Start with Unplugged Activities
“Unplugged” activities are those that teach computing concepts without using a computer. These can be an excellent way to introduce problem-solving and algorithmic thinking. For example:
- Using physical objects to represent data structures (like using cups and marbles to represent arrays)
- Creating human “programs” where students give each other instructions to complete a task
- Playing logic games and puzzles that exercise computational thinking skills
2. Use Visual Programming Tools
Tools like Scratch or Blockly allow students to create programs by dragging and dropping blocks of code. This approach lets students focus on the logic and structure of their programs without getting caught up in syntax details.
3. Incorporate Real-World Problems
Using real-world problems as the basis for coding exercises helps students see the relevance and application of what they’re learning. This could include:
- Designing an algorithm to optimize a delivery route
- Creating a program to analyze and visualize data from a local environmental study
- Developing a simple budgeting application
4. Emphasize Algorithmic Thinking
Teach students to think in terms of algorithms before introducing specific coding syntax. This could involve:
- Writing out step-by-step solutions in plain language
- Creating flowcharts to visualize problem-solving processes
- Analyzing and discussing different algorithmic approaches to the same problem
5. Gradual Syntax Introduction
When it’s time to introduce syntax, do so gradually and always in the context of solving a problem. For example:
- Present a problem
- Develop an algorithm to solve it
- Introduce the specific syntax needed to implement that algorithm
- Have students implement the solution using the newly learned syntax
6. Use Interactive Coding Environments
At AlgoCademy, we use interactive coding environments that allow students to experiment with code and see immediate results. This helps bridge the gap between problem-solving and coding, allowing students to see how their algorithmic solutions translate into working programs.
Overcoming Challenges in the Problem-Solving First Approach
While the problem-solving first approach has many benefits, it’s not without its challenges. Here are some potential obstacles and how to address them:
1. Student Expectations
Challenge: Some students may come in expecting to start writing code immediately and might be frustrated by the focus on problem-solving.
Solution: Clearly communicate the benefits of this approach from the outset. Show students how these problem-solving skills directly translate to coding abilities and are valued in the tech industry.
2. Balancing Theory and Practice
Challenge: There’s a risk of spending too much time on theoretical problem-solving without giving students hands-on coding experience.
Solution: Ensure a balance by integrating coding practice early, even if it’s through simplified means like pseudocode or visual programming tools. Gradually increase the amount of actual coding as students progress.
3. Adapting to Different Learning Styles
Challenge: Some students may struggle with abstract problem-solving and prefer a more concrete, code-first approach.
Solution: Provide a variety of problem-solving activities to cater to different learning styles. This could include visual, kinesthetic, and verbal approaches to problem-solving.
4. Maintaining Motivation
Challenge: Students might lose motivation if they don’t see immediate coding results.
Solution: Incorporate quick wins and demonstrable outcomes even in problem-solving exercises. For example, use coding challenges that have visual outputs or real-world applications to keep students engaged.
The Future of Coding Education
As we look to the future of coding education, the emphasis on problem-solving skills is likely to grow even stronger. Here’s why:
1. Rapid Technological Change
The tech world is evolving at an unprecedented pace. New programming languages and frameworks emerge regularly. By focusing on problem-solving skills, we’re preparing students to adapt to these changes, rather than tying their knowledge to a specific language or technology that may become outdated.
2. Artificial Intelligence and Coding
As AI-assisted coding tools become more prevalent, the role of human programmers is likely to shift. While AI can handle much of the syntax and routine coding tasks, human problem-solving skills will become even more crucial. Programmers will need to focus on high-level problem solving, algorithm design, and creative solutions – exactly the skills emphasized in a problem-solving first approach.
3. Interdisciplinary Applications
Coding skills are increasingly valuable across various fields, from biology to finance to art. A problem-solving first approach to coding education prepares students to apply their skills in diverse contexts, making them more versatile in an interdisciplinary world.
Conclusion
At AlgoCademy, we believe that starting coding education with a focus on problem-solving rather than syntax is the key to producing skilled, adaptable, and confident programmers. This approach not only prepares students for the immediate challenges of learning to code but also equips them with the critical thinking and problem-solving skills they’ll need throughout their careers.
By emphasizing algorithmic thinking, logical reasoning, and creative problem-solving, we’re not just teaching students how to code – we’re teaching them how to think like programmers. This foundation will serve them well whether they’re preparing for technical interviews at top tech companies, building their own innovative applications, or applying their coding skills in other fields.
As the world of technology continues to evolve, the ability to approach problems systematically, think algorithmically, and devise creative solutions will only become more valuable. By starting with problem-solving, we’re not just preparing students for their first programming language – we’re preparing them for a lifetime of learning, innovation, and success in the ever-changing landscape of technology.
Remember, learning to code is a journey, not a destination. By starting that journey with a strong foundation in problem-solving, we set students on a path to becoming not just coders, but computational thinkers ready to tackle the challenges of tomorrow.