How to Get Unstuck: Tactical Approaches for Rebooting Your Brain
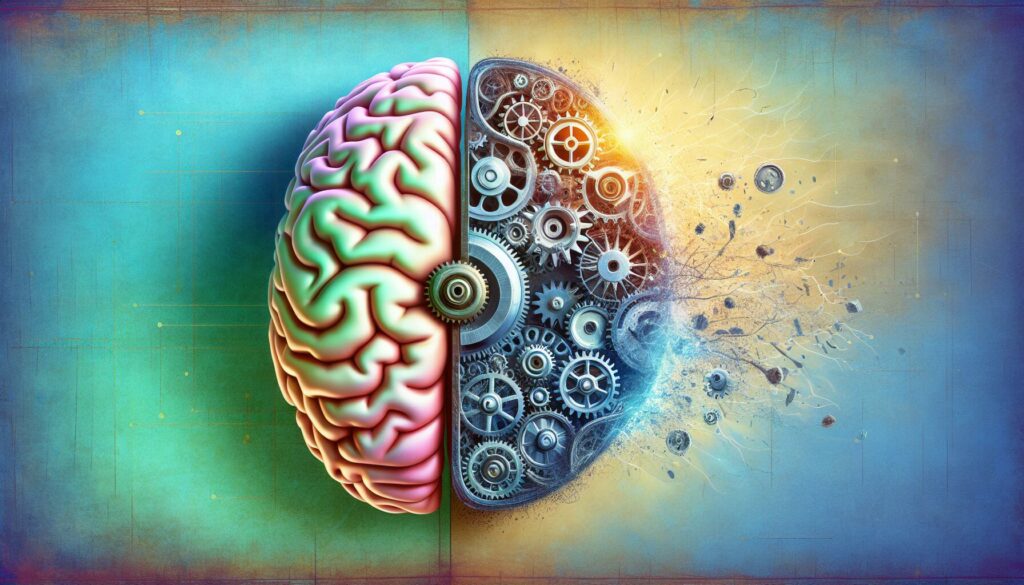
As programmers and coding enthusiasts, we’ve all been there: staring at a screen, fingers hovering over the keyboard, mind blank. Whether you’re tackling a complex algorithm, debugging a stubborn piece of code, or simply trying to start a new project, getting stuck is an inevitable part of the coding journey. But fear not! In this comprehensive guide, we’ll explore tactical approaches to get your brain back in gear and your code flowing again.
Understanding the “Stuck” Phenomenon
Before we dive into solutions, it’s crucial to understand why we get stuck in the first place. Getting stuck isn’t just a coding problem; it’s a cognitive challenge that affects various aspects of our lives. In programming, it often manifests as:
- Analysis paralysis: Overthinking a problem to the point of inaction
- Coder’s block: Similar to writer’s block, but for programming
- Debugging dead-ends: Feeling lost in a maze of error messages
- Overwhelm: Facing a task that seems too complex or large to tackle
Recognizing these patterns is the first step towards overcoming them. Now, let’s explore some tactical approaches to reboot your brain and get back on track.
1. The Pomodoro Technique: Time-Boxing Your Way to Productivity
The Pomodoro Technique is a time management method that can be incredibly effective for programmers. Here’s how to apply it:
- Set a timer for 25 minutes (one “Pomodoro”)
- Work intensely on your task until the timer rings
- Take a short 5-minute break
- After four Pomodoros, take a longer 15-30 minute break
This technique helps in two ways: it creates a sense of urgency that can jumpstart your brain, and it prevents burnout by ensuring regular breaks. During your Pomodoro, focus solely on the task at hand. If you get stuck, make a note of it and move on to another aspect of the problem. Often, solutions will come to you during your breaks or in subsequent Pomodoros.
2. Rubber Duck Debugging: Explain It to Inanimate Objects
Rubber Duck Debugging is a method of debugging code by explaining it, line by line, to an inanimate object (traditionally, a rubber duck). This technique works because it forces you to articulate your code and your problem, often leading to a “eureka” moment as you verbalize your thoughts.
Here’s how to practice Rubber Duck Debugging:
- Find a rubber duck (or any object)
- Explain your code or problem to the duck, in detail
- Go through your explanation step-by-step
- Pay attention to any moments of realization or confusion
This method can help you spot logical errors, missing steps, or alternative approaches that weren’t obvious before. It’s particularly useful when you’re deep in the weeds of a complex problem and need a fresh perspective.
3. Change Your Environment: A New View for New Ideas
Sometimes, the key to getting unstuck is as simple as changing your surroundings. Our brains associate certain environments with certain states of mind, and a change of scenery can trigger new thought patterns. Try these environment-changing tactics:
- Work from a different location (a café, library, or even a different room)
- Rearrange your workspace
- Go for a walk or exercise
- Switch from your computer to pen and paper
Physical movement and new visual inputs can stimulate different parts of your brain, potentially leading to new insights or solutions. Even a short walk around the block can provide the mental reset you need to approach your problem with fresh eyes.
4. Break It Down: Divide and Conquer
When faced with a complex problem, it’s easy to feel overwhelmed. The “Divide and Conquer” approach can help you break down seemingly insurmountable tasks into manageable chunks. Here’s how to apply this strategy:
- Identify the main components of your problem
- Break each component into smaller, actionable tasks
- Prioritize these tasks
- Focus on one small task at a time
For example, if you’re stuck on implementing a complex algorithm, you might break it down like this:
1. Understand the problem
1.1 Read the problem statement carefully
1.2 Identify input and output requirements
1.3 List any constraints or edge cases
2. Plan the approach
2.1 Sketch out a high-level solution
2.2 Identify key data structures needed
2.3 Outline the main steps of the algorithm
3. Implement core functionality
3.1 Write pseudocode for each main step
3.2 Implement each step in actual code
3.3 Test each step individually
4. Handle edge cases and optimize
4.1 Identify potential edge cases
4.2 Add logic to handle these cases
4.3 Analyze time and space complexity
4.4 Optimize if necessary
5. Test and refine
5.1 Create comprehensive test cases
5.2 Run tests and debug
5.3 Refactor for clarity and efficiency
By breaking down the problem into these smaller tasks, you create a roadmap for yourself. This approach not only makes the overall task less daunting but also provides clear, achievable goals that can help maintain motivation and progress.
5. Leverage the Power of Pseudocode
When you’re stuck, sometimes the issue isn’t the logic of your solution, but rather the syntax or implementation details. This is where pseudocode comes in handy. Pseudocode allows you to focus on the algorithmic thinking without getting bogged down in language-specific syntax.
Here’s an example of how you might use pseudocode to outline a solution for finding the maximum element in an array:
function findMaxElement(array):
if array is empty:
return null
set maxElement to first element of array
for each element in array:
if element > maxElement:
set maxElement to element
return maxElement
By writing out your solution in pseudocode, you can:
- Clarify your thinking
- Identify logical errors or missing steps
- Create a blueprint for your actual code
- Communicate your ideas more easily (useful for pair programming or seeking help)
Once you have a solid pseudocode outline, translating it into actual code often becomes much easier, helping you overcome that initial hurdle of getting started.
6. Embrace the Power of Analogies
Sometimes, the best way to understand a complex programming concept or solve a difficult problem is to relate it to something familiar. Analogies can bridge the gap between the abstract world of code and the concrete experiences of everyday life.
For example, you might explain recursion like this:
Imagine you’re standing in a line at a movie theater. To find out your position, you could ask the person in front of you, “What’s your position?” If they don’t know, they’ll ask the person in front of them, and so on, until someone at the front of the line says, “I’m first!” Then, each person adds one to the number they heard and passes it back. This is like a recursive function, where each “person” is a function call, and the base case is the person at the front of the line.
Using analogies can help you:
- Gain new perspectives on familiar problems
- Explain complex concepts to others (or yourself)
- Find creative solutions by drawing parallels to other domains
When you’re stuck, try to think of real-world analogies for your problem. This mental exercise can often lead to breakthrough moments.
7. Implement Pair Programming or Rubber Duck Debugging 2.0
While Rubber Duck Debugging is effective, sometimes you need more than an inanimate object. This is where pair programming or a variation of it can be incredibly useful. If you’re working alone, consider these options:
- Virtual pair programming: Use screen-sharing tools to code with a friend or mentor remotely
- Community forums: Explain your problem on platforms like Stack Overflow or Reddit’s programming communities
- AI coding assistants: Use tools like GitHub Copilot or ChatGPT to discuss your code and get suggestions
The act of articulating your problem to another entity (human or AI) can often lead to insights. Plus, you benefit from external input that might approach the problem from an angle you hadn’t considered.
8. Take Strategic Breaks: The Art of Productive Procrastination
Sometimes, the best way to solve a problem is to stop trying to solve it—at least consciously. Our brains continue to work on problems in the background, even when we’re not actively thinking about them. This phenomenon, known as incubation, can lead to sudden insights or solutions.
To leverage this, try:
- Taking a shower (there’s a reason “shower thoughts” are a thing)
- Going for a walk in nature
- Engaging in a different, preferably creative, activity (drawing, playing music, etc.)
- Getting a good night’s sleep (our brains consolidate information during sleep)
The key is to step away from the problem completely. Don’t try to force a solution; instead, let your subconscious mind work on it while you focus on something else entirely.
9. Learn to Love the Process, Not Just the Outcome
Often, we get stuck because we’re too focused on the end result. We want our code to work perfectly, to be optimized, to impress our peers or potential employers. This pressure can be paralyzing. Instead, try to shift your mindset to appreciate the process of coding itself.
Here are some ways to cultivate this mindset:
- Celebrate small victories (like successfully implementing a helper function)
- Keep a coding journal to track your progress and insights
- Share your learning process with others (through a blog or social media)
- Embrace mistakes as learning opportunities
Remember, every bug you encounter, every optimization you discover, and every refactoring you perform is an opportunity to deepen your understanding and improve your skills.
10. Leverage Online Resources and Coding Platforms
Sometimes, the best way to get unstuck is to see how others have approached similar problems. Platforms like AlgoCademy offer a wealth of resources for this purpose:
- Interactive coding tutorials that break down complex concepts
- Step-by-step guidance for solving algorithmic problems
- AI-powered assistance to help you when you’re stuck
- A community of learners facing similar challenges
These resources can provide the scaffolding you need to build your understanding and overcome obstacles. Don’t hesitate to use them; even experienced programmers regularly consult documentation, tutorials, and community forums.
Putting It All Together: A Tactical Approach to Getting Unstuck
Now that we’ve explored various strategies, let’s put them together into a tactical approach you can use the next time you find yourself stuck:
- Recognize that you’re stuck. Awareness is the first step.
- Take a deep breath and step back from the problem.
- Try explaining the problem out loud (Rubber Duck Debugging).
- If that doesn’t work, break the problem down into smaller components.
- Use pseudocode to outline your approach without getting bogged down in syntax.
- If you’re still stuck, change your environment or take a strategic break.
- Come back to the problem with fresh eyes and try to draw analogies to familiar concepts.
- If you’re still struggling, reach out to the community or use online resources for guidance.
- Implement the Pomodoro Technique to maintain focus and prevent burnout.
- Remember to celebrate your progress, no matter how small.
Conclusion: Embracing the Challenge
Getting stuck is not a sign of failure; it’s an integral part of the learning and problem-solving process. By employing these tactical approaches, you can transform moments of frustration into opportunities for growth and breakthrough.
Remember, every experienced programmer was once a beginner who got stuck often. What sets successful programmers apart is not an absence of obstacles, but a repertoire of strategies for overcoming them. As you apply these techniques, you’ll not only solve the immediate problem at hand but also build resilience and problem-solving skills that will serve you throughout your coding journey.
So the next time you find yourself staring at a blank screen or a stubborn bug, take a deep breath, pick a strategy, and dive back in. Your future self will thank you for the perseverance and the skills you’re developing right now.
Happy coding, and may your “stuck” moments be short and your breakthroughs plentiful!