How to Break Through Mental Blocks When You’re Stuck on a Coding Problem
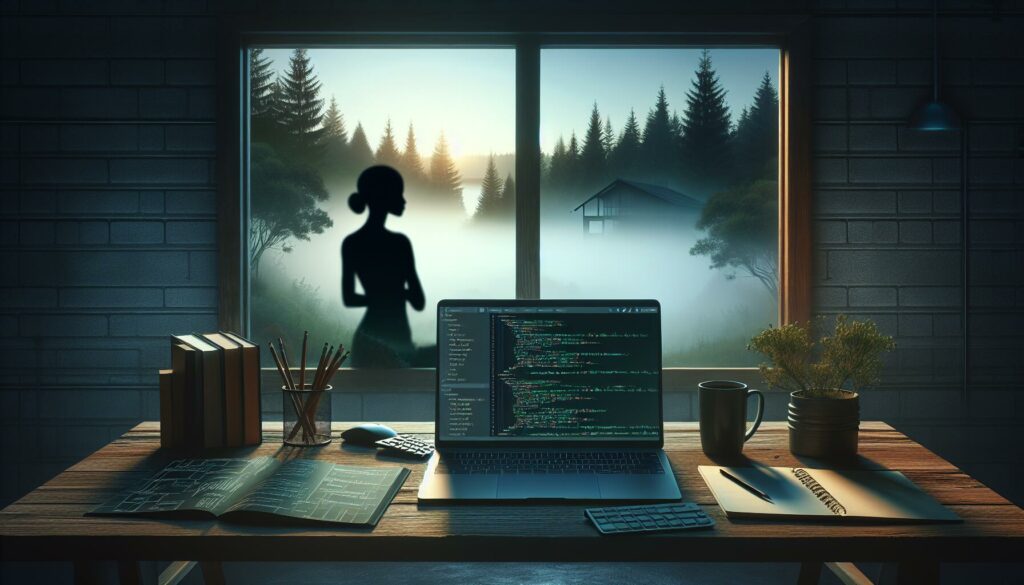
As programmers, we’ve all been there: staring at the screen, fingers hovering over the keyboard, feeling completely stuck on a coding problem. Mental blocks can be frustrating and counterproductive, especially when you’re working on an important project or preparing for a technical interview. In this comprehensive guide, we’ll explore effective strategies to overcome these obstacles and get your coding mojo back. Whether you’re a beginner learning the ropes or an experienced developer tackling complex algorithms, these techniques will help you break through mental blocks and find solutions to even the toughest coding challenges.
Understanding Mental Blocks in Coding
Before we dive into solutions, it’s important to understand what mental blocks are and why they occur. Mental blocks in coding are cognitive barriers that prevent us from solving problems or writing code effectively. They can manifest as:
- Inability to think of a solution
- Feeling overwhelmed by the complexity of a problem
- Fixation on an incorrect approach
- Lack of motivation or focus
- Fear of failure or perfectionism
These blocks can be triggered by various factors, including stress, fatigue, lack of knowledge, or simply the nature of the problem itself. Recognizing when you’re experiencing a mental block is the first step towards overcoming it.
Strategies to Break Through Mental Blocks
1. Take a Step Back and Breathe
When you feel stuck, the first thing to do is to step away from your code. Take a deep breath and give yourself permission to pause. This simple act can help reset your mind and reduce stress. Sometimes, the solution becomes apparent once you’ve allowed yourself to relax and clear your thoughts.
2. Break the Problem Down
Large, complex problems can be overwhelming. Try breaking the problem down into smaller, more manageable parts. This technique, known as decomposition, can make the task less daunting and help you focus on solving one piece at a time.
For example, if you’re working on a sorting algorithm, you might break it down like this:
- Understand the input data structure
- Choose a pivot element
- Implement the partitioning logic
- Recursively sort the sub-arrays
- Combine the results
3. Rubber Duck Debugging
Named after a method described in “The Pragmatic Programmer,” rubber duck debugging involves explaining your code or problem to an inanimate object (traditionally a rubber duck). This process often helps you spot errors or think of new approaches as you verbalize your thoughts.
Even if you don’t have a rubber duck handy, try explaining the problem out loud to yourself or write it down in plain language. This can help clarify your thinking and often leads to “aha!” moments.
4. Use Pseudocode
Sometimes, the syntax of a programming language can get in the way of problem-solving. Try writing out your solution in pseudocode first. This allows you to focus on the logic without worrying about specific language syntax.
Here’s an example of pseudocode for a simple sorting algorithm:
function sort(array):
if length of array <= 1:
return array
choose pivot element
create left array and right array
for each element in array:
if element < pivot:
add to left array
else:
add to right array
recursively sort left array
recursively sort right array
return concatenate(sorted left array, pivot, sorted right array)
5. Research and Learn
If you’re stuck because you lack knowledge in a certain area, it’s time to hit the books (or the internet). Look up relevant algorithms, data structures, or programming concepts. Websites like AlgoCademy offer interactive tutorials and resources that can help fill knowledge gaps and provide new perspectives on problem-solving.
6. Use the Pomodoro Technique
The Pomodoro Technique involves working in focused 25-minute intervals, followed by short breaks. This can help maintain concentration and prevent burnout. During your breaks, step away from your computer to refresh your mind.
7. Pair Programming or Seek Help
Two heads are often better than one. If possible, try pair programming with a colleague or friend. Explaining your problem to someone else can lead to new insights, and they might offer a fresh perspective you hadn’t considered.
If you’re working alone, don’t hesitate to seek help from online communities like Stack Overflow or programming forums. Just be sure to clearly describe your problem and show what you’ve tried so far.
8. Change Your Environment
A change of scenery can do wonders for your mental state. If you’ve been working at your desk for hours, try moving to a different room, a café, or even outside. Sometimes, a new environment can spark creativity and help you see the problem from a different angle.
9. Practice Mindfulness and Take Breaks
Mindfulness techniques, such as meditation or deep breathing exercises, can help clear your mind and reduce stress. Regular breaks are also crucial. Step away from your code periodically to engage in physical activity, even if it’s just a short walk. Physical movement can increase blood flow to the brain and boost cognitive function.
10. Use Visualization Techniques
For some people, visual representations can make abstract concepts more concrete. Try drawing diagrams, flowcharts, or mind maps to visualize your problem and potential solutions. Tools like draw.io or even a simple whiteboard can be incredibly helpful for this purpose.
11. Implement a Brute Force Solution First
If you’re struggling to find an optimal solution, start by implementing a brute force approach. While it may not be efficient, it can help you understand the problem better and potentially lead to insights for optimization.
Here’s an example of a brute force solution for finding a pair of numbers in an array that sum to a target value:
function findPair(arr, target):
for i from 0 to length of arr - 1:
for j from i + 1 to length of arr:
if arr[i] + arr[j] == target:
return [arr[i], arr[j]]
return null // No pair found
12. Review and Refactor Your Code
Sometimes, the act of reviewing and refactoring your existing code can help you spot issues or think of new approaches. Go through your code line by line, explaining to yourself what each part does. This process can often reveal logical errors or areas for improvement.
13. Use Analogies
Try relating the coding problem to a real-world analogy. This can help you think about the problem in a different context and potentially lead to creative solutions. For example, you might think of sorting algorithms as different methods of organizing a deck of cards.
14. Time-Box Your Approach
Set a time limit for trying a particular approach. If you haven’t made progress within that time frame, force yourself to try a completely different method. This prevents you from getting stuck in an unproductive loop and encourages exploring alternative solutions.
15. Practice Regularly
Mental blocks often occur when we’re faced with unfamiliar problems. Regular practice with a variety of coding challenges can help build your problem-solving skills and make you more resilient to mental blocks. Platforms like AlgoCademy offer a wide range of coding problems and algorithmic challenges to keep your skills sharp.
Applying These Strategies in Practice
Let’s walk through a scenario where we apply some of these strategies to solve a coding problem. Imagine you’re tasked with implementing a function to find the longest palindromic substring in a given string.
1. Break the problem down:
- Understand what a palindrome is
- Devise a method to check if a substring is a palindrome
- Figure out how to efficiently check all possible substrings
- Keep track of the longest palindrome found
2. Use pseudocode:
function longestPalindrome(s):
if length of s < 2:
return s
longest = s[0]
for i from 0 to length of s:
for j from i to length of s:
substring = s[i to j]
if isPalindrome(substring) and length of substring > length of longest:
longest = substring
return longest
function isPalindrome(s):
return s == reverse of s
3. Implement a brute force solution:
Based on our pseudocode, we can implement a brute force solution in Python:
def longest_palindrome(s):
if len(s) < 2:
return s
longest = s[0]
for i in range(len(s)):
for j in range(i, len(s)):
substring = s[i:j+1]
if substring == substring[::-1] and len(substring) > len(longest):
longest = substring
return longest
4. Review and refactor:
Upon review, we might realize that our brute force solution is inefficient, with a time complexity of O(n^3). We can refactor to improve efficiency:
def longest_palindrome(s):
if len(s) < 2:
return s
start, max_length = 0, 1
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
for i in range(len(s)):
len1 = expand_around_center(i, i)
len2 = expand_around_center(i, i + 1)
length = max(len1, len2)
if length > max_length:
start = i - (length - 1) // 2
max_length = length
return s[start:start + max_length]
This refactored solution uses the expand around center technique, which is more efficient with a time complexity of O(n^2).
Conclusion
Mental blocks are a common challenge in coding, but they don’t have to derail your progress. By employing these strategies, you can overcome obstacles, enhance your problem-solving skills, and become a more resilient programmer. Remember, persistence is key – even the most experienced developers face mental blocks from time to time.
Platforms like AlgoCademy can be invaluable resources in your journey to mastering coding and algorithmic thinking. They provide structured learning paths, interactive coding environments, and a wealth of practice problems to help you build the skills and confidence needed to tackle any coding challenge.
The next time you find yourself stuck on a coding problem, take a deep breath, step back, and try applying some of these techniques. With practice and perseverance, you’ll find that breaking through mental blocks becomes easier, allowing you to solve increasingly complex problems and advance your programming skills to new heights.