The Growth Mindset Approach to Improving Your Coding Skills
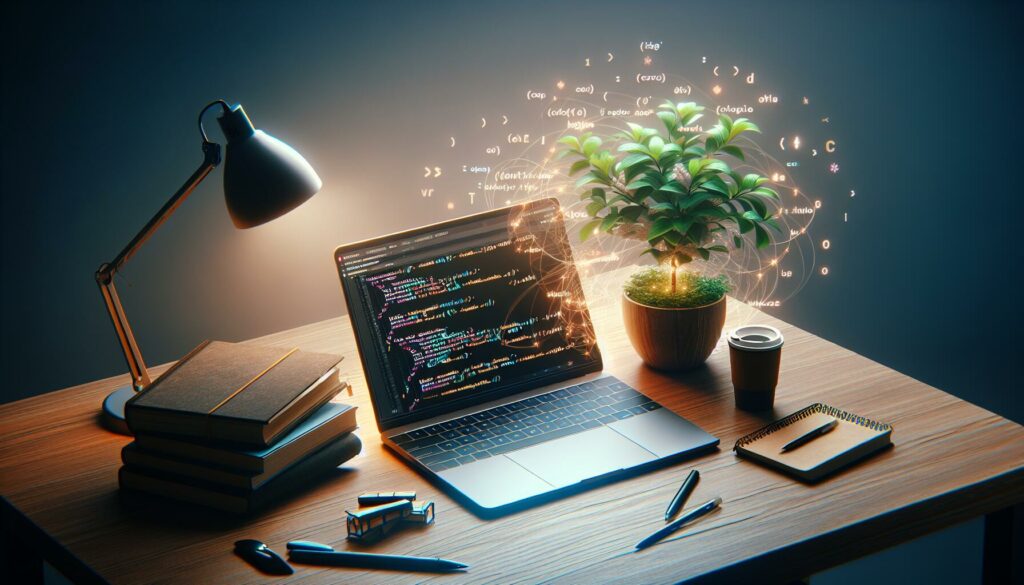
In the ever-evolving world of technology, becoming a proficient programmer is not just about learning a specific set of languages or frameworks. It’s about developing a mindset that embraces challenges, persists in the face of setbacks, and views effort as the path to mastery. This is where the concept of a growth mindset comes into play, especially when it comes to improving your coding skills.
At AlgoCademy, we believe that adopting a growth mindset is crucial for anyone looking to excel in coding and prepare for technical interviews at top tech companies. In this comprehensive guide, we’ll explore how the growth mindset can transform your approach to learning programming, and provide practical strategies to enhance your coding skills using this powerful mental framework.
Understanding the Growth Mindset
Before diving into how the growth mindset applies to coding, let’s first understand what it means. The concept of growth mindset was popularized by psychologist Carol Dweck in her groundbreaking book “Mindset: The New Psychology of Success.” According to Dweck, individuals can be categorized as having either a fixed mindset or a growth mindset.
Fixed Mindset vs. Growth Mindset
- Fixed Mindset: People with a fixed mindset believe that their abilities and intelligence are static traits that cannot be significantly changed.
- Growth Mindset: Those with a growth mindset believe that their abilities can be developed through dedication, hard work, and continuous learning.
In the context of coding and software development, embracing a growth mindset means believing that you can improve your programming skills through practice, learning from mistakes, and persistent effort.
Why a Growth Mindset is Essential for Coders
The field of programming is constantly evolving, with new languages, frameworks, and technologies emerging regularly. This rapid pace of change makes it essential for developers to adopt a growth mindset for several reasons:
- Embracing Challenges: Coding often involves tackling complex problems. A growth mindset helps you see challenges as opportunities to learn and grow, rather than insurmountable obstacles.
- Perseverance: Debugging and troubleshooting are integral parts of programming. A growth mindset fosters the resilience needed to persist through frustrating bugs and errors.
- Continuous Learning: The tech industry demands ongoing skill development. A growth mindset encourages lifelong learning and adaptability.
- Creativity and Innovation: Believing in your ability to grow and improve fosters creative problem-solving and innovative thinking in coding projects.
- Feedback Receptivity: Code reviews and constructive criticism are crucial for improvement. A growth mindset helps you view feedback as valuable input rather than personal criticism.
Applying Growth Mindset Principles to Coding
Now that we understand the importance of a growth mindset in coding, let’s explore practical ways to apply these principles to improve your programming skills:
1. Embrace the Learning Process
Instead of focusing solely on the end result, appreciate the journey of learning to code. Each line of code you write, each bug you fix, and each concept you grasp is a step forward in your development as a programmer.
// Example: Learning a new concept
function learningProcess(concept) {
while (!fullyUnderstood(concept)) {
tryDifferentApproach();
practiceMoreExamples();
seekHelp();
}
celebrate();
}
2. Set Learning Goals, Not Performance Goals
Rather than aiming to be the “best programmer” or to get a job at a specific company, set goals focused on learning and skill development. For example:
- “I will learn one new programming concept each week.”
- “I will complete three coding challenges on AlgoCademy every day.”
- “I will build a small project using a new framework by the end of the month.”
3. Embrace Mistakes as Learning Opportunities
In coding, errors and bugs are inevitable. Instead of getting discouraged, view them as valuable learning experiences. Keep a “bug journal” to document the errors you encounter and how you solved them.
// Example: Learning from mistakes
function debugAndLearn(error) {
console.log("Error encountered: ", error);
analyzeRootCause(error);
implementSolution();
documentLearning();
return improvedCode;
}
4. Practice Deliberate Learning
Engage in focused, intentional practice to improve specific aspects of your coding skills. This could involve:
- Solving algorithmic problems on platforms like AlgoCademy
- Participating in coding challenges or hackathons
- Contributing to open-source projects
- Pair programming with more experienced developers
5. Seek and Embrace Feedback
Actively seek feedback on your code from peers, mentors, or through code reviews. Treat constructive criticism as a valuable tool for improvement, not as a judgment of your abilities.
// Example: Implementing feedback
function implementFeedback(feedback) {
for (let suggestion of feedback) {
if (isValid(suggestion)) {
applyImprovement(suggestion);
} else {
discussAndClarify(suggestion);
}
}
return improvedCodeBase;
}
6. Cultivate a Love for Problem-Solving
Approach coding challenges with enthusiasm. Develop a genuine interest in solving problems and puzzles, which is at the heart of programming.
7. Emphasize Effort and Strategy
When you succeed in solving a difficult problem or implementing a complex feature, acknowledge the effort and strategies you used, not just the outcome. This reinforces the connection between hard work and success.
Overcoming Challenges with a Growth Mindset
Even with a growth mindset, you’ll encounter challenges in your coding journey. Here’s how to approach common obstacles:
Imposter Syndrome
Many programmers, even experienced ones, sometimes feel like they don’t belong or aren’t skilled enough. Combat this by:
- Acknowledging that everyone starts as a beginner
- Keeping a record of your achievements and progress
- Sharing your knowledge with others, which reinforces your own learning
Plateaus in Learning
When you feel like you’re not making progress:
- Try learning a completely new technology or language to gain fresh perspective
- Revisit fundamentals to solidify your understanding
- Set new, challenging goals to push yourself out of your comfort zone
Overwhelming Complexity
When faced with complex problems or large projects:
- Break the problem down into smaller, manageable parts
- Use pseudocode to plan your approach before diving into actual coding
- Seek help and collaborate with others when needed
// Example: Breaking down a complex problem
function tackleComplexProblem(problem) {
const subProblems = breakDownProblem(problem);
for (let subProblem of subProblems) {
const solution = solveManagedablePart(subProblem);
integratePartialSolution(solution);
}
return completeSolution;
}
Tools and Resources for Cultivating a Growth Mindset in Coding
To support your journey in developing a growth mindset and improving your coding skills, consider utilizing the following resources:
1. Interactive Learning Platforms
Platforms like AlgoCademy offer structured learning paths and interactive coding challenges that cater to different skill levels. These platforms often provide immediate feedback and step-by-step guidance, which is crucial for reinforcing the growth mindset approach to learning.
2. Coding Communities and Forums
Engage with other developers through:
- Stack Overflow for problem-solving and knowledge sharing
- GitHub for collaborating on open-source projects
- Reddit communities like r/learnprogramming for discussions and advice
3. Mindset Development Resources
Explore resources specifically focused on developing a growth mindset:
- Books like “Mindset” by Carol Dweck
- Online courses on platforms like Coursera or edX about learning strategies and mindset
- TED Talks and podcasts on personal development and learning psychology
4. Project-Based Learning
Engage in project-based learning to apply your skills in practical scenarios:
- Personal projects that solve real-world problems
- Contribute to open-source projects on GitHub
- Participate in hackathons or coding competitions
5. Mentorship and Peer Learning
Seek out mentorship opportunities or form study groups:
- Find a mentor through platforms like CodeMentor or within your professional network
- Join or create a local coding meetup group
- Participate in pair programming sessions
Measuring Progress and Celebrating Growth
An essential aspect of maintaining a growth mindset is recognizing and celebrating your progress. Here are some ways to track your development and acknowledge your growth:
1. Keep a Coding Journal
Maintain a journal or blog where you document your learning journey. Include:
- New concepts you’ve learned
- Challenges you’ve overcome
- Projects you’ve completed
- Reflections on your problem-solving process
2. Use Version Control
Regularly commit your code to version control systems like Git. This allows you to look back and see how your coding style and skills have evolved over time.
// Example: Committing progress to Git
git add .
git commit -m "Implemented new feature X, demonstrating use of concept Y"
git push origin main
3. Set and Review Milestones
Establish clear, achievable milestones in your learning journey and review them periodically. For example:
- Complete 50 algorithmic challenges on AlgoCademy
- Build and deploy a full-stack web application
- Contribute to 5 open-source projects
4. Seek Feedback and Track Improvements
Regularly ask for code reviews and feedback from peers or mentors. Keep track of the improvements you make based on this feedback.
5. Celebrate Small Wins
Acknowledge and celebrate your progress, no matter how small. This could be solving a tricky bug, understanding a complex concept, or receiving positive feedback on your code.
The Role of AlgoCademy in Fostering a Growth Mindset
At AlgoCademy, we’ve designed our platform with the growth mindset philosophy at its core. Here’s how our features support and encourage a growth-oriented approach to learning coding:
1. Adaptive Learning Paths
Our curriculum adapts to your skill level and learning pace, ensuring that you’re always challenged but not overwhelmed. This supports the growth mindset principle of embracing challenges at the right level of difficulty.
2. Interactive Coding Challenges
Our coding challenges provide immediate feedback and hints, allowing you to learn from mistakes in real-time and encouraging persistence in problem-solving.
3. AI-Powered Assistance
Our AI assistant provides personalized guidance, helping you understand concepts and overcome obstacles. This feature reinforces the idea that seeking help and learning from others is a crucial part of growth.
4. Progress Tracking
AlgoCademy’s progress tracking features allow you to visualize your improvement over time, reinforcing the connection between effort and progress.
5. Community Features
Our platform includes community forums and collaborative learning opportunities, fostering a supportive environment where learners can share experiences and learn from each other.
Conclusion: Embracing Growth in Your Coding Journey
Adopting a growth mindset is not just about improving your coding skills; it’s about transforming your entire approach to learning and personal development. By embracing challenges, persisting through difficulties, learning from feedback, and celebrating your progress, you set yourself up for long-term success in the dynamic field of programming.
Remember, becoming a skilled programmer is a journey, not a destination. Every line of code you write, every bug you fix, and every concept you master is a step forward in your growth. With platforms like AlgoCademy and the right mindset, you have all the tools you need to continuously improve and achieve your coding goals.
So, the next time you face a challenging coding problem or a new technology to learn, approach it with enthusiasm and the belief that you can grow and improve. Your potential as a programmer is not fixed – it’s limitless when coupled with a growth mindset and persistent effort.
Happy coding, and may your journey of growth and learning never cease!