How to Refactor Old Solutions to Understand New Concepts
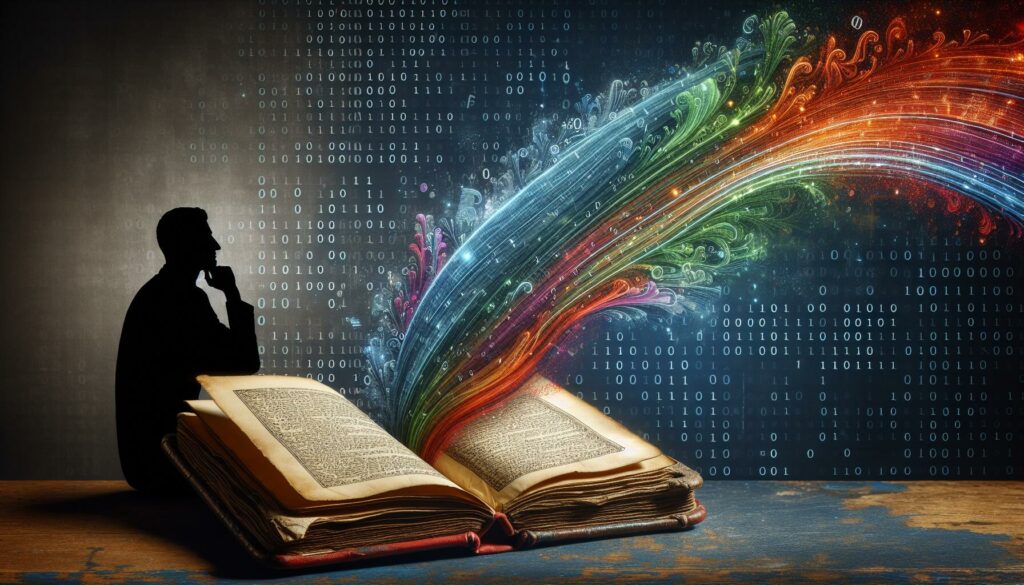
In the ever-evolving world of programming, staying up-to-date with new concepts and techniques is crucial for any developer. One effective way to grasp these new ideas is by refactoring old solutions. This process not only helps in understanding new concepts but also improves the quality of existing code. In this comprehensive guide, we’ll explore the art of refactoring old solutions to understand new concepts, with practical examples and best practices.
Understanding Refactoring
Before we dive into the process of refactoring old solutions, let’s first understand what refactoring means in the context of programming.
Refactoring is the process of restructuring existing code without changing its external behavior. The goal is to improve the code’s internal structure, making it more readable, maintainable, and efficient. When done correctly, refactoring can lead to cleaner, more organized code that’s easier to understand and modify.
Why Refactor Old Solutions?
Refactoring old solutions to understand new concepts offers several benefits:
- Deeper understanding of new concepts: By applying new concepts to familiar code, you can better grasp how these concepts work in practice.
- Improved code quality: Refactoring often leads to more efficient, readable, and maintainable code.
- Enhanced problem-solving skills: The process of refactoring challenges you to think critically about code structure and design.
- Better preparation for technical interviews: Many technical interviews involve discussing or implementing improvements to existing code.
Step-by-Step Guide to Refactoring Old Solutions
Now, let’s walk through the process of refactoring old solutions to understand new concepts:
1. Identify the New Concept
The first step is to clearly identify the new concept you want to understand. This could be a new language feature, a design pattern, or a programming paradigm. For example, let’s say you want to understand the concept of higher-order functions in JavaScript.
2. Choose an Old Solution
Select an old solution that you’re familiar with and that could benefit from the application of the new concept. For our example, let’s consider a simple function that calculates the sum of an array of numbers:
function sumArray(arr) {
let sum = 0;
for (let i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
const numbers = [1, 2, 3, 4, 5];
console.log(sumArray(numbers)); // Output: 15
3. Analyze the Old Solution
Before refactoring, analyze the old solution. Understand its purpose, strengths, and potential areas for improvement. In our example, the function works correctly but uses a traditional for loop and isn’t very flexible.
4. Apply the New Concept
Now, apply the new concept to refactor the old solution. In our case, we’ll use a higher-order function (reduce) to simplify the code:
const sumArray = (arr) => arr.reduce((sum, num) => sum + num, 0);
const numbers = [1, 2, 3, 4, 5];
console.log(sumArray(numbers)); // Output: 15
This refactored version uses the reduce method, which is a higher-order function in JavaScript. It takes a callback function as an argument and applies it to each element of the array, accumulating the result.
5. Compare and Contrast
After refactoring, compare the new solution with the old one. Analyze the differences and think about the advantages and potential drawbacks of the new approach. In our example:
- The new version is more concise and readable.
- It uses a functional programming approach, which can be more declarative and easier to reason about.
- The higher-order function (reduce) abstracts away the iteration logic, allowing us to focus on the operation we want to perform.
6. Extend the Concept
To deepen your understanding, try to extend the use of the new concept. For instance, we could create a more general higher-order function that can perform various operations on an array:
const arrayOperation = (arr, operation) => arr.reduce(operation);
const sum = arrayOperation([1, 2, 3, 4, 5], (acc, num) => acc + num);
const product = arrayOperation([1, 2, 3, 4, 5], (acc, num) => acc * num);
console.log(sum); // Output: 15
console.log(product); // Output: 120
This example demonstrates how higher-order functions can make our code more flexible and reusable.
Best Practices for Refactoring
When refactoring old solutions to understand new concepts, keep these best practices in mind:
1. Maintain Functionality
The refactored code should produce the same output as the original code. Always test your refactored solution to ensure it maintains the original functionality.
2. Take Small Steps
Refactor in small, incremental steps. This approach makes it easier to track changes and debug if issues arise.
3. Use Version Control
Always use version control (like Git) when refactoring. This allows you to revert changes if needed and compare different versions of your code.
4. Write Tests
If possible, write unit tests before refactoring. This helps ensure that your changes don’t introduce new bugs.
5. Document Your Process
Keep notes on your refactoring process. This can be valuable for future reference and for explaining your thought process to others.
Common Refactoring Techniques
Here are some common refactoring techniques you can apply to understand new concepts:
1. Extract Method
This involves taking a piece of code and turning it into its own method. This can help in understanding the concept of modularity and code organization.
2. Replace Conditional with Polymorphism
This technique involves replacing complex conditional statements with polymorphic behavior. It’s useful for understanding object-oriented design principles.
3. Introduce Parameter Object
When a method has too many parameters, you can group them into a single object. This helps in understanding object composition and data structures.
4. Replace Loop with Pipeline
This involves replacing traditional loops with a series of operations on a collection, often using functional programming concepts like map, filter, and reduce.
Real-World Example: Refactoring for Asynchronous Programming
Let’s look at a more complex example of refactoring to understand asynchronous programming concepts in JavaScript. Consider this old solution that uses callbacks to fetch data from an API:
function fetchUserData(userId, callback) {
// Simulating an API call
setTimeout(() => {
const userData = { id: userId, name: 'John Doe', email: 'john@example.com' };
callback(null, userData);
}, 1000);
}
function fetchUserPosts(userId, callback) {
// Simulating an API call
setTimeout(() => {
const posts = [
{ id: 1, title: 'First Post' },
{ id: 2, title: 'Second Post' }
];
callback(null, posts);
}, 1000);
}
function displayUserInfo(userId) {
fetchUserData(userId, (error, userData) => {
if (error) {
console.error('Error fetching user data:', error);
return;
}
console.log('User Data:', userData);
fetchUserPosts(userId, (error, posts) => {
if (error) {
console.error('Error fetching user posts:', error);
return;
}
console.log('User Posts:', posts);
});
});
}
displayUserInfo(123);
This code uses nested callbacks, which can lead to “callback hell” and make the code harder to read and maintain. Let’s refactor this using Promises and async/await to understand these newer asynchronous programming concepts:
function fetchUserData(userId) {
return new Promise((resolve) => {
setTimeout(() => {
const userData = { id: userId, name: 'John Doe', email: 'john@example.com' };
resolve(userData);
}, 1000);
});
}
function fetchUserPosts(userId) {
return new Promise((resolve) => {
setTimeout(() => {
const posts = [
{ id: 1, title: 'First Post' },
{ id: 2, title: 'Second Post' }
];
resolve(posts);
}, 1000);
});
}
async function displayUserInfo(userId) {
try {
const userData = await fetchUserData(userId);
console.log('User Data:', userData);
const posts = await fetchUserPosts(userId);
console.log('User Posts:', posts);
} catch (error) {
console.error('Error:', error);
}
}
displayUserInfo(123);
In this refactored version:
- We’ve replaced callbacks with Promises in the fetchUserData and fetchUserPosts functions.
- The displayUserInfo function is now an async function that uses await to handle the asynchronous operations sequentially.
- Error handling is simplified using a try-catch block.
This refactoring helps us understand several new concepts:
- Promises: A way to handle asynchronous operations that provides a cleaner alternative to callbacks.
- Async/Await: Syntactic sugar for working with Promises, making asynchronous code look and behave more like synchronous code.
- Error handling in asynchronous code: Using try-catch blocks with async/await for more straightforward error management.
Challenges and Considerations
While refactoring old solutions to understand new concepts can be highly beneficial, it’s important to be aware of potential challenges:
1. Overengineering
Sometimes, in the excitement of applying a new concept, you might end up overengineering the solution. Always consider whether the refactoring truly improves the code or if it’s adding unnecessary complexity.
2. Performance Impact
Some refactoring techniques, especially those involving higher levels of abstraction, might impact performance. Always profile your code before and after refactoring to ensure you’re not introducing significant performance regressions.
3. Team Familiarity
If you’re working in a team, consider whether all team members are familiar with the new concepts you’re introducing. Sometimes, simpler code that everyone understands is better than more advanced code that only a few can maintain.
4. Backwards Compatibility
When refactoring, especially in larger projects, ensure that your changes don’t break existing functionality or integrations. This might require maintaining multiple versions or providing migration paths.
Conclusion
Refactoring old solutions to understand new concepts is a powerful learning technique that can significantly enhance your programming skills. It allows you to apply theoretical knowledge to practical scenarios, improving both your understanding of new concepts and the quality of your existing code.
Remember, the goal of refactoring is not just to make code look different, but to make it better – more readable, more maintainable, and more efficient. As you practice refactoring, you’ll develop a keen eye for code quality and a deeper understanding of various programming paradigms and techniques.
Whether you’re preparing for technical interviews, improving your coding skills, or just staying up-to-date with the latest programming trends, mastering the art of refactoring is an invaluable skill. So, the next time you encounter a new programming concept, consider revisiting some of your old code. You might be surprised at how much you can learn and improve through the process of refactoring.