Why Solving the Same Problem Multiple Times Will Make You a Better Programmer
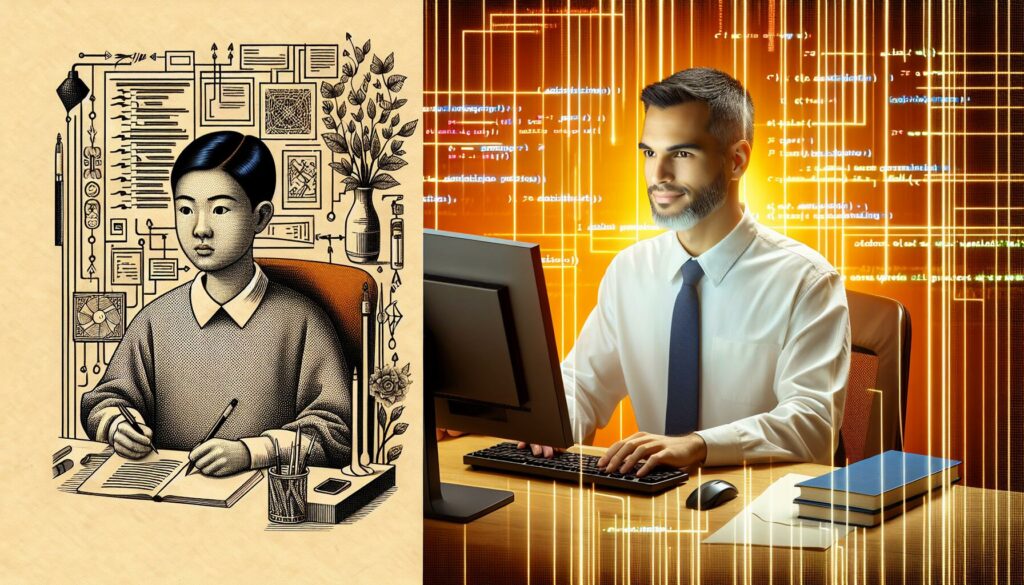
In the world of programming, there’s a common misconception that once you’ve solved a problem, you should move on to the next challenge. However, experienced developers and coding educators know that revisiting and re-solving problems can be a powerful technique for improving your programming skills. This article will explore why solving the same problem multiple times can significantly enhance your abilities as a programmer, particularly in the context of preparing for technical interviews and developing a deeper understanding of algorithms and data structures.
The Power of Repetition in Learning
Before we dive into the specifics of programming, it’s essential to understand the general principle of repetition in learning. Cognitive science has long recognized that repetition is a crucial factor in memory retention and skill development. This concept, often referred to as “spaced repetition,” involves reviewing information at gradually increasing intervals.
When applied to programming, this principle suggests that revisiting problems you’ve already solved can help solidify your understanding and improve your ability to recall and apply relevant concepts. Each time you approach a familiar problem, you’re not just repeating the same steps; you’re reinforcing neural pathways and deepening your comprehension.
Developing Multiple Approaches to Problem-Solving
One of the most significant benefits of solving the same problem multiple times is the opportunity to explore different approaches. The first time you solve a problem, you might use the most obvious or straightforward method that comes to mind. However, when you revisit the problem, you have the chance to consider alternative solutions.
For example, let’s consider the classic problem of finding the maximum element in an array. A beginner might write a simple loop to iterate through the array and keep track of the maximum value:
def find_max(arr):
max_val = arr[0]
for num in arr:
if num > max_val:
max_val = num
return max_val
While this solution works, revisiting the problem might lead you to explore more efficient or elegant solutions, such as using built-in functions or considering edge cases:
def find_max(arr):
if not arr:
return None
return max(arr)
By solving the same problem multiple times, you train yourself to think beyond your initial instincts and consider a broader range of solutions. This flexibility in thinking is crucial for tackling complex programming challenges and optimizing code in real-world scenarios.
Improving Code Efficiency and Optimization
As you gain more experience and knowledge, revisiting old problems allows you to apply new concepts and techniques to improve the efficiency of your solutions. What might have seemed like an optimal solution when you first encountered a problem could now be improved with your expanded skillset.
Consider the problem of reversing a string. A novice programmer might write something like this:
def reverse_string(s):
reversed_str = ""
for char in s:
reversed_str = char + reversed_str
return reversed_str
While this solution works, it’s not the most efficient. As you learn more about Python’s built-in functions and string slicing, you might revise your solution to be more concise and potentially more efficient:
def reverse_string(s):
return s[::-1]
This process of revisiting and optimizing solutions helps you develop a keen eye for code efficiency and teaches you to consider factors like time complexity and space complexity in your implementations.
Enhancing Pattern Recognition
Solving the same problem multiple times, especially with intervals between attempts, can significantly improve your ability to recognize patterns in problem-solving. As you encounter similar problems or variations of familiar challenges, you’ll start to see commonalities and develop a mental toolkit of problem-solving strategies.
For instance, many problems involving arrays or strings can be solved efficiently using two-pointer techniques. By revisiting problems that can benefit from this approach, you’ll become more adept at recognizing when to apply it in new situations.
Consider the problem of determining if a string is a palindrome. Initially, you might solve it like this:
def is_palindrome(s):
return s == s[::-1]
However, as you become more familiar with two-pointer techniques, you might revise your solution to something like:
def is_palindrome(s):
left, right = 0, len(s) - 1
while left < right:
if s[left] != s[right]:
return False
left += 1
right -= 1
return True
This two-pointer approach can be more efficient for very long strings and is a pattern that applies to many other problems, such as reversing an array in-place or finding a subarray with a specific sum.
Preparing for Technical Interviews
For those aiming to land jobs at top tech companies, often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google), solving the same problem multiple times is an invaluable preparation technique. Technical interviews at these companies often involve solving complex algorithmic problems under time pressure.
By revisiting problems, you’re not just memorizing solutions; you’re training your brain to quickly recognize problem patterns and apply appropriate strategies. This practice can help reduce anxiety during interviews and improve your ability to perform under pressure.
Moreover, interviewers often present variations of common problems. If you’ve solved a problem multiple times and explored different approaches, you’ll be better equipped to handle these variations and demonstrate your problem-solving versatility.
Deepening Understanding of Data Structures and Algorithms
Many programming problems are designed to test your understanding of specific data structures and algorithms. By revisiting these problems, you can deepen your comprehension of these fundamental concepts.
For example, consider a problem that involves finding the shortest path in a graph. Initially, you might solve it using a simple breadth-first search (BFS) algorithm:
from collections import deque
def shortest_path(graph, start, end):
queue = deque([[start]])
visited = set([start])
while queue:
path = queue.popleft()
node = path[-1]
if node == end:
return path
for neighbor in graph[node]:
if neighbor not in visited:
visited.add(neighbor)
new_path = list(path)
new_path.append(neighbor)
queue.append(new_path)
return None
As you learn more about graph algorithms, you might revisit this problem and implement Dijkstra’s algorithm for weighted graphs or A* search for more efficient pathfinding:
import heapq
def dijkstra(graph, start, end):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
previous = {start: None}
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_node == end:
path = []
while current_node:
path.append(current_node)
current_node = previous[current_node]
return path[::-1]
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
previous[neighbor] = current_node
heapq.heappush(pq, (distance, neighbor))
return None
This process of revisiting and applying more advanced algorithms to familiar problems helps solidify your understanding of complex data structures and algorithmic concepts.
Building Confidence and Reducing Imposter Syndrome
Programming can be intimidating, especially for beginners or those transitioning into new areas of software development. Imposter syndrome – the feeling that you’re not as competent as others perceive you to be – is common in the tech industry.
Solving the same problem multiple times can be a powerful tool for building confidence and combating imposter syndrome. As you revisit problems and find that you can solve them more quickly or efficiently than before, you gain tangible evidence of your progress and growing expertise.
This boost in confidence can have a ripple effect on your overall programming journey, encouraging you to tackle more challenging problems and persist through difficulties.
Developing a Growth Mindset
The practice of revisiting and re-solving problems aligns closely with the concept of a growth mindset – the belief that abilities can be developed through dedication and hard work. By approaching familiar problems with fresh eyes and a willingness to explore new solutions, you reinforce the idea that your programming skills are not fixed but continuously evolving.
This mindset is crucial for long-term success in programming, as the field is constantly changing and requires ongoing learning and adaptation.
Enhancing Code Readability and Style
As you gain experience and exposure to different coding styles and best practices, revisiting old problems provides an opportunity to improve the readability and maintainability of your code. What might have seemed clear when you first wrote it could now benefit from better variable naming, more efficient data structures, or clearer comments.
For instance, consider this initial solution to finding the factorial of a number:
def f(n):
if n == 0:
return 1
else:
return n * f(n-1)
After gaining more experience, you might revise it to be more readable and robust:
def calculate_factorial(number):
"""
Calculate the factorial of a non-negative integer.
Args:
number (int): The number to calculate factorial for.
Returns:
int: The factorial of the input number.
Raises:
ValueError: If the input is negative.
"""
if not isinstance(number, int):
raise TypeError("Input must be an integer")
if number < 0:
raise ValueError("Factorial is not defined for negative numbers")
if number == 0 or number == 1:
return 1
return number * calculate_factorial(number - 1)
This process of refining and improving code style contributes to your growth as a programmer and helps you develop habits that will be valuable in collaborative, professional environments.
Adapting to Different Programming Languages
As you progress in your programming career, you may need to work with multiple programming languages. Solving familiar problems in a new language can be an excellent way to learn the syntax, idioms, and best practices of that language.
For example, if you’re transitioning from Python to JavaScript, you might revisit the problem of reversing a string:
Python version:
def reverse_string(s):
return s[::-1]
JavaScript version:
function reverseString(s) {
return s.split('').reverse().join('');
}
This practice helps you understand the nuances of different languages and how to adapt your problem-solving skills to various programming paradigms.
Preparing for Real-World Scenarios
In professional software development, you’ll often encounter situations where you need to modify or optimize existing code. By practicing solving the same problem in different ways, you’re preparing yourself for these real-world scenarios.
You’ll develop the ability to look at code critically, identify areas for improvement, and implement more efficient or maintainable solutions. This skill is invaluable when working on large-scale projects or maintaining legacy codebases.
Conclusion
Solving the same problem multiple times is far from a waste of time – it’s a powerful technique for becoming a better programmer. This practice enhances your problem-solving skills, deepens your understanding of algorithms and data structures, improves your code efficiency and readability, and prepares you for technical interviews and real-world programming challenges.
As you continue your journey in programming, whether you’re using platforms like AlgoCademy or other resources, make it a habit to revisit problems you’ve solved before. Approach them with fresh eyes, challenge yourself to find new solutions, and reflect on how your approach has evolved. This iterative process of learning and improvement is key to mastering the art of programming and achieving your goals in the tech industry.
Remember, the goal isn’t just to solve problems – it’s to understand them deeply, approach them creatively, and continuously refine your skills. By embracing this mindset and practice, you’ll not only become a better programmer but also develop the resilience and adaptability needed to thrive in the ever-evolving world of technology.