Why You Should Approach Every Coding Problem Like It’s Your First
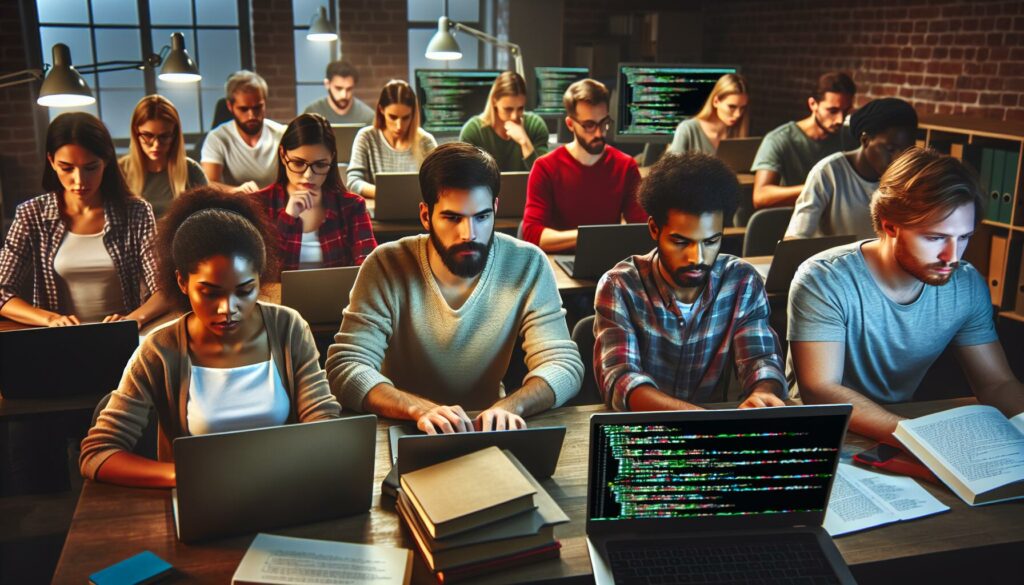
In the ever-evolving world of programming, where new technologies and frameworks emerge at a breakneck pace, one skill remains consistently valuable: the ability to approach coding problems with a fresh perspective. Whether you’re a seasoned developer or just starting your journey in the world of code, treating each problem as if it’s your first can lead to innovative solutions, deeper understanding, and ultimately, better code. In this article, we’ll explore why this mindset is crucial and how it can transform your approach to problem-solving in programming.
The Beginner’s Mind: A Powerful Tool in Coding
The concept of approaching each problem as if it’s your first is rooted in the Zen Buddhist principle of “Shoshin” or “beginner’s mind.” This philosophy encourages an attitude of openness, eagerness, and lack of preconceptions when studying a subject, even at an advanced level. In the context of coding, this mindset can be incredibly powerful.
When we approach a problem with a beginner’s mind, we:
- Question assumptions
- Explore multiple solutions
- Remain open to learning new techniques
- Avoid falling into the trap of “that’s how it’s always been done”
By adopting this approach, we can break free from the constraints of our past experiences and potentially discover more efficient or elegant solutions to coding challenges.
Breaking Down Complex Problems
One of the key benefits of approaching each coding problem like it’s your first is that it encourages you to break down complex problems into smaller, more manageable parts. This is a fundamental skill in programming, often referred to as “decomposition.”
When we encounter a new problem, we naturally tend to:
- Analyze the problem statement carefully
- Identify the core components of the problem
- Consider various approaches to solving each component
- Gradually build up to a complete solution
This step-by-step approach is incredibly valuable, even for experienced programmers. It helps prevent overlooking important details and can lead to more robust, maintainable code.
Avoiding Premature Optimization
A common pitfall in programming is the tendency to optimize code prematurely. When we approach a problem with our existing knowledge and experience, we might jump to conclusions about the most efficient solution before fully understanding the problem at hand.
By treating each problem as if it’s our first, we’re more likely to:
- Focus on correctness before efficiency
- Explore multiple implementation options
- Avoid over-engineering solutions
- Make informed decisions about optimization based on actual requirements
Remember the famous quote by Donald Knuth: “Premature optimization is the root of all evil.” By approaching problems with a fresh perspective, we’re less likely to fall into this trap.
Enhancing Problem-Solving Skills
Treating each coding problem as if it’s your first is an excellent way to continuously enhance your problem-solving skills. This approach encourages you to:
- Think critically about the problem at hand
- Consider multiple perspectives and solutions
- Strengthen your ability to analyze and decompose complex issues
- Develop a more flexible and adaptable approach to coding challenges
These skills are invaluable not just in coding, but in many aspects of life and work. By consistently exercising your problem-solving muscles, you’ll become better equipped to tackle a wide range of challenges, both in and out of the programming world.
Learning and Reinforcing Fundamental Concepts
When we approach a problem as if it’s our first, we’re more likely to revisit and reinforce fundamental programming concepts. This can be incredibly beneficial, even for experienced developers. It helps to:
- Solidify our understanding of core principles
- Identify gaps in our knowledge
- Discover new connections between different concepts
- Stay grounded in the basics, even as we work on complex projects
For example, when solving a problem related to data structures, approaching it with a beginner’s mind might lead you to review the properties of different data structures, their time and space complexities, and their appropriate use cases. This reinforcement of fundamental knowledge can lead to more informed decision-making in your code.
Fostering Creativity and Innovation
Innovation often comes from looking at problems from a new angle. When we approach each coding problem as if it’s our first, we open ourselves up to creative solutions that we might otherwise overlook.
This mindset encourages:
- Thinking outside the box
- Challenging conventional wisdom
- Experimenting with new approaches
- Combining ideas from different domains
Some of the most groundbreaking advancements in computer science and software development have come from individuals who were willing to question established norms and approach problems with fresh eyes.
Improving Code Quality and Maintainability
Approaching each problem as if it’s your first can lead to improved code quality and maintainability. When we take the time to thoroughly understand a problem and consider multiple solutions, we’re more likely to produce code that is:
- Well-structured and organized
- Clearly commented and documented
- Easier for others (and our future selves) to understand and maintain
- More robust and less prone to bugs
This approach encourages us to write code as if we’re explaining it to someone who’s seeing it for the first time – because in a sense, we are. This mindset naturally leads to better coding practices and more maintainable codebases.
Adapting to New Technologies and Paradigms
The field of programming is constantly evolving, with new languages, frameworks, and paradigms emerging regularly. By approaching each problem as if it’s our first, we maintain the flexibility and openness needed to adapt to these changes.
This mindset helps us:
- Embrace new technologies without bias
- Learn and apply new programming paradigms more easily
- Stay current with industry trends and best practices
- Avoid getting stuck in outdated ways of thinking
In an industry that values continuous learning and adaptation, maintaining a beginner’s mindset is crucial for long-term success and growth.
Overcoming Imposter Syndrome
Imposter syndrome is a common experience among programmers, where individuals doubt their skills and feel like frauds despite evidence of their competence. Approaching each problem as if it’s your first can actually help combat these feelings.
By adopting this mindset, you:
- Acknowledge that it’s okay not to know everything
- Focus on the process of learning and problem-solving rather than proving your expertise
- Reduce the pressure to have all the answers immediately
- Cultivate a growth mindset that values learning and improvement
Remember, even the most experienced programmers encounter new challenges and have to learn new things. Embracing this reality can help alleviate the anxiety associated with imposter syndrome.
Practical Tips for Adopting the “First Problem” Mindset
Now that we’ve explored the benefits of approaching each coding problem as if it’s your first, let’s look at some practical tips for adopting this mindset:
- Take a step back: Before diving into a problem, take a moment to clear your mind of preconceptions. Imagine you’re seeing the problem for the first time.
- Ask questions: Don’t be afraid to ask “naive” questions. Sometimes, questioning basic assumptions can lead to breakthrough insights.
- Write it out: Explain the problem and your thought process in writing, as if you’re describing it to someone unfamiliar with the concept.
- Explore multiple solutions: Even if you think you know the “best” way to solve a problem, challenge yourself to come up with at least two or three alternative approaches.
- Use rubber duck debugging: Explain your code and thought process to an inanimate object (like a rubber duck). This can help you spot assumptions or overlooked details.
- Embrace pair programming: Working with others, especially those with different experience levels, can help you see problems from new perspectives.
- Review fundamentals regularly: Periodically revisit basic concepts and algorithms. You might be surprised at what you rediscover or see in a new light.
- Practice with coding challenges: Regularly tackle coding problems on platforms like AlgoCademy, treating each one as a fresh challenge regardless of your experience level.
Real-World Example: Sorting Algorithm Implementation
Let’s consider a practical example of how approaching a problem with a beginner’s mind can lead to insights and improvements, even for experienced programmers. Imagine you’re tasked with implementing a sorting algorithm.
If you approach this as a familiar problem, you might immediately jump to implementing a quick sort or merge sort algorithm, as these are known to be efficient for many scenarios. However, by approaching it as if it’s your first time encountering the problem, you might:
- Start by implementing a simpler algorithm like bubble sort to understand the basics of sorting
- Analyze the strengths and weaknesses of different sorting algorithms
- Consider the specific requirements of your use case (e.g., is stability important? What’s the expected size of the input?)
- Experiment with implementing multiple algorithms and benchmark their performance
- Explore less common but potentially more suitable algorithms for your specific needs
This process might lead you to discover that for your particular use case, a counting sort or radix sort might be more efficient, or that a hybrid algorithm combining elements of different sorting methods could provide optimal performance.
Here’s a simple example of how you might implement a bubble sort in Python, approaching it as if it’s your first time:
def bubble_sort(arr):
n = len(arr)
# Explain each step of the algorithm
print("Starting the bubble sort algorithm")
# Outer loop: we need to do n-1 passes through the array
for i in range(n):
print(f"Pass {i+1}")
# Flag to check if any swaps occurred in this pass
swapped = False
# Inner loop: compare adjacent elements and swap if they're in the wrong order
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
swapped = True
print(f" Swapped {arr[j+1]} and {arr[j]}")
# If no swaps occurred, the array is already sorted
if not swapped:
print("Array is sorted, exiting early")
break
print("Sorting complete")
return arr
# Test the function
test_arr = [64, 34, 25, 12, 22, 11, 90]
print("Original array:", test_arr)
sorted_arr = bubble_sort(test_arr)
print("Sorted array:", sorted_arr)
This implementation includes detailed comments and print statements to explain each step of the process, as you might do when encountering the problem for the first time. It also includes an optimization (the swapped
flag) that you might discover as you think through the problem carefully.
By approaching the problem with a beginner’s mind, you not only reinforce your understanding of the basic sorting algorithm but also open yourself up to potential optimizations and deeper insights into the nature of sorting algorithms.
Conclusion: Embracing the Journey of Continuous Learning
In the dynamic field of programming, the ability to approach each problem with fresh eyes and an open mind is invaluable. By treating every coding challenge as if it’s your first, you cultivate a mindset of continuous learning, creativity, and growth.
This approach allows you to:
- Break down complex problems more effectively
- Avoid premature optimization and over-engineering
- Enhance your problem-solving skills
- Reinforce fundamental concepts
- Foster creativity and innovation
- Improve code quality and maintainability
- Adapt more easily to new technologies and paradigms
- Overcome imposter syndrome
Remember, every expert was once a beginner, and maintaining that beginner’s mindset can be a powerful tool throughout your programming career. Embrace the journey of continuous learning, stay curious, and don’t be afraid to question assumptions – even your own.
As you tackle your next coding challenge, whether it’s a simple algorithm implementation or a complex system design, try approaching it as if it’s your very first programming problem. You might be surprised at the insights you gain and the innovative solutions you discover.
Happy coding, and may your beginner’s mind lead you to new heights in your programming journey!