How to Maintain Curiosity While Struggling Through Coding Challenges
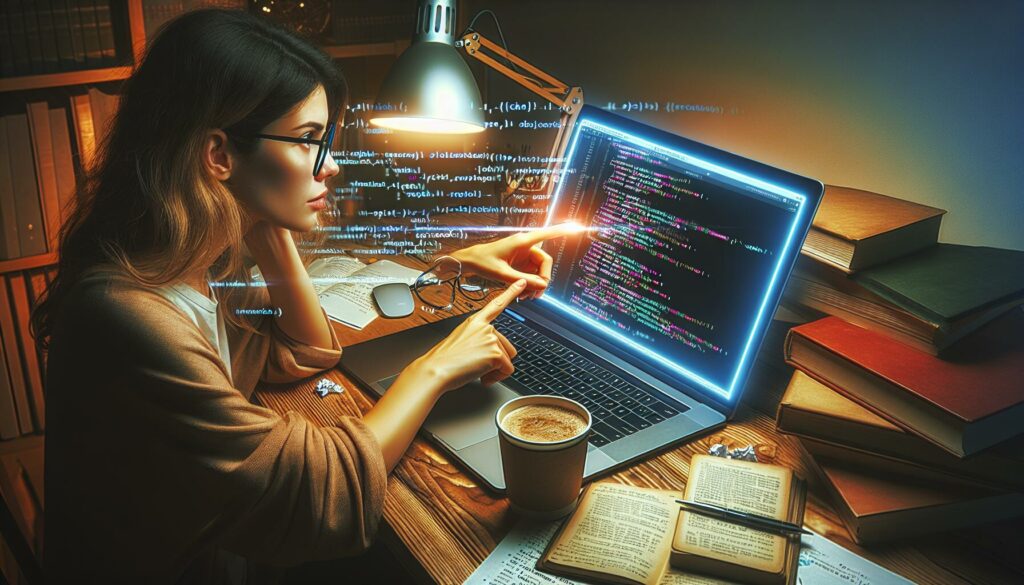
Learning to code can be an exhilarating journey filled with moments of triumph and discovery. However, it’s also a path that often leads to frustration, especially when faced with complex coding challenges. As you progress from basic syntax to intricate algorithms, maintaining your curiosity and motivation becomes crucial. This article will explore strategies to keep your coding curiosity alive, even when the going gets tough.
Understanding the Importance of Curiosity in Coding
Curiosity is the fuel that drives learning and innovation in programming. It’s what pushes you to explore new languages, tackle difficult problems, and continuously improve your skills. When you’re curious, you’re more likely to:
- Experiment with different solutions
- Dive deeper into concepts you don’t fully understand
- Seek out new challenges to test your abilities
- Stay motivated during long coding sessions
Maintaining this curiosity is especially important when preparing for technical interviews at major tech companies, often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google). These companies are known for their rigorous interview processes that test not just your coding skills, but also your problem-solving abilities and algorithmic thinking.
Strategies to Maintain Curiosity During Coding Challenges
1. Embrace the Learning Process
One of the most effective ways to maintain curiosity is to shift your focus from the end result to the learning process itself. Instead of getting frustrated when you can’t solve a problem immediately, try to approach each challenge as an opportunity to learn something new.
For example, if you’re struggling with a dynamic programming problem, take a step back and ask yourself:
- What new concept am I learning from this challenge?
- How can this problem-solving approach be applied to other scenarios?
- What patterns can I identify in this type of problem?
By reframing challenges as learning opportunities, you’ll find it easier to stay curious and engaged, even when facing difficult problems.
2. Break Down Complex Problems
Large, complex coding challenges can be overwhelming and may dampen your curiosity. To combat this, practice breaking down problems into smaller, manageable parts. This approach, often called “divide and conquer,” not only makes the problem less daunting but also allows you to maintain curiosity by focusing on solving one piece at a time.
Here’s a simple process you can follow:
- Understand the problem requirements
- Identify the main components or steps needed to solve the problem
- Break each component into smaller, solvable tasks
- Tackle each small task one by one
- Integrate the solutions to form the complete answer
This approach keeps you engaged and curious about how each piece fits into the larger puzzle.
3. Explore Different Solutions
Once you’ve solved a coding challenge, don’t stop there. Explore different ways to solve the same problem. This practice not only broadens your understanding but also keeps your curiosity piqued by showing you that there’s often more than one way to approach a problem.
For instance, if you’ve solved a sorting problem using a bubble sort algorithm, challenge yourself to implement the same solution using quicksort or mergesort. Compare the efficiency and readability of different approaches. This exploration will deepen your understanding and maintain your curiosity about various problem-solving techniques.
4. Engage with the Coding Community
Coding doesn’t have to be a solitary activity. Engaging with other programmers can reignite your curiosity and provide fresh perspectives on challenging problems. Consider:
- Joining online coding forums or communities
- Participating in coding meetups or hackathons
- Collaborating on open-source projects
- Sharing your solutions and learning from others on platforms like GitHub
Interacting with other coders exposes you to different approaches and can spark new ideas, keeping your curiosity alive.
5. Set Personal Coding Challenges
While working through structured tutorials and courses is important, setting personal coding challenges can reignite your curiosity. These could be projects that align with your interests or solve a problem you’ve encountered in your daily life.
For example, you could challenge yourself to:
- Build a simple game using a new programming language
- Create a web scraper to collect data on a topic you’re interested in
- Develop a mobile app that solves a personal problem
Personal projects allow you to apply your skills creatively, maintaining your curiosity by connecting coding to your interests and real-world applications.
6. Celebrate Small Victories
When tackling difficult coding challenges, it’s easy to focus on what you haven’t accomplished and lose sight of your progress. Celebrating small victories along the way can help maintain your curiosity and motivation.
Keep a coding journal or use a task management tool to track your progress. Acknowledge when you:
- Successfully debug a tricky piece of code
- Implement a new algorithm you’ve been studying
- Improve the efficiency of your code
- Learn a new concept or programming technique
Recognizing these small wins will fuel your curiosity to achieve more and tackle increasingly complex challenges.
7. Utilize Interactive Learning Tools
Interactive coding platforms like AlgoCademy can be invaluable in maintaining your curiosity. These platforms often provide:
- Step-by-step guidance through complex problems
- Immediate feedback on your code
- AI-powered assistance to help you when you’re stuck
- A variety of challenges that gradually increase in difficulty
The immediate feedback and structured progression can help keep you engaged and curious about what comes next in your coding journey.
Overcoming Common Curiosity Killers
Even with these strategies, there are common pitfalls that can dampen your curiosity. Let’s explore how to overcome them:
Imposter Syndrome
Feeling like you don’t belong or aren’t “good enough” at coding is a common experience, especially when preparing for interviews at top tech companies. To combat imposter syndrome:
- Remind yourself that everyone starts as a beginner
- Focus on your growth and progress rather than comparing yourself to others
- Celebrate your achievements, no matter how small
- Seek support from mentors or peers who can provide perspective
Fear of Failure
The fear of not being able to solve a problem can paralyze your curiosity. To overcome this:
- Reframe failure as a learning opportunity
- Set realistic expectations for yourself
- Practice problem-solving techniques without the pressure of finding the perfect solution immediately
- Remember that even experienced programmers face challenges and make mistakes
Overwhelm from Information Overload
The vast amount of information in the coding world can be overwhelming. To manage this:
- Focus on one concept or skill at a time
- Create a structured learning plan
- Use resources like AlgoCademy that provide a curated learning path
- Take regular breaks to avoid burnout
Practical Exercises to Reignite Your Coding Curiosity
Sometimes, the best way to maintain curiosity is through hands-on practice. Here are some exercises you can try:
1. The Daily Coding Challenge
Commit to solving one small coding problem every day. This could be a simple algorithm challenge or a short programming task. The key is consistency. Here’s an example of a daily challenge:
// Challenge: Write a function that reverses a string
function reverseString(str) {
// Your code here
}
// Test your function
console.log(reverseString("hello")); // Should output: "olleh"
Try implementing this function in different ways, such as using a loop, recursion, or built-in methods. This daily practice will keep your skills sharp and your curiosity active.
2. The Refactoring Challenge
Take a piece of code you’ve written before and challenge yourself to refactor it. This exercise helps you think critically about code efficiency and readability. For example:
// Original function
function findMax(numbers) {
let max = numbers[0];
for (let i = 1; i < numbers.length; i++) {
if (numbers[i] > max) {
max = numbers[i];
}
}
return max;
}
// Refactored version
function findMaxRefactored(numbers) {
return Math.max(...numbers);
}
// Test both functions
const numbers = [5, 2, 9, 1, 7];
console.log(findMax(numbers));
console.log(findMaxRefactored(numbers));
Reflect on the differences between the two versions. Which is more readable? More efficient? This process of improvement and reflection keeps your curiosity engaged.
3. The Cross-Language Challenge
If you’re comfortable with one programming language, challenge yourself to solve a familiar problem in a different language. This exercise broadens your perspective and maintains curiosity by exposing you to different syntax and programming paradigms.
For instance, if you’re familiar with JavaScript, try solving the same problem in Python:
// JavaScript version
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
# Python version
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n - 1) + fibonacci(n - 2)
# Test both versions
print(fibonacci(10)) # Should output: 55
Comparing how the same logic is expressed in different languages can spark curiosity about language design and efficiency.
Leveraging AI for Continuous Learning
Artificial Intelligence (AI) is revolutionizing the way we learn to code. Platforms like AlgoCademy use AI to provide personalized learning experiences that can help maintain your curiosity throughout your coding journey.
AI-Powered Code Analysis
AI can analyze your code and provide instant feedback, helping you understand your mistakes and suggesting improvements. This immediate feedback loop keeps you engaged and curious about how to optimize your code.
Personalized Learning Paths
AI algorithms can track your progress and tailor challenges to your skill level, ensuring that you’re always working on problems that are challenging enough to maintain your interest but not so difficult that they become discouraging.
Intelligent Hints and Explanations
When you’re stuck on a problem, AI can provide context-aware hints that guide you towards the solution without giving it away completely. This approach maintains your curiosity by encouraging you to think through the problem while providing support when needed.
The Role of Algorithmic Thinking in Sustaining Curiosity
Developing strong algorithmic thinking skills is crucial for maintaining curiosity in coding, especially when preparing for technical interviews at top tech companies. Algorithmic thinking involves:
- Breaking down complex problems into smaller, manageable steps
- Identifying patterns and commonalities across different problems
- Analyzing the efficiency of different solutions
- Applying abstract problem-solving techniques to concrete coding challenges
By focusing on algorithmic thinking, you shift your attention from merely writing code to solving problems creatively. This shift can reignite your curiosity by presenting each coding challenge as a puzzle to be solved rather than a task to be completed.
Practicing Algorithmic Thinking
To develop your algorithmic thinking skills and maintain curiosity:
- Study classic algorithms and data structures
- Analyze the time and space complexity of your solutions
- Practice explaining your thought process out loud (great preparation for technical interviews)
- Explore different approaches to solving the same problem
Remember, the goal is not just to find a solution that works, but to find the most efficient and elegant solution possible. This pursuit of optimization can be a powerful driver of curiosity.
Balancing Challenge and Achievement
Maintaining curiosity in coding is often about finding the right balance between challenge and achievement. If problems are too easy, you may become bored; if they’re too difficult, you might become discouraged. The key is to work in your “zone of proximal development” – a sweet spot where challenges are just beyond your current abilities but still within reach with some effort.
Tips for Finding Your Coding Sweet Spot
- Start with problems you can solve, then gradually increase the difficulty
- Set time limits for solving problems to add an extra challenge
- Revisit problems you’ve solved before and try to optimize your solutions
- Use platforms like AlgoCademy that adapt to your skill level
- Alternate between familiar concepts and new challenges to maintain confidence while pushing your boundaries
Conclusion: Cultivating a Lifelong Coding Curiosity
Maintaining curiosity while struggling through coding challenges is not just about overcoming immediate obstacles; it’s about cultivating a mindset that will serve you throughout your programming career. By embracing the learning process, breaking down complex problems, exploring different solutions, engaging with the coding community, setting personal challenges, celebrating small victories, and utilizing interactive learning tools, you can keep your curiosity alive and thriving.
Remember that every programmer, no matter how experienced, faces challenges and moments of frustration. What sets successful programmers apart is their ability to maintain curiosity and persevere through these difficult moments. With the strategies and exercises outlined in this article, you’re well-equipped to nurture your coding curiosity, tackle complex problems with enthusiasm, and continue growing as a programmer.
As you prepare for technical interviews or work on personal projects, let your curiosity be your guide. Embrace the challenges, celebrate the victories (big and small), and always keep asking, “What can I learn from this?” With this approach, you’ll not only maintain your curiosity but also develop the resilience and problem-solving skills that are invaluable in the world of coding.
Happy coding, and may your curiosity lead you to new discoveries and innovations in the vast and exciting world of programming!