How to Reframe Your Inability to Solve a Problem as a Learning Opportunity
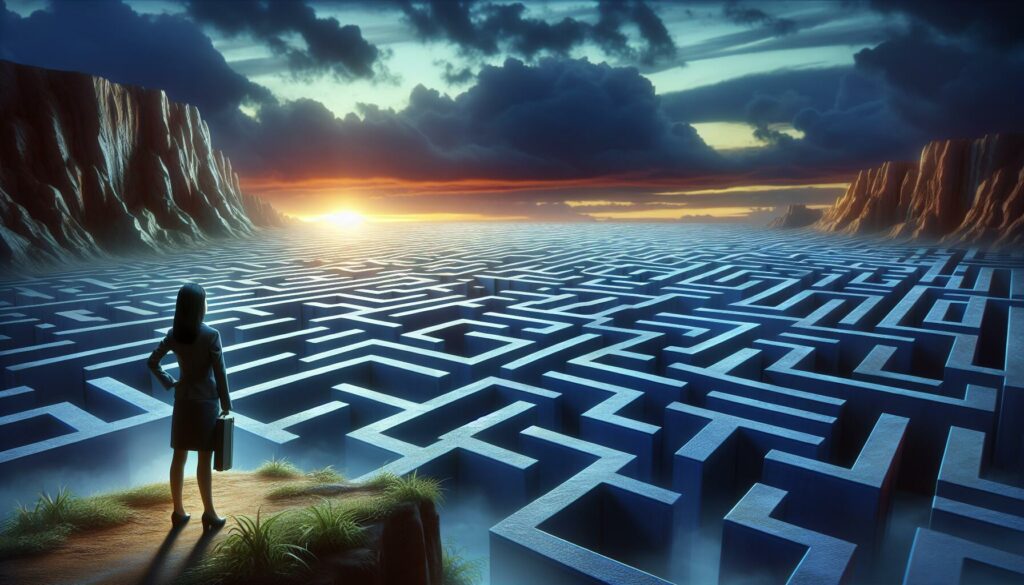
In the world of coding and programming, encountering problems you can’t immediately solve is not just common—it’s an essential part of the learning process. Whether you’re a beginner just starting your journey or an experienced developer tackling complex algorithms, the ability to reframe challenges as opportunities for growth is a crucial skill. This article will explore how to transform your perceived failures into stepping stones for success, particularly in the context of coding education and skill development.
Understanding the Learning Mindset
Before diving into specific strategies, it’s important to understand the concept of a growth mindset. Coined by psychologist Carol Dweck, a growth mindset is the belief that abilities and intelligence can be developed through dedication and hard work. This is in contrast to a fixed mindset, which assumes that our basic qualities, like intelligence or talent, are fixed traits.
In the context of coding and problem-solving:
- A fixed mindset might say: “I can’t solve this problem. I’m not smart enough.”
- A growth mindset would say: “I can’t solve this problem yet. What can I learn from this challenge?”
Adopting a growth mindset is the first step in reframing your inability to solve a problem as a learning opportunity.
The Value of Struggle in Coding Education
In platforms like AlgoCademy, which focus on coding education and programming skills development, the struggle to solve problems is not just expected—it’s designed into the learning process. Here’s why:
- Deep Learning: When you struggle with a problem, you engage more deeply with the material. This leads to better retention and understanding.
- Problem-Solving Skills: Each challenge you face helps you develop better problem-solving strategies, which are crucial for technical interviews and real-world coding scenarios.
- Resilience: Overcoming difficulties builds resilience, a key trait for success in the tech industry.
- Creativity: When standard solutions don’t work, you’re forced to think creatively, leading to innovative approaches.
Strategies for Reframing Challenges
1. Analyze the Problem
When you’re stuck, take a step back and break down the problem:
- What exactly is the problem asking?
- What do you understand about it?
- What parts are you struggling with?
This analysis helps you identify specific areas for learning and improvement. For example, if you’re working on a sorting algorithm and can’t figure out the efficiency, you’ve identified that algorithm complexity is an area you need to focus on.
2. Document Your Process
Keep a learning journal or use comments in your code to document your thought process:
// Attempted bubble sort, but it's too slow for large datasets
// TODO: Research more efficient sorting algorithms
function bubbleSort(arr) {
// ... implementation ...
}
// Trying quicksort, but struggling with choosing the pivot
// Question: How do I select an optimal pivot?
function quickSort(arr) {
// ... implementation ...
}
This practice not only helps you track your progress but also allows you to reflect on your learning journey later.
3. Use the Feynman Technique
Named after physicist Richard Feynman, this technique involves explaining the concept you’re struggling with in simple terms, as if teaching it to someone else. This process can help you identify gaps in your understanding and clarify your thoughts.
For instance, if you’re struggling with recursion, try explaining it out loud or writing it down in plain language:
“Recursion is when a function calls itself. It’s like a Russian nesting doll – each doll contains a smaller version of itself until you reach the smallest doll. In coding, each function call solves a smaller part of the problem until you reach a simple case that doesn’t need further recursion.”
4. Seek Multiple Perspectives
When you’re stuck, don’t hesitate to seek help. However, instead of just looking for the solution, try to understand different approaches to the problem. This could involve:
- Discussing the problem with peers
- Reviewing similar problems and their solutions
- Utilizing AI-powered assistance, like the features offered by AlgoCademy, to get hints and explanations
Each perspective you encounter adds to your problem-solving toolkit.
5. Embrace the Power of Yet
Add the word “yet” to your vocabulary when dealing with challenges. Instead of saying “I don’t understand this algorithm,” say “I don’t understand this algorithm yet.” This simple change acknowledges that learning is a process and that your current struggle is temporary.
Practical Application in Coding Challenges
Let’s apply these strategies to a common coding challenge: implementing a binary search algorithm. Suppose you’re struggling to write an efficient implementation.
Step 1: Analyze the Problem
Break down what you know and what you’re struggling with:
- You know binary search is more efficient than linear search for sorted arrays.
- You understand it involves dividing the search interval in half repeatedly.
- You’re struggling with the implementation details and edge cases.
Step 2: Document Your Process
Start writing your code with comments explaining your thought process:
function binarySearch(arr, target) {
// Start with the entire array
let left = 0;
let right = arr.length - 1;
// Continue searching while there are elements to check
while (left <= right) {
// Calculate the middle index
// TODO: Why use Math.floor here? Understand integer division
let mid = Math.floor((left + right) / 2);
// Check if the middle element is the target
if (arr[mid] === target) {
return mid; // Found the target
}
// If the target is greater, ignore the left half
if (arr[mid] < target) {
left = mid + 1;
}
// If the target is smaller, ignore the right half
else {
right = mid - 1;
}
}
// If we get here, the target wasn't found
return -1;
}
// Questions:
// 1. How do I handle an empty array?
// 2. What if the target is not in the array?
// 3. Is this the most efficient implementation?
Step 3: Use the Feynman Technique
Explain binary search in simple terms:
“Binary search is like guessing a number. You start in the middle of a sorted list. If your guess is too high, you eliminate the upper half. If it’s too low, you eliminate the lower half. You keep halving your search space until you find the number or run out of numbers to check.”
Step 4: Seek Multiple Perspectives
After attempting the implementation, you might:
- Look up different binary search implementations online
- Discuss your approach with a study group or mentor
- Use AlgoCademy’s AI-powered assistance to get hints on optimization
Step 5: Embrace the Power of Yet
Reframe your thoughts:
- Instead of: “I can’t implement an efficient binary search.”
- Say: “I haven’t implemented an efficient binary search yet, but I’m learning and improving with each attempt.”
Learning from Each Iteration
As you work through the binary search problem and apply these strategies, you’ll likely go through several iterations. Each iteration is a learning opportunity:
- First Attempt: You might write a basic implementation that works but isn’t optimized.
- Code Review: You learn about edge cases you didn’t consider, like handling empty arrays.
- Optimization: You discover techniques to make your code more efficient, like using bitwise operations for integer division.
- Variations: You explore recursive implementations and learn about their pros and cons compared to iterative approaches.
Each of these steps contributes to your overall understanding and skill development.
The Long-Term Benefits of Reframing
Consistently reframing your inability to solve problems as learning opportunities leads to several long-term benefits:
1. Improved Problem-Solving Skills
By approaching each challenge as a learning opportunity, you develop a more systematic approach to problem-solving. This skill is invaluable not just in coding, but in all aspects of life and work.
2. Enhanced Resilience
The more you reframe challenges, the more resilient you become to setbacks. This resilience is crucial for success in the tech industry, where rapid changes and new challenges are constant.
3. Deeper Understanding
When you struggle with a concept and work through it, you often gain a deeper understanding than if you had grasped it immediately. This depth of knowledge becomes a strong foundation for more advanced learning.
4. Increased Creativity
Facing and overcoming challenges fosters creativity. You learn to approach problems from different angles and come up with innovative solutions.
5. Better Preparation for Technical Interviews
The process of working through difficult problems and learning from them is excellent preparation for technical interviews, especially for major tech companies. These companies often look for candidates who can articulate their problem-solving process, not just those who know all the answers.
Implementing This Mindset in Your Coding Journey
To effectively implement this mindset in your coding education:
- Set Learning Goals, Not Just Performance Goals: Instead of focusing solely on solving X number of problems, set goals like “Understand and implement three different sorting algorithms.”
- Celebrate the Process: Acknowledge your progress and learning, not just your successes. Did you learn a new concept while trying to solve a problem? That’s worth celebrating!
- Use Tools and Resources Wisely: Platforms like AlgoCademy offer various resources like AI-powered assistance, step-by-step guidance, and interactive coding tutorials. Use these not just to get answers, but to deepen your understanding.
- Practice Regularly: Consistent practice helps reinforce this mindset. Set aside time each day or week to work on coding challenges.
- Reflect and Review: Periodically review your progress. Look at problems you struggled with in the past and see how your understanding has improved.
Conclusion
Reframing your inability to solve a problem as a learning opportunity is a powerful shift in perspective. It transforms frustration into curiosity, failure into growth, and challenges into stepping stones. In the world of coding and programming, where new technologies and challenges constantly emerge, this mindset is not just beneficial—it’s essential.
Remember, every expert was once a beginner who faced countless problems they couldn’t solve. The difference lies in how they approached these challenges. By embracing each problem as a chance to learn and grow, you’re not just becoming a better coder; you’re developing a mindset that will serve you well throughout your career and life.
So the next time you face a coding challenge that seems insurmountable, take a deep breath and ask yourself: “What can I learn from this?” Your future self will thank you for the growth opportunity.