The Beginner’s Advantage: Why New Coders Often Solve Problems More Creatively
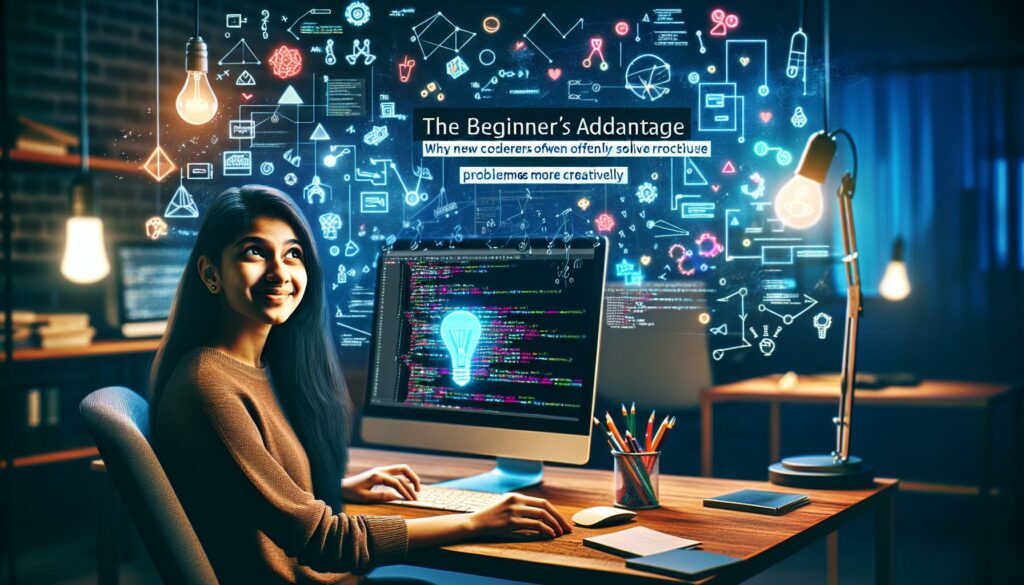
In the world of coding and software development, there’s a common misconception that experience always trumps novice enthusiasm. While it’s true that seasoned programmers bring a wealth of knowledge and tried-and-true methods to the table, beginners often possess a unique advantage that shouldn’t be overlooked: creativity in problem-solving. This phenomenon, which we’ll call “The Beginner’s Advantage,” can lead to innovative solutions and fresh perspectives that experienced coders might miss. In this article, we’ll explore why new coders often approach problems with more creativity and how this can be beneficial in the ever-evolving landscape of technology.
The Unencumbered Mind of a Beginner
When you’re new to coding, your mind is like a blank canvas. You haven’t yet been indoctrinated with the “right” way to do things or the “best practices” that experienced developers swear by. This lack of preconceived notions can be a powerful asset. Here’s why:
- No Mental Barriers: Beginners aren’t limited by what they think is impossible or impractical. They’re more likely to attempt solutions that experienced coders might dismiss outright.
- Fresh Perspective: Without years of coding habits, newcomers can look at problems from angles that might not occur to veterans.
- Willingness to Experiment: New coders are often more open to trying different approaches, even if they seem unconventional.
This unencumbered mindset can lead to breakthrough solutions that challenge the status quo and push the boundaries of what’s possible in coding.
The Power of Naïve Optimism
Beginners in coding often approach problems with a sense of optimism that can be both endearing and powerful. They haven’t yet faced the countless roadblocks and frustrations that can jade experienced developers. This naïve optimism manifests in several ways:
- Fearlessness: New coders aren’t afraid to tackle big problems because they don’t yet know how difficult they might be.
- Persistence: Without a history of failures, beginners are more likely to keep trying different solutions until they find one that works.
- Enthusiasm: The excitement of learning something new can fuel creative thinking and problem-solving.
This optimistic approach can lead to innovative solutions that more experienced developers might not attempt due to perceived limitations or past failures.
Cross-Pollination of Ideas
Many new coders come to programming from diverse backgrounds. This diversity of experience can be a wellspring of creative problem-solving. Here’s how:
- Interdisciplinary Thinking: Beginners might apply concepts from their previous fields to coding problems, leading to novel approaches.
- Real-World Context: New coders often have a fresh perspective on how coding solutions can be applied to real-world problems they’ve experienced.
- Unconventional Associations: Without years of programming patterns ingrained in their minds, beginners might make unexpected connections between concepts.
This cross-pollination of ideas from various disciplines can result in innovative solutions that experienced coders, who may be more siloed in their thinking, might overlook.
The Beginner’s Mindset in Practice
Let’s look at a practical example of how a beginner’s approach to problem-solving can lead to creative solutions. Consider the classic coding challenge of reversing a string. An experienced programmer might immediately think of using a built-in function or a standard algorithm. A beginner, however, might come up with a more unconventional approach.
Here’s how an experienced coder might solve it in Python:
def reverse_string(s):
return s[::-1]
print(reverse_string("Hello, World!")) # Output: !dlroW ,olleH
Now, let’s consider a more creative solution that a beginner might come up with:
def creative_reverse(s):
return ''.join(s[i] for i in range(len(s)-1, -1, -1))
print(creative_reverse("Hello, World!")) # Output: !dlroW ,olleH
While both solutions achieve the same result, the beginner’s approach demonstrates a deeper understanding of string manipulation and loop constructs. It might not be the most efficient solution, but it shows creative thinking and a willingness to build a solution from scratch rather than relying on built-in functions.
Embracing the Beginner’s Advantage in Coding Education
Recognizing the value of the beginner’s mindset is crucial in coding education. Platforms like AlgoCademy understand this and incorporate it into their teaching methodologies. Here’s how the beginner’s advantage is leveraged in coding education:
- Encouraging Exploration: Good coding education platforms allow beginners to explore different solutions without immediately pushing them towards “best practices.”
- Valuing Creativity: Innovative solutions are celebrated, even if they’re not the most efficient, to encourage creative thinking.
- Problem-Based Learning: Presenting real-world problems allows beginners to apply their unique perspectives to find solutions.
- Collaborative Learning: Pairing beginners with experienced coders can lead to a fusion of creative thinking and practical knowledge.
By fostering an environment that values the beginner’s perspective, coding education can produce more well-rounded and innovative programmers.
The Role of AI in Enhancing Creative Problem-Solving
Artificial Intelligence is playing an increasingly important role in coding education, and it can be particularly effective in nurturing the creative problem-solving skills of beginners. AI-powered coding assistants, like those used in AlgoCademy, can enhance the beginner’s advantage in several ways:
- Personalized Guidance: AI can adapt to a beginner’s unique thought process, providing tailored hints that encourage creative thinking rather than prescribing solutions.
- Pattern Recognition: AI can identify when a beginner is approaching a problem in a novel way and provide support for developing that idea further.
- Infinite Patience: Unlike human tutors, AI assistants can tirelessly support beginners as they experiment with different approaches, fostering a safe environment for creative exploration.
Here’s an example of how an AI assistant might interact with a beginner who’s trying to solve a problem creatively:
Beginner: I want to find the most frequent character in a string, but I don't want to use any built-in functions. Can you help?
AI Assistant: That's an interesting approach! Let's think about this step by step:
1. We need to count each character.
2. We need to keep track of which character appears most often.
How about we start with a loop to go through each character? Can you try writing that?
Beginner: Okay, I'll try:
for char in string:
# Count the character here
AI Assistant: Great start! Now, how can we count each character? We could use a dictionary to keep track of counts. Let's build on your code:
counts = {}
for char in string:
if char in counts:
counts[char] += 1
else:
counts[char] = 1
This counts each character. Can you think of how to find the most frequent one?
Beginner: Maybe we can loop through the dictionary and keep track of the highest count?
AI Assistant: Excellent thinking! Let's implement that:
max_char = ''
max_count = 0
for char, count in counts.items():
if count > max_count:
max_char = char
max_count = count
return max_char
You've just created a creative solution without using any built-in functions! Well done!
This interaction demonstrates how AI can guide beginners through their creative problem-solving process, encouraging them to develop their own solutions rather than simply providing a standard answer.
Balancing Creativity with Best Practices
While the beginner’s advantage in creative problem-solving is valuable, it’s important to balance this with an understanding of best practices and efficient coding techniques. As beginners progress in their coding journey, they should be encouraged to maintain their creative thinking while also learning from established patterns and solutions.
Here are some ways to strike this balance:
- Code Refactoring Exercises: After creating a creative solution, beginners can be guided through the process of refactoring their code to make it more efficient or align with best practices.
- Comparative Analysis: Encouraging beginners to compare their solutions with standard approaches can help them understand the trade-offs between creativity and efficiency.
- Performance Testing: Introducing beginners to performance testing can help them see the practical implications of different approaches to problem-solving.
- Code Reviews: Regular code reviews, either by peers or mentors, can provide valuable feedback on creative solutions while also introducing industry standards.
Let’s look at an example of how a creative beginner’s solution might be refactored to be more efficient while still maintaining its innovative spirit:
# Original creative solution
def find_most_frequent_char(string):
counts = {}
for char in string:
if char in counts:
counts[char] += 1
else:
counts[char] = 1
max_char = ''
max_count = 0
for char, count in counts.items():
if count > max_count:
max_char = char
max_count = count
return max_char
# Refactored solution
from collections import Counter
def find_most_frequent_char_refactored(string):
return Counter(string).most_common(1)[0][0]
# Testing both solutions
test_string = "abracadabra"
print(f"Original: {find_most_frequent_char(test_string)}")
print(f"Refactored: {find_most_frequent_char_refactored(test_string)}")
In this example, the beginner’s creative solution is preserved, but a more efficient method using Python’s Counter class is introduced. This allows the beginner to see how their thought process aligns with established libraries and functions, bridging the gap between creative thinking and practical implementation.
The Long-Term Benefits of the Beginner’s Advantage
As beginners progress in their coding journey, the creative problem-solving skills they develop early on can have lasting benefits throughout their careers. Here are some of the long-term advantages of nurturing the beginner’s mindset:
- Adaptability: Coders who maintain a beginner’s mindset are often more adaptable to new technologies and paradigms.
- Innovation: The habit of approaching problems creatively can lead to innovative solutions in complex projects.
- Debugging Skills: Creative problem-solvers often excel at debugging, as they’re willing to consider unconventional causes for issues.
- Interdisciplinary Application: The ability to think creatively about coding problems can translate to other areas of technology and business.
- Leadership: Developers who can balance creativity with practical knowledge often make excellent team leaders and mentors.
Cultivating Creativity in Experienced Developers
While we’ve focused on the advantages that beginners bring to problem-solving, it’s important to note that experienced developers can also benefit from adopting aspects of the beginner’s mindset. Here are some strategies for seasoned coders to reignite their creative problem-solving skills:
- Regular Skill Stretching: Tackle problems outside your comfort zone or in unfamiliar languages to force fresh thinking.
- Pair Programming with Beginners: Working alongside newcomers can expose you to different ways of approaching problems.
- Embrace Constraints: Set artificial constraints on your coding (e.g., solve a problem without using loops) to encourage creative solutions.
- Cross-Disciplinary Learning: Study fields outside of computer science to gain new perspectives that can be applied to coding.
- Teach Others: Explaining concepts to beginners can help you see familiar topics in a new light.
Conclusion: Harnessing the Power of Beginner Creativity
The beginner’s advantage in creative problem-solving is a powerful asset in the world of coding. By approaching problems without preconceived limitations, new coders often stumble upon innovative solutions that can push the boundaries of what’s possible. As we’ve seen, this creativity can be nurtured and balanced with best practices to produce well-rounded, adaptable, and innovative programmers.
Platforms like AlgoCademy recognize the value of this beginner’s mindset and incorporate it into their teaching methodologies. By encouraging exploration, valuing creativity, and leveraging AI to provide personalized guidance, these platforms are helping to shape the next generation of creative problem-solvers in the tech industry.
Whether you’re a beginner just starting your coding journey or an experienced developer looking to reignite your creative spark, remember that there’s power in approaching problems with fresh eyes and an open mind. Embrace the beginner’s advantage, and you might just find yourself solving problems in ways you never thought possible.
As we continue to face increasingly complex challenges in technology and beyond, the ability to think creatively and approach problems from new angles will be more valuable than ever. So, whether you’re writing your first lines of code or architecting complex systems, never lose touch with your inner beginner. It might just be your greatest asset in the ever-evolving world of programming.