How to Ask the Right ‘What If?’ Questions When Faced With a New Problem
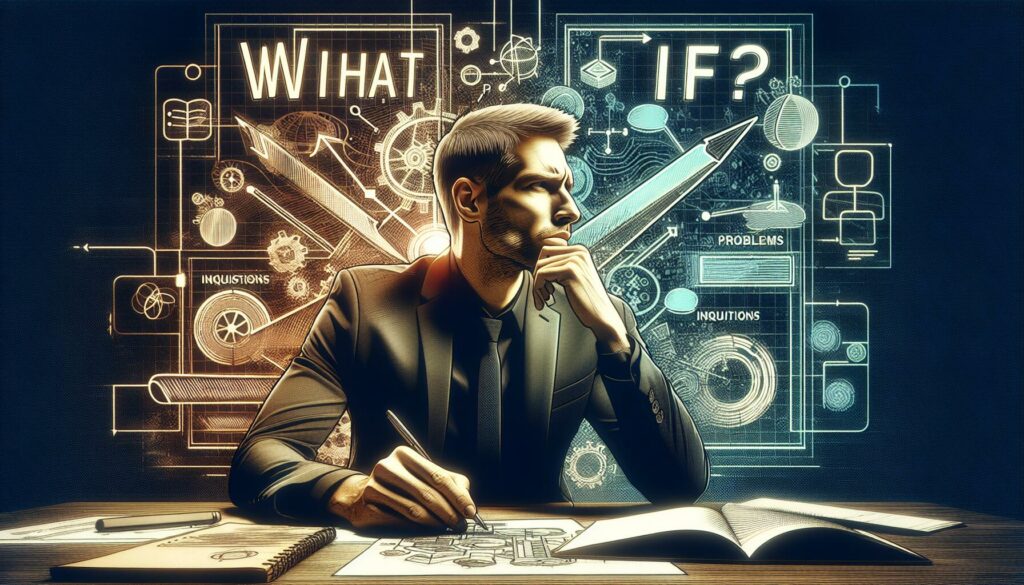
In the world of coding and problem-solving, asking the right questions can be just as important as finding the right answers. When faced with a new problem, especially in the context of algorithmic challenges or technical interviews, the ability to ask insightful “What if?” questions can be a game-changer. This skill is not only crucial for software developers but also for anyone looking to enhance their critical thinking and problem-solving abilities. In this comprehensive guide, we’ll explore how to master the art of asking “What if?” questions, particularly in the realm of coding education and programming skills development.
Understanding the Power of “What If?” Questions
Before diving into the specifics of how to ask these questions, it’s essential to understand why they’re so powerful. “What if?” questions serve several crucial purposes:
- They help explore edge cases and potential scenarios
- They encourage creative thinking and out-of-the-box solutions
- They assist in identifying potential pitfalls or limitations in proposed solutions
- They foster a deeper understanding of the problem at hand
- They can lead to more robust and comprehensive solutions
In the context of coding and algorithmic problem-solving, these questions can be the difference between a solution that works in theory and one that stands up to real-world applications and edge cases.
The Framework for Asking Effective “What If?” Questions
To ask the right “What if?” questions, it’s helpful to have a framework. Here’s a step-by-step approach you can use when faced with a new problem:
1. Understand the Problem Thoroughly
Before you can start asking “What if?” questions, you need to have a solid grasp of the problem at hand. This involves:
- Reading the problem statement carefully
- Identifying the inputs and expected outputs
- Clarifying any ambiguities in the problem description
- Considering the constraints and limitations mentioned
2. Start with the Basic Scenarios
Begin by asking “What if?” questions about the most straightforward scenarios. For example:
- What if the input is empty?
- What if there’s only one element in the input?
- What if all elements are the same?
3. Explore Edge Cases
Move on to more extreme scenarios that might push the boundaries of your solution:
- What if the input is extremely large?
- What if the input contains negative numbers or zero?
- What if the input is not in the expected format?
4. Consider Performance and Scalability
Think about how your solution might perform under different conditions:
- What if we need to process millions of elements?
- What if memory usage is a constraint?
- What if we need real-time processing?
5. Think About Real-World Applications
Relate the problem to potential real-world scenarios:
- What if this algorithm was used in a high-traffic website?
- What if we needed to handle concurrent users?
- What if the data is constantly changing?
6. Consider Error Handling and Robustness
Ask questions about potential errors and how to handle them:
- What if the input is malformed?
- What if there’s a network failure during processing?
- What if we encounter unexpected data types?
Applying “What If?” Questions in Coding Scenarios
Let’s look at how we can apply this framework to a common coding problem: sorting an array of integers. We’ll use the bubble sort algorithm as an example.
Basic Implementation of Bubble Sort
First, let’s consider a basic implementation of bubble sort in Python:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Example usage
print(bubble_sort([64, 34, 25, 12, 22, 11, 90]))
Now, let’s apply our “What if?” framework to improve this implementation and make it more robust.
1. What if the input array is empty?
Our current implementation doesn’t handle this case explicitly. We can modify it to return an empty array if the input is empty:
def bubble_sort(arr):
if not arr:
return []
# ... rest of the implementation
2. What if the array contains non-integer elements?
We might want to add type checking to ensure all elements are comparable:
def bubble_sort(arr):
if not all(isinstance(x, (int, float)) for x in arr):
raise TypeError("All elements must be numbers")
# ... rest of the implementation
3. What if we need to sort in descending order?
We can add a parameter to allow for both ascending and descending sorts:
def bubble_sort(arr, reverse=False):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if (arr[j] < arr[j+1]) if reverse else (arr[j] > arr[j+1]):
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
4. What if we’re dealing with a very large array?
Bubble sort has a time complexity of O(n^2), which is inefficient for large arrays. We might consider using a more efficient algorithm like quicksort or mergesort for larger inputs:
def sort(arr, reverse=False):
if len(arr) > 1000: # Arbitrary threshold
return sorted(arr, reverse=reverse)
else:
return bubble_sort(arr, reverse)
5. What if we need to sort objects based on a specific attribute?
We can modify our function to accept a key function:
def bubble_sort(arr, key=lambda x: x, reverse=False):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if (key(arr[j]) < key(arr[j+1])) if reverse else (key(arr[j]) > key(arr[j+1])):
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Example usage
students = [
{'name': 'Alice', 'grade': 88},
{'name': 'Bob', 'grade': 73},
{'name': 'Charlie', 'grade': 92}
]
sorted_students = bubble_sort(students, key=lambda x: x['grade'], reverse=True)
The Role of “What If?” Questions in Technical Interviews
When preparing for technical interviews, especially for major tech companies often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google), the ability to ask and answer “What if?” questions is crucial. Interviewers often use these questions to assess a candidate’s problem-solving skills, attention to detail, and ability to think critically about edge cases and potential issues.
Tips for Handling “What If?” Questions in Interviews
- Think out loud: Verbalize your thought process as you consider different scenarios. This gives the interviewer insight into your problem-solving approach.
- Start with simple cases: Begin with straightforward scenarios before moving on to more complex ones.
- Consider constraints: Think about time and space complexity, and how your solution might need to change under different constraints.
- Draw from real-world experience: If possible, relate the problem to real-world scenarios you’ve encountered or might encounter in the job.
- Be proactive: Don’t wait for the interviewer to ask all the “What if?” questions. Show initiative by proposing and considering different scenarios yourself.
Developing Your “What If?” Skills
Like any skill, asking effective “What if?” questions improves with practice. Here are some ways to develop this skill:
1. Practice with Coding Challenges
Platforms like AlgoCademy offer a wide range of coding challenges. As you work through these problems, make it a habit to ask yourself “What if?” questions before submitting your solution. This practice will help you identify edge cases and potential issues more easily.
2. Code Review
If you have the opportunity to participate in code reviews, use this as a chance to practice asking “What if?” questions about others’ code. This not only helps improve the code but also sharpens your ability to think critically about different scenarios.
3. Real-World Projects
Apply this questioning technique to real-world projects you’re working on. Consider how your code might behave under different conditions or with unexpected inputs.
4. Study System Design
System design questions often require considering various scenarios and trade-offs. Studying system design will expose you to complex problems where asking the right questions is crucial.
5. Engage in Discussions
Participate in coding forums or discussion groups. When others present problems or solutions, practice asking “What if?” questions to explore different aspects of their approach.
Conclusion
Mastering the art of asking the right “What if?” questions is a valuable skill that can significantly enhance your problem-solving abilities, especially in the realm of coding and software development. By systematically considering different scenarios, edge cases, and potential issues, you can develop more robust and comprehensive solutions to complex problems.
Remember, the goal isn’t just to find problems but to anticipate challenges and improve your solutions proactively. Whether you’re tackling a coding challenge, preparing for a technical interview, or working on a real-world project, cultivating this skill will serve you well throughout your career in technology.
As you continue to develop your coding skills on platforms like AlgoCademy, make it a habit to incorporate “What if?” thinking into your problem-solving process. With practice, you’ll find that this approach not only leads to better code but also deepens your understanding of the problems you’re solving and the solutions you’re creating.